Git is a version control system for tracking changes in code, GitHub is a cloud-based platform for hosting and collaborating on Git repositories, while GitLab is a similar platform that also offers integrated CI/CD tools, enabling both private and public project hosting.
git clone https://github.com/username/repository.git
Understanding Git
What is Git?
Git is a distributed version control system designed to handle everything from small to very large projects with speed and efficiency. Created by Linus Torvalds in 2005, Git allows multiple developers to work on a project simultaneously without overwriting each other's contributions. This collaborative environment is a cornerstone of modern software development, making Git a vital tool in any developer's toolbox.
Key Features of Git
Distributed Version Control means that every developer has a complete copy of the repository, including its history. This setup offers several advantages:
- Speed: Most operations are performed locally, which avoids the latency of network connections.
- Flexibility: Developers can work offline, making changes and commits without needing immediate access to a central server.
Local and Remote Repositories are critical to understanding Git's functionality. A local repository resides on your own machine, while a remote repository (commonly hosted on platforms like GitHub or GitLab) enables collaboration among developers.
Branching and Merging are among Git's most powerful features. Branches allow you to isolate changes related to new features, fixes, or experiments without affecting the main project. When features are complete, you can merge these branches back into the main branch.
# Create a new branch
git checkout -b new-feature
# Merge the branch back to the main branch
git checkout main
git merge new-feature
This flexibility allows teams to innovate without risking the stability of the main codebase.
Common Git Commands
Initialization and Setup are the first steps when starting a new project. You can create a new repository with the following command:
git init
This command initializes a new Git repository in your current directory.
Staging Changes is the next crucial step. By using the `git add` command, you can prepare files for committing. You can stage individual files or all files at once:
# Stage a specific file
git add filename.txt
# Stage all changes
git add .
Committing Changes is where you save your staged changes to the repository. A good commit message is essential to communicate the purpose of the changes:
git commit -m "Add new feature for user login"
This command saves your changes and adds a helpful message that will be part of the project’s history.
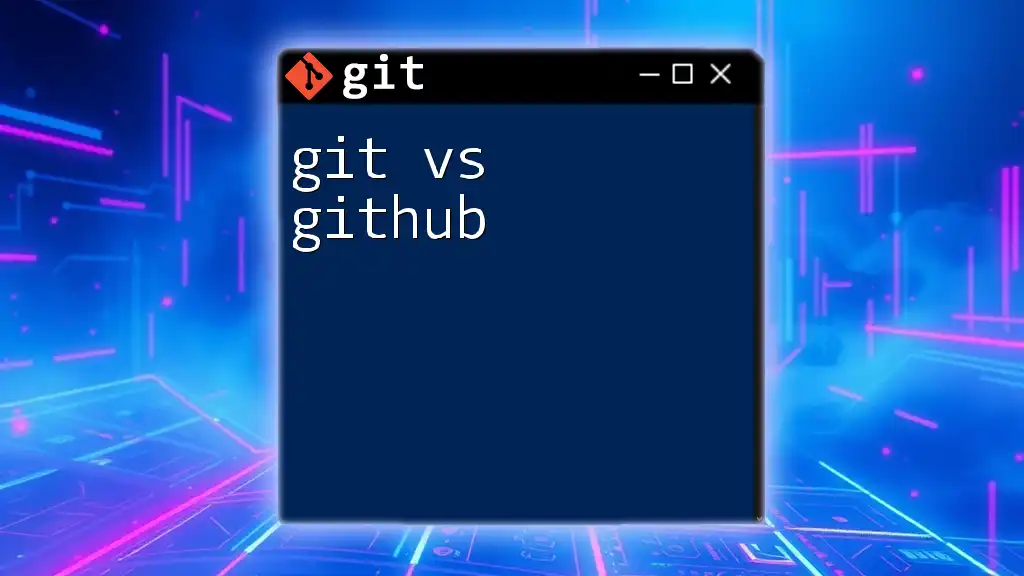
Introducing GitHub
What is GitHub?
GitHub is an online platform that uses Git to provide hosting for software development projects. It plays a significant role in version control, offering additional collaborative tools that enhance teamwork. Founded in 2008, GitHub has grown to be the most popular place for developers to share and contribute to coding projects globally.
Key Features of GitHub
Collaboration Tools are at the forefront of GitHub's utility. Two of the standout features are:
- Pull Requests: Allow contributors to propose changes to a project. Other developers can review these changes, discuss potential modifications, and approve them before they are merged into the main codebase.
- Code Review: Enhances code quality by facilitating discussions among developers about changes and improvements.
Project Management features, such as issues, milestones, and project boards, help teams track bugs, plan new features, and monitor progress effectively. For example, you can create issues to report bugs or request enhancements for better project maintenance.
GitHub Actions provides a powerful framework for running automated workflows. CI/CD (Continuous Integration/Continuous Deployment) capabilities streamline the development process. For example, you can automate builds and tests whenever code is pushed to the repository.
How GitHub Works with Git
Git and GitHub work hand-in-hand, enhancing the developer's ability to work collaboratively. After making changes locally, you can push those changes to your GitHub repository using:
git push origin main
This command sends your code to the remote repository, making it accessible to your team members.
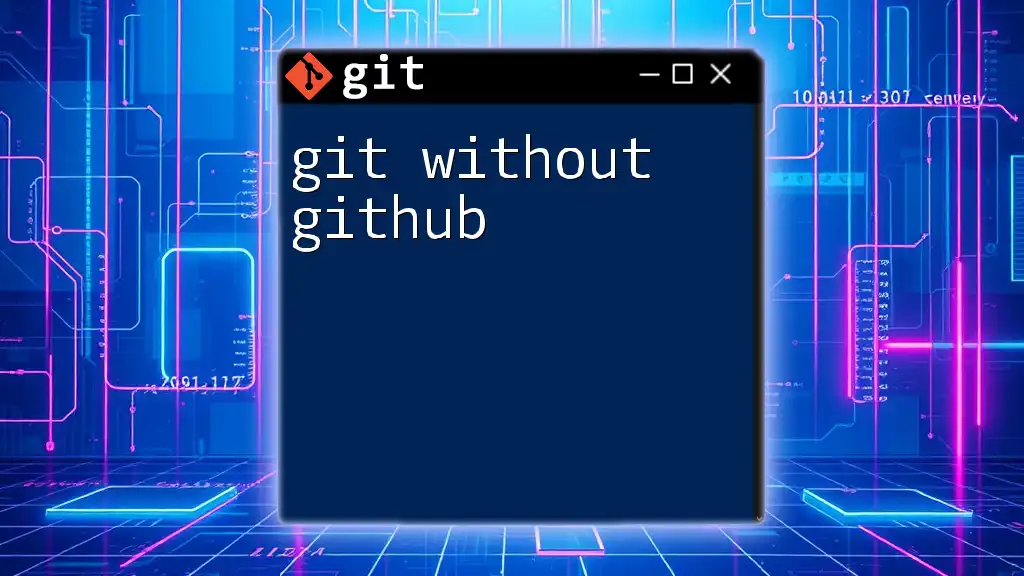
Introducing GitLab
What is GitLab?
GitLab is another web-based Git repository manager that provides features similar to GitHub, but also expands further into the realm of DevOps lifecycle management. Since its launch in 2011, GitLab has gained popularity among developers looking for an integrated environment that supports the entire software development process.
Key Features of GitLab
Complete DevOps Lifecycle is a hallmark of GitLab's offering. It combines version control with other tools, enabling seamless CI/CD processes directly integrated into your repositories. Unlike GitHub, GitLab incorporates built-in CI/CD functionality that allows you to automate testing and deployment without needing third-party tools.
Code Review Tools in GitLab facilitate collaboration through merge requests. These allow developers to propose their changes and request a review before merging into the main codebase, making it easier for teams to maintain code quality.
How GitLab Works with Git
GitLab also offers a close relationship with Git, working efficiently as a hosting environment for Git repositories. For instance, a simple `.gitlab-ci.yml` file can define the CI/CD pipeline like this:
stages:
- build
- test
- deploy
build_job:
stage: build
script:
- echo "Building the application..."
test_job:
stage: test
script:
- echo "Running tests..."
deploy_job:
stage: deploy
script:
- echo "Deploying the application..."
This configuration automates the build, test, and deployment stages each time changes are pushed to the repository.
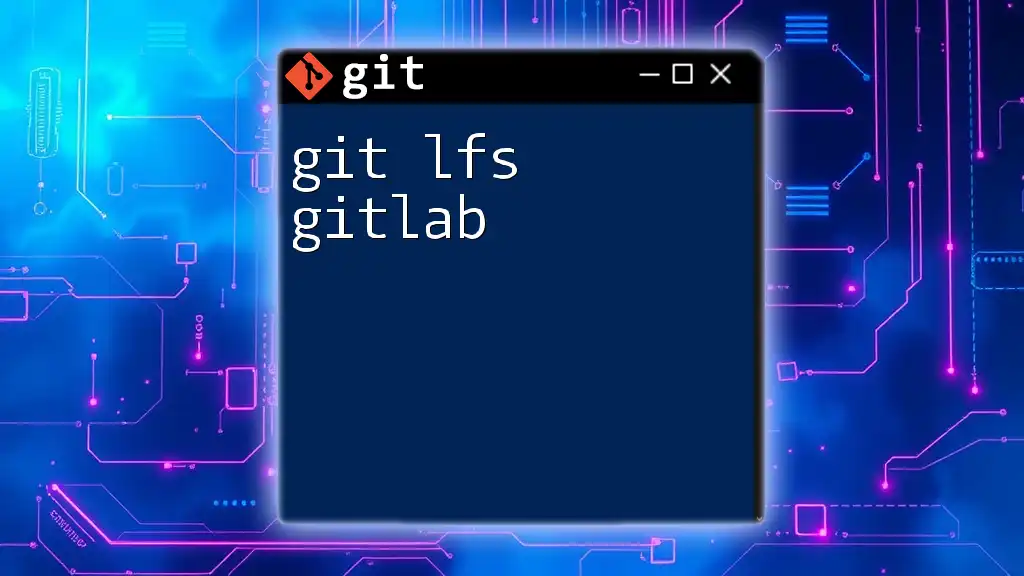
Comparing Git, GitHub, and GitLab
Overview of Differences
In the git vs github vs gitlab discussion, it's crucial to understand their distinct roles.
- Git is the underlying technology—a distributed version control system that allows you to manage your source code history.
- GitHub and GitLab are hosting platforms that utilize Git for version control and offer a suite of additional features enhancing collaborative development.
Key Features Comparison
While both GitHub and GitLab provide collaboration tools, issue tracking, and code reviews, GitLab stands out with its integrated CI/CD capabilities. GitHub Actions have improved GitHub's CI/CD functionality, but GitLab's built-in features convey a more seamless DevOps experience.
Use Cases for Each
- Git can be utilized independently for personal projects or local development environments without the need for remote hosting.
- GitHub is often the preferred choice for open-source projects and communities that value visibility and collaboration.
- GitLab is better suited for teams focused on stringent DevOps practices, needing tight integration between version control, code review, and deployment processes.
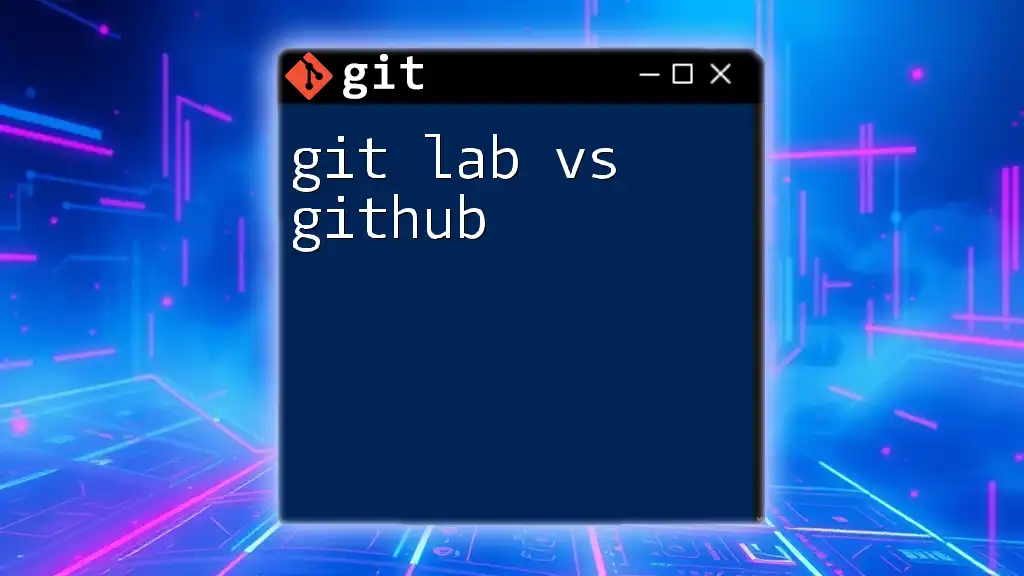
Conclusion
In summary, while Git serves as the foundational tool for version control, GitHub and GitLab enhance collaborative development through their unique features. Choosing between GitHub and GitLab ultimately depends on your specific project needs and team workflows.
FAQs
Is GitHub necessary if I use Git?
While you can use Git independently, platforms like GitHub significantly simplify collaboration, issue tracking, and project management.
Can I use GitLab without Git?
GitLab is built around Git; using GitLab generally requires Git for version control to manage your source code.
Which platform is better for beginners?
For beginners, GitHub might provide a more user-friendly experience, especially with its extensive documentation and large community support. However, GitLab's focus on integrated DevOps can also be beneficial for those interested in learning the full lifecycle of software development.
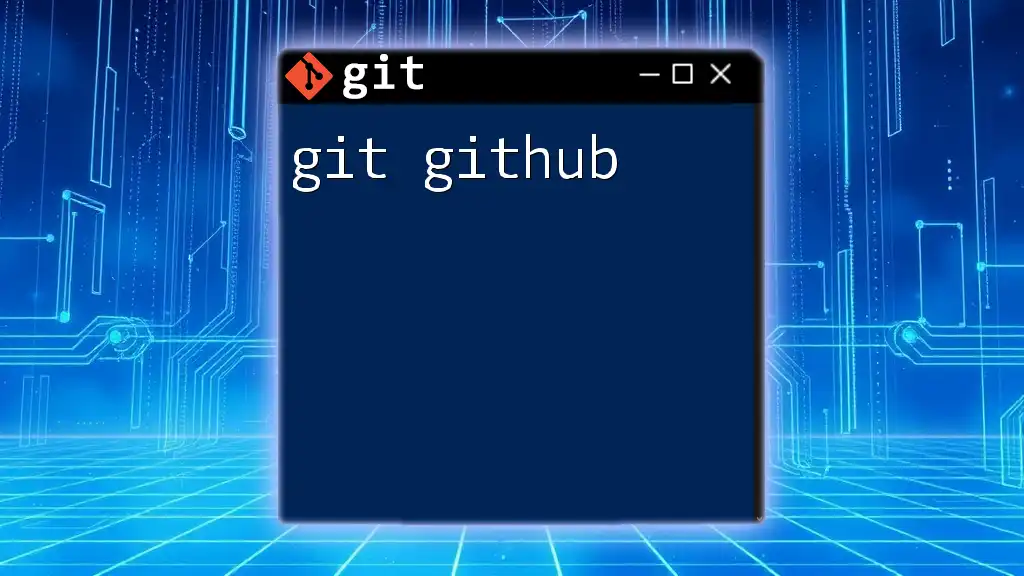
Call to Action
Ready to dive deeper into Git and enhance your version control skills? Explore our courses designed to teach you Git commands in a quick, short, and concise manner! Start mastering your workflow today!