The "git pickaxe" feature allows you to search for changes in your Git repository by displaying a diff of the specific lines modified in a file, making it particularly useful for tracking down changes related to a particular keyword or phrase.
Here's an example command using the pickaxe:
git log -S'search_term' -- path/to/file
What is Git?
Git is a distributed version control system that allows teams to collaborate on code in an efficient and organized manner. Unlike traditional version control systems that are centralized, Git empowers developers to work locally and manage changes independently before syncing them with a shared repository. This approach not only enhances speed and flexibility but also enables robust collaboration among team members.
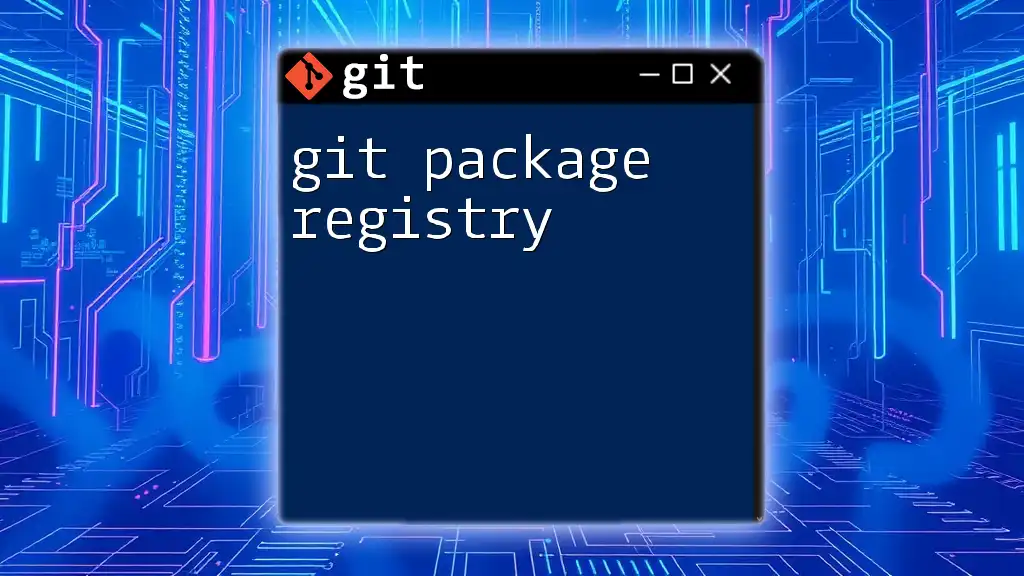
What is a Git Command?
In Git, commands are the fundamental tools for interacting with the repository. They allow users to perform operations such as creating branches, merging changes, and, importantly, searching through the commit history. Commands can be issued via the command line or a Git GUI, enabling a range of functionalities tailored to the developer's needs.
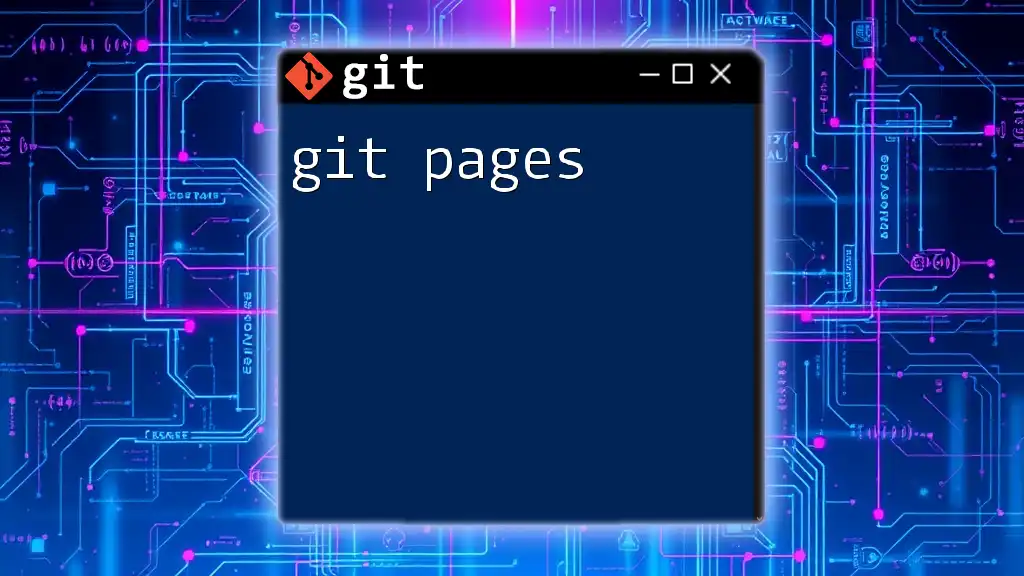
Definition of Git Pickaxe
The Git Pickaxe is a powerful feature that enables users to search for changes in their Git repository’s history, specifically looking for additions or deletions of specified content. The term "pickaxe" reflects its utility in digging through revision history to find exactly what was altered in the codebase. This feature is essential for effective debugging and change tracking.
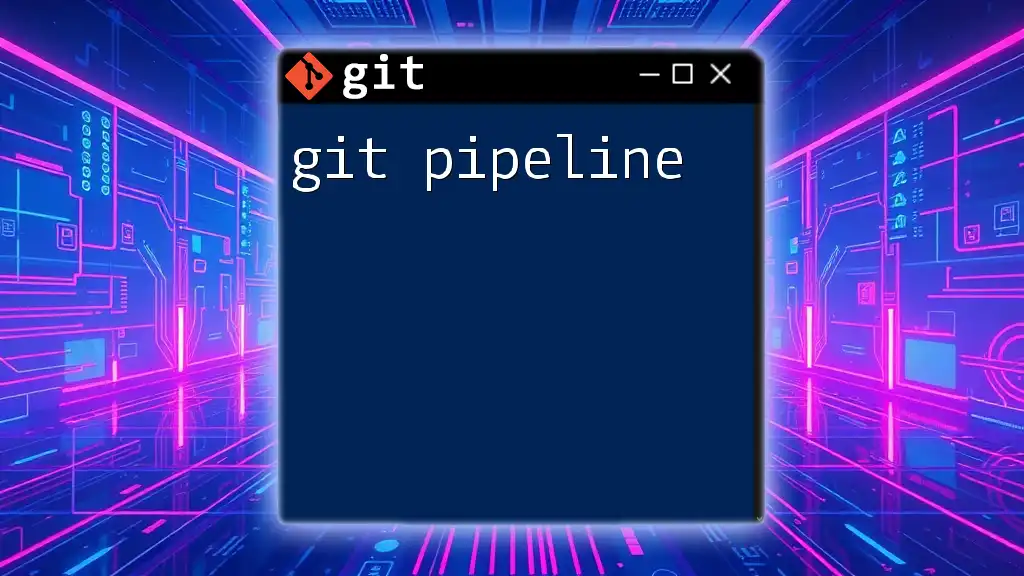
How Git Pickaxe Works
Git Pickaxe allows you to quickly investigate the history of changes associated with a particular string or pattern. At its core, the pickaxe feature uses specific Git commands to locate any commits that introduce or remove a specified string. This functionality is particularly useful when you need to analyze how a particular function or variable has evolved over time, providing insights into potential issues or improvements.
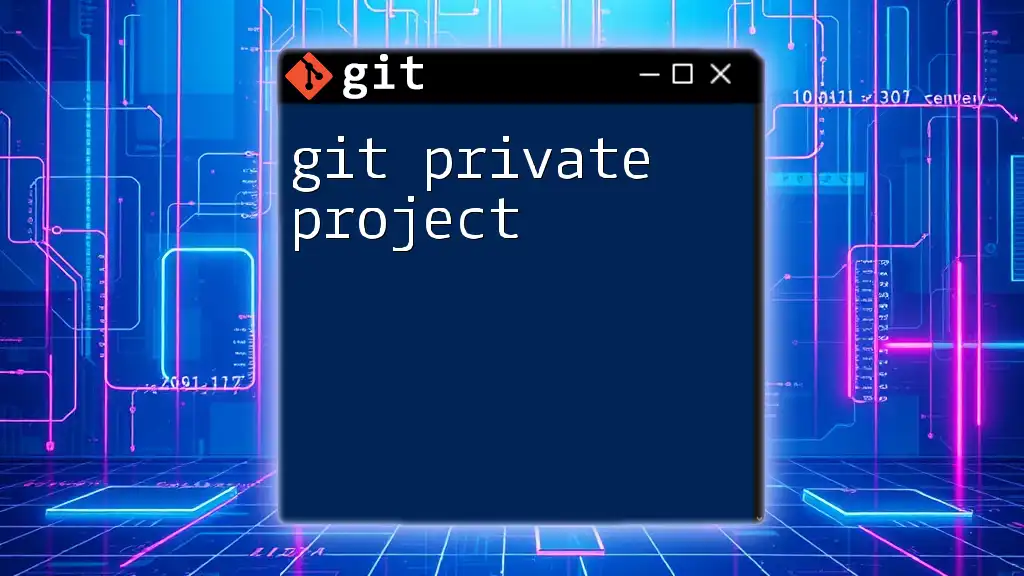
Basic Syntax of Git Pickaxe
The basic syntax for using Git Pickaxe is straightforward:
git log -S'string'
In this command, the `-S` option signifies that you want to search for changes in the addition or deletion of the specified string.
For example, to find all commits that added or removed a function called `functionName`, you would run:
git log -S'functionName'
This command will produce a history of commits that contain changes to `functionName`, allowing you to trace its evolution through your codebase.
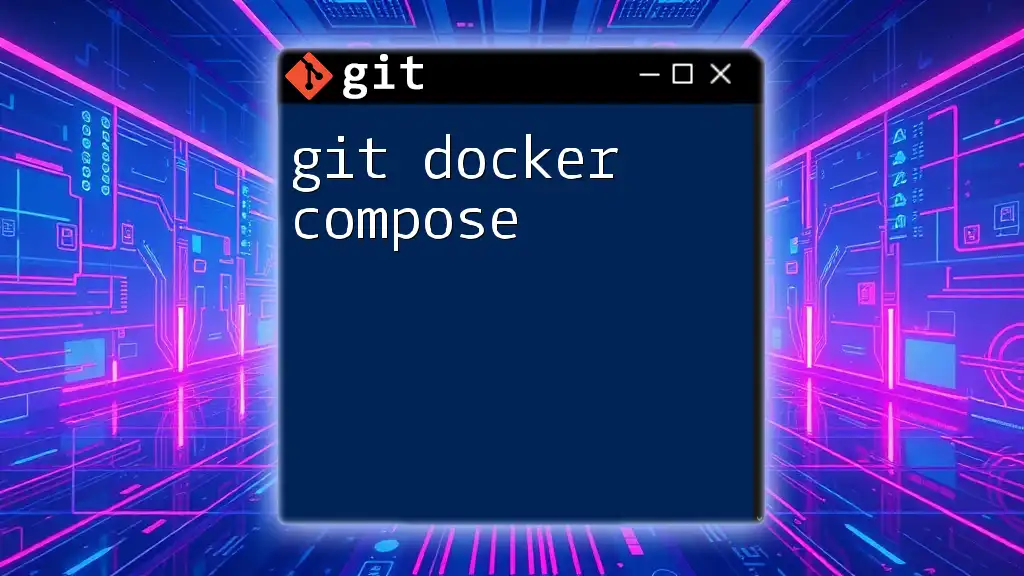
Searching for Added or Removed Lines
When looking to see if a piece of code has been introduced or eliminated, the `-G` option complements the pickaxe feature beautifully. While `-S` focuses on specific strings, `-G` searches for changes matching a regular expression.
git log -G'pattern'
This command lists all commits where lines added or removed match a defined pattern.
It’s important to contrast these two options:
- `-S`: Use when you’re searching for a specific string being added or removed.
- `-G`: Use for more complex patterns that may have varying forms (e.g., variable names that might change).
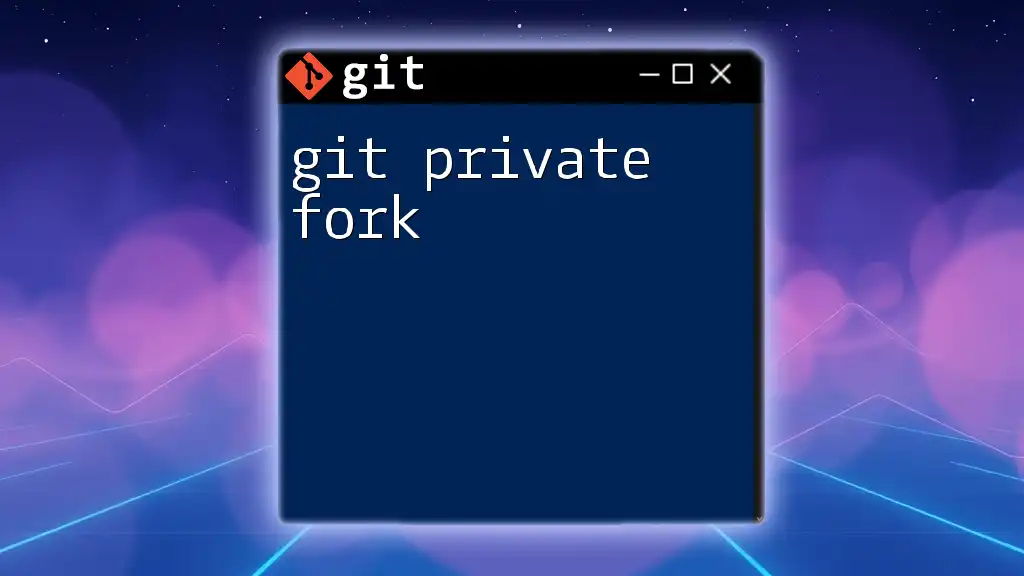
Finding Changes in a Specific File
When you want to narrow your search to a specific file, you can easily add a path to your command. This can drastically reduce the noise from unrelated commits:
git log -S'functionName' -- path/to/file
This command allows you to focus solely on changes made to `functionName` within `path/to/file`, giving you a clear view of relevant modifications.
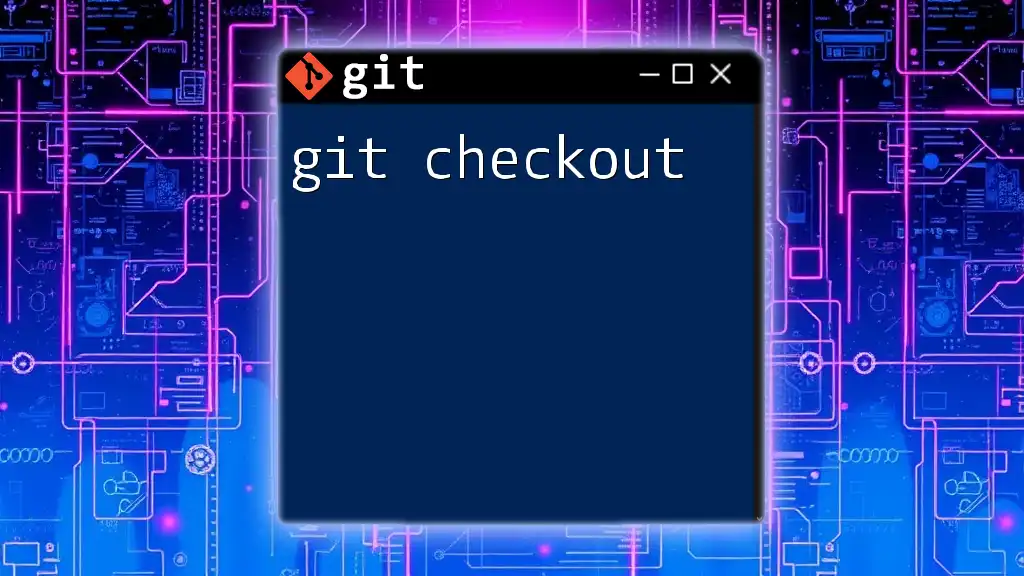
Combining Git Pickaxe with Other Options
To refine your results further, you can combine the pickaxe commands with other Git log options. For instance, if you want to include the differences in code (`-p` option) along with your search, you can execute:
git log -p -S'functionName'
This adds an explanation of changes in the output, allowing you to see what specific modifications were made to `functionName`.
If you'd like a summary of changes rather than a detailed diff, you may use:
git log --stat -S'pattern'
Both commands illustrate the versatility of the Git Pickaxe in combination with other options, significantly enhancing your search capability.
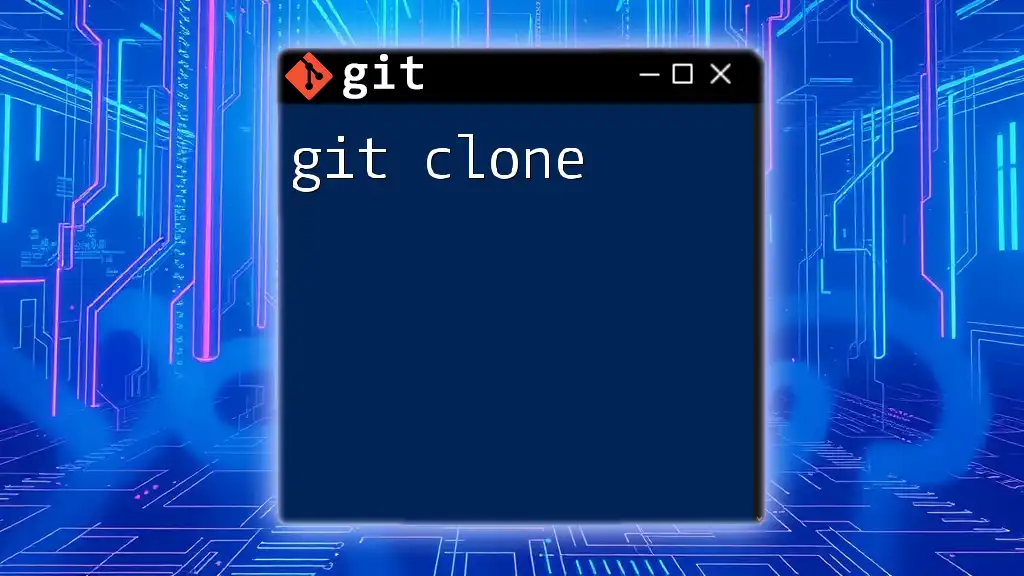
Coloring Output for Better Visibility
Clarity in your command-line output can be crucial when dealing with extensive commit histories. To help distinguish hits from the noise, consider configuring color coding in your `.gitconfig` file:
[color]
ui = auto
[log]
decorate = full
By doing so, commits that match your pickaxe search can be emphasized, making it easier to parse through the output quickly.
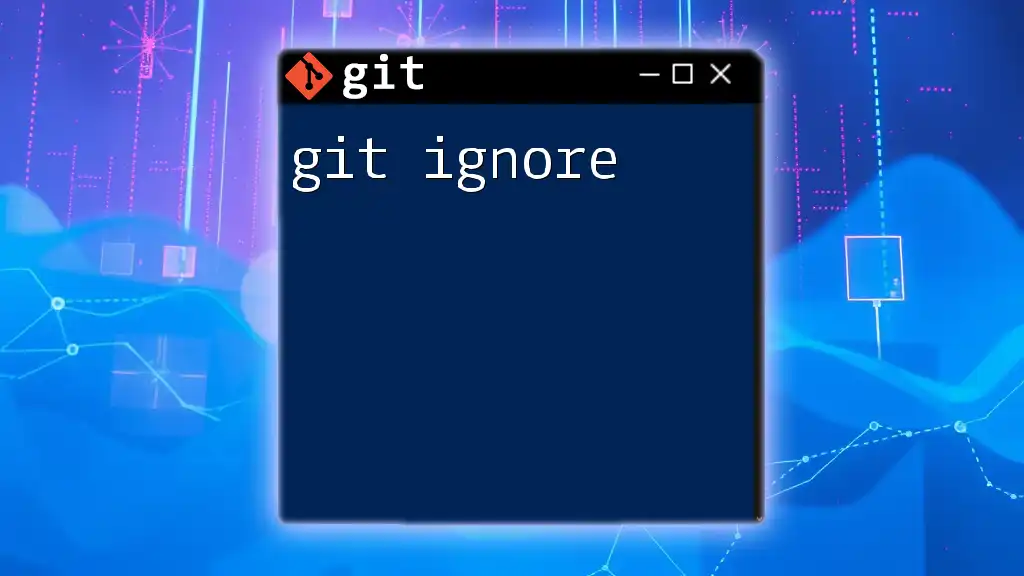
Practical Examples
Example Scenario: Debugging a Commit
Imagine you've identified a bug in your application but aren't sure when an erroneous change was introduced. Using Git Pickaxe facilitates the process. Assuming you're searching for a problematic function called `calculateTotal`, you would run:
git log -S'calculateTotal'
Examine the output, which will provide you with the list of commits that have affected `calculateTotal`. From there, you can check the specific changes in each commit, allowing you to pinpoint when the bug was introduced.
Example Scenario: Refactoring Code
Refactoring is common when evolving a codebase, and the Git Pickaxe can assist in tracking down functions that were adjusted. Suppose you’re interested in how a function `updateRecord` has changed over time:
git log -S'updateRecord'
You can follow the history of that function throughout the development process, gleaning insights that can guide your approach as you refactor surrounding code.
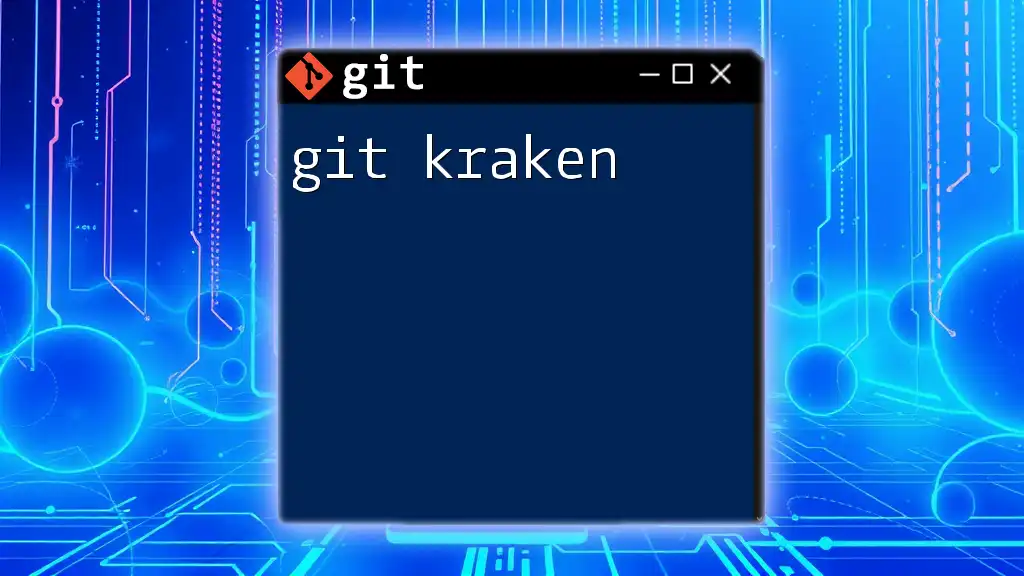
Tips for Effective Use of Git Pickaxe
Best Practices
- Use specific terms: The more accurate your search terms are, the easier it will be to locate relevant commits.
- Limit searches to files as necessary: Especially in larger repositories, narrowing down your search scope can significantly enhance efficiency.
- Combine with visual tools: Consider using GUI tools that represent Git visually for a more intuitive analysis of changes.
Common Mistakes to Avoid
- Overlooking the context of your search—it’s easy to stray from your focus when handling broad terms.
- Ignoring the power of the `-G` option, which may uncover changes that `-S` would miss.
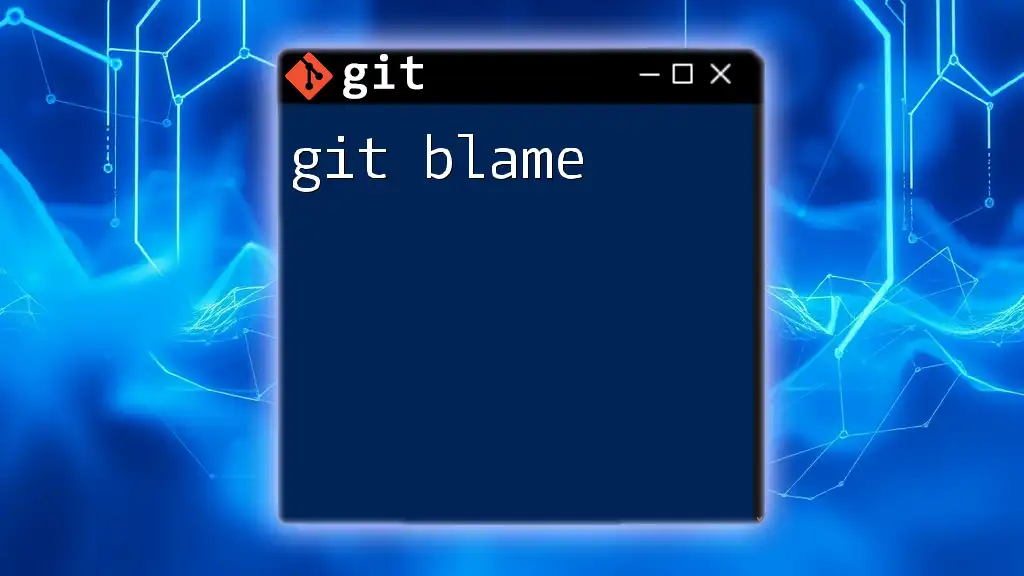
Recap of Git Pickaxe Importance
In summary, the Git Pickaxe is a critical tool for developers, enabling them to navigate through complex code histories efficiently. Whether you are debugging or performing a code review, mastering the pickaxe feature will enrich your Git skills and enhance your overall productivity.
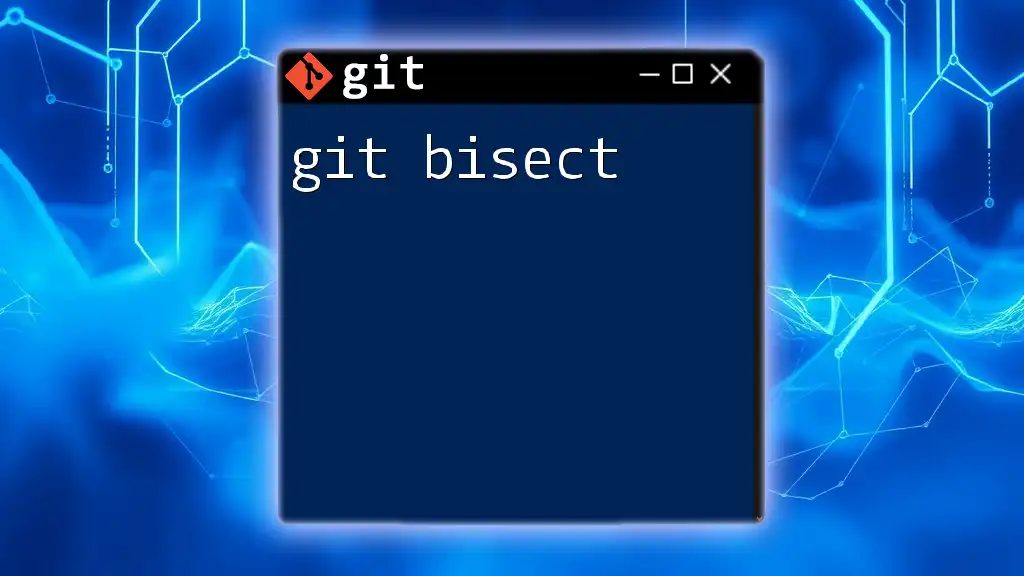
Additional Resources
Further Reading
- Official Git Documentation: Explore the [Git documentation](https://git-scm.com/doc) for comprehensive insights into advanced options and ergonomics for Git commands.
- Tutorials: Look for interactive Git tutorials online that focus on command usage and best practices.
Community and Support
Join forums like Stack Overflow and GitHub discussions to share experiences and learn from the community. By engaging with others, you can gain valuable knowledge and tips regarding Git commands and productivity techniques. Consider participating in Git workshops or meet-ups for hands-on learning opportunities.