The `git split commit` technique allows you to separate changes from a single commit into multiple commits to better organize your code history.
git reset HEAD^ # Reset to the previous commit, keeping changes in the working directory
git add -p # Interactively split changes and stage them for new commits
git commit -m "Your first commit message" # Commit the first part of changes
git add -p # Stage the next part of changes separately
git commit -m "Your second commit message" # Commit the second part of changes
Understanding Git Split Commit
What is a Split Commit?
A split commit refers to the practice of breaking down changes made to a project's codebase into separate commits. By doing this, each commit can focus on a distinct aspect of the overall work, thus providing clarity and ease of understanding in your project's history.
Why Split Commits Matter
Using split commits has several inherent advantages:
- Improved Project Clarity: When changes are organized into individual commits, it becomes easier to see what specific changes were made at a glance.
- Enhanced Collaboration: Team members can review changes more efficiently, simplifying the process of code reviews and contributions.
- Easier Debugging and Rollback Processes: If an issue arises, it’s simpler to identify when and where a change occurred, allowing for swift resolutions.
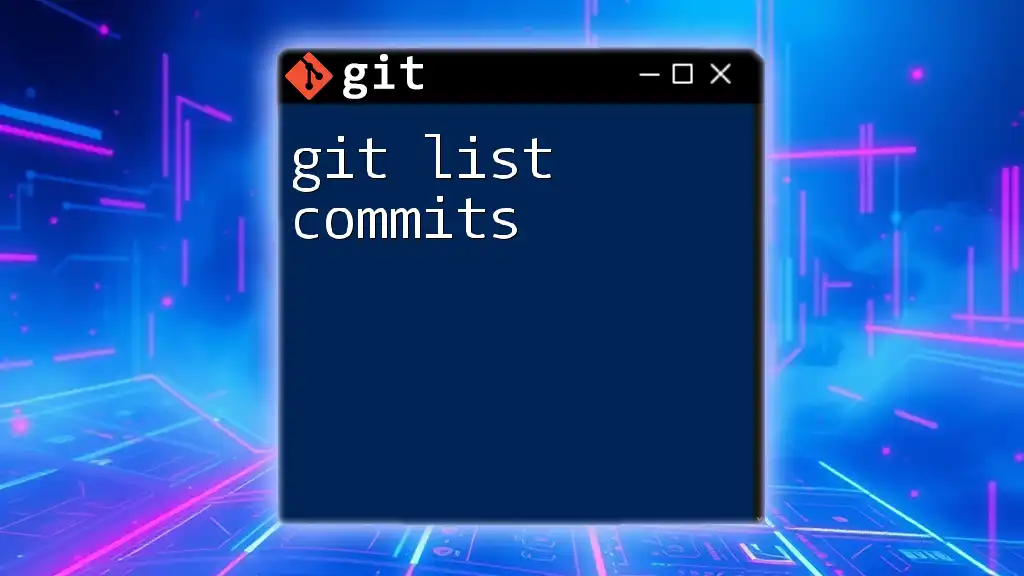
The Git Split Commit Process
Overview of the Split Commit Workflows
The workflow for executing a split commit can typically be broken down into three parts:
- Preparing changes: Ensuring your working directory is clean and identifying what needs to be committed.
- Splitting commits: Staging and committing changes separately based on the features or fixes they pertain to.
- Organizing your Git history: Leveraging tools like interactive rebase to refine commit history if necessary.
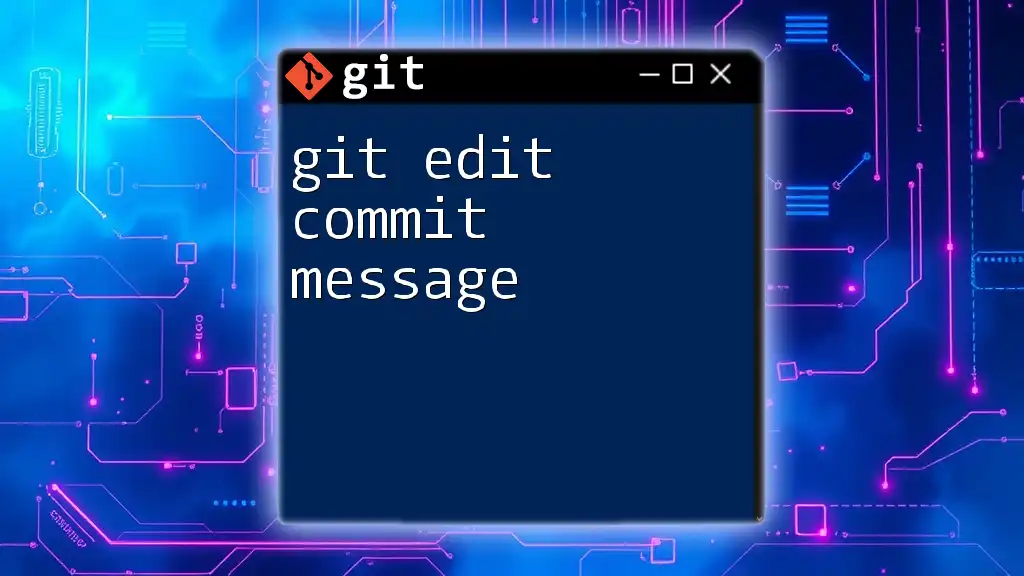
Preparing for a Split Commit
Ensuring Your Repository is Clean
Before you begin splitting your commits, it is crucial to ensure that your working directory is clean. You can check this by running:
git status
This command will provide you with a summary of your current state. Ideally, there shouldn’t be any untracked or unstaged changes.
The Staging Area: What You Need to Know
Understanding the Staging Area
The staging area, also known as the index, is where Git tracks changes that will be included in the next commit. It acts as a buffer between your working directory and your commit history.
Staging Changes Selectively
To stage specific changes selectively, you can invoke the following command:
git add -p
This command allows you to review each change (hunk) in your repository, offering options to:
- stage this hunk
- skip it
- split it further
This level of granularity ensures you're only capturing pertinent changes in each commit.
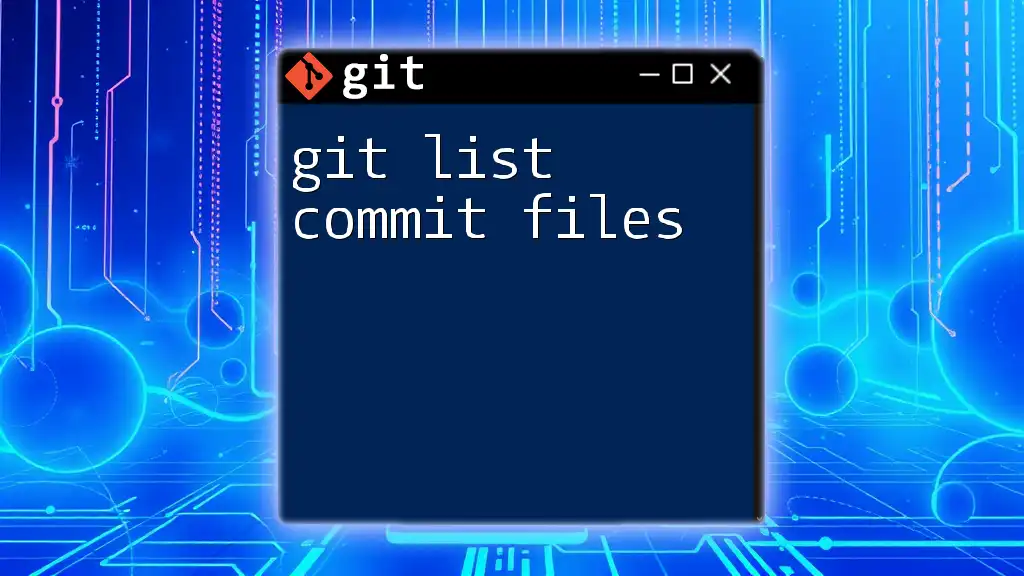
Executing a Git Split Commit
Creating the First Commit
After you’ve staged the appropriate changes for the first part of your feature or fix, you can create the first commit. Here’s how you do it:
git commit -m "First part of the feature"
Writing a clear commit message helps contextualize what the commit achieves.
Creating Additional Commits
How to Stage Remaining Changes
Next, if you have remaining changes to commit, you’ll need to stage those as well. Use the following command:
git add <file-name>
This allows you to stage either whole files or select changes from files, depending on what’s necessary for your next commit.
Committing the Remaining Changes
Finally, you can commit the changes that were staged:
git commit -m "Second part of the feature"
Again, the commit message should reflect the specific changes this commit encompasses.
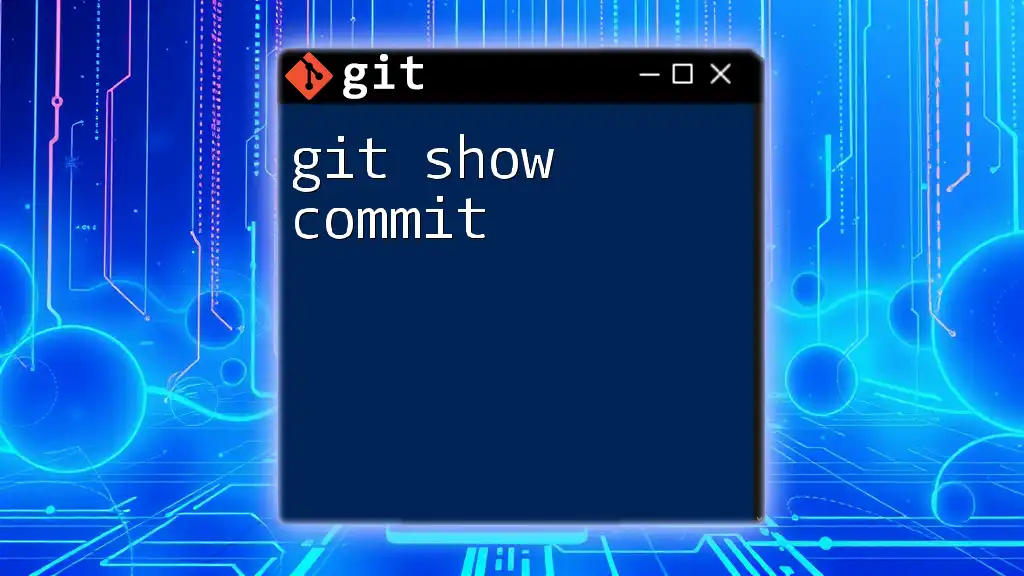
Utilizing Interactive Rebase for Split Commits
Introduction to Interactive Rebase
Interactive rebase is a powerful feature in Git that allows you to rework commits, making it an excellent tool for splitting commits post-factum.
Step-by-Step Guide to Interactive Rebase
Initiate Interactive Rebase
To begin, you can start an interactive rebase of the last two commits, which is useful right after you've already committed work without proper segregation:
git rebase -i HEAD~2
This opens an editor where you can manipulate the commits.
Marking Commits to Edit
In the text editor, change `pick` to `edit` for the commits you intend to split. This tells Git that you would like to amend those commits.
Editing Commits
Once you’ve marked the commit to edit, Git pauses the rebase process allowing you to amend the specific commit:
git commit --amend
Make your changes in this commit, then save and exit.
Continuing the Rebase
After amending the first commit, you need to continue the rebase process. Use:
git rebase --continue
This commits your changes and leads you back into the rebase process if there are additional commits marked for editing.
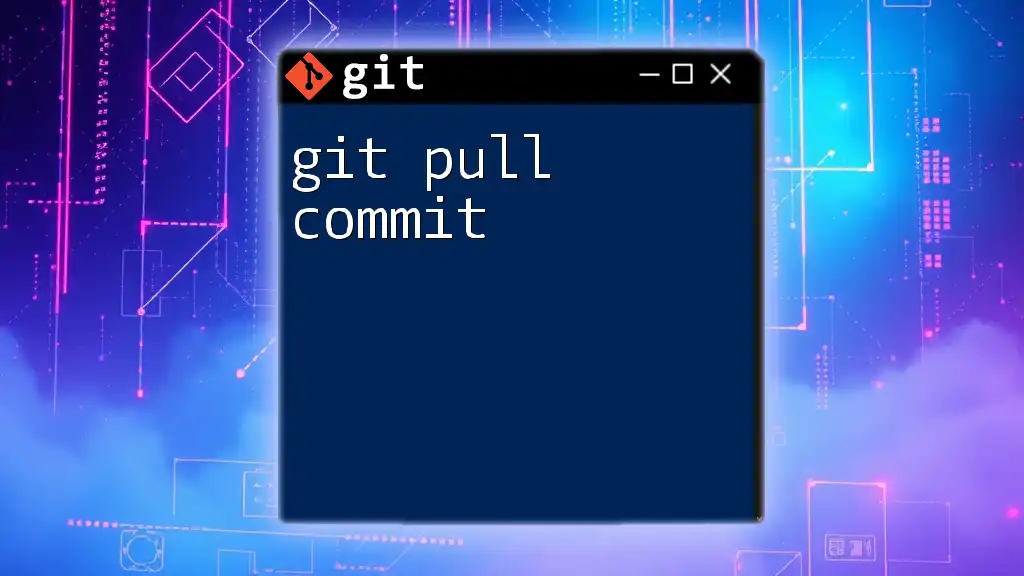
Best Practices for Split Commits
When to Split Commits
Understanding when to split commits is critical. Common scenarios include:
- Implementing a new feature with several components.
- Fixing multiple bugs in one area of the codebase.
Naming Your Commits
Well-defined commit messages are essential. They should be concise yet descriptive enough to convey the purpose of the changes, aiding other developers (and your future self) in grasping the project timeline.
Keeping History Clean
Aim to maintain a clean Git history. This means organized, coherent, and purposeful commit messages and avoiding commits that are too large or poorly defined.
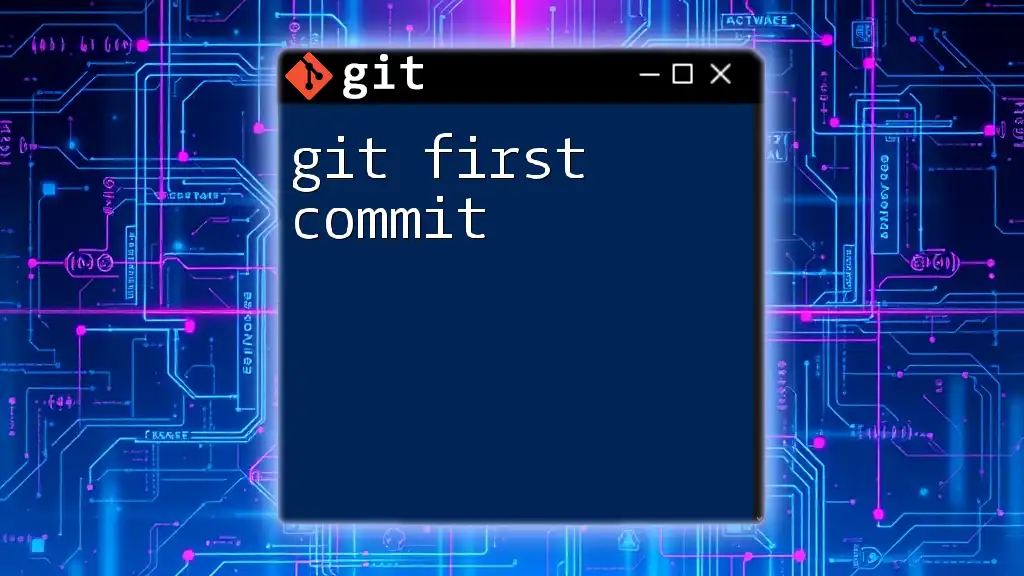
Problem-Solving with Split Commits
Common Issues and Solutions
Unstaged Changes After Committing
If you find out that you’ve forgotten to stage changes after making a commit, confirm your changes with:
git status
If you missed them, you can stage and commit those changes immediately afterward.
Overcommit Issues
If you've committed changes that need to be split or altered due to oversight, you can reset the last commit using:
git reset HEAD~1
This returns your last commit to the staging area, allowing you to re-stage as necessary.
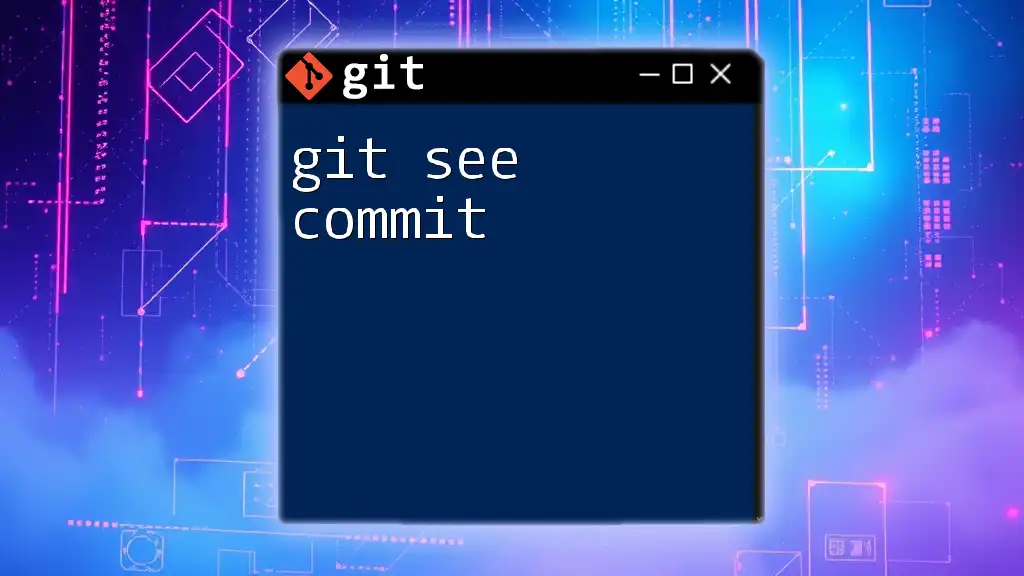
Conclusion
Summary of Git Split Commit Benefits
Through the use of split commits, developers can maintain a more organized codebase, making it easier to navigate, review, and rollback changes when needed.
Further Learning Resources
For those looking to expand their Git skills, consider exploring official documentation, dedicated Git tutorials, and community discussions to deepen your knowledge.