Git group repositories refer to managing multiple related repositories together under a single namespace, allowing for easier collaboration and organization of projects.
Here's a basic example of how to create a new group repository on GitLab using the command line:
git clone https://gitlab.com/your-username/group-repo.git
What Are Git Group Repositories?
Git group repositories are centralized spaces where teams can collaborate on projects using Git. These repositories allow multiple users to contribute to a shared codebase, making it essential for collaborative software development. By using group repositories, teams can efficiently manage their workflow, track changes, and maintain version control across different branches and features.
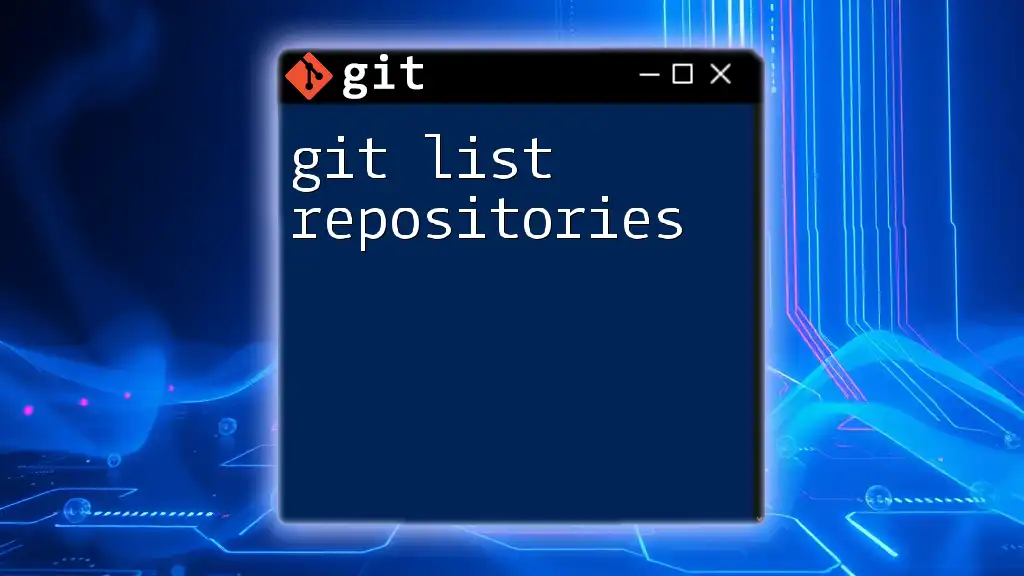
Why Use Git Group Repositories?
Utilizing Git group repositories offers several advantages, including:
- Enhanced Collaboration: Multiple developers can work simultaneously on different features without interfering with each other's work.
- Version Control: Each change made to the code is tracked, allowing teams to revert to previous versions if necessary.
- Centralized Management: Group repositories provide a single source of truth for the project's code, simplifying access and organization.
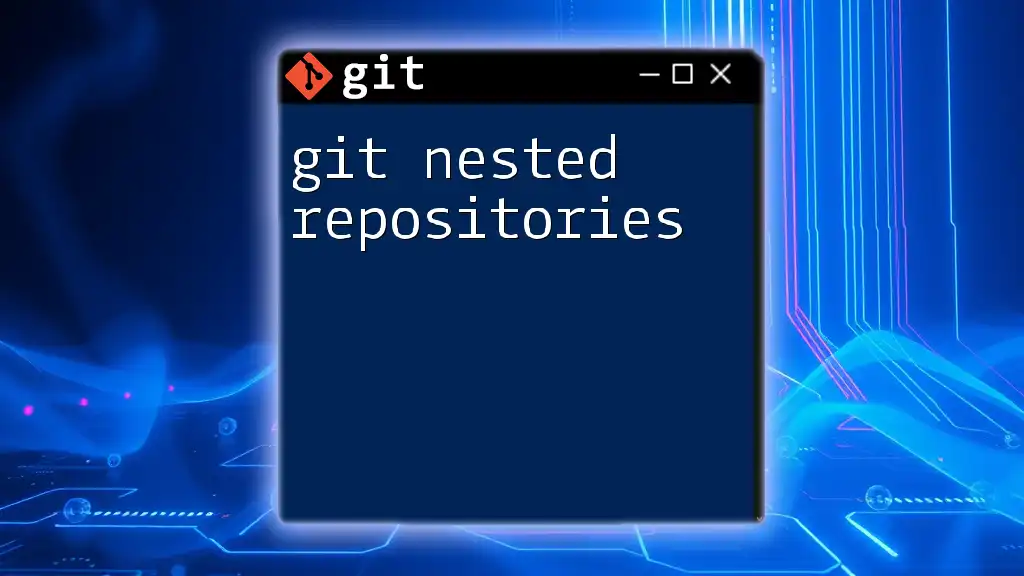
Setting Up Git Group Repositories
Understanding the Basics
Before setting up a group repository, ensure you have the necessary tools and accounts configured. Most commonly, services like GitHub, GitLab, and Bitbucket are used. These platforms offer user-friendly interfaces to help you manage your repositories effectively.
Creating a New Group Repository
To establish a new group repository, follow this step-by-step guide tailored for various platforms.
-
GitHub:
- Sign in to your GitHub account.
- Click on the "+" icon in the top right corner and select "New repository."
- Provide a name, choose "Public" or "Private," and check "Initialize this repository with a README."
- Click "Create repository."
-
GitLab:
- Log in to GitLab.
- On the dashboard, click "New project."
- Choose "Create blank project," fill in the necessary details, and set visibility.
- Click "Create project."
-
Bitbucket:
- Log into Bitbucket.
- Click on the "+" icon and select "Repository."
- Enter the repository details and select the access level.
- Click "Create repository."
To start your repository locally, you can use the following commands:
git init my-group-repo
cd my-group-repo
Configuring Group Permissions
Setting access controls is crucial for ensuring that only authorized individuals can make changes to the repository.
Types of Permissions:
- Read: Users can view the repository.
- Write: Users can modify the repository.
- Admin: Users can manage settings, collaborators, and permissions.
To invite team members, navigate to your repository's settings and look for an option to add collaborators or members. Assign permissions according to each member's role in the project.
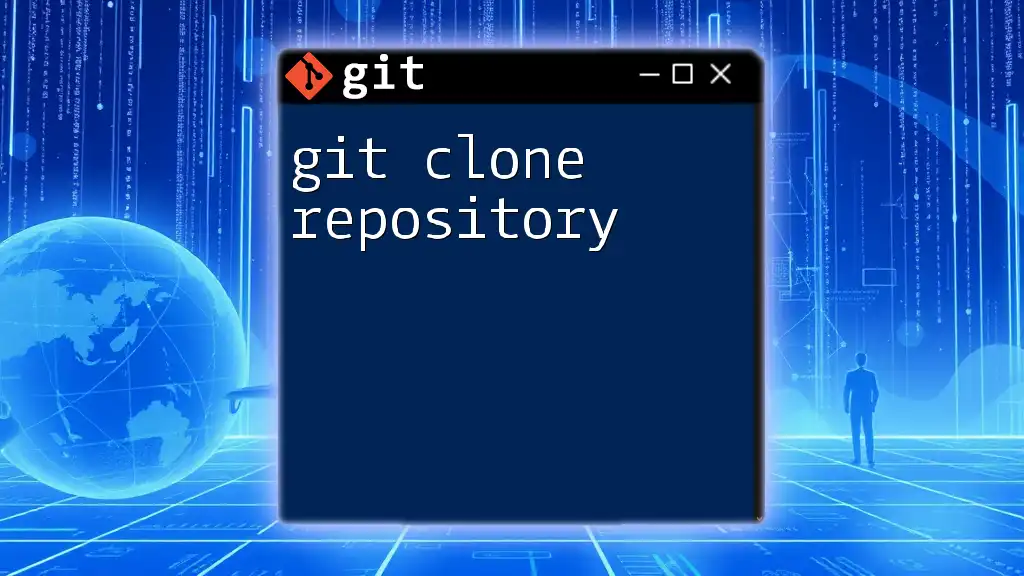
Working with Git Group Repositories
Cloning the Repository
Cloning allows team members to work on a local copy of the repository. To clone a group repository, use the following command:
git clone https://github.com/your-org/my-group-repo.git
This command creates a local copy of the repository on your machine, enabling you to start working immediately.
Creating and Managing Branches
Branching is vital for a collaborative workflow. It allows different team members to work on separate features without affecting the main codebase.
To create a new branch, you can use:
git checkout -b feature/new-feature
This command creates and switches to a new branch named `feature/new-feature`. Each feature or fix can be developed on its own branch, later merging them into the main branch when complete.
Pull Requests and Code Reviews
Understanding Pull Requests
Pull requests (PRs) are a way to propose changes to the codebase. When a team member wants to merge their changes into the main branch, they create a PR, which facilitates peer review and ensures quality control.
Creating a Pull Request
Once you push your branch to the remote repository, you can create a pull request. Navigate to the repository on the platform (like GitHub or GitLab) and select the "Pull Requests" tab. Click "New Pull Request," choose the branches you want to merge, and provide a descriptive title and message.
Code Review Process
Conducting code reviews is essential for maintaining code quality. When reviewing, look for clarity, efficiency, and adherence to coding standards. Use inline comments to provide feedback, and discuss any changes that may need further attention.
After the review, the pull request can be approved and merged if everything is satisfactory.
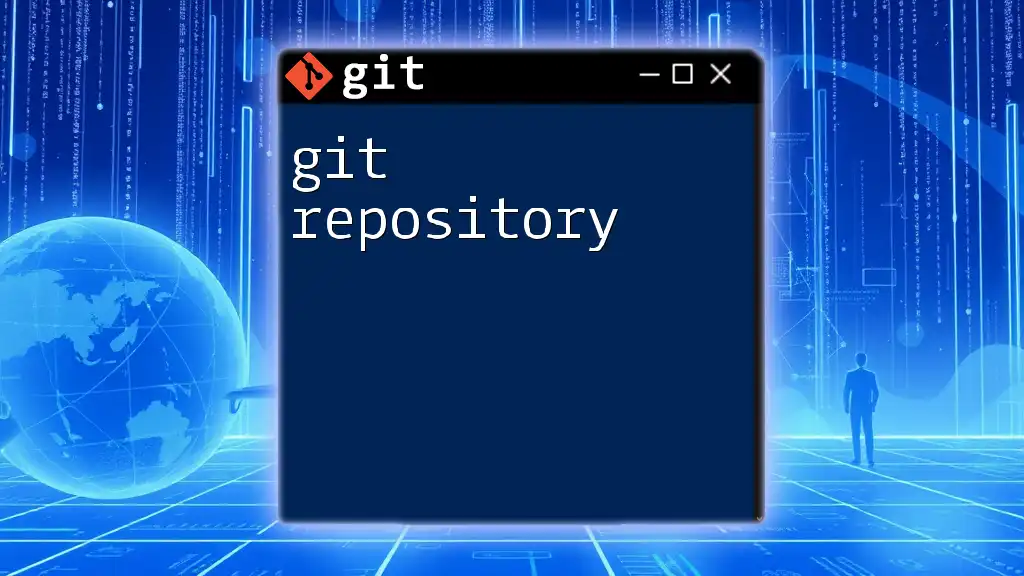
Best Practices for Git Group Repositories
Commit Standards
Writing meaningful commit messages is crucial for understanding the project's chronology. Each message should convey the essence of the changes made. For example, instead of "fixed stuff," a better message would be:
Fix issue with user login by changing validation logic.
This clarity helps team members comprehend the project's progress quickly.
Keeping the Repository Organized
The organization of your repository greatly affects its usability. A well-structured directory system ensures that files can be easily located. Common structures include directories for source code, tests, and documentation.
Handling Conflicts
Merge conflicts occur when two or more changes overlap in the code. Ensuring clearness in your team's workflow, such as regular communication about branch usage, can minimize conflicts.
Resolving Conflicts Step-by-Step
When a conflict arises, Git will indicate the conflicting files. You can check the status:
git status
Then use a merge tool to resolve conflicts, mark files as resolved, and commit the changes:
git mergetool
git add .
git commit -m "Resolved merge conflicts in feature/new-feature"
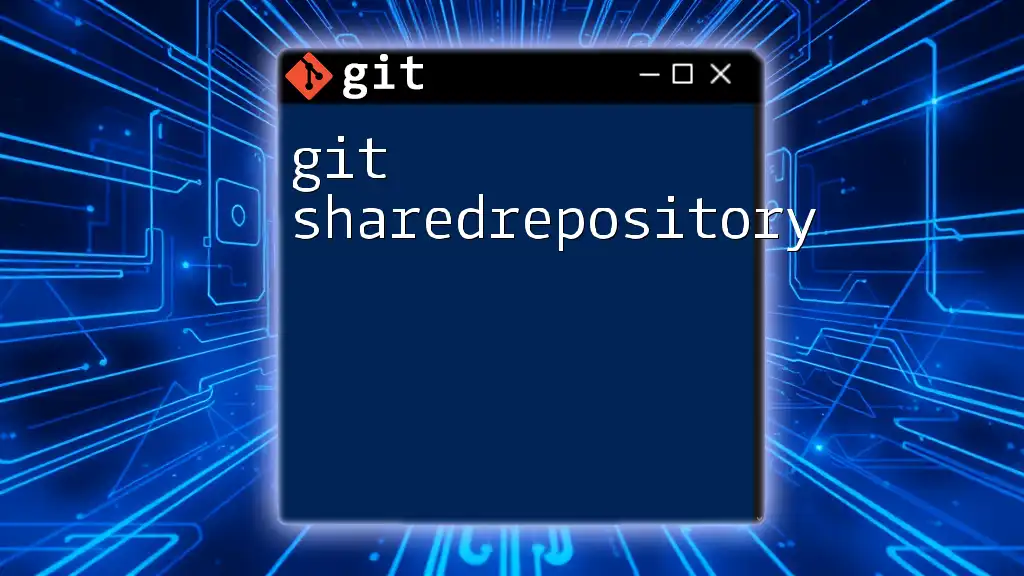
Integrating Continuous Integration/Continuous Deployment (CI/CD)
What Is CI/CD?
Continuous Integration (CI) and Continuous Deployment (CD) are practices that integrate code changes frequently and deploy them automatically. This ongoing process helps teams detect issues sooner and ensure higher quality in their software.
Setting Up CI/CD Pipelines
Using tools like GitHub Actions or GitLab CI/CD simplifies implementing CI/CD protocols. For instance, a simple GitHub Actions workflow might look like this:
name: CI
on: [push]
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Run Tests
run: ./run_tests.sh
This YAML configuration defines a basic CI pipeline that executes on every push to the repository, helping automate testing and quality checks efficiently.
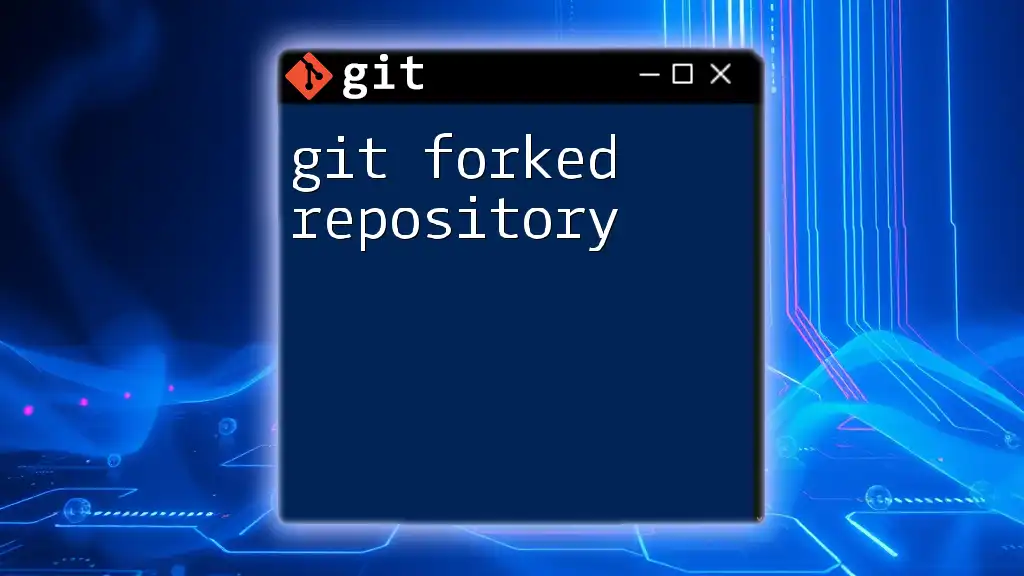
Conclusion
Git group repositories are an indispensable part of modern software development, facilitating collaboration and organized version control. By following best practices and utilizing the rich features provided by platforms like GitHub and GitLab, teams can optimize their workflows and achieve their development goals more effectively. Embrace the power of Git group repositories to foster a collaborative environment and enhance productivity in your projects.
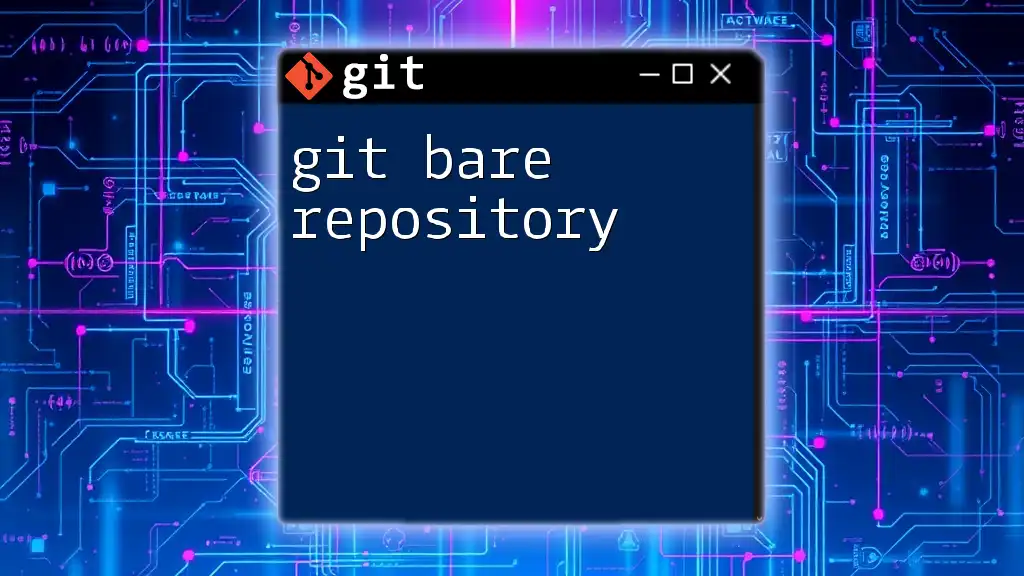
Additional Resources
To deepen your understanding of Git and group repositories, consider exploring the official Git documentation, and enrolling in recommended tutorials and courses tailored for collaboration and version control.