A Git hard reset will remove all changes in the working directory and staging area, resetting your current branch to a specified commit, which can be done with the following command:
git reset --hard <commit>
Replace `<commit>` with the desired commit hash or reference.
Understanding Git Reset
What is Git Reset?
The Git reset command is a powerful tool used in the Git version control system. It allows users to move the current `HEAD` to a specified state, effectively altering the commit history. Git reset can be categorized into three types: soft, mixed, and hard. Each serves different purposes based on how you wish to handle changes in your working directory and staging area.
- Soft Reset: This moves the `HEAD` to a specified commit, keeping the changes in the staging area. It’s mainly used if you want to adjust your last commits but keep the changes.
- Mixed Reset: The default reset that moves `HEAD` and resets the staging area while keeping changes in the working directory. This is useful for un-staging files without losing actual modifications.
- Hard Reset: This command erases all changes in both the staging area and the actual files in your working directory, aligning them with a specified commit.
The Concept of Resetting
Resetting affects several components in the Git ecosystem:
- Current HEAD Position: The `HEAD` represents your current commit or your position in the repository’s history. Understanding where it points is crucial before executing any reset.
- Staging Area: The changes you have staged for the next commit can be discarded or maintained, depending on which reset you perform.
- Working Directory: The files you see in your project folder also get affected, and with `git hard reset`, they will revert to match a desired commit.
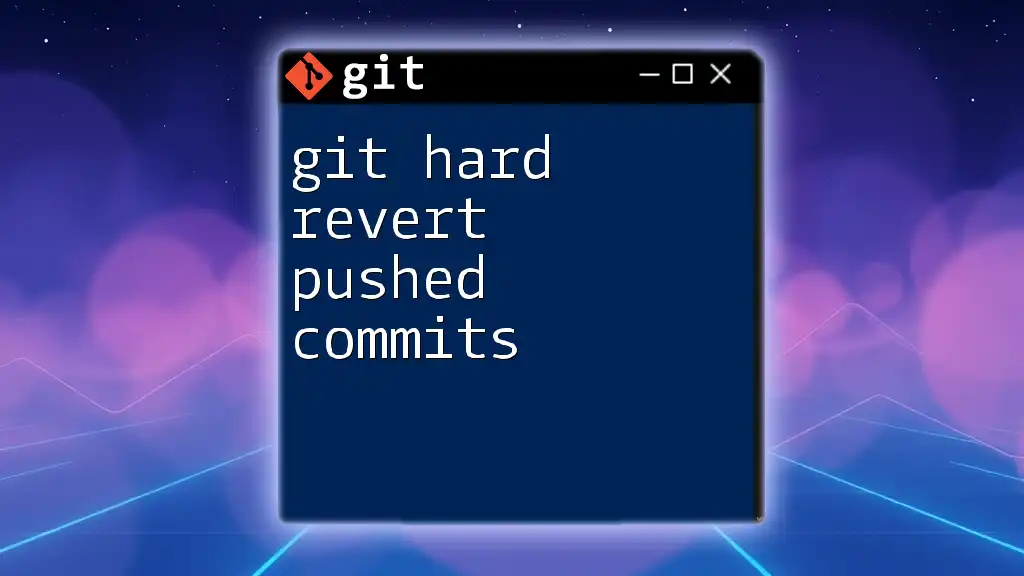
The Hard Reset Command
What Does `git reset --hard` Do?
Executing `git reset --hard` instructs Git to:
-
Impact on Staging Area: The command clears the staging area by aligning it with the specified commit. It means that all staged changes will be lost.
-
Impact on Working Directory: All changes made in tracked files since the last commit will be discarded. This means that any edits you've made will be irreversibly removed from your files.
-
Impact on History: The command alters the commit history by moving the `HEAD` pointer to a previous commit, effectively “forgetting” all commits that came after it.
Syntax of the Command
The general syntax for performing a hard reset is as follows:
git reset --hard [commit]
- The `[commit]` parameter represents the commit hash to which you want to reset. Omitting it defaults to the most recent commit (`HEAD`). If you execute `git reset --hard` without specifying a commit, you risk losing all uncommitted changes.
Common Use Cases for Hard Reset
-
Reverting to a Previous Commit: If you've committed code that broke your application, you can quickly return to the previous working state:
git reset --hard HEAD~1
This command resets the current branch to one commit prior.
-
Undoing Unwanted Changes: If you've made changes that you no longer want in your working directory, a hard reset can be effective:
git reset --hard
This will discard any changes after the last commit.
-
Resetting a Branch to Match Remote: Sometimes, you need to align your local branch with what exists on the remote. This command will force your local branch to mirror the remote branch:
git reset --hard origin/main
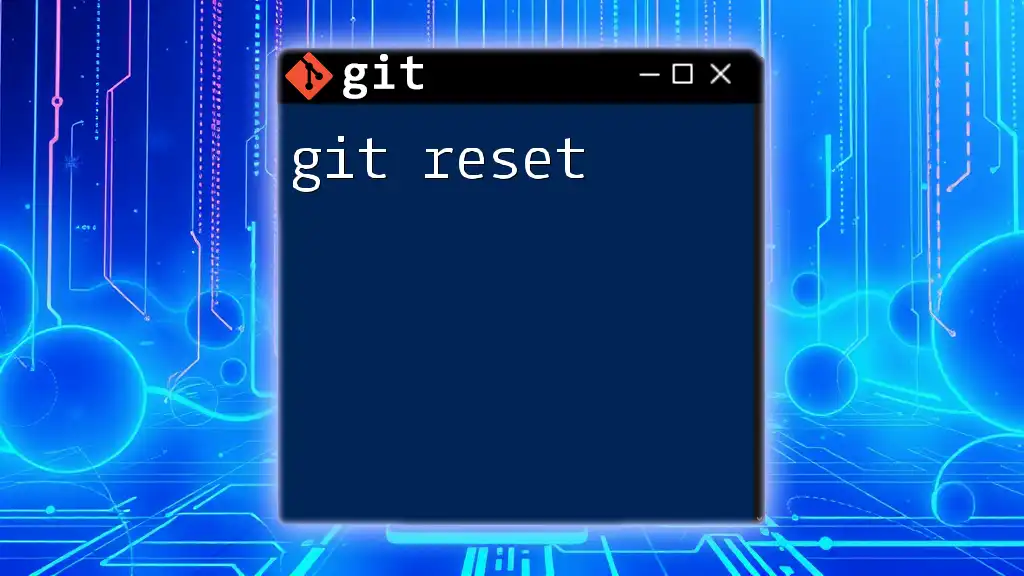
Risks and Precautions
Understanding the Risks
Using `git reset --hard` carries certain risks, primarily loss of uncommitted changes. Any tracked files modified and those in the staging area will disappear when executing this command. It can lead to potential data loss if you reset without being fully aware of what you will lose.
Precautionary Measures
Before using `git reset --hard`, consider taking the following precautions:
-
Backup your changes: You can temporarily save uncommitted changes in the stash using:
git stash
-
Creating a New Branch: Another good practice is to create a new branch as a backup before performing a hard reset:
git checkout -b backup-branch
This way, you can always return to your changes if you realize you need them post-reset.
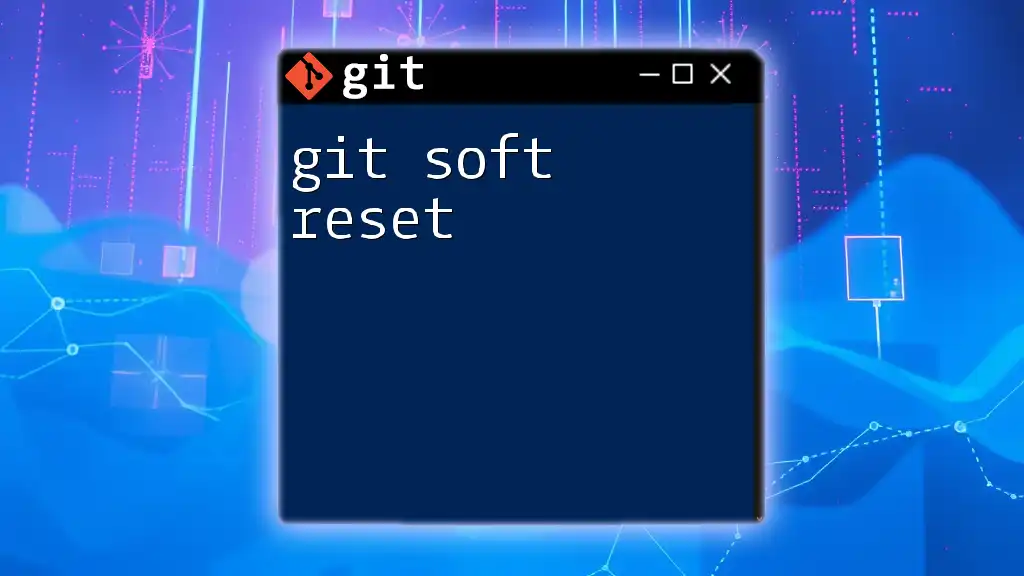
Practical Examples
Example Scenario: Reverting a Mistake
Imagine you've committed several changes but realize that a specific commit has introduced bugs. In this case, you can resolve the issue by reverting back:
- Identify the commit's hash that contains the last known good code.
- Execute the reset command:
git reset --hard [commit-hash]
Replace `[commit-hash]` with the actual hash. This will give you a clean slate to work from.
Example Scenario: Syncing with Remote
You're working on a feature branch and have made several changes. However, you decide that you need to discard these changes to align strictly with what’s on the remote branch. Here’s what you would do:
- Ensure you're stationed on your feature branch.
- Execute:
git reset --hard origin/main
This command will erase all local changes and match your feature branch exactly with the latest state of the `main` branch on the remote.
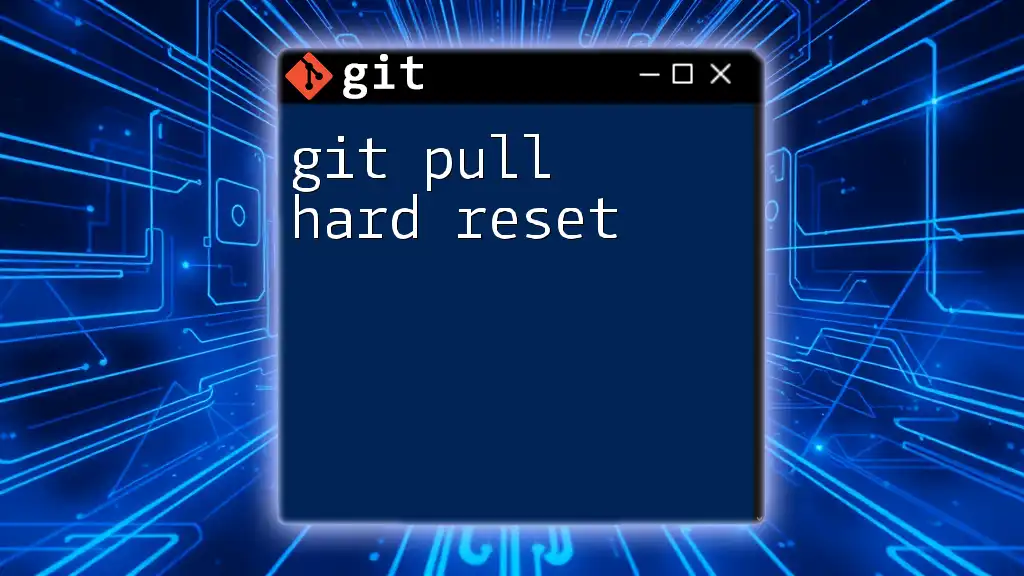
Alternatives to Hard Reset
Using `git revert`
When you need to undo changes but want to maintain the history intact, using `git revert` is advisable. This command creates a new commit that counters the changes made in specified commits but does not remove them from the history. It’s the safer choice when you want to maintain an audit trail.
Using `git checkout`
Another alternative is to use `git checkout` to switch to a previous commit without modifying the commit history. This allows you to explore different states of your repository without permanently discarding current changes.
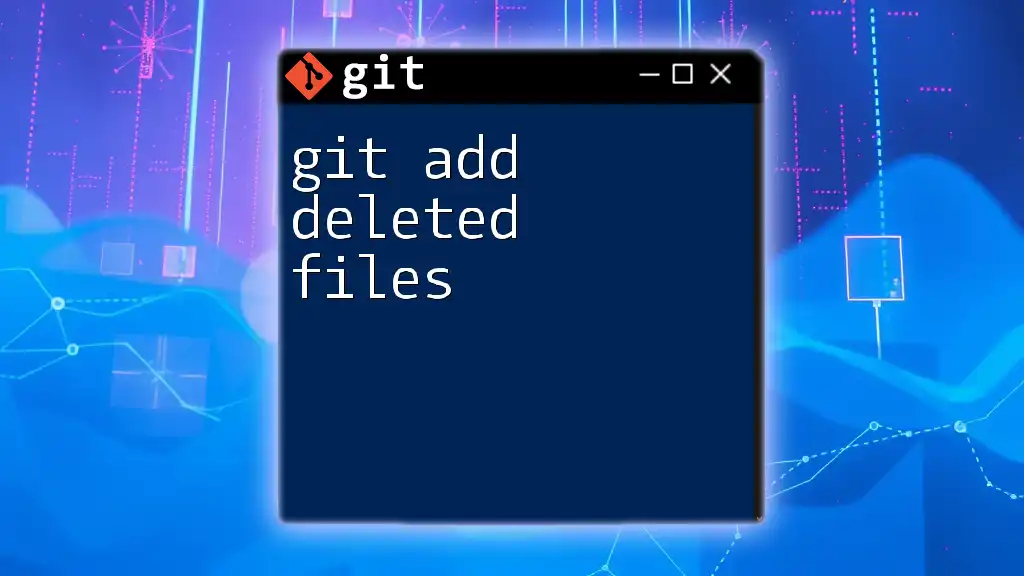
Conclusion
In summary, understanding how to utilize git hard reset effectively is crucial for managing changes in your Git repository. While it offers a straightforward solution for undoing changes, it comes with significant risks. Always consider best practices to safeguard your work, and when in doubt, opt for safer alternatives like `git revert`. Regular practice and familiarity with the command will bolster your confidence as you navigate the world of version control with Git.
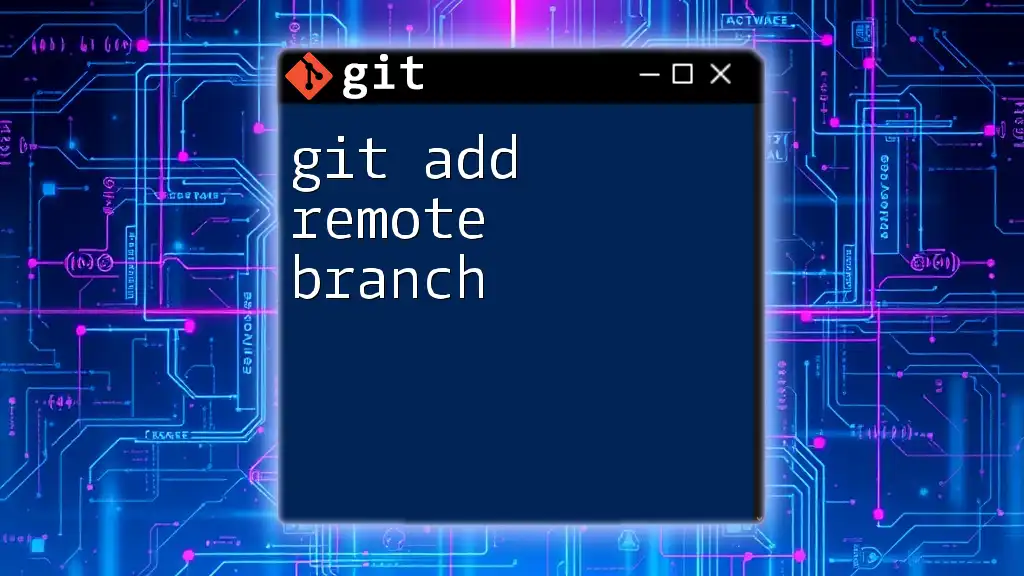
FAQ Section
-
What happens if I do a hard reset and then remember an important change? After a hard reset, it’s often impossible to recover uncommitted changes directly. However, if your changes were committed before the reset, you could reference your commit history to find the lost changes.
-
Can I recover lost changes after performing a hard reset? It’s challenging to recover changes lost in a hard reset. If you didn't back them up, they're typically gone unless you had previously pushed those changes to a remote or used `git stash`.
-
How does `git reset --hard` affect branches? `git reset --hard` affects the current branch by moving the `HEAD` to the specified commit while removing all modifications to tracked files. It's important to know how this could affect collaborative work in a shared repository.