A Git template repository is a repository that contains files and configurations to be reused in new repositories created from it, enabling you to quickly set up standard project structures.
Here's a code snippet on how to create a new repository from a template:
git clone --bare https://github.com/username/template-repo.git
cd template-repo.git
git push --mirror https://github.com/username/new-repo.git
What is a Git Template Repository?
A git template repository is a special type of repository that serves as a blueprint for initializing new Git repositories. It allows you to set up a new project with a predefined structure, configurations, and files. This can significantly enhance productivity and maintain consistency across projects, especially in collaborative environments.
The primary purpose of a template repository is to save time and effort in the initial setup phase. Instead of starting from scratch with each new project, you can simply clone or initialize from the template, ensuring that all the needed files and configurations are ready to go.
Use Cases
Template repositories are beneficial in various scenarios:
- Onboarding New Projects: When starting a new project, especially in a team setting, you want to ensure that everyone has the same starting point.
- Consistent Structure Across Teams: For organizations with multiple teams or departments, using a common template helps maintain a uniform project structure.
- Standardizing Development Practices: Including best practices, such as coding styles, linting configurations, and CI/CD setup in the template promotes adherence to organizational coding standards.
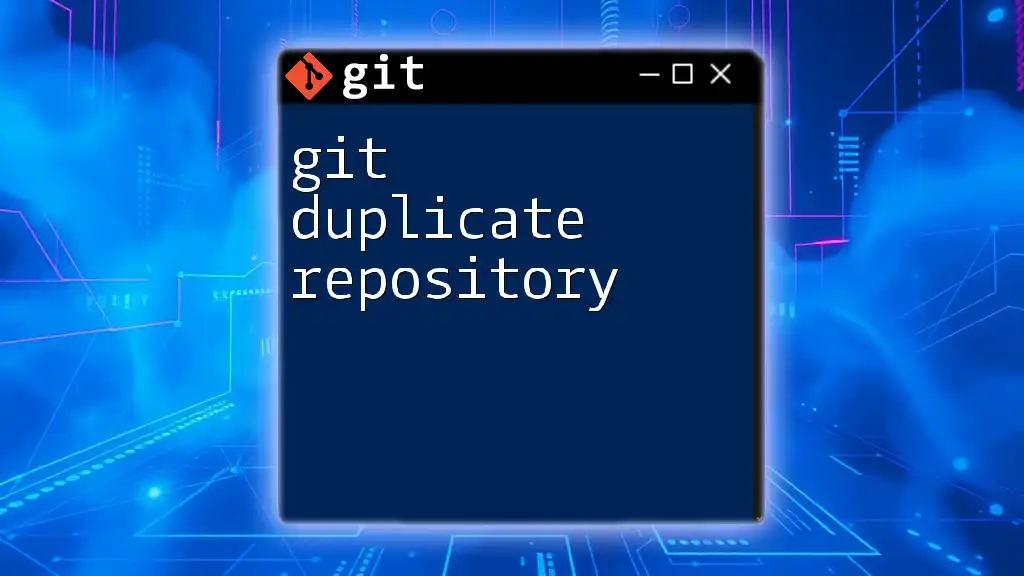
Setting Up Your Template Repository
Creating a New Template Repository
To create a git template repository, the first step is to set it up on your preferred Git hosting platform, such as GitHub, GitLab, or Bitbucket.
- Create a new repository in your account.
- Initialize the repository from your local machine to start adding files. Use the following commands:
mkdir my-template-repo
cd my-template-repo
git init
Structuring Your Template
When organizing your template repository, consider including the following essential files and folders:
- README.md: A mandatory file that provides information about the project, including how to use it, installation steps, and contribution guidelines.
- LICENSE: Specify the licensing terms for your repository, ensuring users understand their rights and obligations.
- .gitignore: List files and directories that should be ignored by Git to prevent committing unnecessary files (like logs or temporary files).
Additionally, consider creating directories for:
- Source code, ideally separated by language or module (e.g., `/src`, `/lib`).
- Documentation (e.g., `/docs`).
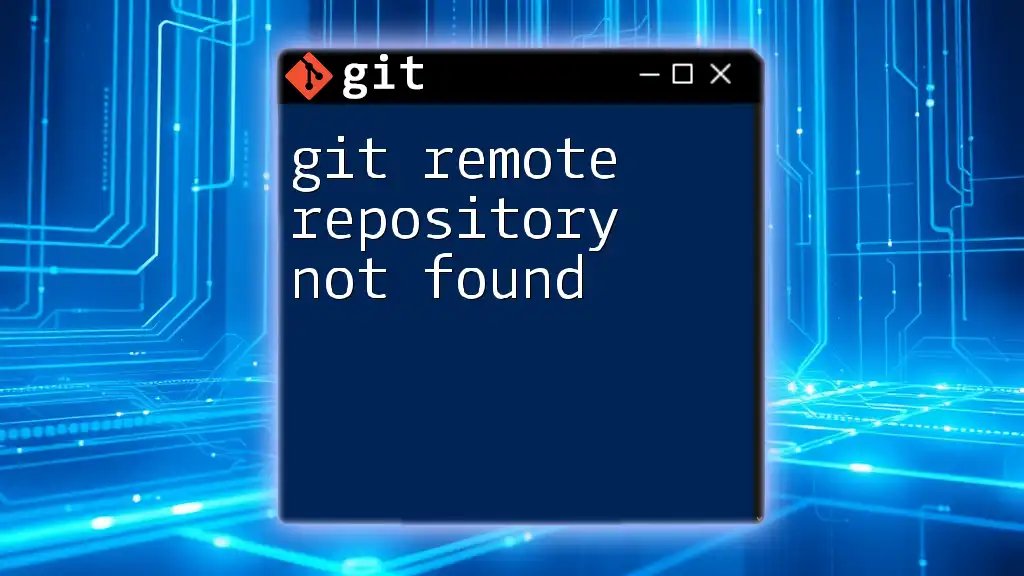
Customizing Your Template Repository
Adding Custom Configurations
One powerful aspect of git template repositories is the ability to include custom configurations. These might include:
- Git hooks: Scripts that run at certain points in the Git lifecycle (e.g., before making a commit). For instance, you could add a pre-commit hook to automatically run linters on the code.
- Branch structures: Define a default branch or multiple branches tailored for specific development workflows.
Using the `git init` Command with Templates
Once your template is set up, initiating a new repository from your template is straightforward. You can use the `git init` command with the `--template` option. Here’s how you would do it:
git init --template=/path/to/your/template/repo
In this command, replace `/path/to/your/template/repo` with the actual path to your template repository. By doing this, Git uses your template's structure and configurations for the new repository automatically.
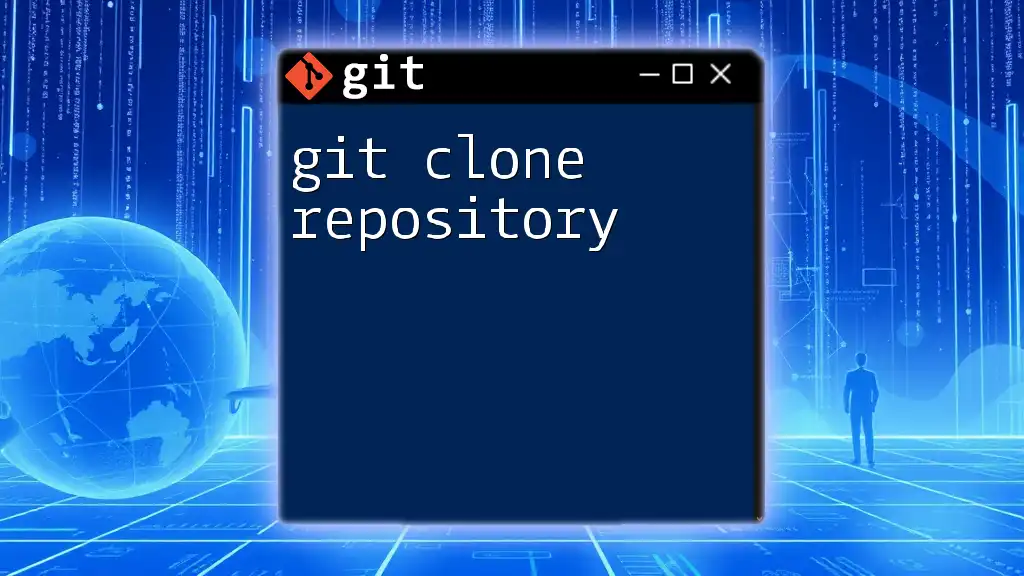
Cloning the Template Repository
Using Template Repositories on GitHub
If your template repository is hosted on platforms like GitHub, you can quickly create a new project repository based on it:
- Navigate to your template repository.
- Click on the "Use this template" button (which appears if your repository is set up as a template).
- Fill in the details for your new repository (name, description, visibility) and click "Create repository from template."
To clone the newly created repository on your local machine, use the following command:
git clone https://github.com/username/my-template-repo.git new-project
Replace `username` and `my-template-repo` with your actual username and template repository name. The `new-project` is the directory where the cloned repository will reside.
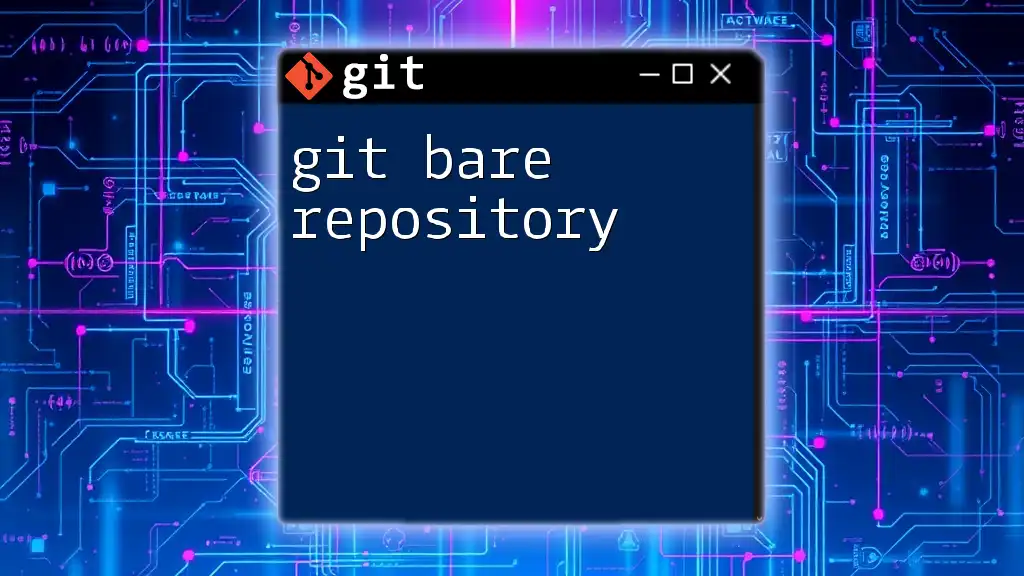
Best Practices for Managing a Template Repository
Maintaining and Updating Your Template
Keeping your git template repository up-to-date is crucial to ensuring new projects benefit from the latest configurations and best practices. Here are strategies to maintain it:
- Regularly review and modify the template based on feedback from team members.
- Implement version control to track changes to the template itself, making it easier to revert to previous states if needed.
Sharing Your Template
Depending on your audience or team, decide whether to make your template public or private. Sharing it generally encourages collaborative contributions, where team members can suggest improvements or modifications.
Make sure to include guidelines in your README for contributing back to the template repository, fostering an environment of continuous improvement.
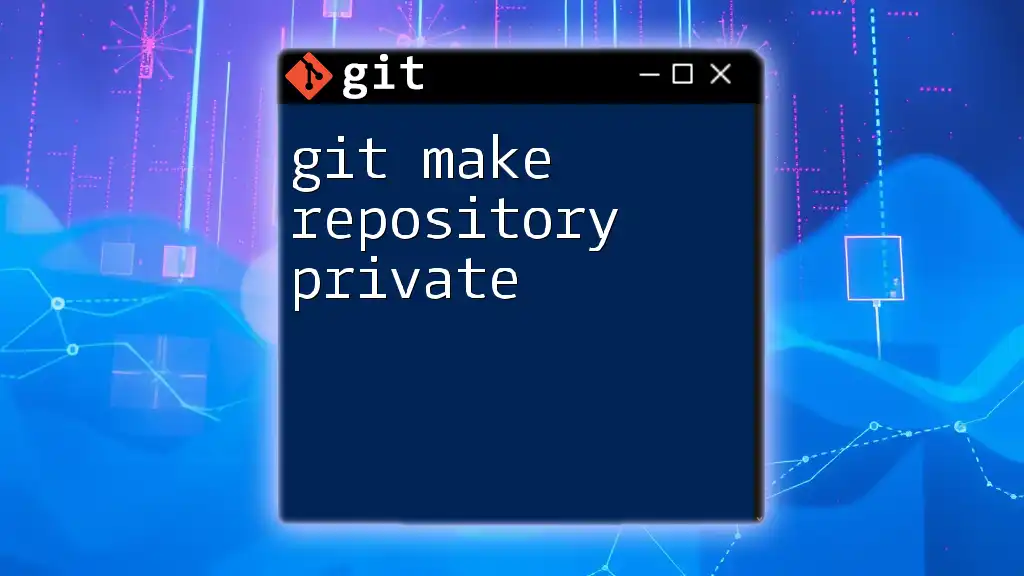
Automation and Integration
Integrating CI/CD Pipelines
Integrating Continuous Integration and Continuous Deployment (CI/CD) pipelines into your template repository sets the foundation for automated testing, building, and deployment of projects. You can include configuration files for popular CI/CD services like GitHub Actions or Travis CI right in your template.
For example, a simple GitHub Actions configuration might look like this:
name: CI
on:
push:
branches:
- main
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Set up Node.js
uses: actions/setup-node@v2
with:
node-version: '14'
- name: Install dependencies
run: npm install
- name: Run tests
run: npm test
This configuration ensures that every push to the main branch triggers the CI process, promoting continuous delivery of your code.
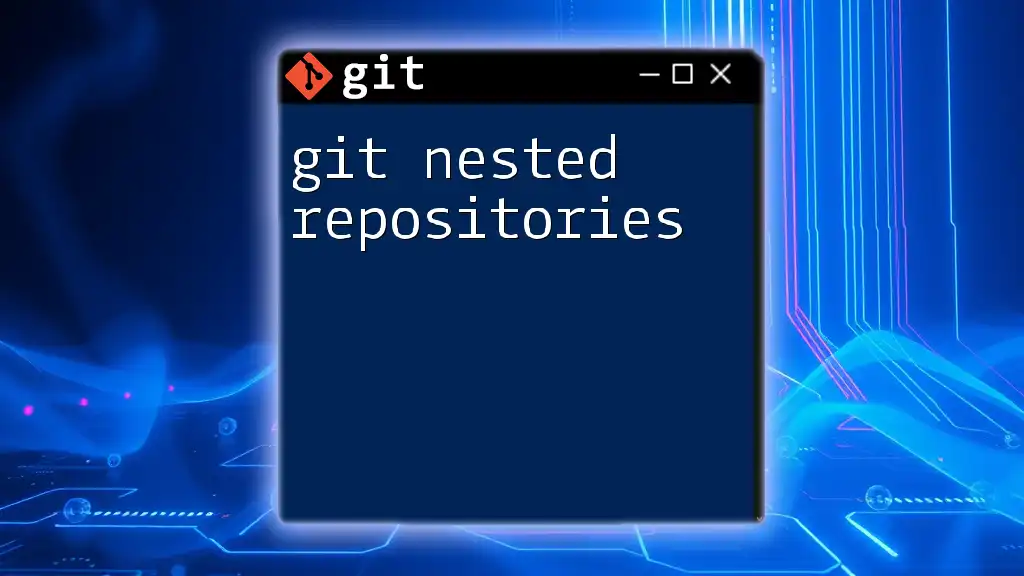
Common Issues and Troubleshooting
Potential Pitfalls and How to Avoid Them
When working with git template repositories, you may encounter some common mistakes:
- Not keeping the template updated: Failing to update a template can lead to projects being initialized with outdated configurations.
- Inadvertently including sensitive information in the template: Always ensure no credentials, tokens, or sensitive data are hardcoded into the template.
If you run into troubleshooting issues while using your template, check for mismatches between expected files and those initialized. Recheck your template repository’s path and settings to ensure everything is set up correctly.
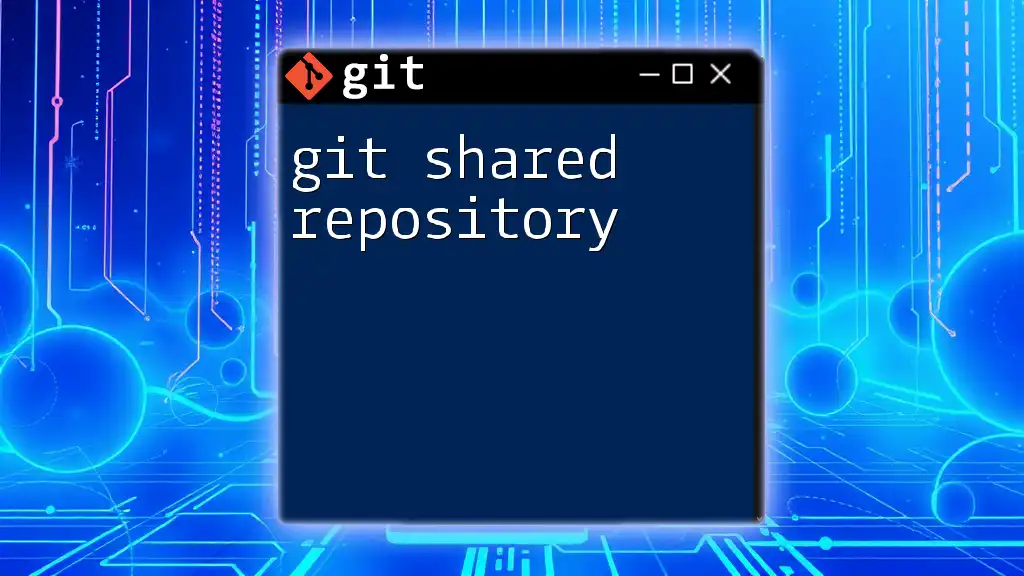
Conclusion
Utilizing a git template repository fosters greater efficiency during project initialization, allowing teams to focus more on development rather than project setup. By leveraging templates, you can standardize practices across projects, streamline development workflows, and ultimately enhance overall productivity.
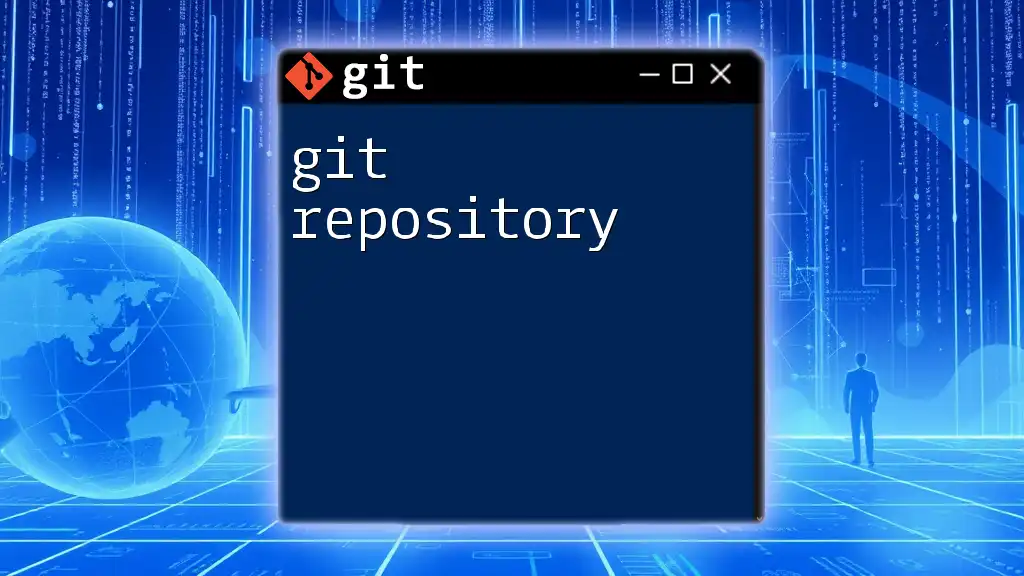
Call to Action
We encourage you to try creating your own git template repository and share your experiences! Did it simplify your project setup? Are there any specific customizations that you found particularly useful? Share your thoughts or suggest topics you would like to explore further in the world of Git.
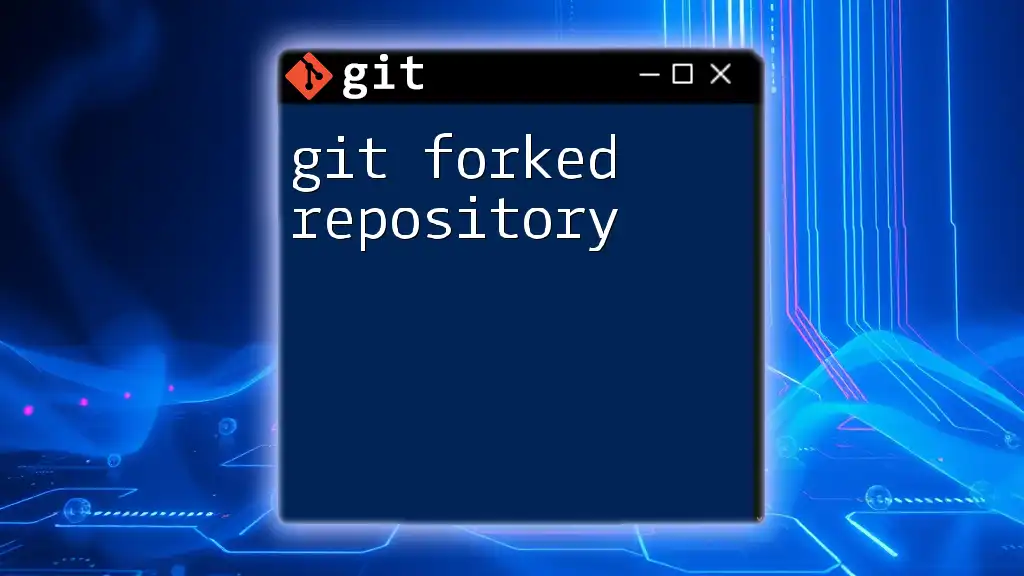
Additional Resources
To dive deeper into git template repositories, consult the official Git documentation for more insights. Additionally, numerous online courses and tools can further enhance your Git skills and understanding.
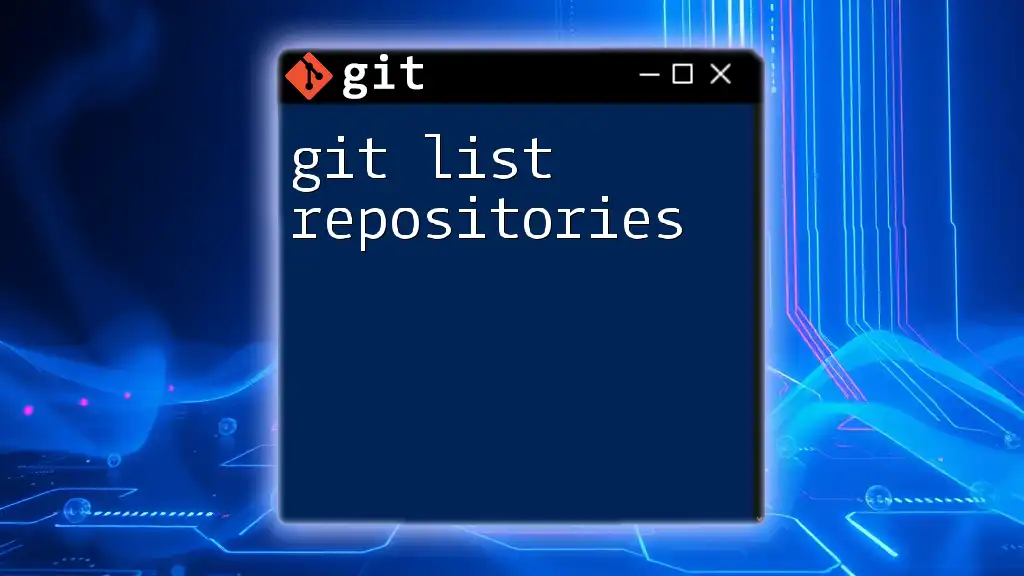
Frequently Asked Questions (FAQ)
What is the difference between a regular repository and a template repository?
A regular repository serves as a storage space for a project, while a template repository is designed to create new repositories with a predefined structure.
Can I use template repositories for multiple programming languages?
Absolutely! You can create a template repository that supports any language or framework, simply by organizing the specific structure needed for each.
Are there any limitations when using a Git template repository?
The primary limitation may arise from the complexity of the template itself. If the template includes many configurations or specialized tools, users may need additional documentation to fully understand and effectively use it.