The command `git abort commit` is not a valid Git command, but if you want to abort a commit that is in progress (for example, during an interactive rebase or after running `git commit` with the `--amend` option), you can use:
git reset --soft HEAD~1
This will undo the last commit without affecting your changes in the staging area.
Understanding Git and Its Commit Process
What is Git?
Git is a powerful version control system that helps developers manage their code changes efficiently. It allows multiple individuals to work on the same project simultaneously without interfering with each other's work. By tracking every modification made to files, Git ensures that the complete revision history is preserved, facilitating easier error correction and collaboration.
The Commit Process in Git
In Git, a commit serves as a snapshot of your changes at a specific point in time. When you commit changes, you are essentially recording your modifications to the repository along with an accompanying message that describes the changes made. The commit process often involves two stages:
- Staging: This is where you select which changes to include in your next commit. Use the `git add` command to stage individual files or sets of changes.
- Committing: Once you are satisfied with the changes in the staging area, you execute the `git commit` command to finalize those changes and add them to the project history.
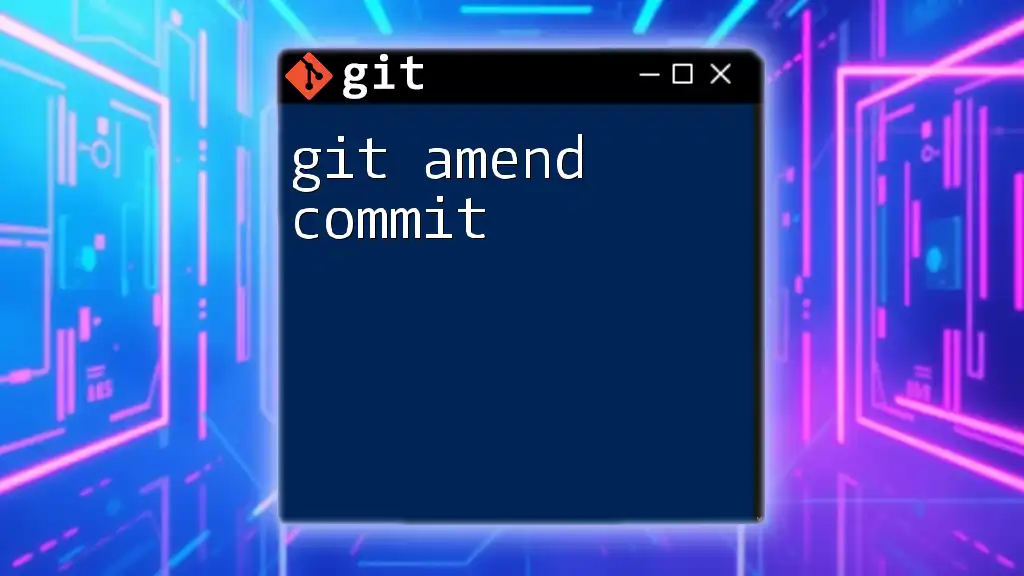
When Do You Need to Abort a Commit?
Common Scenarios for Aborting a Commit
There are scenarios when you may realize that your commit isn't ready to be finalized. For instance:
- You may have mistyped your commit message or made it unclear.
- You might accidentally include unwanted changes, such as files that were not meant to be committed.
- You may determine that the code needs further revisions before it's suitable for a commit.
In such cases, knowing how to "git abort commit" can save you from potential issues.
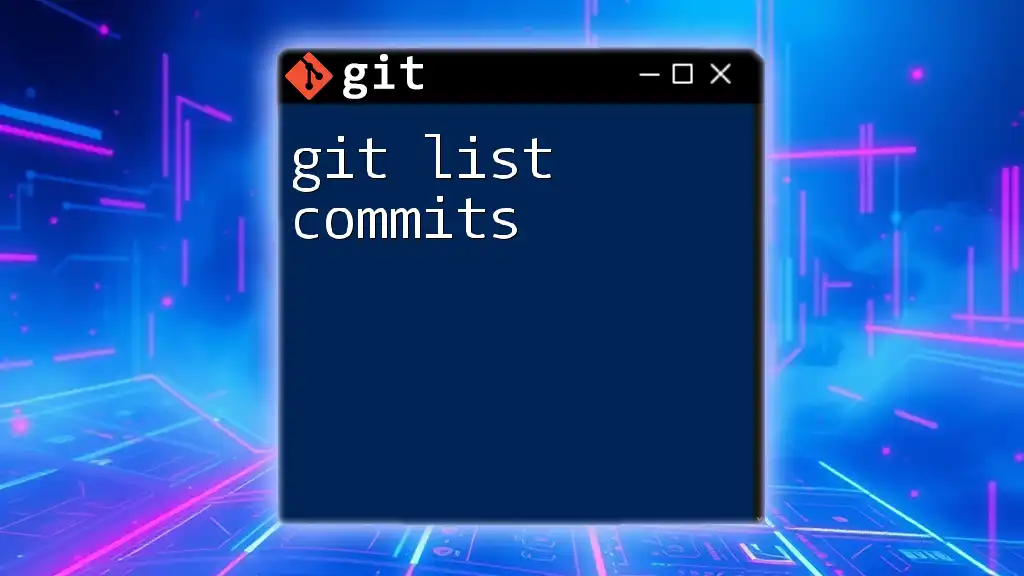
How to Abort a Commit Safely
Using git reset
When it comes to aborting your last commit, one of the most useful commands is `git reset`. This command allows you to revert the last commit while retaining your changes in the staging area.
Command: `git reset --soft HEAD~1`
This command undoes the last commit but keeps your changes staged, ready for more adjustments or modifications.
Code Snippet
git reset --soft HEAD~1
With this command, you'll have a chance to recompose your commit message or add/remove files from the commit before trying again.
Using git restore
If you want to unstage specific files rather than the entire commit, the `git restore` command is your friend.
Command: `git restore --staged <file>`
This command allows you to remove a file from the staging area without altering the version in your working directory. This is particularly useful when you realize a file should not have been included in the last commit.
Code Snippet
git restore --staged <file>
Using this command ensures that you can adjust your staging area to reflect only the changes you want to commit.
Discarding Changes with git checkout
In situations where you want to discard changes you’ve made to a file since your last commit completely, you can use the `git checkout` command.
Command: `git checkout -- <file>`
This command allows you to restore the file to its last committed state, wiping out any modifications made after.
Code Snippet
git checkout -- <file>
Be cautious: this action is irreversible, as any unsaved changes will be lost, thus it's best used when you are certain of the need to discard modifications.
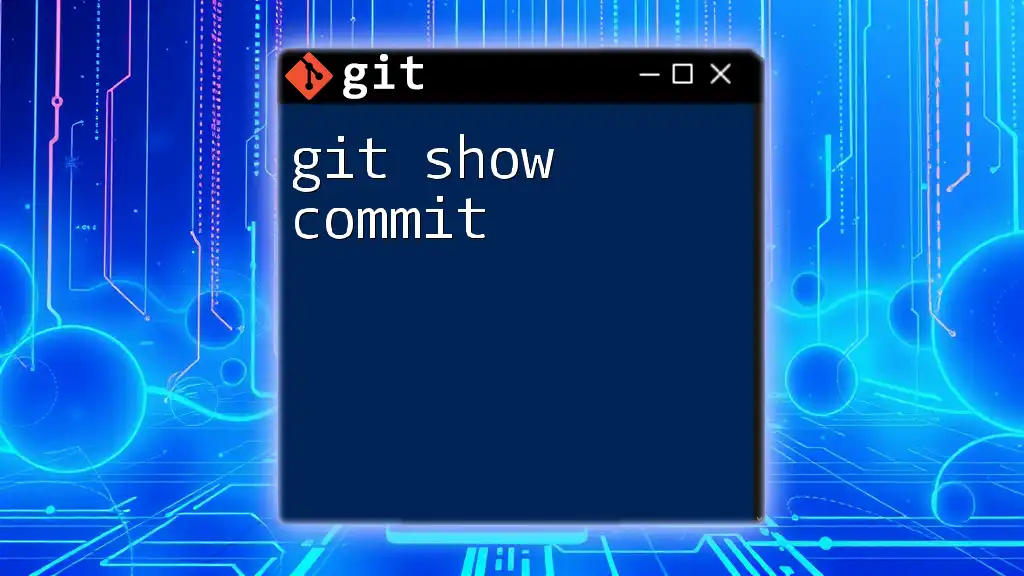
Aborting a Commit in Interactive Rebase
What is Interactive Rebase?
The interactive rebase feature in Git allows you to reorder, squash, or edit commits in your branch history. It is a powerful tool for revising commits that you have already made.
Aborting a Commit During Interactive Rebase
If you encounter issues during an interactive rebase, the command `git rebase --abort` allows you to exit the rebase process without altering your working directory.
Command: `git rebase --abort`
This command is especially useful if you're trying to address conflicts and determine that you need to backtrack.
Code Snippet
git rebase --abort
Using this command will return your branch to its original state, effectively allowing you to start your rebase process anew without losing any work.
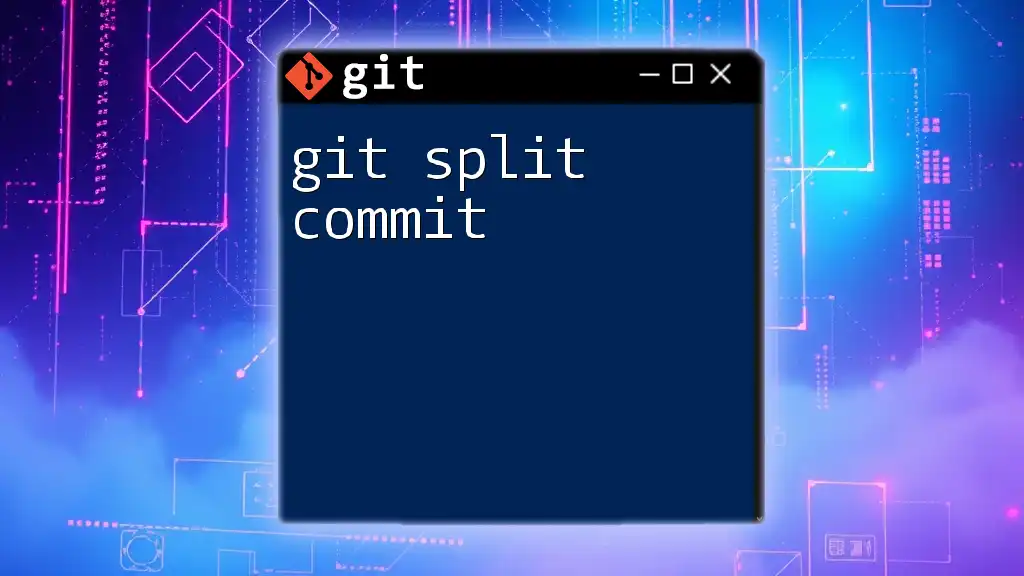
Tips for Avoiding the Need to Abort Commits
Write Meaningful Commit Messages
To minimize the chance of needing to abort a commit, always draft clear and concise commit messages that accurately describe the changes made. This practice not only helps you in the long run but is also beneficial for your team members who might read your commit history.
Review Changes Before Committing
By using commands like `git diff` and `git status`, you can review your changes before committing. This review process allows you to catch mistakes early, avoiding the need to abort commits.
Commit Smaller Changes
Focusing on committing smaller, discrete sets of changes instead of larger updates can lead to a clearer commit history. It minimizes the likelihood of including unwanted changes, making aborting commits less necessary.
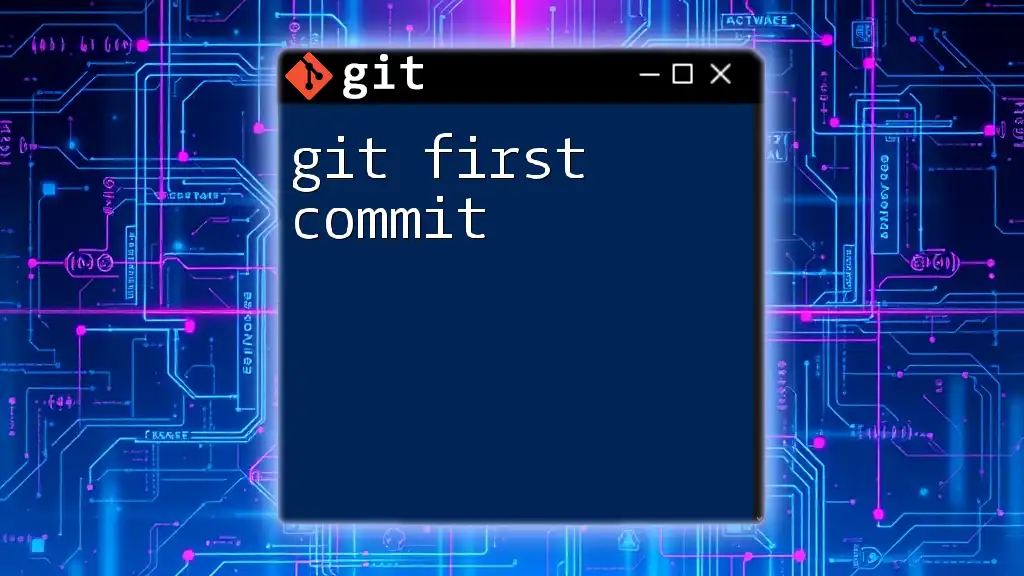
Conclusion
Recap of Key Points
In this guide, we explored various strategies for "git abort commit," including using `git reset`, `git restore`, and `git checkout`. Additionally, we discussed the importance of thoughtful commit practices and how to effectively manage your commit history through interactive rebase.
Encouragement to Practice
Practicing these commands can greatly enhance your Git skills and streamline your development workflow. The more you familiarize yourself with these options, the quicker and more efficiently you'll handle your source code.
Call to Action
Explore further Git commands and enroll in workshops offered by our company to master the art of version control. Empower yourself to manage your coding projects with confidence!