To push your local Git repository to a new remote repository, use the following command after initializing the remote URL.
git remote add origin <REMOTE_URL>
git push -u origin master
Setting Up a New Repository
Creating a New Local Repository
To begin using Git, you first need to create a local repository on your machine. This process starts by initializing a new repository. You can do this with the following command:
git init my-repo
This command creates a new directory called `my-repo` and sets up a Git repository inside it. You will now have a hidden folder named `.git`, which contains all the metadata for your Git repository. Understanding the directory structure is key; this `.git` folder manages all your versioning data, such as commits, branches, and history.
Creating a New Remote Repository
Next, you will want to create a remote repository where you can push your code so that it can be accessed later or shared with collaborators. To accomplish this, you need to choose a hosting service. Some popular options include GitHub, GitLab, and Bitbucket.
Once you've chosen your service, the steps to create a new remote repository on GitHub are straightforward:
- Log into your GitHub account.
- Click on the New repository button.
- Fill out the repository name and description.
- Choose whether it should be public or private.
- Click Create repository.
Your new remote repository will be empty and ready to receive content.
Understanding Repository Settings
When you create a repository, it's important to understand the distinction between public and private repositories. Public repositories are visible to everyone, which is great for open-source projects. On the other hand, private repositories restrict access to selected users, making them suitable for sensitive or proprietary projects.
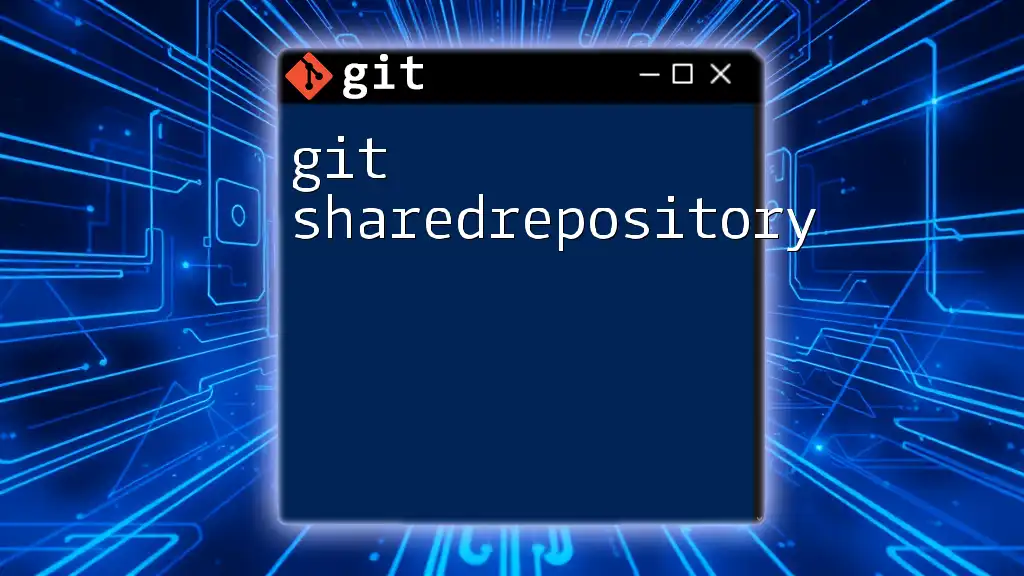
Linking Local Repository to Remote Repository
Adding the Remote Origin
After setting up your remote repository, the next step is to link it to your local repository. This is done by adding a remote called "origin," which is a conventional name for the primary remote repository. You can do this by running the following command:
git remote add origin https://github.com/username/my-repo.git
Verifying the Remote Configuration
To ensure that your local repository is correctly linked to your remote repository, you can check your remote settings with:
git remote -v
The output will display the remote repositories you have configured. Typically, it will show the URL you added under the `origin` label. If it's there, then you've successfully established the connection!
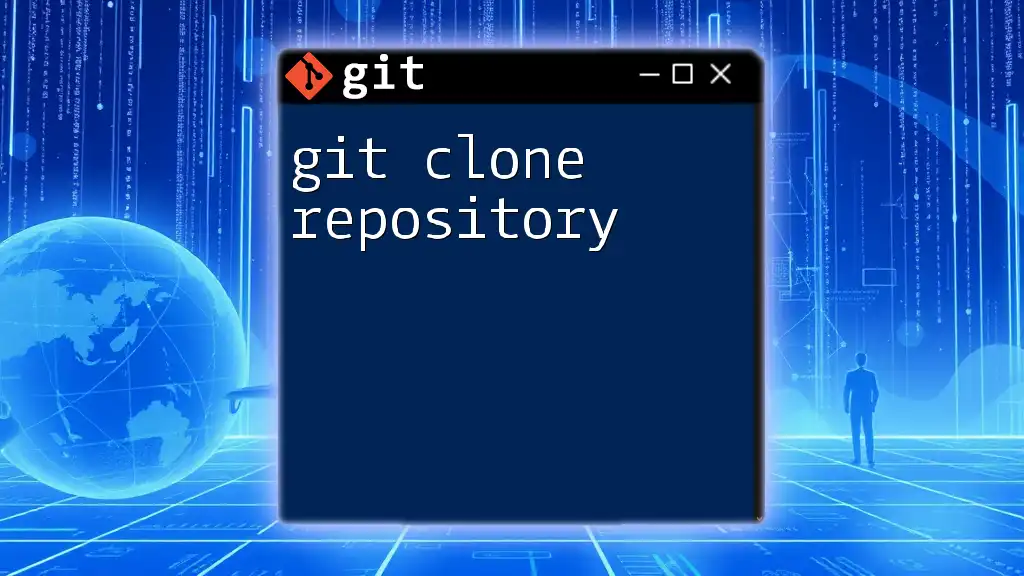
Making Your First Commit
Staging Changes
Before you can push your code, you’ll need to tell Git which changes to track. This is done through the staging area. Think of the staging area as a preparation zone before you make a commitment to save your changes permanently. You can stage all changes using:
git add .
This command stages all modified files for the next commit, allowing you to maintain a clean and organized history.
Committing Your Changes
Once your changes are staged, it’s time to commit them. A commit is a snapshot of your code at a specific point in time. To make your first commit, use the following command:
git commit -m "Initial commit"
Here, the `-m` flag allows you to add a message explaining the purpose of your commit. Writing clear and descriptive commit messages is essential as it helps you and your collaborators understand the history of changes over time.
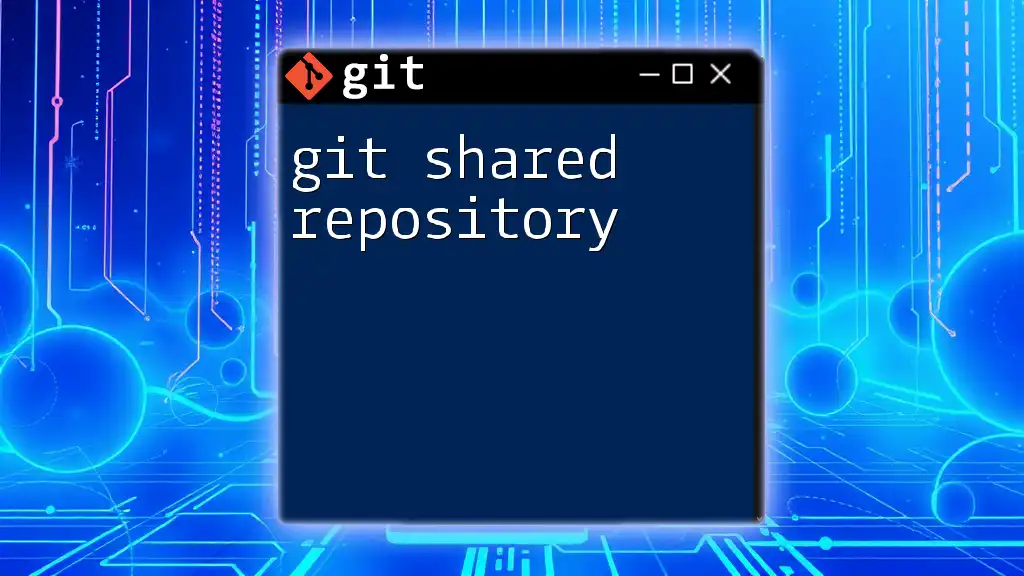
Pushing to the New Repository
The Git Push Command
Now that your changes are committed locally, it’s time to push them to your remote repository. The command for this is straightforward, yet powerful:
git push -u origin master
The `-u` flag is significant because it sets the upstream branch, meaning that your local `master` branch will be linked to the remote `origin/master`. This allows you to easily push changes in the future without additional arguments.
Understanding the Command Options
The command can have multiple options, but understanding its core functionality is crucial. By using `origin`, you specify where to push your changes, while `master` is the name of the primary branch. Git commonly uses `master`, but if you are on a different branch, replace `master` with your branch name.
Handling Push Errors
Sometimes errors may occur when pushing changes, such as non-fast-forward updates. This can happen if your local branch is behind the remote branch. In such cases, you will need to fetch the changes first:
git fetch origin
git merge origin/master
If you're confident that you want to overwrite the remote branch’s history, you can use the `--force` option, but exercise caution as this can lead to loss of data.
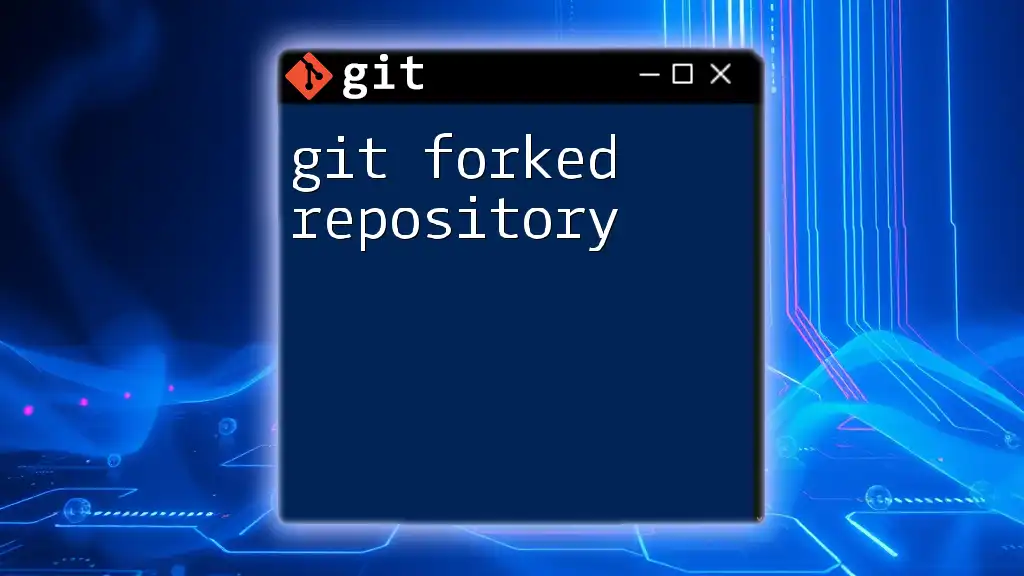
Confirming Successful Push
Verifying Changes on the Remote Repository
After executing the push command, it's essential to verify that your changes have been successfully uploaded to the remote repository. You can easily do this by navigating to your repository on GitHub. You should see your files and the commit message reflecting your changes.
Using Command-line Tools
To ensure your local repository is synchronized with the remote, you can check the branch status with:
git fetch
git status
This will provide you with current information about your branches, including how they relate to their remote counterparts.
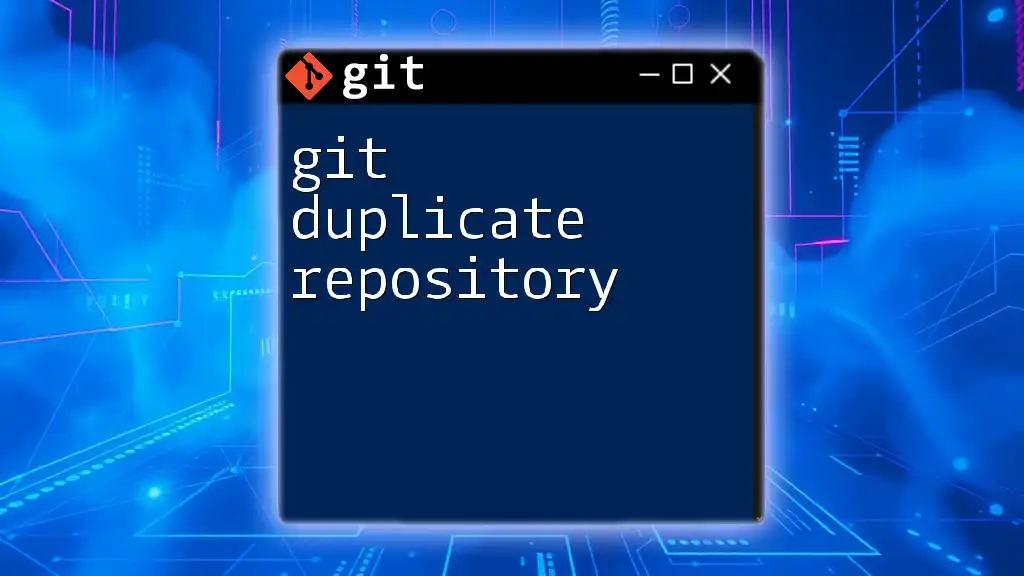
Conclusion
Succinctly, we've covered the process of git push to new repository through initial setup, linking, committing changes, and finally pushing your work to a remote repository. Mastering this flow will set a strong foundation for using Git effectively in your development tasks.
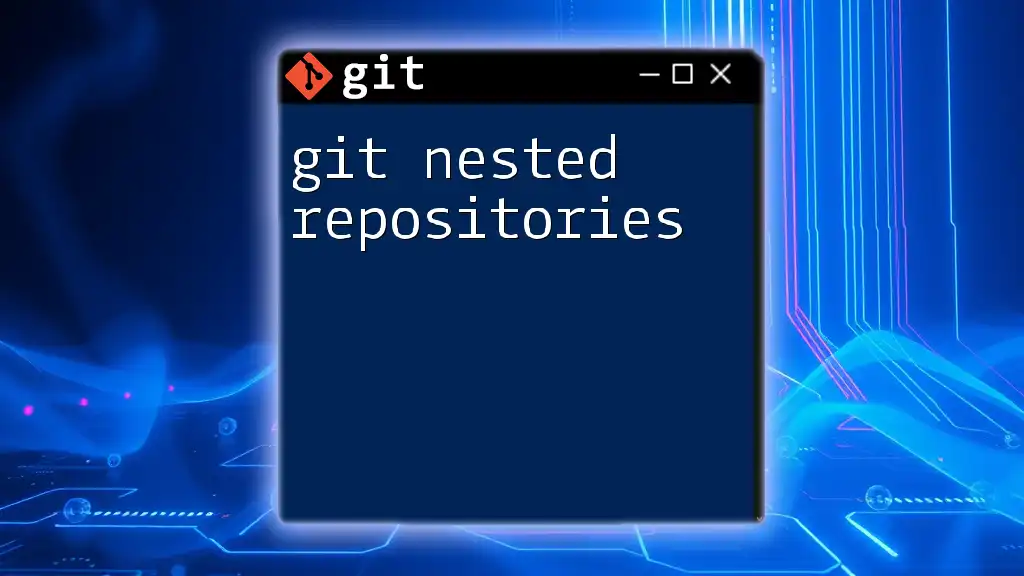
Additional Resources
To expand your Git skills further, consider exploring the official documentation. Platforms like GitHub offer invaluable resources and tutorials that can deepen your understanding of more advanced concepts and commands.
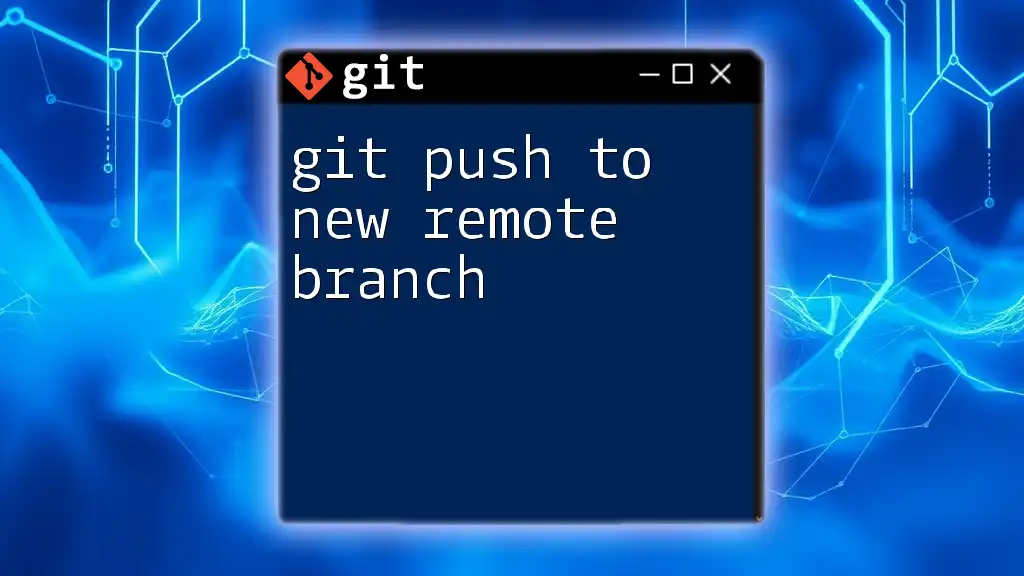
Call to Action
We would love to hear your thoughts or questions about this guide. Feel free to share your experiences or challenges in the comments below, and don't forget to check out our Git command workshops for more in-depth learning!