Git is a distributed version control system that allows developers to track changes in their code, while GitHub is a cloud-based platform that hosts Git repositories and facilitates collaboration among developers.
Here’s a simple command to initialize a new Git repository:
git init my-repo
Understanding Git Concepts
What is Version Control?
Version control systems (VCS) are essential tools in modern software development that manage changes to files and directories over time. They allow multiple developers to work on a project simultaneously without conflicting with each other's changes. By keeping a history of modifications, version control provides the ability to revert to previous states, collaborate effectively, and track who made which changes.
Key Terminology
Repository (Repo): A repository is a storage space where your project resides. It can be hosted locally on your machine or on platforms like GitHub. To create a new repository locally, you can use the command:
git init
Commit: A commit is a snapshot of your changes at a particular point in time. Commits are essential for maintaining a history of your project and for reverting back if necessary. You can make a commit with a descriptive message using the command:
git commit -m "Your message here"
Branch: Branches allow you to work on different versions of your project simultaneously. While the main branch (often called `main` or `master`) typically contains the stable version of your code, you create separate branches to develop new features or fix bugs without affecting the main codebase. To create a new branch, use:
git branch <branch-name>
Git Workflow
A typical Git workflow consists of several key steps:
- Clone: You start by cloning an existing repository, which copies its contents to your local machine. This can be done using:
git clone <repository-url>
-
Modify: After cloning, make changes to files locally to add features or fix bugs.
-
Stage: Before committing your changes, you need to stage them. Staging allows you to prepare changes, ensuring only specific changes are included in the next commit. You can stage files with:
git add <file-name>
-
Commit: With your changes staged, you can then create a commit to save those changes with a meaningful message.
-
Push: Finally, you push your local commits to the remote repository, making them available to others. The command for this is:
git push origin <branch-name>
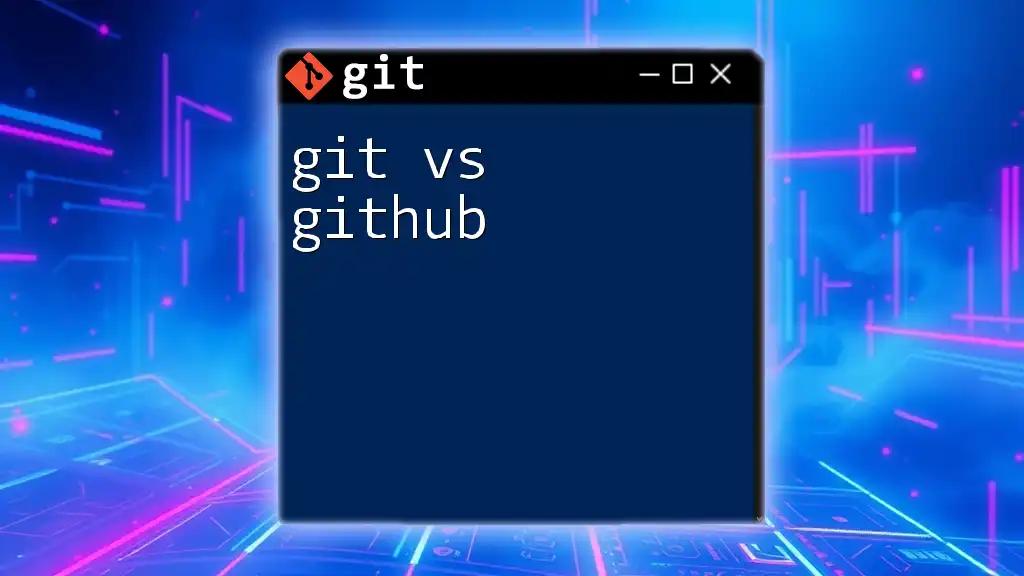
Getting Started with Git
Installing Git
To begin using Git, you first need to install it. Git can be installed on various operating systems:
- Windows: Download the Git installer from the official Git website and follow the installation instructions.
- macOS: You can install Git using Homebrew with the command:
brew install git
- Linux: Use your package manager, for example, on Ubuntu:
sudo apt-get install git
After installation, verify it by checking the version:
git --version
Configuring Git
Once Git is installed, it’s crucial to configure your identity. This information will be associated with your commits. You can set your username and email with the following commands:
git config --global user.name "Your Name"
git config --global user.email "your@example.com"
Setting these configurations ensures that your commits are properly attributed.
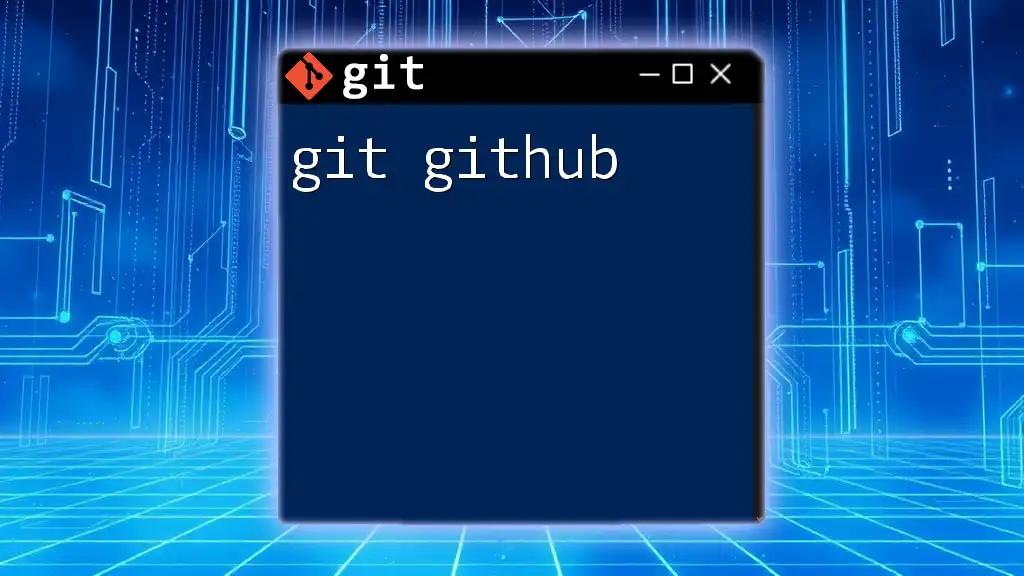
Introduction to GitHub
Creating a GitHub Account
To utilize GitHub, start by creating an account at [GitHub.com](https://github.com). The sign-up process is straightforward and typically involves providing a username, email address, and password. Once your account is set up, you can explore repositories and collaborate with others.
Navigating the GitHub Interface
GitHub provides a user-friendly interface that helps you manage repositories, create issues, submit pull requests, and access settings. Familiarize yourself with the dashboard, which displays your repositories, contributions, and notifications.
Creating a Repository on GitHub
Creating a new repository on GitHub can be achieved through the following steps:
- Click the "+" icon in the upper right corner and select "New Repository."
- Provide a repository name and description.
- Choose to initialize the repository with a README file if desired.
- Set the repository's visibility to public or private.
After creating a repository, you can push your local commits or start a new project directly on GitHub.
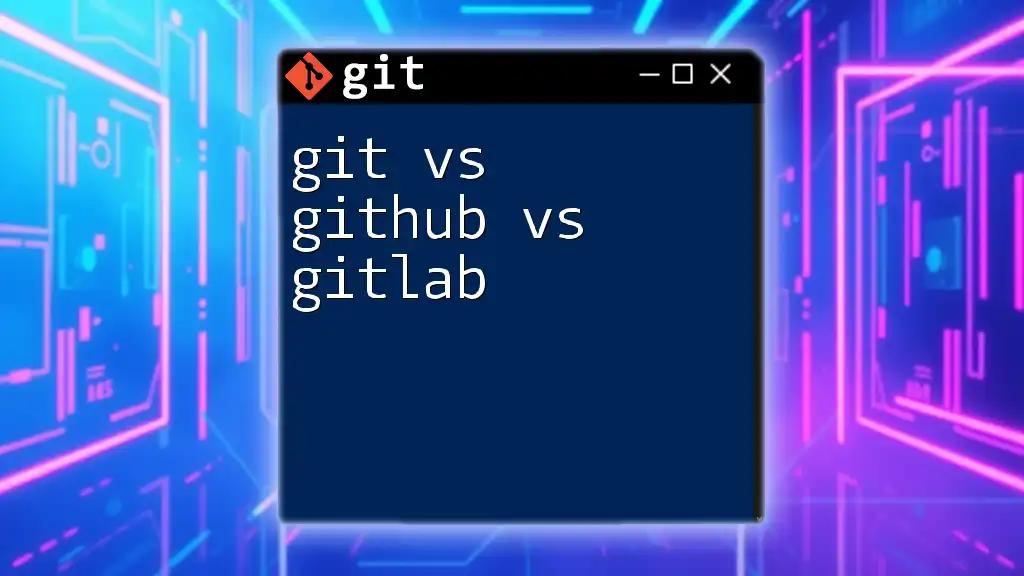
Collaborating with Git and GitHub
Cloning a GitHub Repository
When working on a collaborative project, you'll often need to clone a repository from GitHub. This command copies the repository to your local machine:
git clone <repository-url>
Branching and Pull Requests
Creating and Switching Branches
To avoid conflicts during development, you should create branches for new features or bug fixes. You can create a new branch and switch to it using:
git branch <new-branch-name>
git checkout <new-branch-name>
Feature branches help keep your main codebase stable while allowing you to develop and test new functionalities independently.
Creating a Pull Request
After making changes and committing them to your branch, you can propose merging your changes into the main branch by creating a pull request (PR) on GitHub. This process typically involves:
- Pushing your local branch to GitHub:
git push origin <branch-name>
- Navigating to your repository on GitHub and selecting "Pull requests."
- Clicking "New pull request," selecting your branch, and following the prompts to complete the process.
Pull requests enable code review and discussions, making collaborative development more structured and efficient.
Resolving Merge Conflicts
Merge conflicts occur when multiple contributors make conflicting changes to the same line in a file or when one contributor deletes a file that another contributor modified. To resolve conflicts:
- Attempt to merge using:
git merge <branch-name>
-
If there are conflicts, Git will indicate which files need your attention. Open these files, review the conflicting sections, and modify the code manually to resolve the issues.
-
After resolving conflicts, stage the changes and create a new commit.
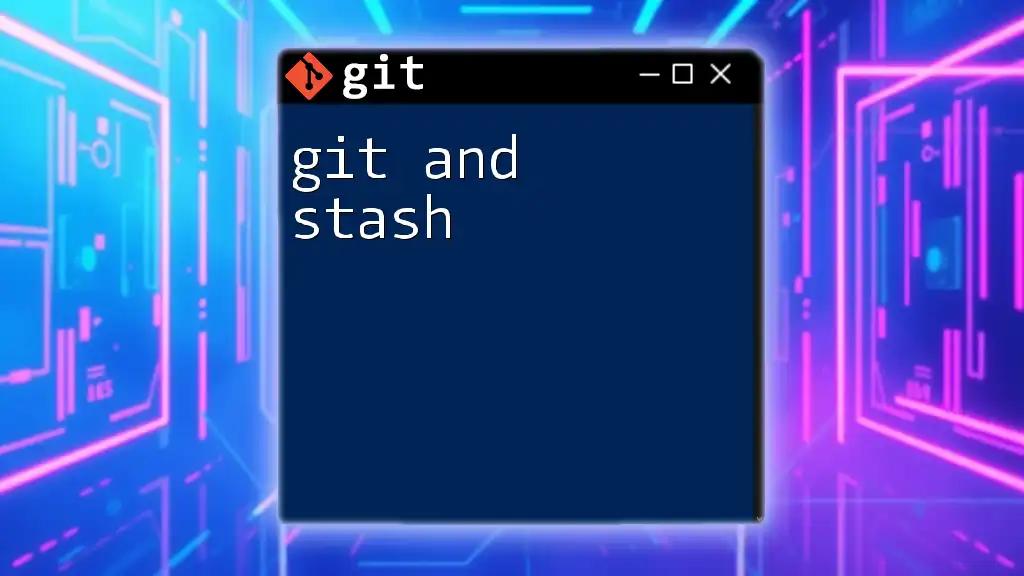
Best Practices with Git and GitHub
Writing Good Commit Messages
Writing meaningful commit messages is crucial for maintaining a clear project history. A good commit message should briefly summarize what was changed and why. Follow this format for clarity:
[type]: [subject]
[body]
[footer]
For example:
feat: add login functionality
Implement user authentication for better security.
Keeping Your Repository Clean
To maintain a clean repository, practice regular merges and delete branches that are no longer in use. After a feature branch has been merged, you can delete it locally with:
git branch -d <branch-name>
This helps reduce clutter and keeps your development focus sharp.
Utilizing Issues and Project Boards
GitHub offers robust issue tracking and project management tools. Use GitHub issues to report bugs, suggest changes, and discuss project features. Create project boards to organize tasks and visualize the development process, making collaboration more effective.
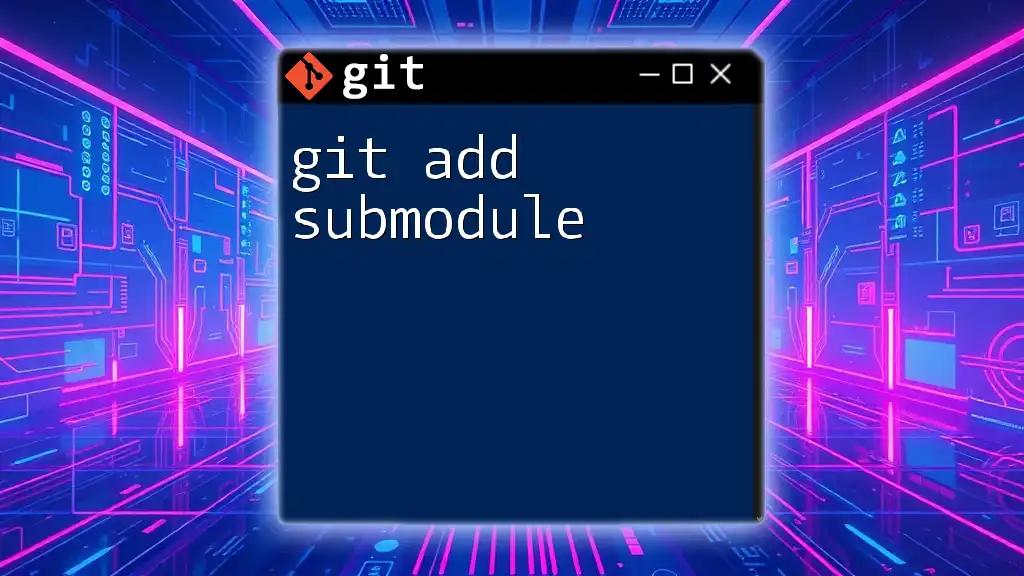
Conclusion
Git and GitHub are vital tools for software developers, enhancing collaboration and ensuring a systematic approach to version control. Mastering these tools empowers developers to contribute to projects effectively while maintaining a clear history of changes.
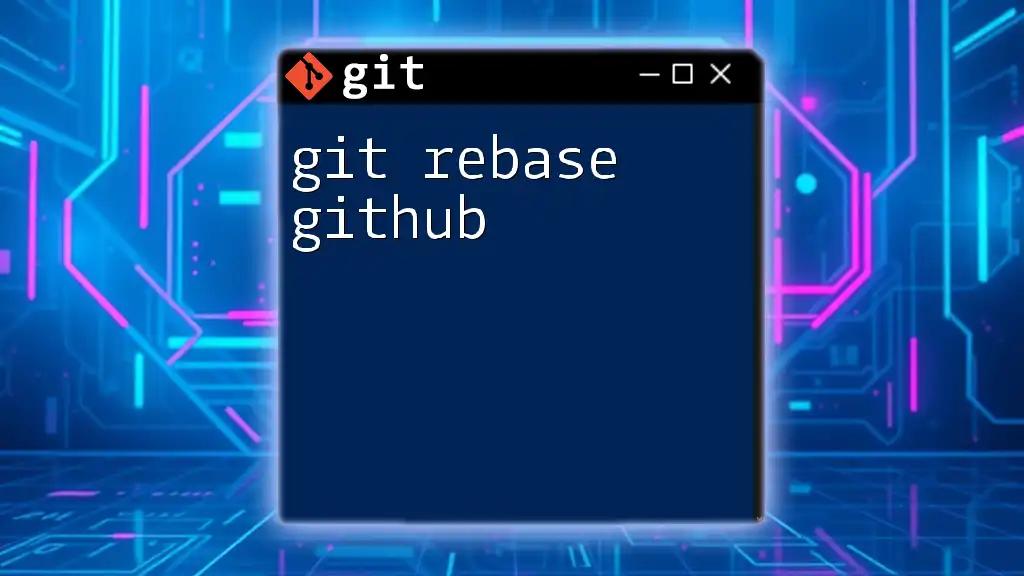
Additional Resources
For further learning, consider exploring the official Git documentation and taking advantage of the GitHub Learning Lab. Along with this, recommended books and online courses can provide deeper insights into mastering Git and GitHub.