Git is often used in conjunction with Docker Compose to manage version control for application configurations encapsulated in containers, allowing teams to efficiently collaborate on deployment setups. Here's a code snippet that demonstrates how to set up a basic Docker Compose file and commit it with Git:
# Create a basic Docker Compose file and commit changes
echo "version: '3'\nservices:\n app:\n image: myapp:latest" > docker-compose.yml
git add docker-compose.yml
git commit -m "Add initial Docker Compose configuration"
Understanding Git and Docker Compose
What is Git?
Git is a version control system that allows developers to track and manage changes to files within a project. Unlike traditional version control systems, Git offers a distributed architecture, allowing every user to have a complete local repository. This means users can work off their own copies of the repository, making it easier to experiment and branch out while retaining a history of all changes made.
Key features of Git include:
- Branching and Merging: Developers can create branches to work on features or fixes without affecting the main codebase. Once completed, changes can be merged back.
- Distributed Architecture: Each clone of the repository is a complete version of the project, making it resilient to data loss and allowing users to work offline.
- Staging Area: It allows developers to prepare changes before committing them with context and clarity.
These capabilities make Git an essential tool in modern software development.
What is Docker Compose?
Docker Compose is a tool that simplifies working with multi-container Docker applications. You define services, networks, and volumes in a `docker-compose.yml` file, allowing you to manage complex applications with a single command. This streamlines the process of starting and stopping containers while maintaining a clear configuration.
Key features and benefits of Docker Compose include:
- Multi-Container Management: Efficiently run multiple interdependent containers as a single service.
- Configuration as Code: Use a simple YAML configuration file for reproducibility and versioning.
- Environment Configuration: Easily configure different settings for various environments, such as development, testing, and production.
Docker Compose is invaluable for developers looking to simplify their local development environments while ensuring reproducibility.
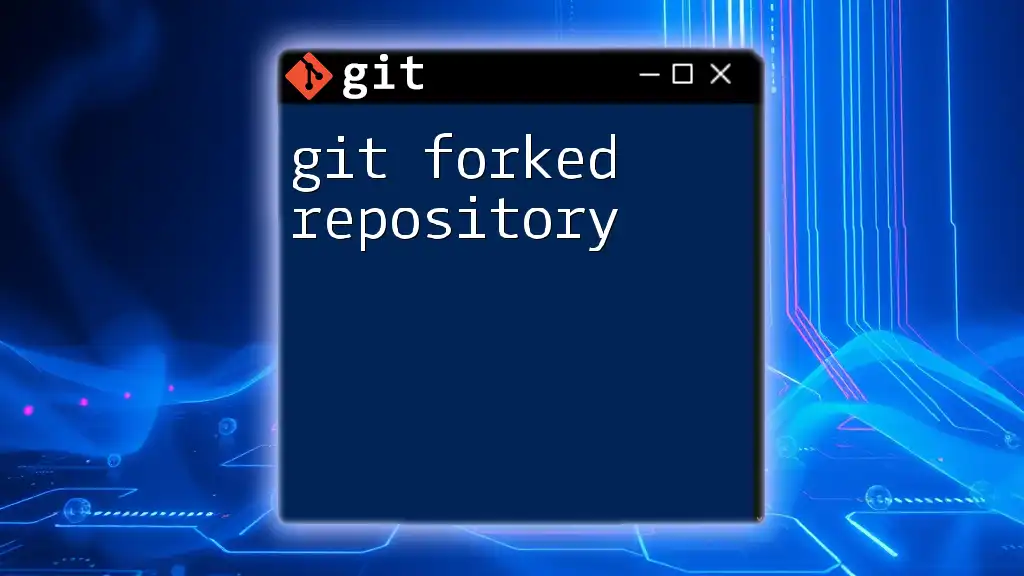
Setting Up Your Environment
Prerequisites
Before diving into Git Docker Compose, ensure you have Git and Docker installed on your machine.
- Git Installation: Follow the official [Git installation guide](https://git-scm.com/book/en/v2/Getting-Started-Installing-Git).
- Docker and Docker Compose Installation: For instructions, refer to [Docker's installation page](https://docs.docker.com/get-docker/) and ensure Docker Compose is included.
Initializing a New Git Repository
Once your environment is set up, you can initialize a new Git repository for your project.
Creating a New Directory
First, create a new directory for your project:
mkdir my-git-docker-project
cd my-git-docker-project
Adding Initial Files
It's essential to include a `.gitignore` file to specify which files and directories to exclude from version tracking. For instance:
node_modules/
*.log
This ensures that unnecessary files do not clutter your repository, keeping the version history clean.
Creating a Simple Docker Compose File
Next, let’s create a basic `docker-compose.yml` file. This file outlines the services, networks, and volumes your application will use.
A simple example might look like this:
version: '3.8'
services:
app:
image: node:14
working_dir: /usr/src/app
volumes:
- .:/usr/src/app
ports:
- '3000:3000'
In this example, we define a service called `app`, using a Node.js image, and exposing port 3000.
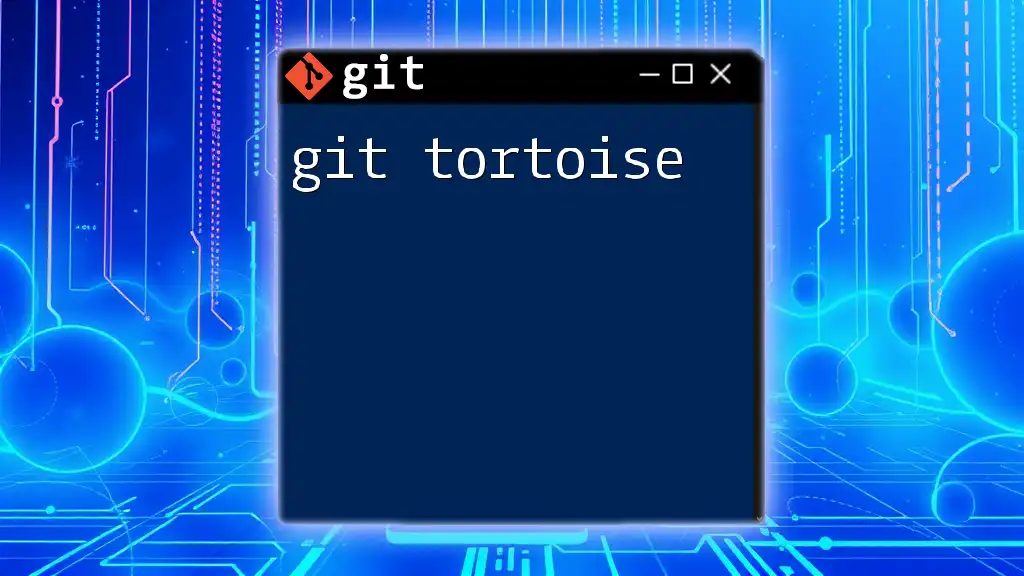
Utilizing Git with Docker Compose
Version Control for Docker Compose Files
It’s essential to track your `docker-compose.yml` file in Git. This allows you to maintain a history of changes and collaborate effectively.
When you make changes to your `docker-compose.yml`, add it to the staging area:
git add docker-compose.yml
git commit -m "Add initial docker-compose setup"
This practice ensures that you can revert to previous configurations and understand how your application’s architecture evolves over time.
Common Git Workflows with Docker Compose
Collaborative Development
When collaborating with others, you will likely clone an existing repository that utilizes Docker Compose.
git clone <repository-url>
cd my-git-docker-project
After cloning, to start your application, you would run:
docker-compose up
This command starts the containers defined in your `docker-compose.yml`, allowing you to see the application’s functionality immediately.
Branching Strategies
Using branches in Git allows you to work on new features without disrupting the main codebase. To create a feature branch:
git checkout -b new-feature
Make the necessary changes to your `docker-compose.yml` or application code, and periodically commit your progress with clear messages.
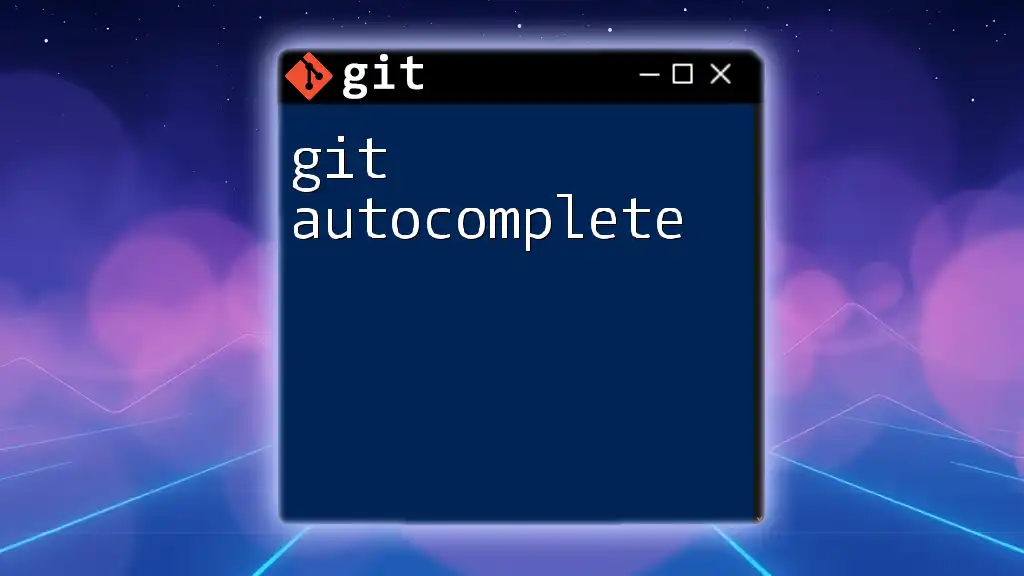
Managing Docker Compose with Git
Tracking and Committing Changes
As you continue developing, keep tracks of changes. If you modify service definitions in your `docker-compose.yml`, remember to stage and commit:
git add .
git commit -m "Update app service definition in Docker Compose"
This habit not only maintains clarity but also allows team members to sync easily with your updates.
Merging Changes
When you're satisfied with your changes, you'll want to merge them back into the main branch. It’s essential to handle conflicts thoughtfully, especially in `docker-compose.yml`, where changes in services might overlap with those from other collaborators.
Tips for Debugging Docker Compose Issues
If you encounter problems starting your services, utilize Docker logs for troubleshooting:
docker-compose logs
Inspect the output to determine any issues, whether they stem from port conflicts, misconfigured paths, or environment variables.
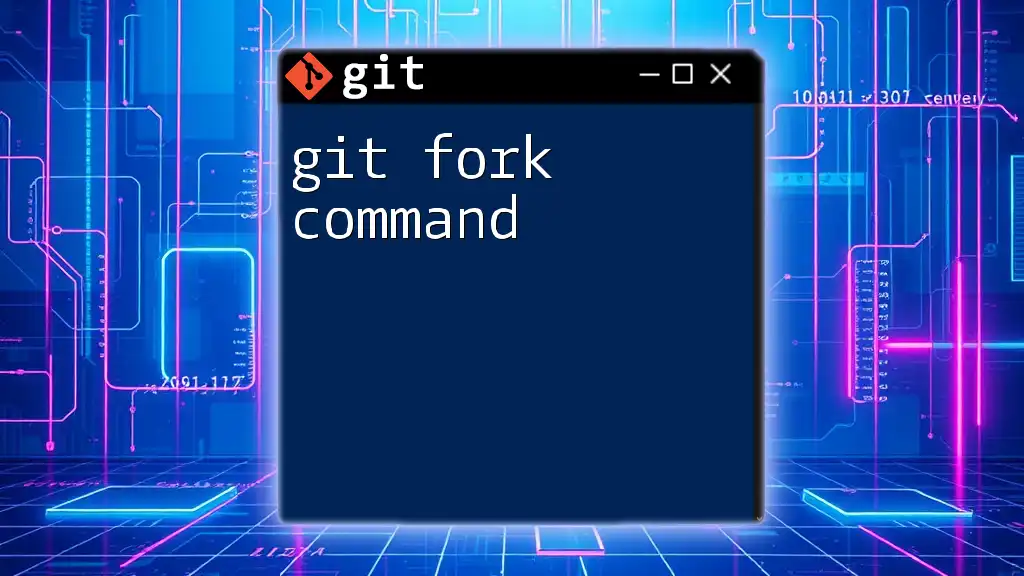
Best Practices for Using Git with Docker Compose
Maintain Readable Docker Compose Files
To foster collaboration and improve maintainability, ensure your `docker-compose.yml` file remains clear and concise. Adhere to code style guidelines, and consider adding comments where necessary to explain complex configurations.
Regularly Commit Changes
Frequent commits are advantageous, providing clarity on changes implemented and enabling easy rollbacks in case of issues. Aim for meaningful commit messages that reflect the purpose of the changes.
Use Tags for Stable Releases
Tagging releases in Git provides a straightforward way to mark specific points in your history, such as stable releases or version changes.
To tag a release, you can use the following command:
git tag -a v1.0 -m "Initial stable release"
This practice allows users to identify stable versions quickly and revert to them if the need arises.
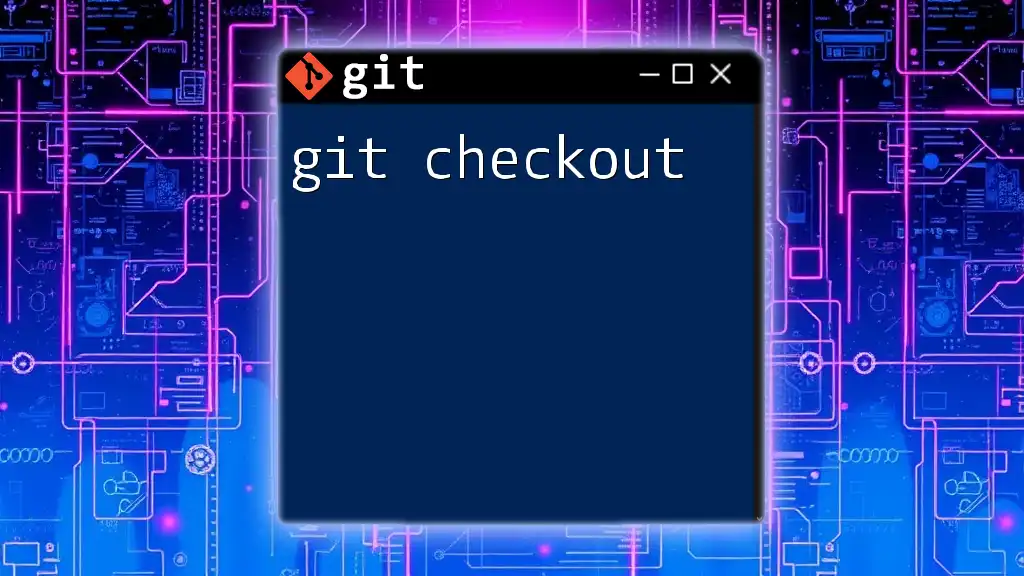
Troubleshooting Common Issues
Common Git Errors with Docker Compose
During your work with Git and Docker Compose, you might encounter some common errors. For instance, merge conflicts may arise when two developers modify the same lines in the `docker-compose.yml` file.
In such cases, carefully inspect the conflicting lines and decide which version to retain or how to merge both changes effectively.
Debugging Docker Compose Issues
When Docker services fail to start, the first step is to check for issues in your configuration or networking setup. Use commands like `docker-compose ps` to inspect the status of your services and troubleshoot accordingly.
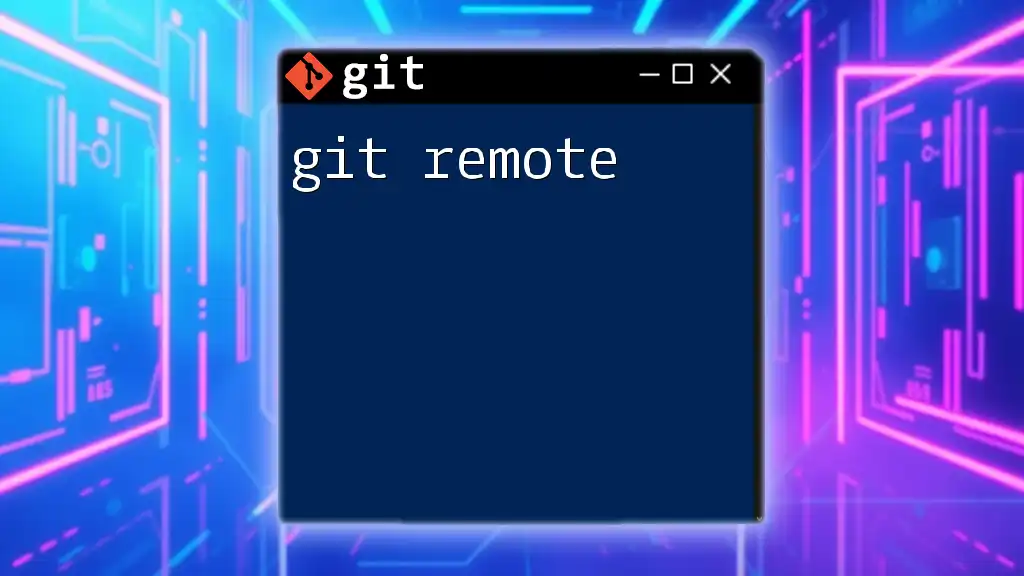
Conclusion
In summary, leveraging Git with Docker Compose is crucial for managing containerized applications effectively. By utilizing version control alongside Docker’s powerful container management capabilities, you can foster collaboration and ensure a clean development workflow. As you begin incorporating these practices into your projects, you will find that your development process becomes much more organized, efficient, and reproducible.
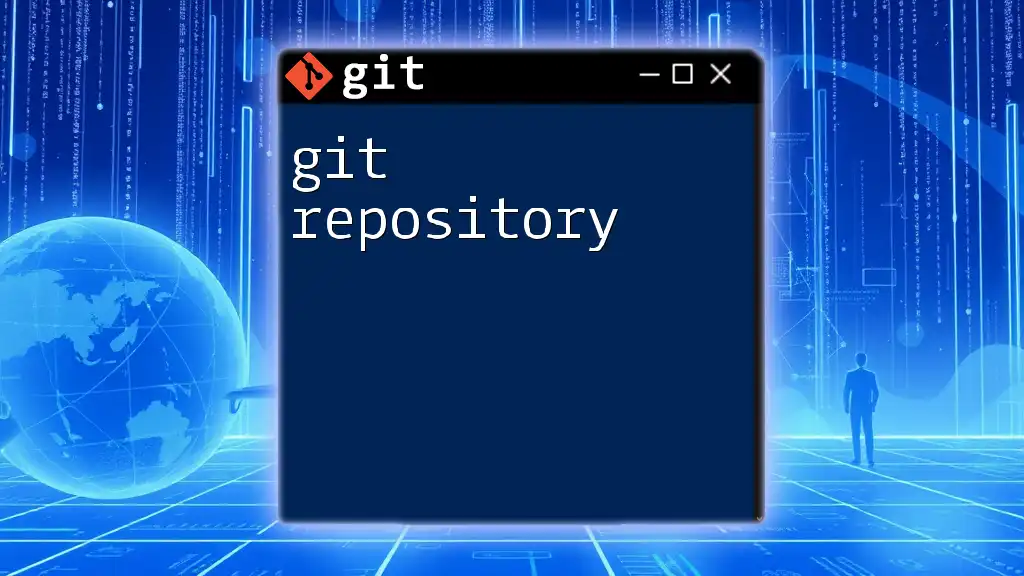
Call to Action
Stay tuned for more insights and tutorials on Git, Docker, and other essential development tools. Join our community to share your experiences, ask questions, and expand your knowledge in these vital areas of modern software development!