A Git shared repository is a central location where multiple users can collaborate on a project by pushing and pulling changes to and from the Git repository.
git clone https://github.com/username/repository.git
Understanding Git Shared Repositories
What is a Shared Repository?
A shared repository in Git is a centralized storage location that multiple users can access to collaborate on a project. This allows teams to work together more efficiently, as everyone can contribute, review, and merge changes seamlessly.
The primary difference between a shared repository and a local repository is that the former is accessible to multiple collaborators who can read from and write to it, while the latter is private to a single user. When considering version control in collaborative environments, utilizing a shared repository is essential for tracking changes and coordinating efforts.
Example: Imagine a team of developers working on a software project. Each member can clone the shared repository, make their changes independently, and then push those changes back to the shared repository for integration. This ensures that everyone is on the same page and can maintain a clear project timeline.
Types of Shared Repositories
Centralized vs. Distributed Repositories
Shared repositories can be classified into two main types: centralized and distributed.
Centralized systems require all users to communicate directly with a central server to access and modify the repository. While more straightforward, this model can lead to bottlenecks and single points of failure.
In contrast, distributed systems, like Git, allow each user to have their own local copy of the repository in addition to the shared one. This empowers users with flexible workflows, including offline work, merging, and branching.
-
Pros of Centralized Repositories:
- Simplicity in access management.
- Direct control over changes in a single location.
-
Cons of Centralized Repositories:
- Risk of server downtime impacting development.
- Limited collaboration options for offline use.
-
Pros of Distributed Repositories:
- Enables extensive collaboration, even offline.
- Each user has a complete history of changes.
-
Cons of Distributed Repositories:
- Steeper learning curve for beginners.
- Potential complexity in managing multiple local copies.
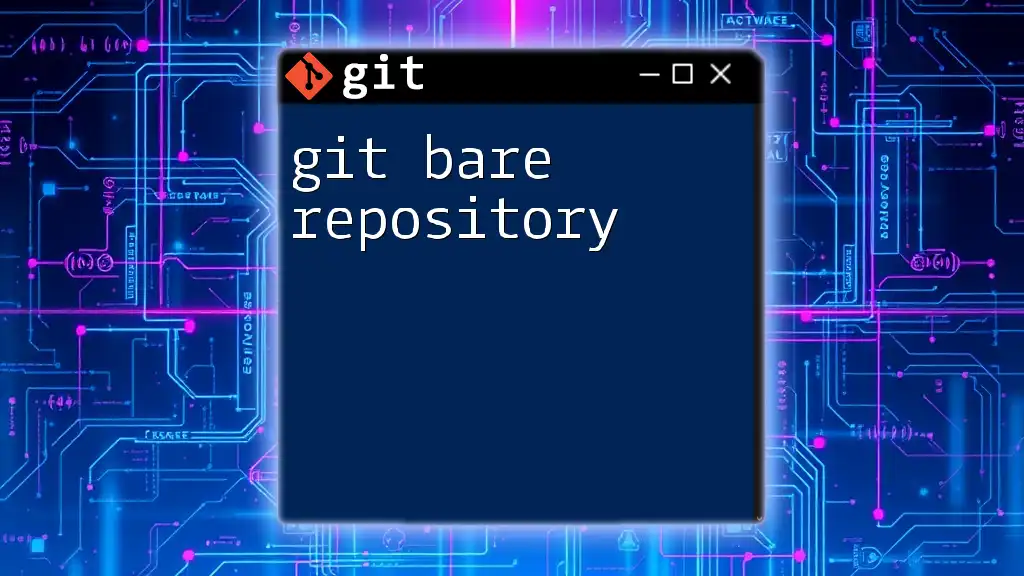
Setting Up a Shared Repository
Hosting Options for Shared Repositories
There are several popular platforms where teams can host their shared repositories, including GitHub, GitLab, and Bitbucket.
GitHub
To create a shared repository on GitHub, follow these steps:
- Sign in to your GitHub account.
- Click on the "+" icon at the top right and select "New repository."
- Fill in the repository name, description, and choose whether it will be public or private.
- Click the "Create repository" button.
After creating your repository, push your local changes using the following command:
git push origin main
GitLab
GitLab is known for its comprehensive features suited for teams. To set up a repository in GitLab:
- Sign in to GitLab and navigate to your dashboard.
- Click on "New Project."
- Choose to create a new repository or import one.
- Set the project visibility and click "Create project."
Bitbucket
Bitbucket provides an integrated approach for teams to manage both Git and Mercurial repositories. Setting up a repository on Bitbucket is similar:
- Log in to your Bitbucket account.
- Click on "Create Repository."
- Fill in the necessary details (repository name, type).
- Click "Create repository" to finalize.
Configuring Access Permissions
Understanding and managing access permissions is crucial in a shared repository. You can set different roles for users:
- Admin: Full control, including managing access.
- Write: Can modify content in the repository.
- Read: Can view the content but not make changes.
For instance, on GitHub, navigate to Settings > Manage access to assign roles to collaborators, ensuring everyone has the appropriate permissions for their contributions.
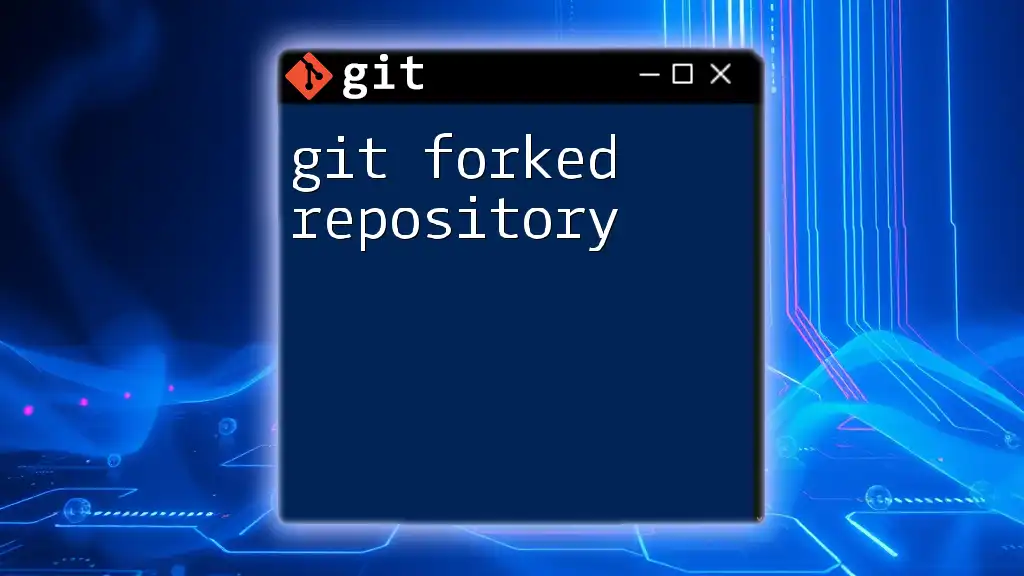
Collaborating with a Shared Repository
Cloning the Repository
When joining an existing shared repository, the first step is to clone it to your local machine. Use the following command:
git clone <repository-url>
This command creates a local copy of the shared repository, including all files and the entire history of the project. Having a local clone allows you to work on changes offline and stage them for submission later.
Making Changes and Committing
Best Practices for Commit Messages
Commit messages are essential for conveying the history of changes. It's important to write clear and informative messages that reflect the purpose of your changes. Here are some guidelines:
- Use the imperative mood (e.g., "Fix bug" instead of "Fixed bug").
- Keep messages concise yet descriptive.
- Reference issues or tickets when applicable.
Example: A good commit message would read "Add user authentication feature" compared to a less useful one like "Update files."
Handling Merge Conflicts
Merge conflicts arise when two or more changes clash during a merge operation. This is common in shared repositories. Here’s how to resolve a merge conflict:
- Use the command `git merge <branch-name>` to merge changes from one branch to another.
- If conflicts occur, Git will mark the files in conflict. Open these files and look for the conflict markers (`<<<<<<<`, `=======`, `>>>>>>>`).
- Resolve the conflicts by choosing which changes to keep or combining changes.
- After resolving, stage the resolved files:
git add <resolved-file>
- Complete the merge process with:
git commit
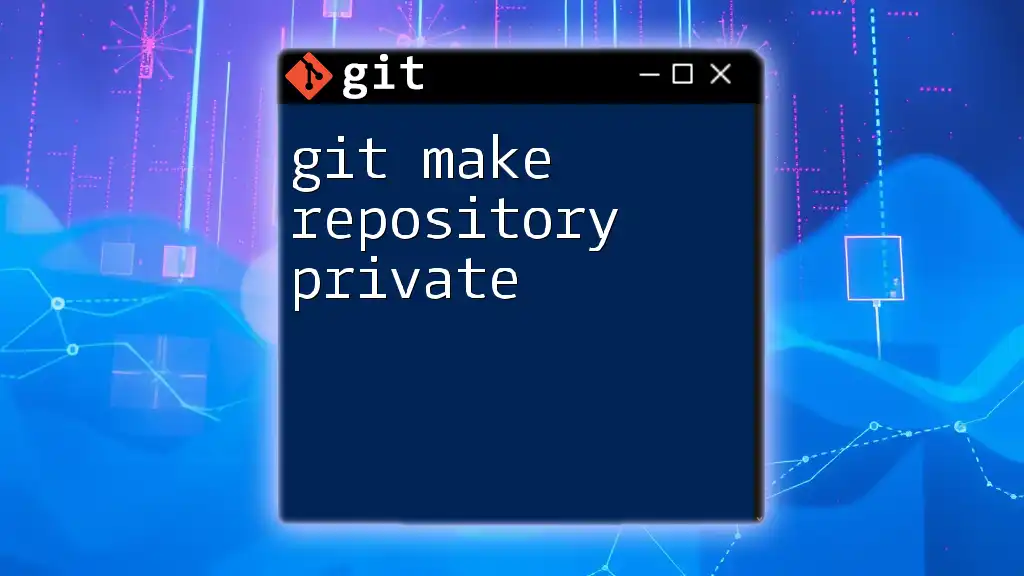
Advanced Features of Shared Repositories
Pull Requests
Pull requests (PRs) are essential in facilitating code reviews and discussions before merging changes into the main branch. They allow team members to review, comment, and suggest changes to code before it becomes part of the shared repository.
To create a pull request on GitHub:
- Push your branch to GitHub.
- Navigate to the repository page.
- Click on the "Pull requests" tab and then "New pull request."
- Select your branch and create the pull request with a detailed description.
Example: A typical pull request might include a note about the changes implemented and any issues resolved, prompting team members to review before merging.
Branching Strategies
Branching is a critical concept in Git that facilitates working on features independently. Two popular branching strategies include:
- Git Flow: A structured branching model that involves multiple branches for features, releases, and hotfixes. Ideal for larger projects with structured release cycles.
- GitHub Flow: A simpler approach focusing on continuous delivery. It uses a single main branch and feature branches.
To create and merge a branch, you can use:
git checkout -b <new-branch-name>
Followed by making commits, and when ready to merge:
git checkout main
git merge <new-branch-name>
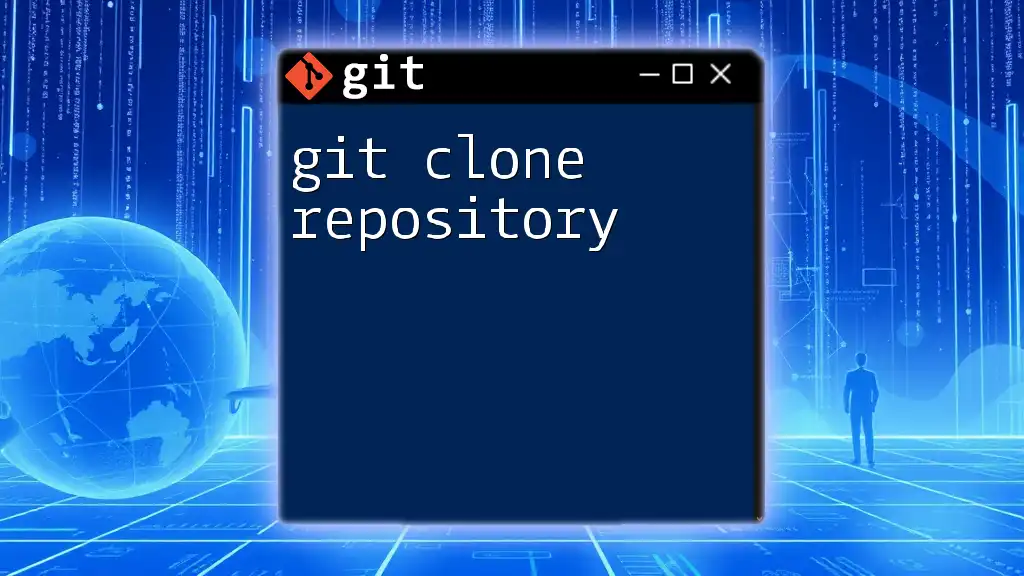
Best Practices for Using Shared Repositories
Maintain a Clean History
Keeping a clean commit history is essential for any shared repository as it helps others understand the evolution of the project. Consider using `git rebase` to streamline commits before merging. For example, the command:
git rebase -i HEAD~<number>
allows you to condense or reorder your commits for clarity.
Regularly Syncing with the Main Branch
To avoid potential merge conflicts, regularly sync your branch with the main branch. Use these commands:
git fetch origin
git pull origin main
This ensures that you are up-to-date with the latest changes before pushing your own contributions.
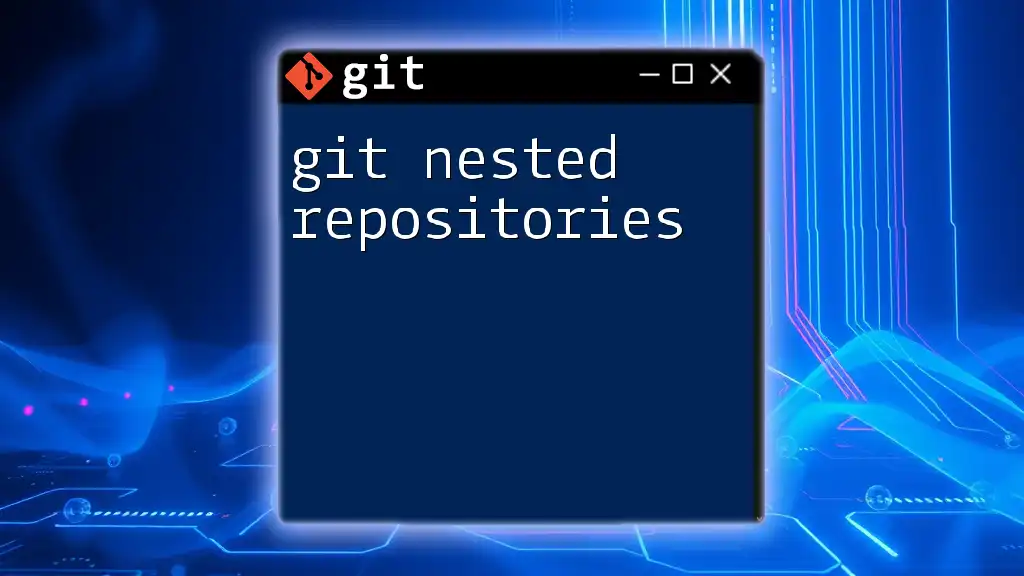
Conclusion
Embracing the concept of a git shared repository is fundamental for any collaboration in software development. Understanding how to set it up, collaborate effectively, and utilize advanced features will assure a seamless workflow for you and your team. By adopting best practices, you can maintain a structured and efficient development environment, ultimately leading to successful project outcomes.
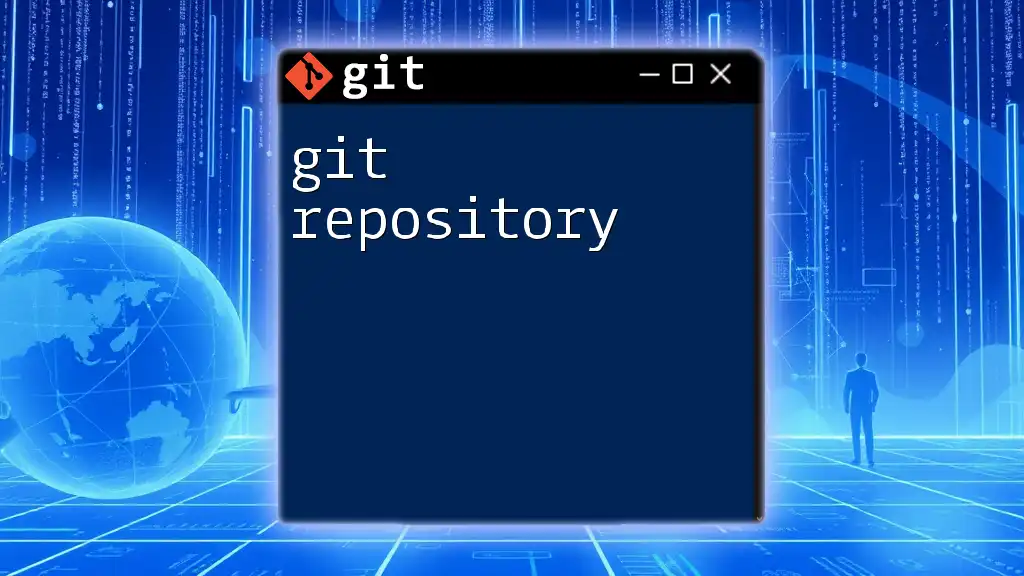
Additional Resources
For further exploration of Git and its features, consider visiting the following resources:
- [Official Git Documentation](https://git-scm.com/doc)
- [GitHub Guides](https://guides.github.com/)
- [GitLab Documentation](https://docs.gitlab.com/)
- [Git Community Forums](https://git-scm.com/community)
By utilizing these resources, you can deepen your understanding of Git and enhance your skills in managing shared repositories.