GitLab and GitHub are both popular platforms for version control and collaboration using Git, with GitLab offering integrated CI/CD features and self-hosting options, while GitHub is known for its extensive community and marketplace of tools.
Here’s a simple Git command that can be used on both platforms to clone a repository:
git clone https://github.com/username/repository.git
Understanding Git and Version Control
What is Git?
Git is a distributed version control system that allows multiple developers to work on a project concurrently without overwriting each other's contributions. Unlike traditional version control systems, Git enables developers to create branches easily, facilitating features, bug fixes, and experiments without affecting the main codebase.
The importance of Git in software development cannot be overstated. It provides a safety net through version control, allowing for easy retrieval of previous code versions and aiding in debugging. Some key features include:
- Branching: Create isolated environments for features or fixes.
- Merging: Combine changes from different branches, integrating various contributions seamlessly.
- Distributed Architecture: Every developer has a complete copy of the repository, enhancing collaboration and redundancy.
Why Use a Version Control System?
Implementing a version control system (VCS) is a foundational practice in software development. The benefits are numerous:
- Collaboration: Multiple developers can work on the same project simultaneously without conflicting changes.
- Code History: Developers can track changes over time, making it easier to revert to previous states if necessary.
- Branching and Merging: Effortlessly manage features, bug fixes, and tasks in a separate environment.
Real-world example: Consider a team that's developing a web application. With Git, one developer can work on implementing a new feature in a branch, while another can address a critical bug in the main codebase. Once ready, they can merge their changes, maintaining a clean workflow.
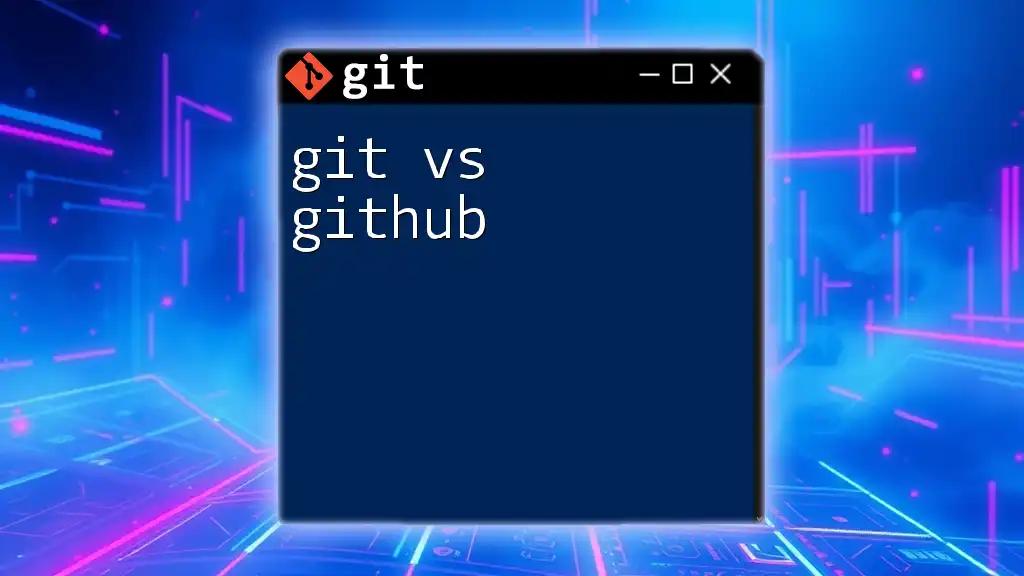
Overview of GitHub and GitLab
What is GitHub?
GitHub is a cloud-based platform that provides hosting for Git repositories and a wide array of tools for project management and collaboration. Established in 2008, it quickly became a hub for developers, especially for open-source projects.
Key features of GitHub include:
- Repository Hosting: Store Git repositories in the cloud.
- Issues Tracking: Manage tasks, features, and bugs with an integrated issue tracker.
- Pull Requests: Facilitate code reviews and discussions before merging changes.
- Community and Open-source Contributions: Access to a vast community supporting millions of open-source projects.
What is GitLab?
GitLab is an integrated DevOps platform that provides both repository management and a suite of tools for the entire software development lifecycle. Launched around the same time as GitHub, it has steadily gained traction with its robust features.
Key features of GitLab include:
- Built-in CI/CD: Continuous Integration and Continuous Deployment tools are deeply embedded within the platform, enhancing the development workflow.
- Issue Tracking: Offers a flexible issue tracker that allows for customizing workflows.
- Merge Requests: Similar to pull requests, designed to facilitate code reviews and discussions.
- Self-hosted Option: Allows organizations to run GitLab on their servers for enhanced control over their data and environment.
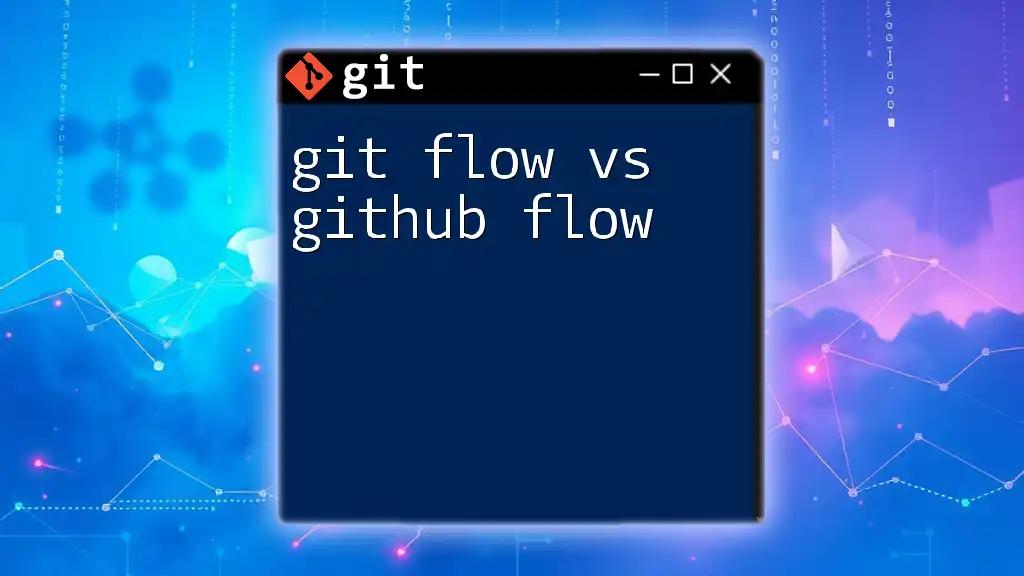
Feature Comparison: GitHub vs GitLab
User Interface and Usability
The user interface plays a crucial role in daily operations. GitHub is often praised for its clean, intuitive UI that simplifies navigating projects. Users can quickly create repositories and manage branches from the dashboard.
In contrast, GitLab offers a more comprehensive interface that integrates various DevOps tools directly into the experience. While it may appear more complex initially, it provides robust functionalities that are valuable for larger teams.
Example: Navigating the dashboards, GitHub displays projects in a straightforward list format, while GitLab presents a more data-rich environment with metrics and actionable insights.
Collaboration Tools
Code Review and PRs
GitHub's Pull Requests offer a streamlined process for reviewing code changes. Developers can comment on lines of code, suggest changes, and approve merges.
GitLab's Merge Requests serve a similar purpose but include additional features like inline commenting, which makes discussions more focused.
Code Snippet: Example of a simple pull/merge request process
# Creating a branch
git checkout -b feature/new-feature
# Making changes and committing
git add .
git commit -m "Add new feature"
# Pushing the branch
git push origin feature/new-feature
Issue Tracking
Both platforms include issue tracking, but with distinct differences.
- GitHub: Users can create issues tied to specific commits, branch discussions, and pull requests.
- GitLab: Users can customize issue labels, milestones, and assignees greatly enhancing project management capabilities.
Example: Creating an issue in GitHub involves filling out a simple form, while GitLab allows for customizable templates and workflows in issue management.
Continuous Integration and Deployment
GitHub Actions
GitHub Actions provide a flexible CI/CD framework that allows users to automate workflows directly within their repositories.
Example of a GitHub Actions setup:
name: CI
on:
push:
branches:
- main
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Run tests
run: npm test
GitLab CI/CD
GitLab boasts a fully integrated CI/CD system right in its core functionality. This feature emphasizes using `.gitlab-ci.yml` files stored at the root of a repository to define the CI pipeline.
Example of a simple `.gitlab-ci.yml` file:
image: node:latest
stages:
- test
test_job:
stage: test
script:
- npm install
- npm test
The built-in CI/CD in GitLab often becomes a deciding factor for teams looking for a more collaborative environment that encompasses both repository management and deployment solutions.
Project Management Features
When it comes to project management, GitHub offers boards similar to a Kanban system, mainly focused on tracking issues and tasks. Milestones can be created to group issues, but can feel limited compared to GitLab.
GitLab, however, provides a more comprehensive experience with built-in project management features. Users can create epics, track burn-down charts, and manage multiple projects under one roof, making it ideal for larger organizations.
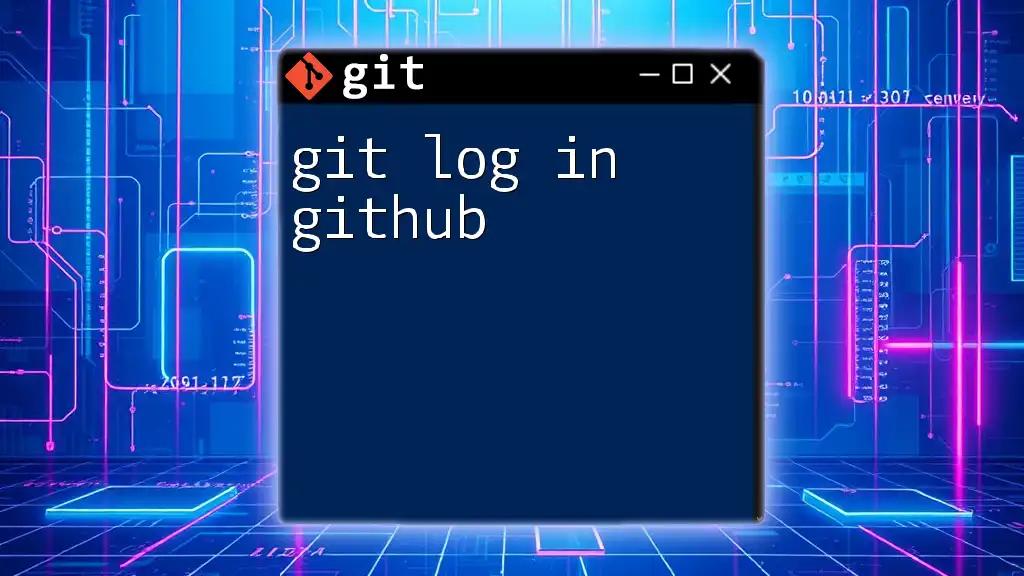
Pricing and Plans
GitHub Pricing Tiers
GitHub offers various pricing tiers, from free accounts with unlimited public repositories to paid plans that include features like advanced collaboration tools and private repositories.
Free Tier Features:
- Unlimited public and private repositories
- Basic collaboration tools
- GitHub Actions limited capabilities
Paid Plans:
- Advanced team features
- Enhanced security options
- More CI/CD minutes for automation
GitLab Pricing Models
GitLab also provides a free tier that provides unlimited features but includes limited CI/CD minutes. Paid plans unlock advanced capabilities like enterprise-level support, enhanced security features, and additional storage.
Unique features of GitLab’s pricing model include:
- Comprehensive DevOps features even in the free tier.
- Flexible billing options for larger teams or enterprises.
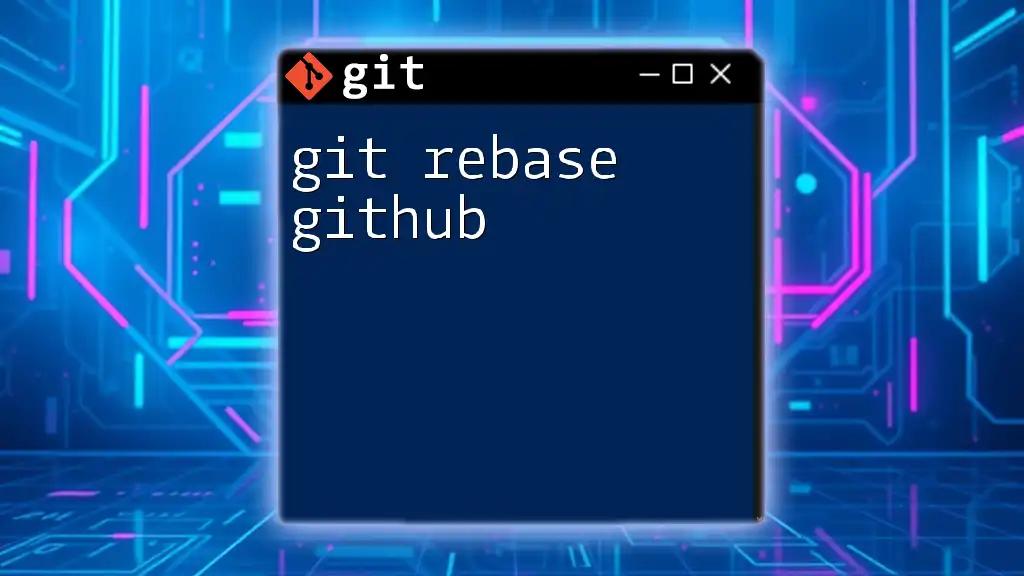
Community and Support
GitHub Community
GitHub has cultivated a thriving community with millions of developers worldwide. This ecosystem fosters collaboration and encourages contributions to open-source projects. Besides, extensive documentation and community resources are readily available, making troubleshooting easier.
GitLab Community
Similarly, GitLab promotes an active community contributing to its growth. With numerous resources, including forums and guides, users find support and collaboration opportunities. GitLab’s commitment to open source contributes to a sense of community ownership and engagement.
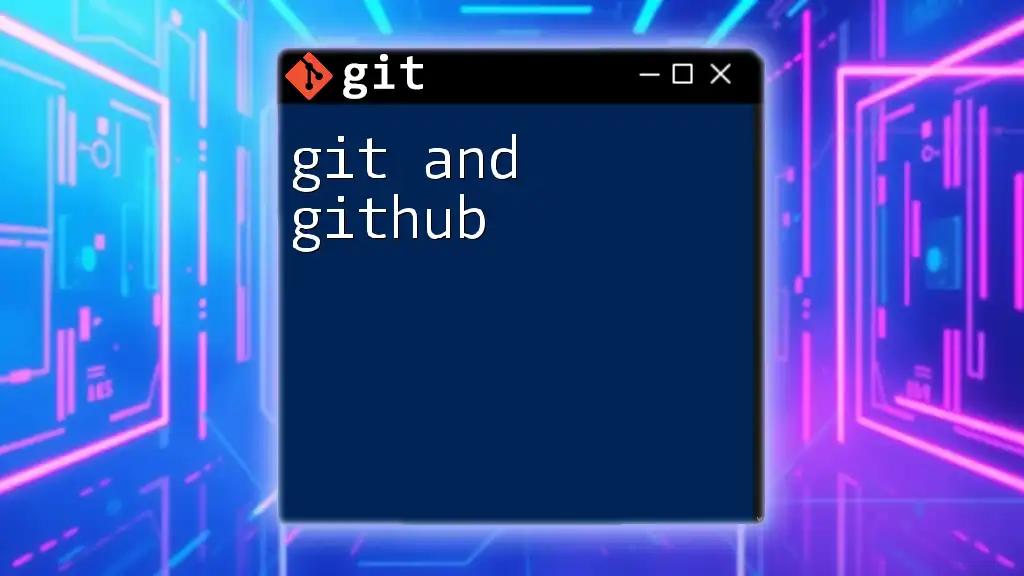
Use Cases: When to Choose GitHub vs GitLab
Choosing GitHub
GitHub stands out when:
- You're primarily focused on open-source projects.
- You desire a large community for collaboration and contributions.
- You prefer a simpler user experience for smaller teams.
Examples of popular projects on GitHub include libraries and frameworks like React, TensorFlow, and many others that rely on community engagement.
Choosing GitLab
Consider GitLab when:
- Your team requires integrated CI/CD pipelines in your workflow.
- You prefer a more robust project management system.
- You need capabilities to self-host the application for better control.
Examples of successful teams using GitLab include companies needing complex project workflows and robust security features for sensitive projects.
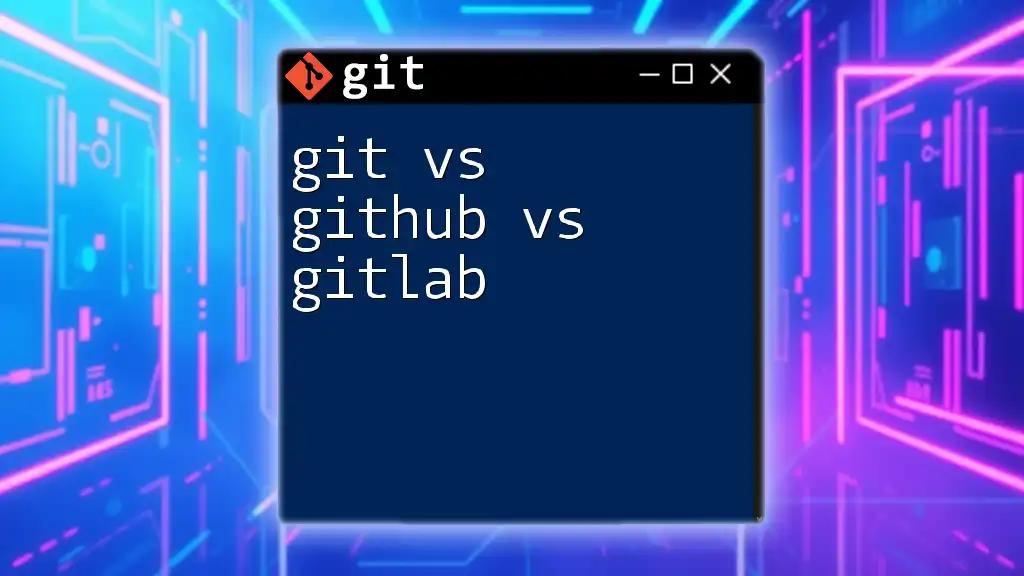
Security Features
GitHub Security
GitHub offers a variety of security features designed to protect code and manage vulnerabilities. These features include dependency graphs to identify outdated libraries, secret scanning to prevent sensitive data exposure, and automated security updates.
GitLab Security
In contrast, GitLab places a strong emphasis on integrated security features. Its SAST/DAST integrations provide comprehensive security testing, and users can manage permissions meticulously to minimize risk. This makes GitLab an appealing choice for organizations prioritizing security.
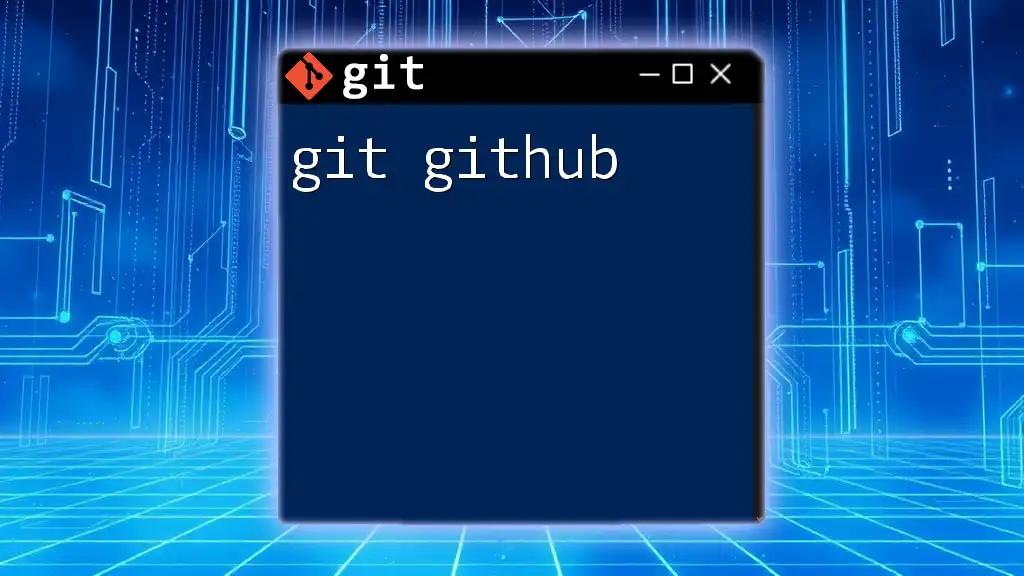
Conclusion
In summary, when comparing GitLab vs GitHub, both platforms present unique advantages and features tailored to different team needs and workflows. While GitHub shines with its community and simplicity, GitLab excels with its integrated capabilities and comprehensive project management. Ultimately, the best choice will depend on your specific requirements, project complexity, and team dynamics.
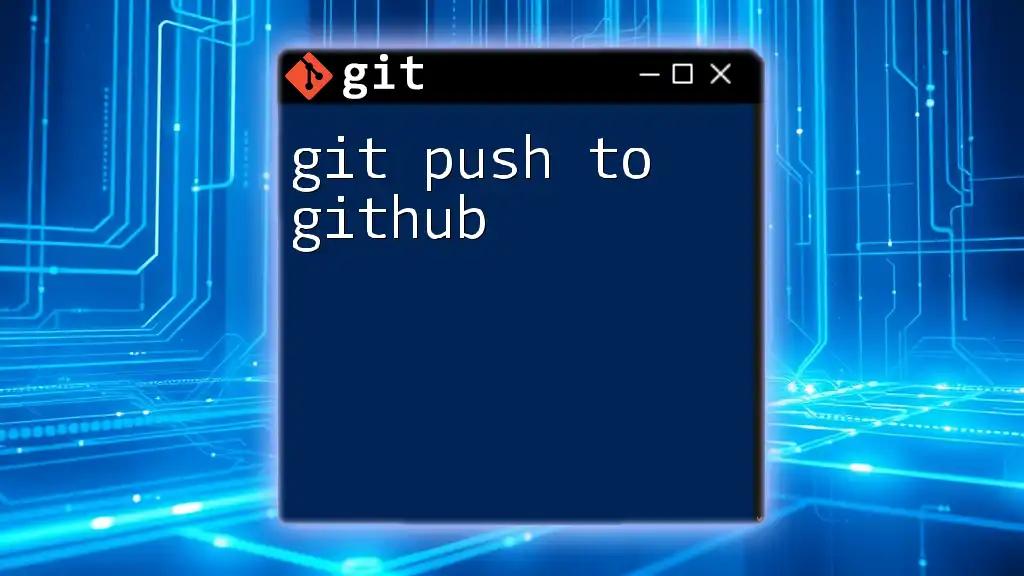
Frequently Asked Questions (FAQs)
Is GitHub better than GitLab?
Determining which platform is better varies greatly based on individual project needs. GitHub is often favored for open-source projects due to its large community, while GitLab appeals to organizations seeking comprehensive DevOps solutions and enhanced security.
Which is more suited for open-source projects?
GitHub is typically considered more suited for open-source projects due to its extensive support network and visibility, allowing easier collaboration and contribution.
Can I migrate from GitHub to GitLab?
Migrating repositories from GitHub to GitLab is feasible and generally involves using GitLab's import tools or performing manual Git operations to transfer code and history.
How can I integrate other tools with GitHub/GitLab?
Both GitHub and GitLab support a wide range of integrations with third-party tools, helping extend functionalities for project management, CI/CD, and error tracking.
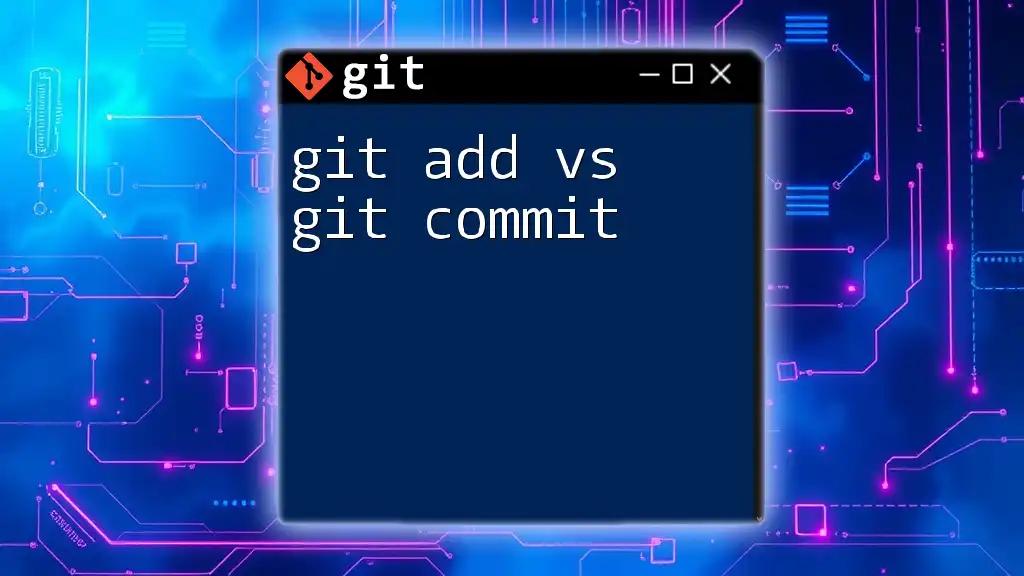
Additional Resources
- [GitHub Documentation](https://docs.github.com/)
- [GitLab Documentation](https://docs.gitlab.com/)
- Recommended training tutorials for mastering Git commands and workflows.
This comprehensive analysis of GitLab vs GitHub should guide developers in choosing the right platform for their project needs. Whether you opt for GitHub or GitLab, knowing their strengths will significantly enhance your development workflow.