A "git repository in a git repository," commonly known as a nested or submodule repository, allows you to include one git repository as a subdirectory within another, enabling modular project management.
Here's a code snippet to initialize a submodule:
git submodule add <repository-url> <path>
Understanding Git Repositories
What is a Git Repository?
A Git repository is a structured storage system that holds all the files and their history for a project. It enables users to track changes, collaborate with others, and revert back to previous versions if necessary. The key components of a Git repository include:
- Commits: Snapshots of your project at a specific point in time.
- Branches: Different lines of development, allowing multiple features or fixes to be worked on simultaneously.
- Remotes: Versions of your repository that are hosted on the internet or another network.
Understanding these components is crucial because they form the backbone of how version control systems operate.
Types of Git Repositories
Git repositories can be categorized into two main types: Local and Remote.
- Local Repositories: These reside on your machine and allow you to work offline. Changes made here can be pushed to remote repositories later.
- Remote Repositories: Stored on servers, remote repositories allow you to share your project with collaborators. They serve as a central hub for collaboration and can be hosted on platforms like GitHub, GitLab, or Bitbucket.
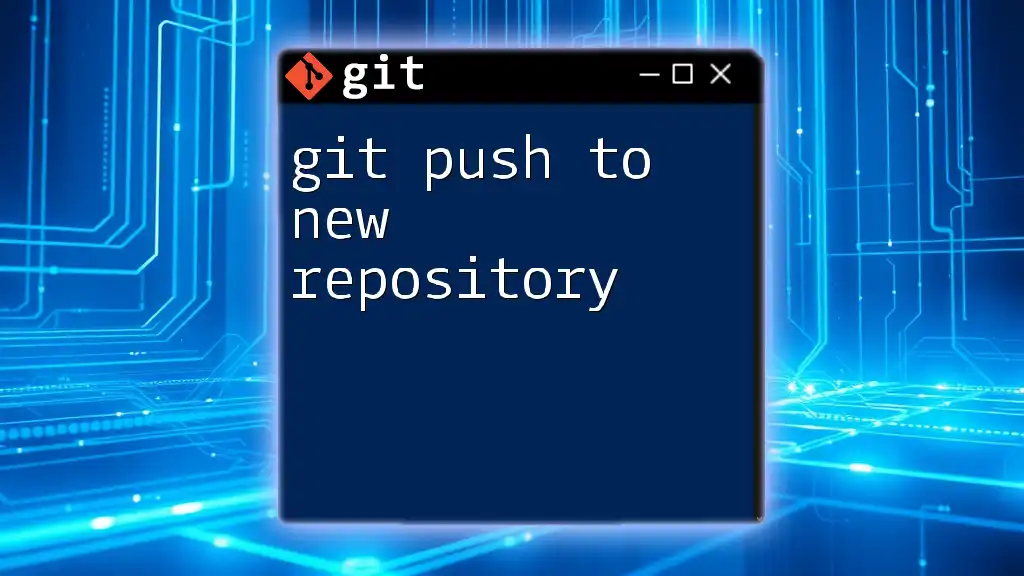
The Concept of Nested Git Repositories
What is a Nested Git Repository?
A nested Git repository is simply a repository located within another repository. This structure can be beneficial in scenarios involving complex projects that require separation of components or when working with various dependencies.
For example, a large application might consist of distinct microservices or libraries, each of which can be housed within its own repository as a form of organization. This approach can streamline management and enhance collaboration in a multi-project environment.
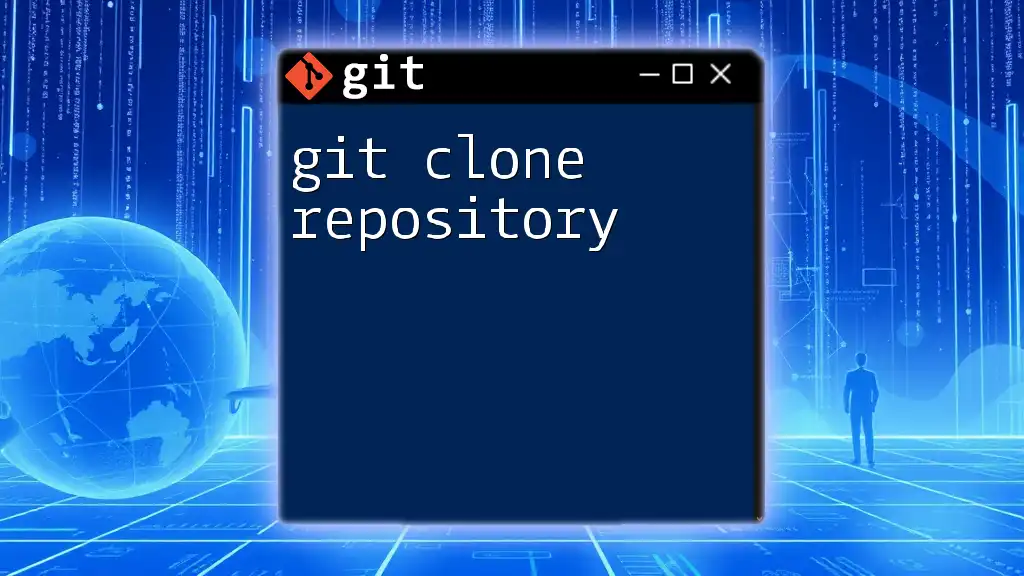
Creating a Nested Repository
Step-by-Step Guide
Initializing a New Git Repository
To begin using Git, you first need to initialize a repository. This can be accomplished with the following commands:
git init my-project
cd my-project
This command creates a new directory called `my-project` and initializes it as a Git repository. You can now start adding files and tracking changes.
Creating a Nested Repository
Once you've established your main repository, you can create a nested one. Navigate to your main project and follow these steps:
mkdir nested-repo
cd nested-repo
git init
Here, you create a directory named `nested-repo` within your main project directory and initialize it as a Git repository. This setup allows you to keep separate version histories for different parts of the same project.
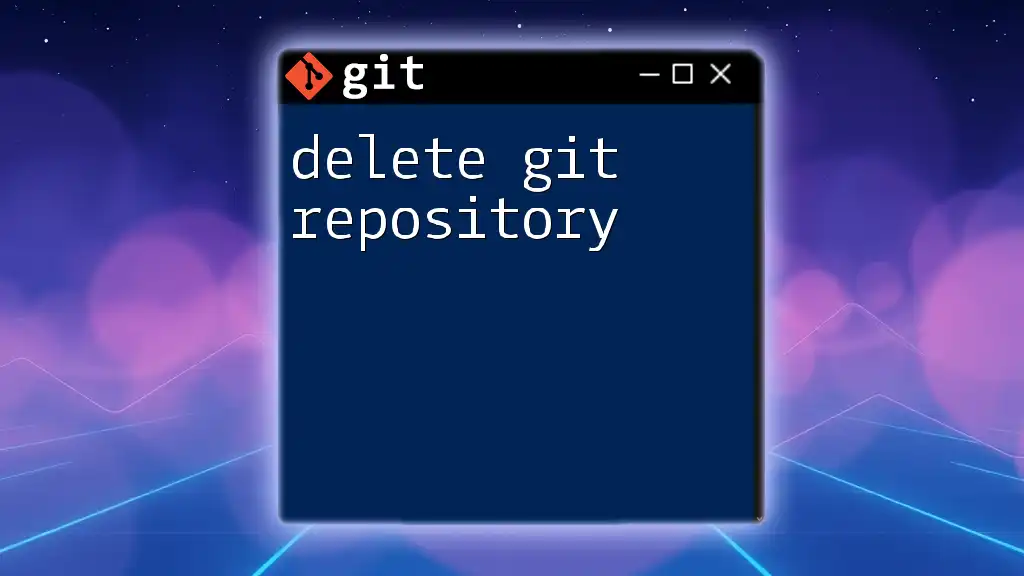
Practical Use Cases for Nested Repositories
Project Organization
Nested repositories are particularly helpful for organizing large projects. For example, if you are developing a web application, you may wish to separate the frontend and backend codebases. Each could have its own repository, providing clarity and reducing the complexity of managing a single repository.
Version Control in Multi-Project Environments
In a typical multi-project setup, where a primary product uses various modules, nested repositories help maintain clear boundaries. For instance, you might have a core framework as a main repository, while different libraries or plugins can be stored in nested repositories. This allows each component to evolve independently while still being part of the bigger picture.
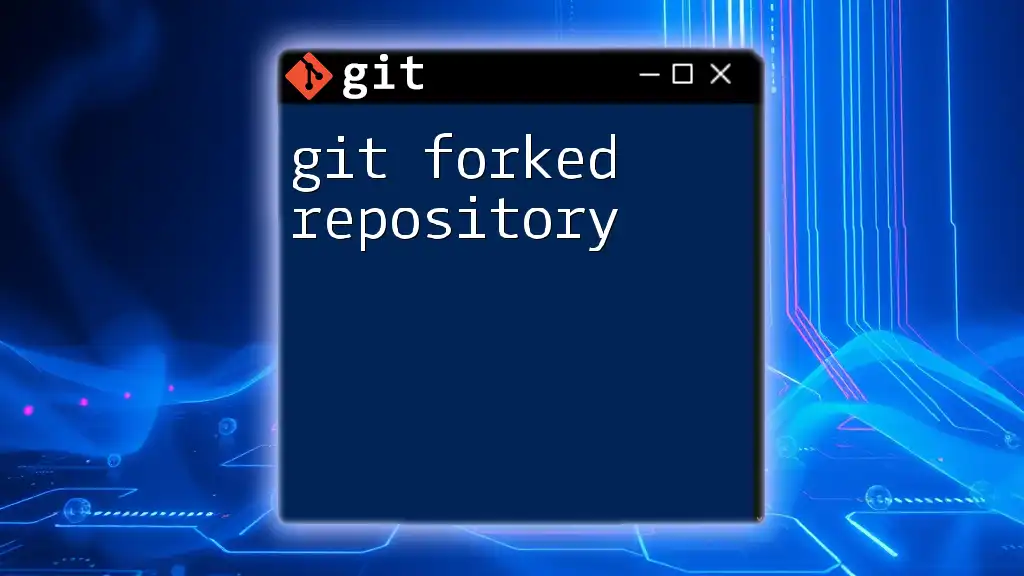
Managing Nested Git Repositories
Cloning a Repository with a Nested Repository
When working with repositories that contain nested ones, cloning them correctly is crucial. You can clone a repository with all its nested repositories by using the following command:
git clone --recursive <repository-url>
By using the `--recursive` flag, Git fetches all submodules and nested repositories, ensuring that your local copy matches the structure of the original repository.
Best Practices for Working with Nested Repositories
To manage nested repositories effectively, consider these best practices:
- Maintain Clear Documentation: Ensure that each repository is well-documented so that team members understand its purpose and how it connects to the main project.
- Use Consistent Naming Conventions: Stick to a uniform naming scheme for directories and repositories to avoid confusion.
- Regularly Sync Changes: Keep a routine for merging changes between the main repository and nested repositories to prevent drift.
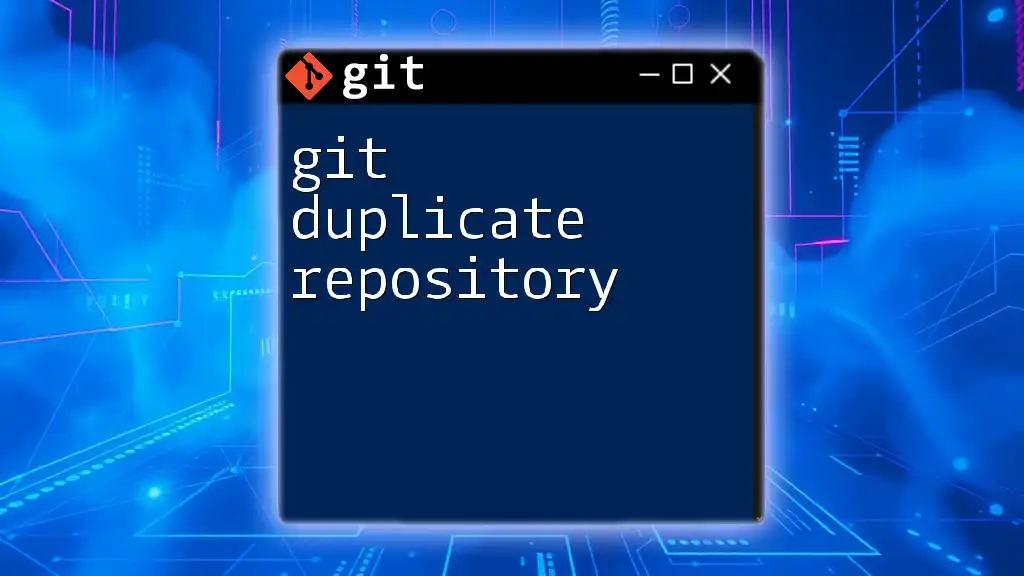
Challenges with Nested Repositories
Common Issues
Despite their advantages, nested repositories come with challenges. Some common issues include:
- Pushing Changes: When working with nested repositories, you may accidentally push changes from a nested repository instead of the main one.
- Merging Conflicts: If multiple contributors are working on interconnected parts of a project, the likelihood of merge conflicts increases.
Solutions and Workarounds
To mitigate these challenges, you can:
- Use Explicit Commands: Always specify the repository you are working in when executing Git commands to avoid unintentional actions.
- Implement Branch Protection Rules: Establishing rules on your main repository can help prevent unauthorized changes from nested repositories.
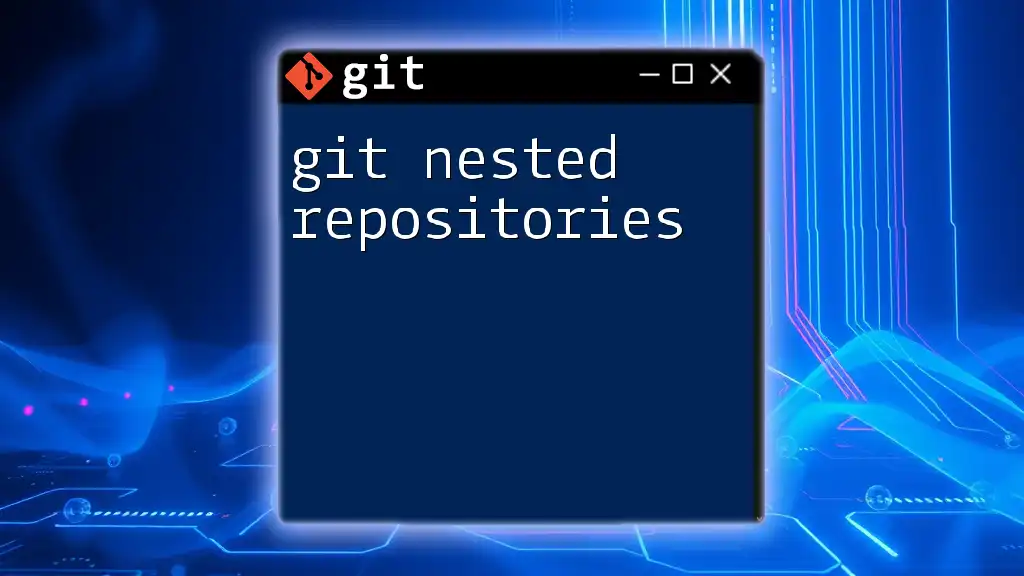
Alternatives to Nested Repositories
Using Git Submodules
Instead of creating a nested repository, consider using Git submodules. Submodules allow you to include one Git repository within another as a sub-directory, facilitating better dependency management. To add a submodule, use the command:
git submodule add <repository-url>
Submodules maintain their own commit history and allow independent version control.
Project Monorepo vs. Nested Repositories
In some situations, a monorepo (a single large repository containing multiple projects) can serve as an effective alternative to nested repositories. The advantages of a monorepo include simplified dependency management and easier collaboration. However, it may also introduce complexity when it comes to project organization.
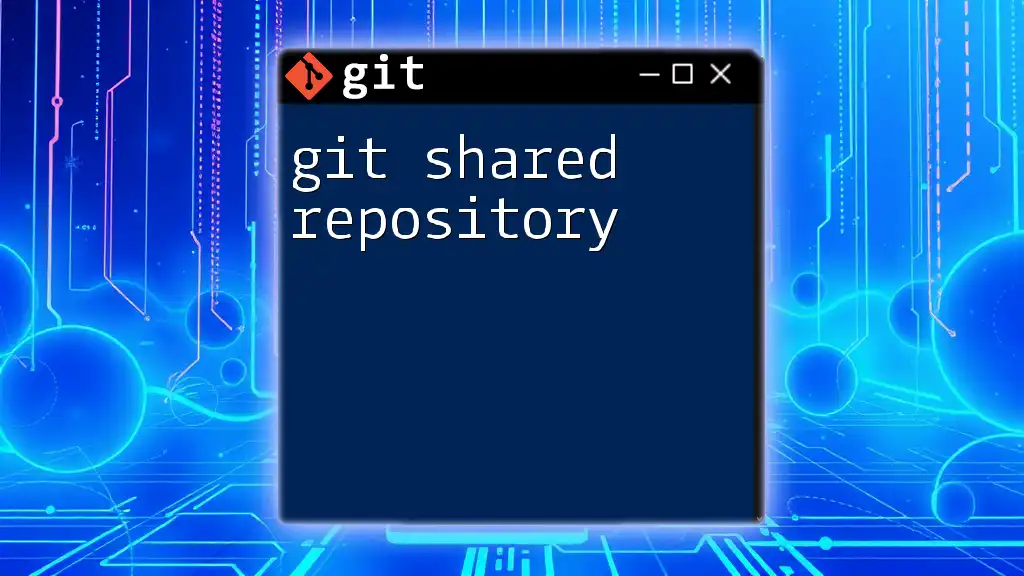
Conclusion
Understanding the concept of a git repository in a git repository is essential for any developer working with projects that require compartmentalization or specialized components. By leveraging nested repositories correctly, you can enhance your project’s organization, support better collaboration in multi-project settings, and maintain clarity in version control.
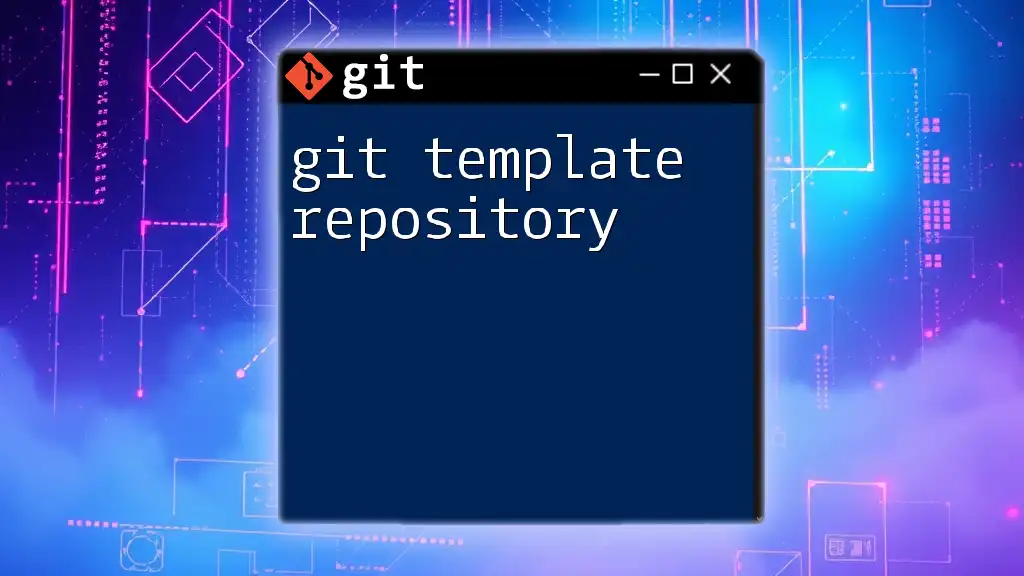
Additional Resources
For further exploration, refer to the official Git documentation and other learning platforms focusing on advanced Git techniques. These resources will deepen your understanding and practical application of nested repositories.
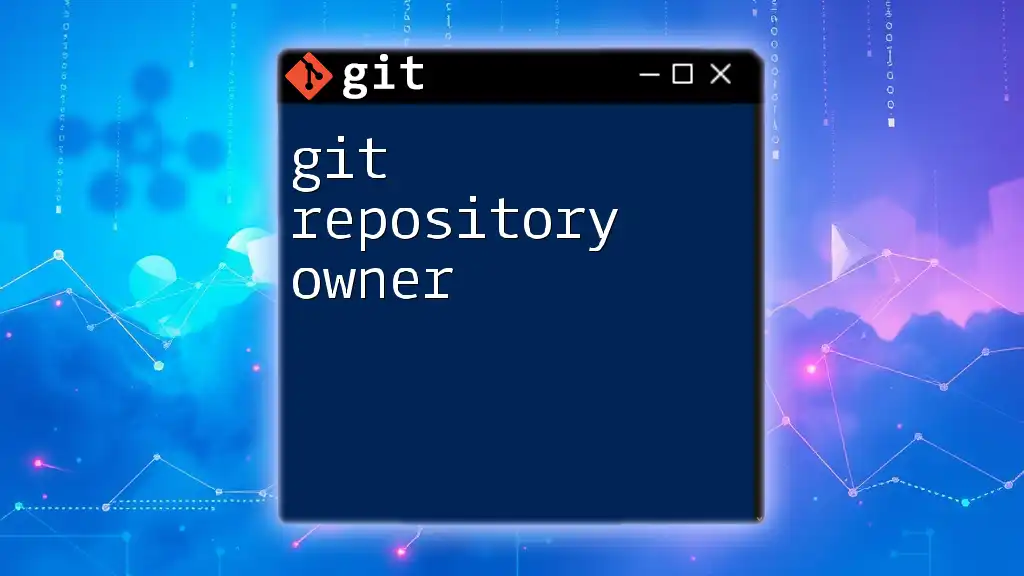
FAQs
What are the risks of having a nested Git repository?
Nested repositories can complicate version control workflows and may lead to confusion regarding commit histories and changes between projects. These risks necessitate careful management and regular synchronization.
Can I track a nested repository with a single parent repository?
Yes, a nested repository can still be tracked as part of a parent repository, but it is essential to manage the commits and changes independently to prevent conflicts.
How do I remove a nested repository?
To remove a nested repository, navigate to the parent repository and delete the folder containing the nested repository, then commit this change to the parent repository to update the history.