To list the files changed in a specific commit in Git, you can use the following command:
git show --name-only <commit_hash>
Replace `<commit_hash>` with the actual hash of the commit you want to inspect.
Understanding Commits in Git
What Are Commits?
Commits in Git are snapshots of your code at specific points in time. Every time you make a change and commit it, you are essentially documenting that version of your files. This is crucial for tracking the evolution of your project, enabling you to revert to earlier stages if necessary or understand how your code has changed over time. Each commit contains not only the changes made to the files but also metadata, such as the commit message, author, and timestamp.
The Commit History
The commit history in Git is a chronological sequence of commits, forming a timeline for your project. Each commit points to its parent(s), creating a history that can branch and merge. Understanding the commit structure is essential when you wish to list commit files, as it allows you to navigate through past changes easily.
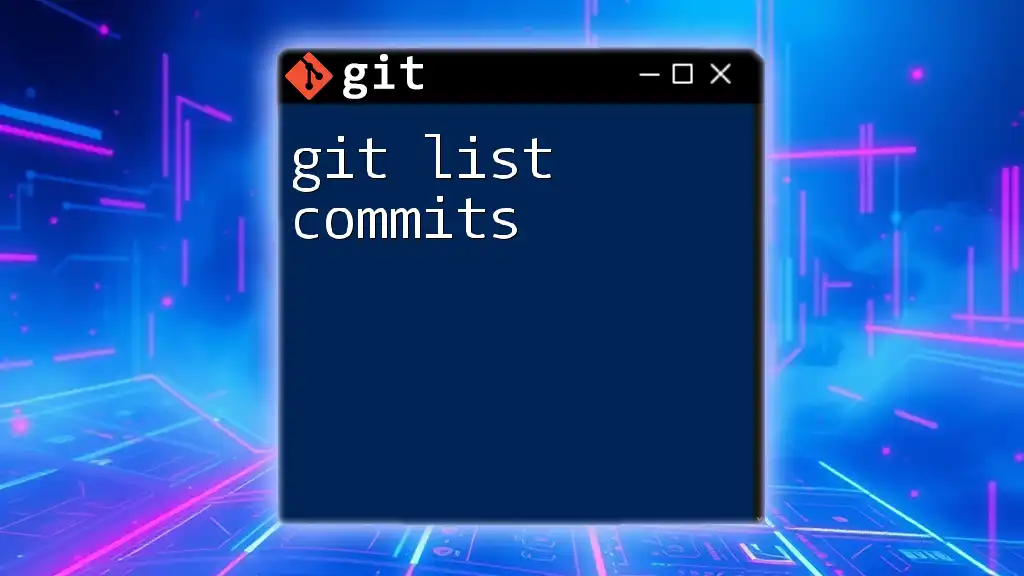
Why List Commit Files?
Listing commit files is instrumental for developers. By identifying which files changed in a commit, you can:
- Identify Changes: Quickly see what has been modified without scanning through entire files.
- Track Progress: Understand how features or fixes have evolved.
- Facilitate Collaboration: Aid teammates in reviewing changes that might affect their work.
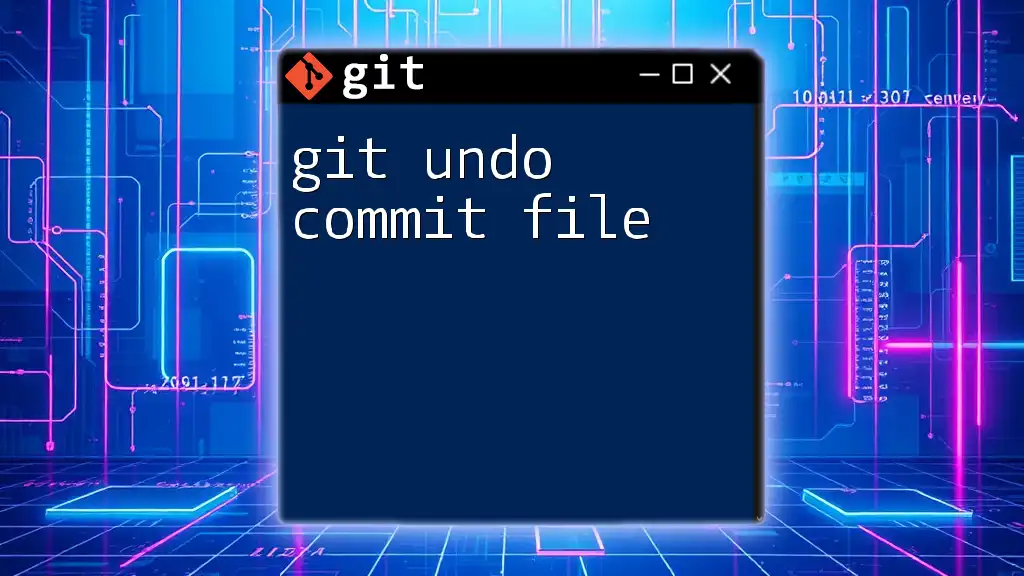
Basic Commands to List Commit Files
git log
The `git log` command is the starting point when it comes to exploring your commit history. When you run this command, it presents a chronological list of commits, showing commit hashes, authors, dates, and messages.
Command Syntax:
git log
You can enhance this command with options such as `--oneline` for a concise summary or `--stat` to include a summary of file changes.
For example, using `--oneline` will output each commit as a single line, which helps in quickly scanning through the logs:
git log --oneline
Viewing Specific Commits
To explore a specific commit's changes and view which files were modified, you will need to use the commit hash.
Using a Commit Hash: Command Syntax:
git show <commit-hash>
For example, to see the details of a specific commit:
git show 4a72c53
The output will display the differences introduced by that commit, including details about the modified files, which can be instrumental in reviewing changes.
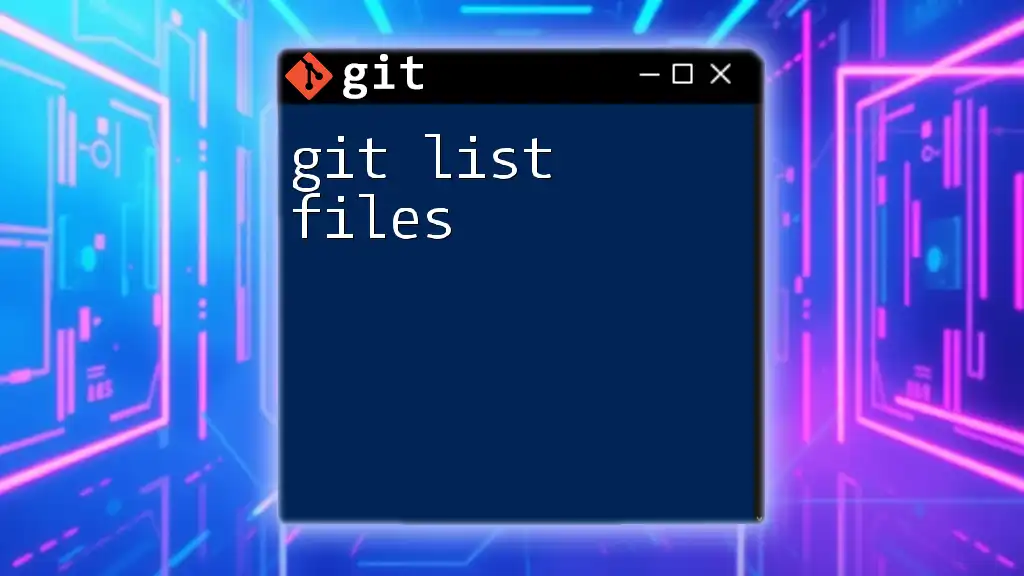
Advanced Commands for Listing Files
Using git diff
Listing Changed Files
If you want to see the files changed between two commits, `git diff` is your go-to command. It shows the differences between commits, branches, trees, and more.
Command Syntax:
git diff --name-only <commit-hash> <commit-hash>
Example: To compare the last commit with the one before it:
git diff --name-only HEAD~1 HEAD
This will list just the names of files that changed, which is often all you need to quickly understand the impact of a commit.
Using git diff-tree
For a more detailed analysis, `git diff-tree` can be used. It is specifically designed to list the changes made in a specific commit.
Overview: This command allows you to display information about the changes in a particular commit, showing only the file names.
Command Syntax:
git diff-tree --no-commit-id --name-only -r <commit-hash>
Practical Example: To check the files changed in a specific commit:
git diff-tree --no-commit-id --name-only -r abc123
The output will consist of the names of files changed in that particular commit, enhancing your visibility of project modifications.
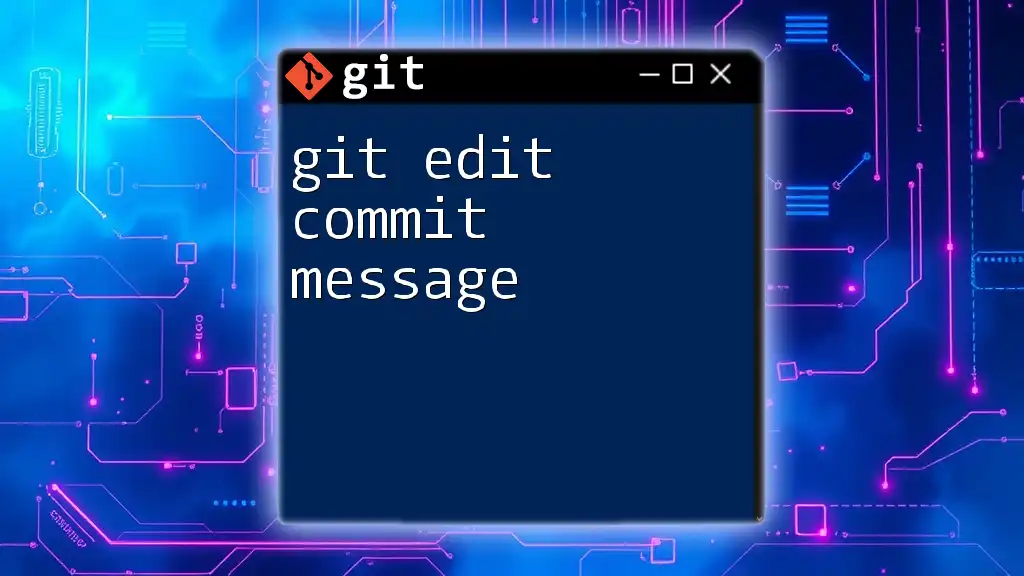
Customizing Output
Filtering by File Types
Sometimes, you may want to filter the changes by specific file types. This can help you focus on the changes relevant to your area of interest.
Command Syntax:
git diff --name-only <commit-hash> -- 'file-pattern'
Example: To filter for Python files:
git diff --name-only <commit-hash> -- '*.py'
This command isolates changes to only the specified file type, streamlining your review process.
Limiting Commits to Show Changes
When you are only interested in a specific number of recent commits, you can use the `--oneline` flag with the `-n` parameter, which limits the output.
Command Syntax:
git log --name-only --oneline -n <number>
Example: Limiting the log to the last five commits:
git log --name-only --oneline -n 5
This provides a clean view of the most recent changes and the files involved.
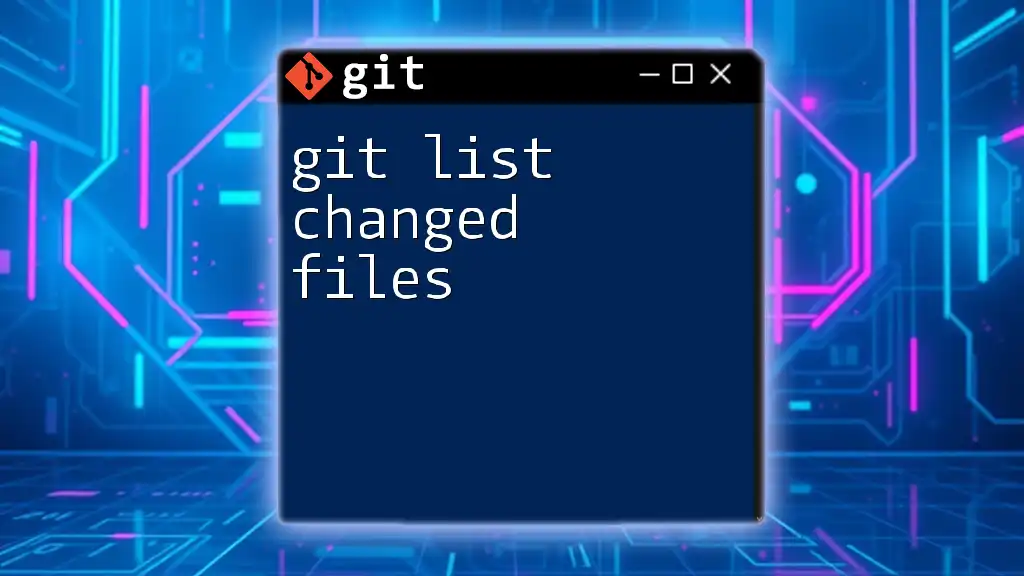
Combining with Other Git Commands
Using git log with grep
If you need to search through commit messages for specific keywords while also viewing the associated files, the combination of `git log` and `grep` is very powerful.
Command Syntax:
git log --grep="keyword" --name-only
This allows you to quickly find commits that match your search criteria along with the changing files, making it easier to track down information relevant to your work or project.
Getting Statistics with git diff
Tracking overall changes can also be advantageous, and `git diff` supports a statistics view that summarizes the changes between commits.
Command Example:
git diff --stat <commit1> <commit2>
This will provide a summary of files changed, lines added, and lines removed, giving you insight into the scope of changes without diving deep into each file.
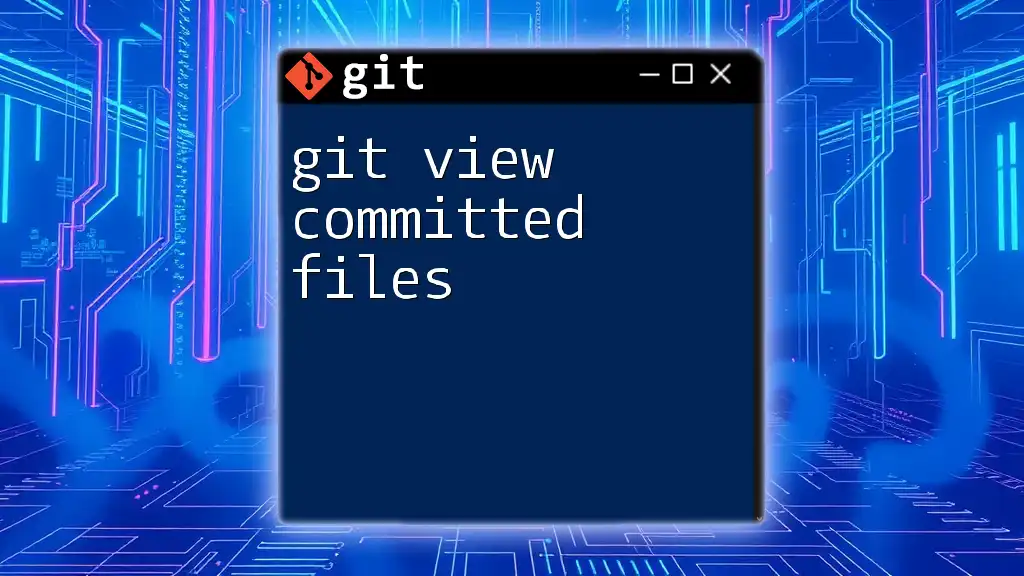
Conclusion
Listing commit files in Git is an essential skill for any developer. Not only does it inform you about what has changed, but it also aids in collaboration and project tracking. By mastering commands like `git log`, `git diff`, and `git diff-tree`, you can navigate your project's history with ease and confidence. Practice these commands regularly to become more efficient in your version control workflow.
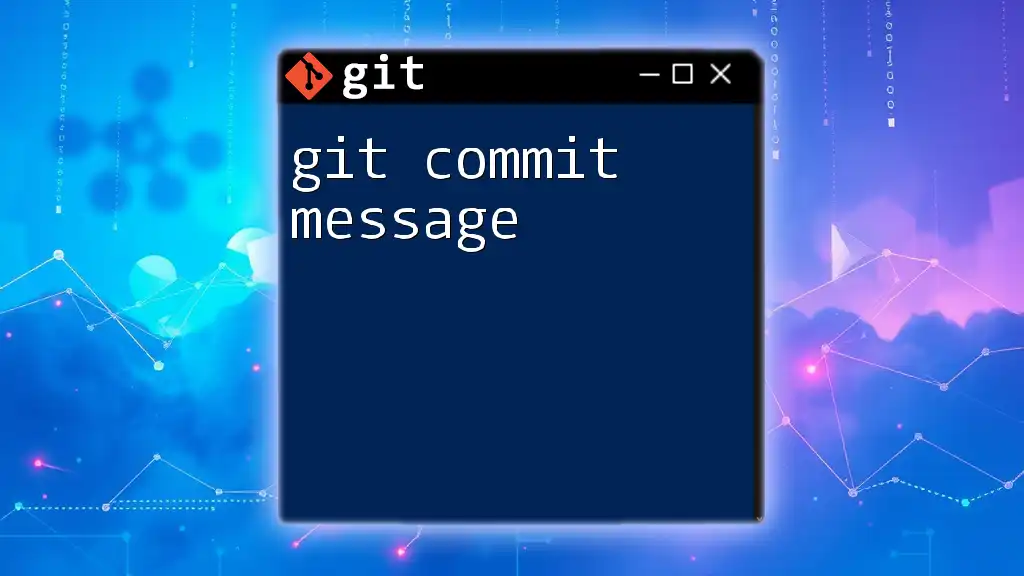
Additional Resources
For deeper insights and further exploration, refer to the official Git documentation, online courses, or version control best practices to enhance your Git proficiency and project management skills.