In Git, the `stash` command temporarily shelves (or stashes) changes you've made to your working directory, allowing you to switch contexts without committing those changes.
git stash
Understanding Git Stash
What is Git Stash?
`git stash` is a powerful feature in Git that allows you to temporarily save changes that you have made to your working directory without committing those changes to your local repository. This can be particularly useful when you need to switch branches or pull updates but haven't finished your current work. By stashing your changes, you can keep your working directory clean while you focus on other tasks.
The primary purpose of `git stash` is to help you manage unfinished changes without cluttering your branch history. It's ideal for developers who occasionally find themselves needing to change context quickly, allowing them to come back later and resume work right where they left off.
How `git stash` Works Behind the Scenes
When you execute `git stash`, Git creates a new commit that includes your modified tracked files and records the state of the working directory and the index. It's important to understand that when you stash your changes, you don't lose them—they're safely stored in your stash stack.
Each time you stash changes, you create a new entry in the stash stack, which can be viewed, applied, or dropped later as needed. Unlike a commit, stashes are not part of the branch history, which makes them transient by design.
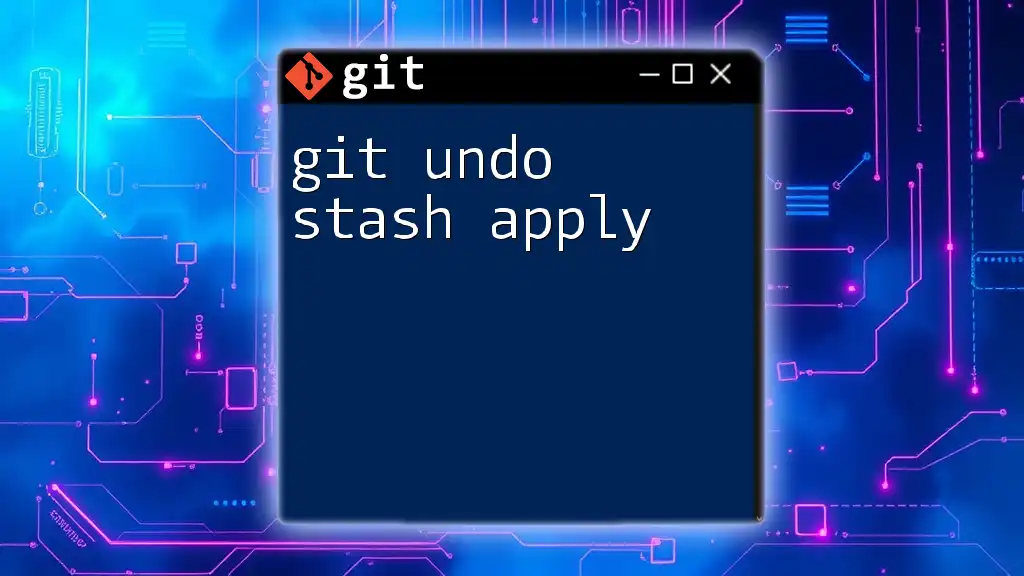
Basic Git Stash Commands
Stashing Changes
To stash your changes, use the following command:
git stash
This command will save your modifications and revert your working directory to match the last commit on your current branch. You can use `git stash` anytime you find your workspace cluttered with local changes that you do not wish to commit yet.
Stashing untracked files: If you want to stash changes that include new, untracked files, you can do so using the `-u` option:
git stash -u
This is particularly handy if you're experimenting with new features but still want to update or switch branches without committing anything unfinished.
Viewing Stashed Changes
To see a list of all the stashed changes you've completed thus far, use:
git stash list
The output will show a list of stashes, each identified by a name like `stash@{0}`, `stash@{1}`, etc., along with the commit messages that indicate when they were created. Reviewing your stashes helps you determine which one you want to apply later.
Applying Stashed Changes
To apply the most recent stash (without removing it from the stash list), use the command:
git stash apply
If you want to apply a specific stash, you can refer to it by its identifier:
git stash apply stash@{0}
Keep in mind that applying stashed changes can result in merge conflicts if there are conflicting modifications between your stashed changes and the files in your working directory.
Using `git stash pop`
The `git stash pop` command works similarly to `git stash apply`, but with the crucial difference that it removes the stash from the stash list after applying it:
git stash pop
This is an efficient way to work since it cleans up your stash like a regular commit would, ensuring you don’t develop a backlog of stashed changes that you forget about.
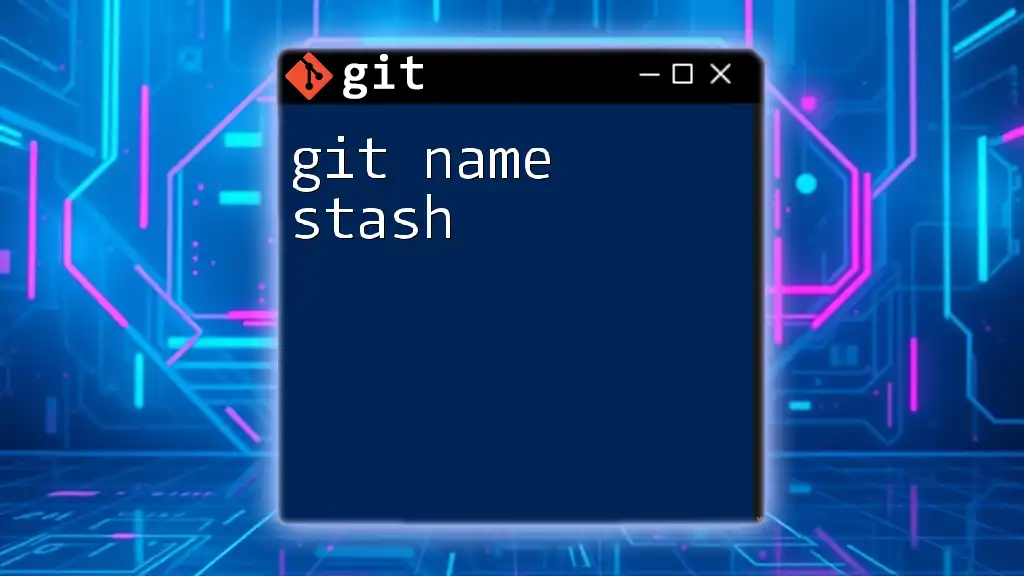
Advanced Stashing Techniques
Stashing with Messages
To make it easier to identify stashed changes later, you can add a descriptive message when you stash:
git stash save "WIP: Fix login bug"
Using clear, meaningful messages helps you keep track of multiple stashes, especially when working on several features or bug fixes.
Stashing Specific Files
If you only want to stash a particular file instead of everything in your working directory, you can specify the file path:
git stash push <file-path>
This allows you to selectively stash changes, providing greater flexibility in managing your changes without affecting all files in your workspace.
Dropping and Clearing Stashes
To remove a specific stash from your stash stack, use:
git stash drop stash@{0}
This command will delete the specified stash entry. If you ever want to remove all stashes, you can use:
git stash clear
Be cautious with this command; once you clear the stash, those changes are permanently lost. It's a good practice to review your stashes periodically and clean them up when they're no longer needed.
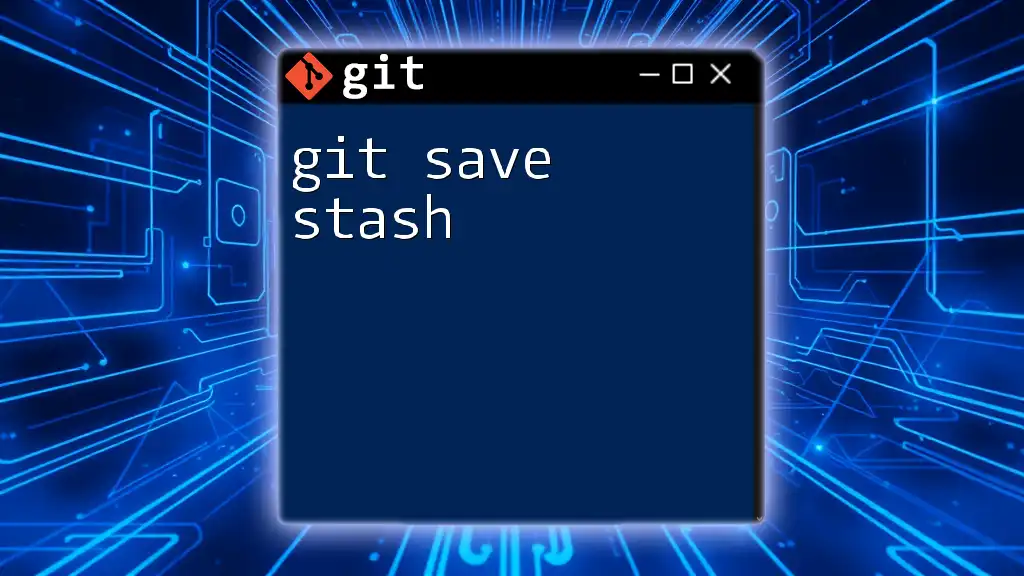
Use Cases for Git Stash
Example 1: Switching Branches
Imagine you're in the middle of a feature implementation but need to switch branches to fix an urgent bug. By following these steps, you can manage your unfinished work smoothly:
- Stash your current changes:
git stash
- Switch to the appropriate branch:
git checkout master
- Apply your stashed changes when you're ready to return:
git stash pop
Example 2: Code Review or Collaboration
When preparing for a code review, you might have local changes that are not quite ready to be committed. Using `git stash` allows you to tidy up your workspace:
- Stash changes that need further work:
git stash
- Pull the latest changes from the repository and run the review process.
- After the review, retrieve your stashed changes:
git stash pop
Example 3: Integrating Work from Multiple Sources
In situations where you're working with features that involve collaboration with others, it may become necessary to consolidate changes. You can easily stash your local modifications, pull in changes from the remote repository, and then apply your stashed changes:
- Stash local changes:
git stash
- Update your local branch:
git pull
- After resolving any potential merge conflicts, apply your stashed changes:
git stash pop
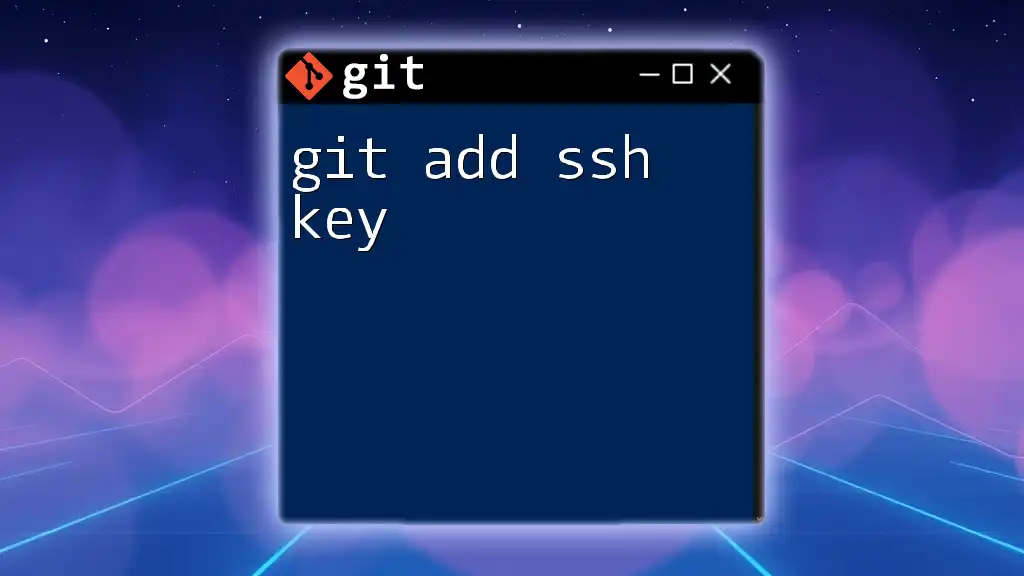
Common Pitfalls and Best Practices
Common Issues When Using Git Stash
Merge conflicts often arise when applying stashed changes, especially if other modifications have been made to the same files. Be prepared to resolve these conflicts in your working directory after applying a stash.
You may also lose track of multiple stashes, leading to confusion about which changes belong to which task. Regularly using `git stash list` can help keep you informed about your stashes.
Best Practices for Using Git Stash
- Use meaningful messages with each stash to keep track of changes easily.
- Regularly review and clean up your stashes to avoid unnecessary clutter. Set resolutions or temporary deadlines for yourself to determine when it's necessary to apply or drop stashed changes.
- Avoid relying on stash as your primary tool for managing changes; it should supplement a more structured version control workflow. Remember that stashes are intended for temporary or transitional periods of work.
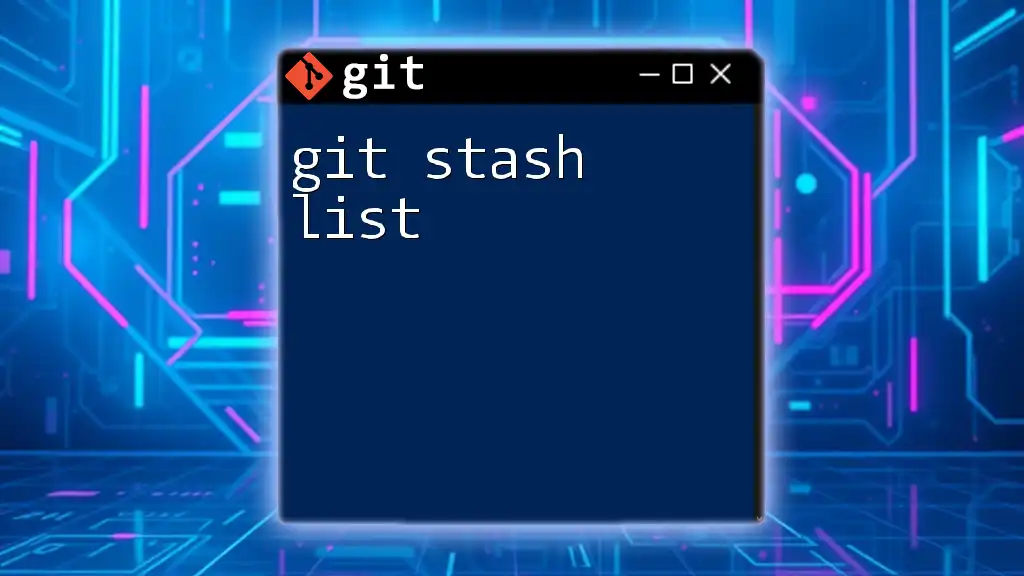
Conclusion
`git stash` is a vital tool in your Git arsenal, enabling developers to manage context switching effectively while keeping their workspaces tidy. By mastering the techniques of stashing, applying, and dropping changes, you can enhance your workflow and increase productivity. Practice using these commands in real-life scenarios to deepen your understanding of Git. Whether you're dealing with multiple features, urgent fixes, or code reviews, `git stash` provides the flexibility you need to manage your developments effectively.
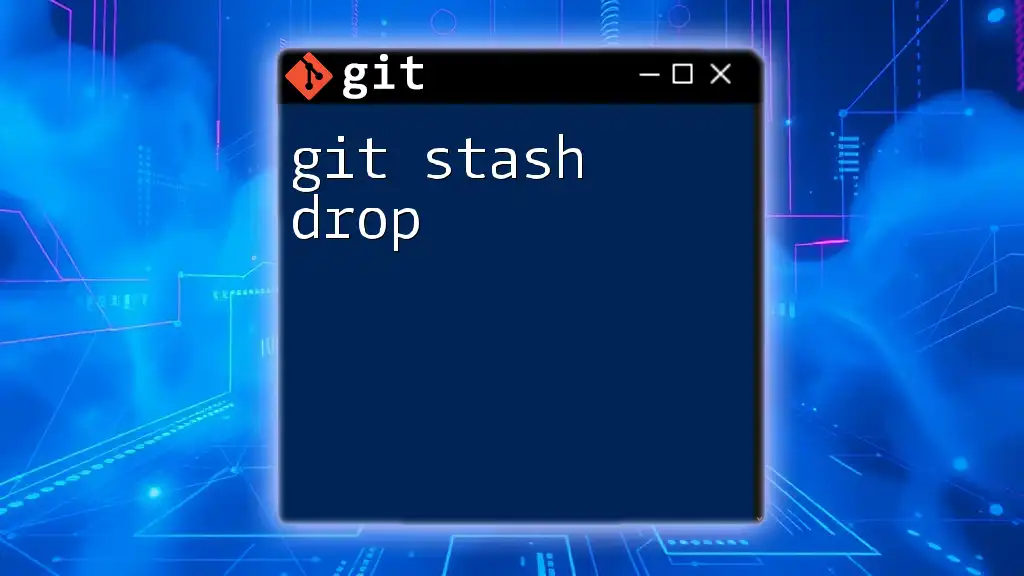
Additional Resources
For further exploration of Git commands and workflows, you may refer to the official Git documentation or consider enrolling in dedicated courses that focus on mastering Git and version control practices.