To view the files that have been committed in your Git repository, you can use the following command, which displays the list of files changed in the latest commit.
git show --name-only
Understanding Git Commits
What is a Git Commit?
A Git commit is a snapshot of your project at a specific point in time. It captures the state of your files and directories, along with a message detailing what changes were made. Each commit has a unique identifier known as a hash, which you can use to reference it later. This makes it easy to track your project's history and control changes over time.
Why View Committed Files?
Viewing committed files is crucial for several reasons:
- Tracking Changes: It allows you to see the evolution of your project and understand the reasoning behind changes made by you and your collaborators.
- Debugging: When issues arise, you can quickly identify when a feature was added or modified, making it easier to locate the root cause.
- Code Reviews: Understanding what changes have been made helps in effectively reviewing code before merging into the main branch.
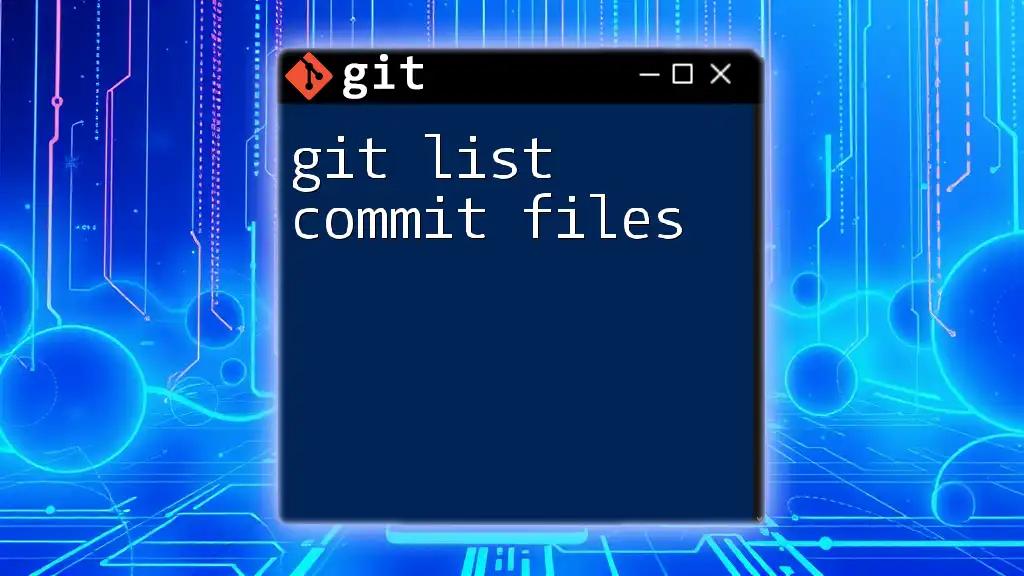
Viewing Committed Files in Git
Using Basic Git Commands
The `git log` Command
The `git log` command is one of the most fundamental tools in Git for viewing your commit history. It displays a log of commits in reverse chronological order.
Syntax:
git log
Output: When you run this command, you’ll see a list of commits displayed with the commit hash, author, date, and commit message. This information provides context about changes made in each commit, but it does not show the details of the file changes.
The `git show` Command
For a more detailed view of a specific commit, you can use the `git show` command. This command shows the changes made in that commit, including the differences in files.
Syntax:
git show <commit-hash>
Example:
git show 1a2b3c4
In this output, you will see the commit information followed by the unified diff of changes, which clearly indicates what was added or removed in each file. This is beneficial for reviewing the specifics of your or others' code.
The `git diff` Command
To compare two different commits and see what changes were made between them, the `git diff` command serves this purpose well.
Syntax:
git diff <commit-hash> <commit-hash>
Example:
git diff 1a2b3c4 5d6e7f8
When executed, this command will highlight the changes between two commits, allowing you to easily identify what has changed in your project over time.
Exploring Alternatives to View Committed Files
Viewing Files with `git diff --name-only`
If you only want a list of files that changed between two commits without seeing the actual changes, you can use the `--name-only` option.
Syntax:
git diff --name-only <commit-hash1> <commit-hash2>
This command provides a concise list of filenames, helping you quickly see which files were affected.
Using GUI Tools for Visualizing Commits
While command line tools are powerful, sometimes a graphical user interface (GUI) can make it easier to visualize your commit history. Tools like GitKraken, SourceTree, or GitHub Desktop offer user-friendly interfaces that allow you to explore your commits visually. These tools can display commit graphs, file changes, and more, providing a holistic view of your project’s history without the need to memorize commands.
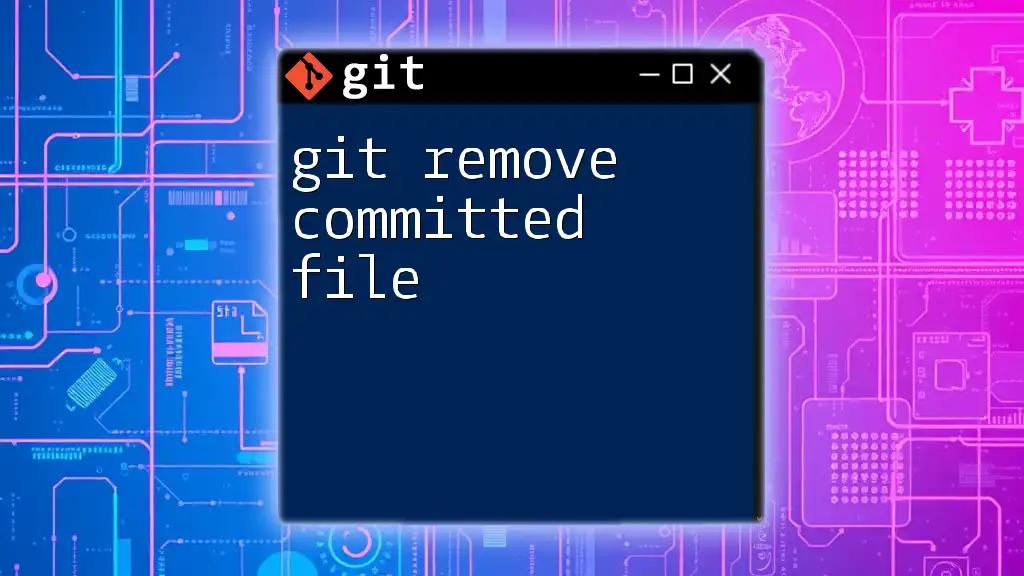
Advanced Techniques for Viewing Commits
Accessing File History with `git log` Options
Showing a Specific File's History
To focus on the changes made to a specific file, the `git log` command can be tailored to show just that.
Syntax:
git log -- <file-path>
Example:
git log -- src/example.js
This will show a history of commits that affected `example.js`, allowing you to track changes specific to that file.
Filtering Commits by Author or Date
You can also filter commits by specific criteria such as the author or date to narrow down your search for relevant changes.
Syntax:
git log --author="Author Name"
git log --since="2 weeks ago"
These filters help you hone in on commits pertinent to your current focus, simplifying the review process.
Using `git blame` to Track Changes Line-by-Line
The `git blame` command is a unique tool for seeing who last modified each line in a file. This is particularly useful when you want to understand the origin of a particular change or feature.
Syntax:
git blame <file-name>
Example:
git blame src/example.js
By using this command, you will receive line-by-line annotations of the specified file, showing which commit introduced each line. This insight is valuable for auditing code and understanding the source of various functionalities or potential issues.
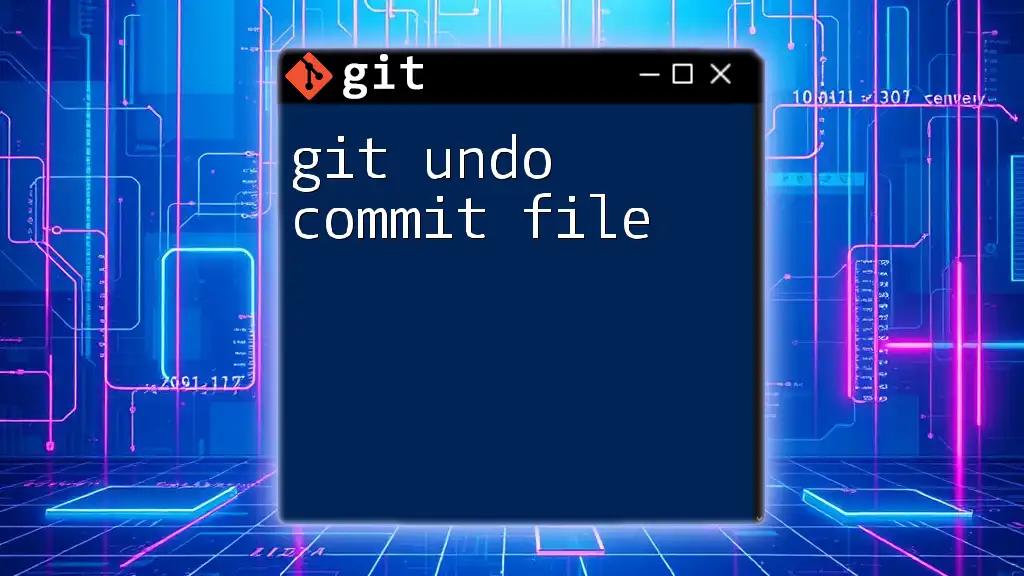
Conclusion
Viewing committed files is an essential skill in Git that can vastly improve your workflow. Whether tracking changes, debugging, or reviewing code, mastering these commands allows you to make the most of version control. Practice using the commands and techniques discussed here, and explore the vast capabilities of Git for maintaining your projects effectively.
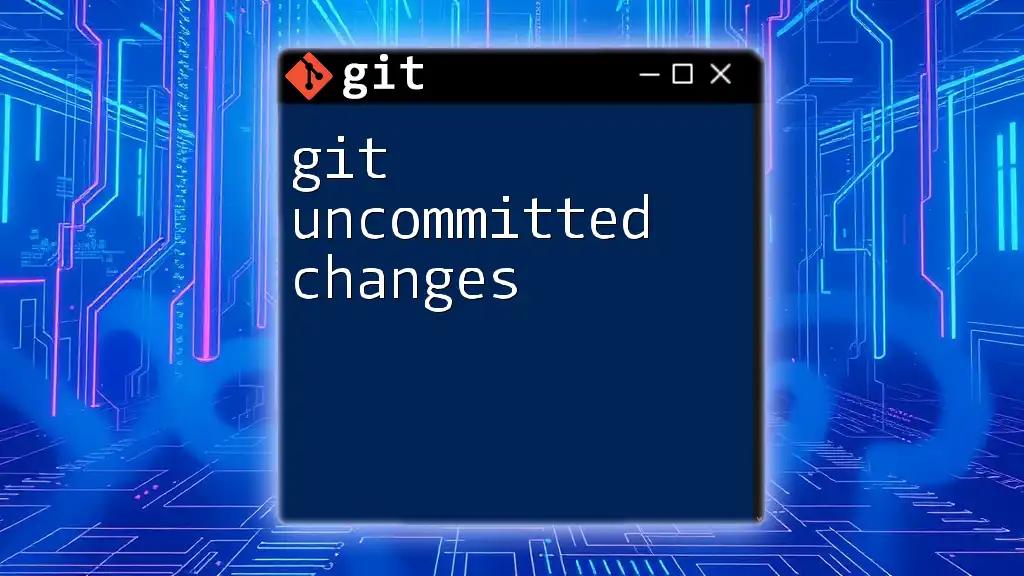
Additional Resources
- Visit the official Git documentation for comprehensive command references.
- Check out tutorials on platforms like GitHub or GitLab for visual guides and hands-on examples.
- Explore readings on advanced Git features to enhance your workflow further.