A git repository is a storage space where your project files and their entire version history are kept, allowing for efficient collaboration and version control.
Here's an example of how to initialize a new git repository:
git init my-repo
Types of Git Repositories
Local Repository
A local repository is where you can store your work on your machine independently of a remote location. It allows you to track changes, create branches, and manage your code base without needing an internet connection. The highlighted characteristics of a local repository include offline access and the ability to make immediate changes at your discretion.
To create a local repository, you can use the `git init` command, followed by the name of your project. Here’s an example:
git init my-local-repo
This command generates a new directory named `my-local-repo`, establishing a Git repository that is ready to capture all your changes.
Remote Repository
On the other hand, a remote repository exists on a server, which facilitates collaboration among multiple developers. It serves as a centralized space where everyone can contribute and retrieve code. Key benefits of a remote repository include its collaboration features, such as code reviews, and its ability to maintain a history of contributors.
Several popular services host remote repositories, including GitHub, GitLab, and Bitbucket. Each offers unique features like issue tracking and CI/CD integration. Understanding these can help you choose the right tool for your project.
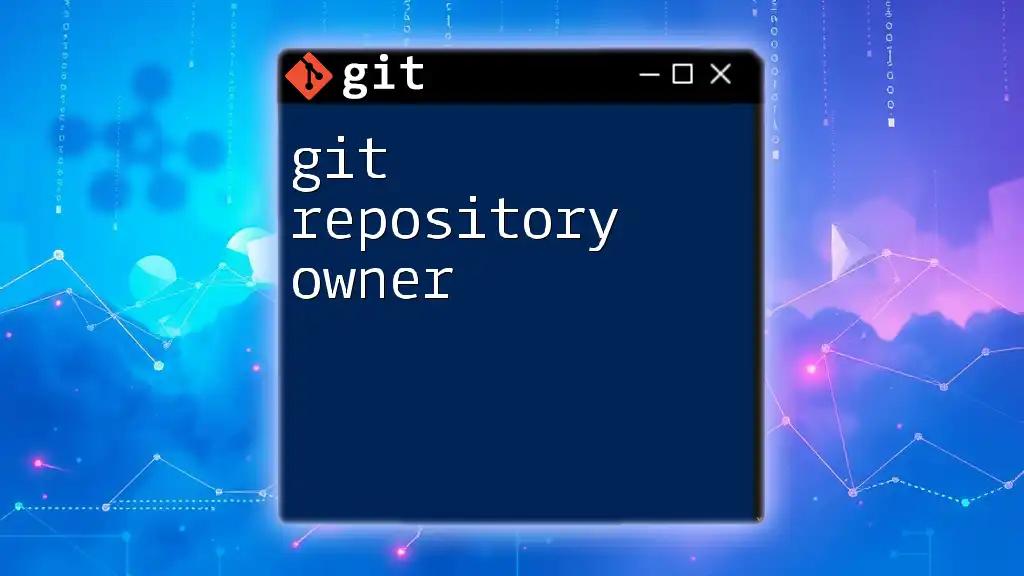
Initializing a Git Repository
Starting a New Project
When embarking on a new project, initializing a Git repository is straightforward. Begin by creating a new directory for your project and navigate into it. Then, run the following commands:
mkdir new-project
cd new-project
git init
This series of commands sets up a new directory called `new-project` and initializes it as a Git repository. You'll notice that a hidden directory named `.git` is created, storing all the version control information.
Cloning an Existing Repository
Cloning an existing repository allows you to create a copy of a remote repository on your local machine. This is particularly useful for collaboration, as it gives you access to the complete codebase and history.
You can clone a repository using the `git clone` command followed by the URL of the repository. For example:
git clone https://github.com/username/repo.git
This command retrieves all files, branches, and commit history into a new directory on your local machine.
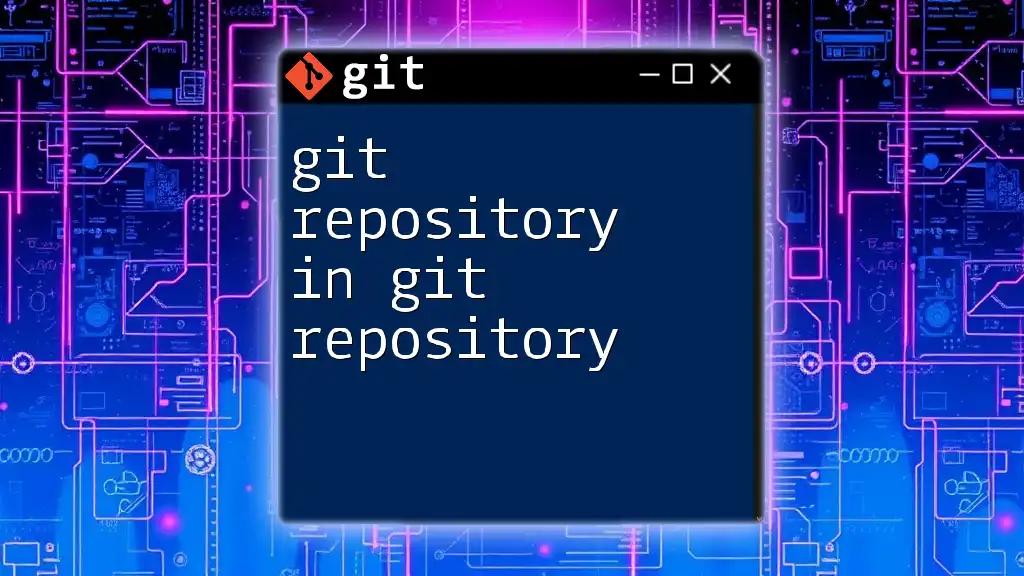
Basic Git Commands for Repository Management
Viewing Repository Status
A fundamental command in Git is `git status`. This command provides insight into the current state of your repository, showing files that are untracked, modified, or staged for commit. This visibility is essential for ensuring your changes are properly prepared before committing.
git status
Adding Files to the Repository
To track changes in your files, you first need to stage them. This process involves adding files to what is called the staging area, which is akin to a preparation area for commits.
You can add files to the staging area using the `git add` command. For instance, to add a specific file, you would use:
git add filename.txt
If you want to stage all modified files at once, you can use a period (`.`) to signify all files in the directory:
git add .
Committing Changes
After staging your files, the next step is to commit your changes. A commit captures a snapshot of your changes and saves them in the project history. It’s essential to provide a meaningful commit message that describes the changes you've made.
You commit changes with the `git commit` command, followed by the `-m` flag to include a message. Here’s an example:
git commit -m "Your meaningful commit message here"
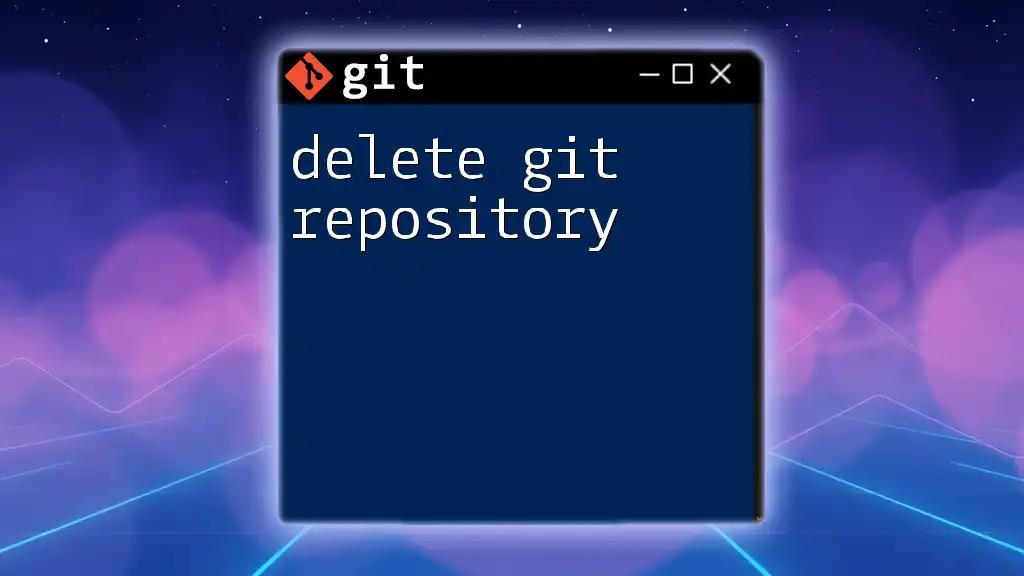
Managing Branches in a Git Repository
Understanding Branches
In Git, a branch is essentially a divergent line of development. Branches allow you to work on features or fixes separately from the main production code base, which is typically called `main` or `master`. Utilizing branches promotes an organized development workflow, minimizing the risks of altering the core code inadvertently.
Creating a New Branch
Creating a new branch can be done with the `git branch` command, which signifies the initiation of a new line of development. Here’s how to create a branch named `new-feature`:
git branch new-feature
This command adds a new branch to your repository without switching to it yet.
Switching Branches
To start working on that new branch, you need to switch your working directory context to it. You use the `git checkout` command:
git checkout new-feature
Alternatively, you can combine the creation and checkout into a single command with:
git checkout -b new-feature
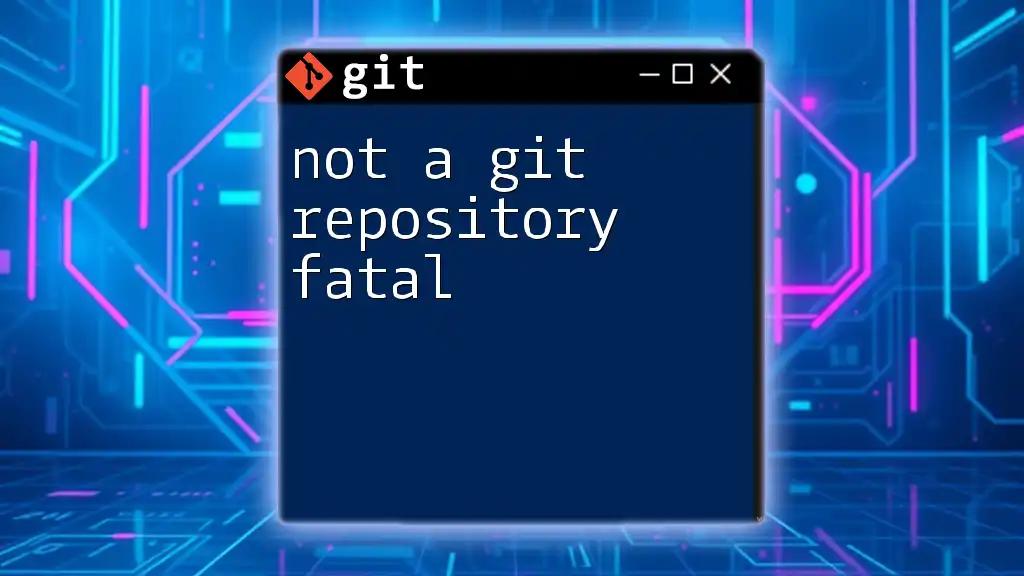
Working with Remote Repositories
Pushing Changes
Once you've made your changes and committed them locally, you can share these changes with others by pushing them to a remote repository. This is accomplished with the `git push` command, setting the target branch on the remote.
Use the following syntax:
git push origin branch-name
`origin` typically refers to the default name for your remote repository but can be customized based on your preferences.
Pulling Updates
To keep your local repository synchronized with the remote counterpart, use the `git pull` command. This command fetches changes from the remote and merges them into your current branch. It’s crucial for avoiding conflicts and ensuring that you are working with the latest version.
For example:
git pull origin main
This fetches and integrates changes from the `main` branch on the remote.
Fetching Changes
Sometimes, you may want to update your local repository without merging changes immediately. This is where `git fetch` comes into play. It retrieves updates without altering your working directory or current branch.
The command looks like this:
git fetch origin
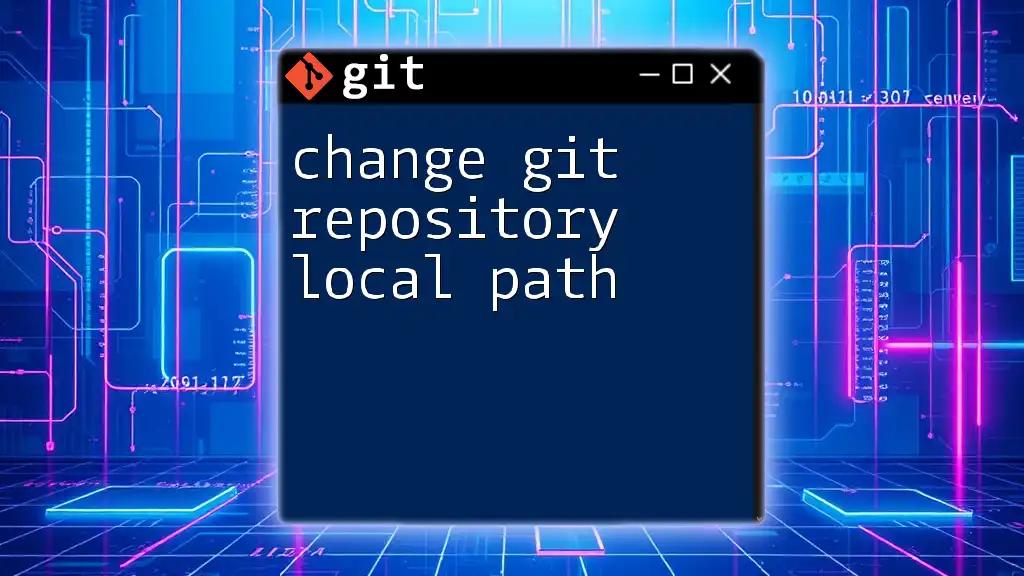
Managing Clone Differences
Merging Changes
When collaborating with others, you may find yourself needing to combine different branches of work. The `git merge` command allows for this. It integrates changes from one branch into another.
For instance, assuming you want to merge the branch `new-feature` into `main`, you would first check out the main branch:
git checkout main
git merge new-feature
Resolving Conflicts
When multiple developers work on the same part of the code, conflicts can occur during merging. A merge conflict arises when changes in two branches are incompatible. In such cases, Git will mark the conflicts directly in the file, requiring you to manually resolve them.
To handle a conflict:
- Open the conflicted file to see both sets of changes.
- Decide how to resolve the conflict, then edit the file accordingly.
- Stage the resolved file using `git add`.
- Finally, complete the merge with `git commit`.

Best Practices for Git Repositories
Commit Message Guidelines
Your commit messages should convey the essence of the changes being made. Good practices for writing commit messages include:
- Using the present tense (e.g., "adds feature" not "added feature").
- Providing context if the change is not self-explanatory.
- Keeping messages concise yet descriptive.
Regular Pushing and Pulling
To maintain synchronization with the remote repository, make it a habit to push frequently after your changes, and regularly pull to integrate others' changes. This proactive approach minimizes the probability of conflicts and ensures a seamless workflow for everyone involved.
Backup and Recovery
Utilize various strategies to protect your work. The `git stash` command can temporarily save changes if you're not ready to commit. You can also regularly push changes to the remote repository for an added layer of backup. Overall, being diligent about committing and pushing changes will safeguard your progress and facilitate recovery from errors.
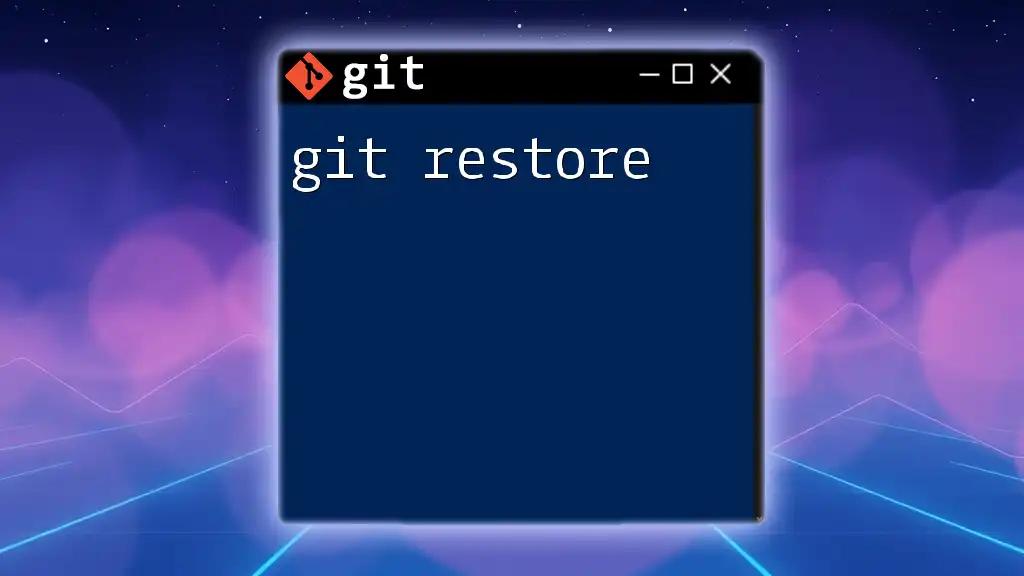
Conclusion
In summary, understanding how to effectively use a git repository is crucial for any developer. It not only streamlines your workflow but also enhances collaboration among teams, ensuring that everyone works with the most up-to-date code. By familiarizing yourself with commands for initializing, managing, and collaborating in Git, you will significantly bolster your development experience.
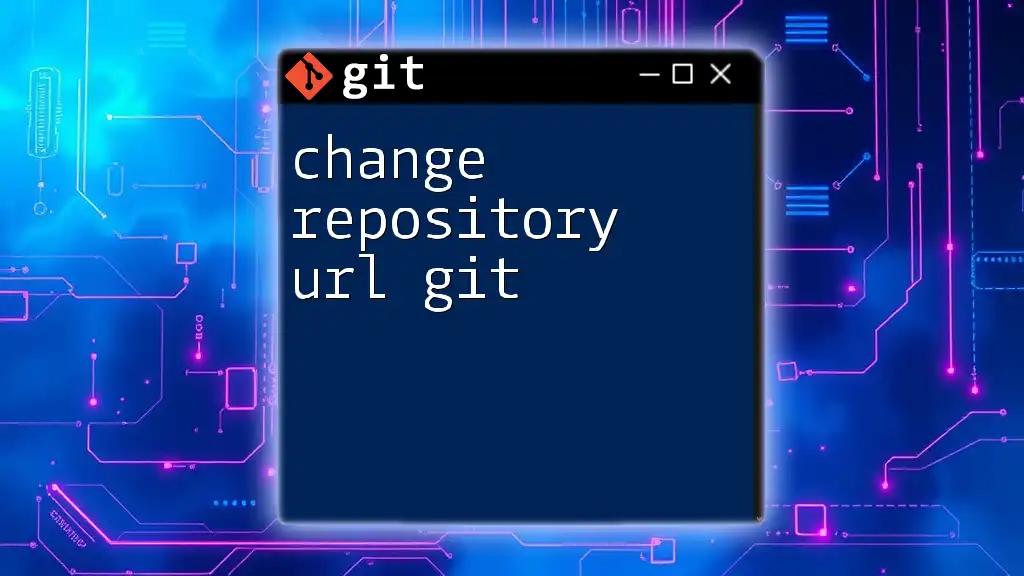
Additional Resources
To continue your journey into mastering Git, consider exploring recommended books and websites dedicated to version control. Engaging with communities can also help deepen your understanding and resolve any queries you may have along the way. Join forums, participate in discussions, and connect with fellow learners to benefit from their experiences.