To list the changed files in a Git repository, you can use the following command:
git diff --name-only
Understanding Changed Files in Git
What Are Changed Files?
In Git, changed files refer to any file that has been modified in any way since the last commit. These files can be in various states:
- Modified: The file has been changed but not yet staged or committed.
- Staged: The file is ready to be committed, meaning it has been added to the staging area.
- Untracked: The file exists in your working directory but has not been added to Git's tracking.
Importance of Tracking Changes
Keeping track of modified files is vital for effective project management and collaboration. Not only does it allow developers to review their changes, but it also helps in understanding the impact of those changes on the overall project. By being aware of which files have changed, you can prevent conflicts and ensure smoother integration during team collaboration.
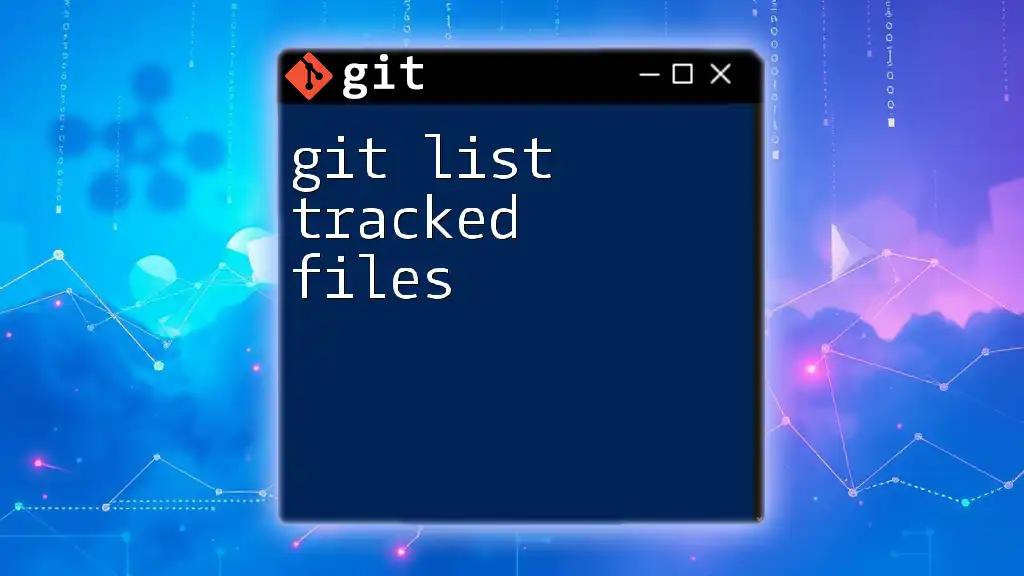
Overview of Git Commands for Listing Changed Files
Git provides several commands to efficiently list changed files. The most relevant commands include:
- `git status`: Gives an overview of the working directory and staging area.
- `git diff`: Shows differences between commits, branches, and more.
- `git ls-files`: Lists files and their states.
- `git show`: Displays information about commits and changes.
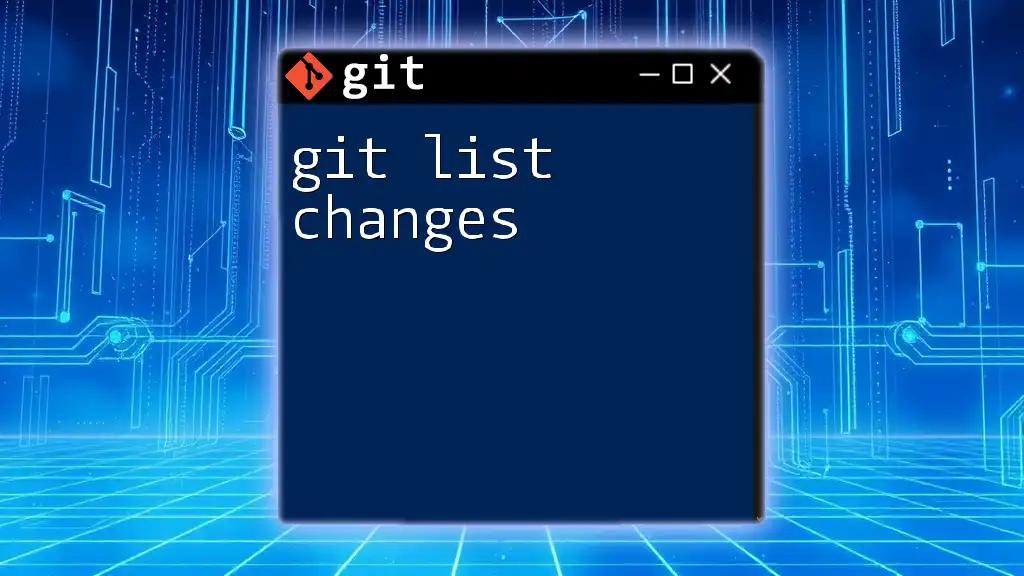
Using `git status` to List Changed Files
What Does `git status` Do?
The `git status` command is one of the most commonly used commands in Git. It provides a detailed overview of the current state of your repository, indicating:
- Changes that are staged for the next commit.
- Changes that are not staged yet.
- Untracked files that are not being tracked by Git.
Example Usage
To check the status of your Git repository, you can run:
git status
This command might return output similar to:
On branch main
Your branch is up to date with 'origin/main'.
Changes to be committed:
(use "git restore --staged <file>..." to unstage)
modified: file1.txt
new file: file2.txt
Changes not staged for commit:
(use "git add <file>..." to update what will be committed)
modified: file3.txt
Untracked files:
(use "git add <file>..." to exclude from showing up in status)
file4.txt
In this output, you can easily interpret the listed files and their states: `file1.txt` is staged, `file3.txt` is modified but not staged, and `file4.txt` is untracked.
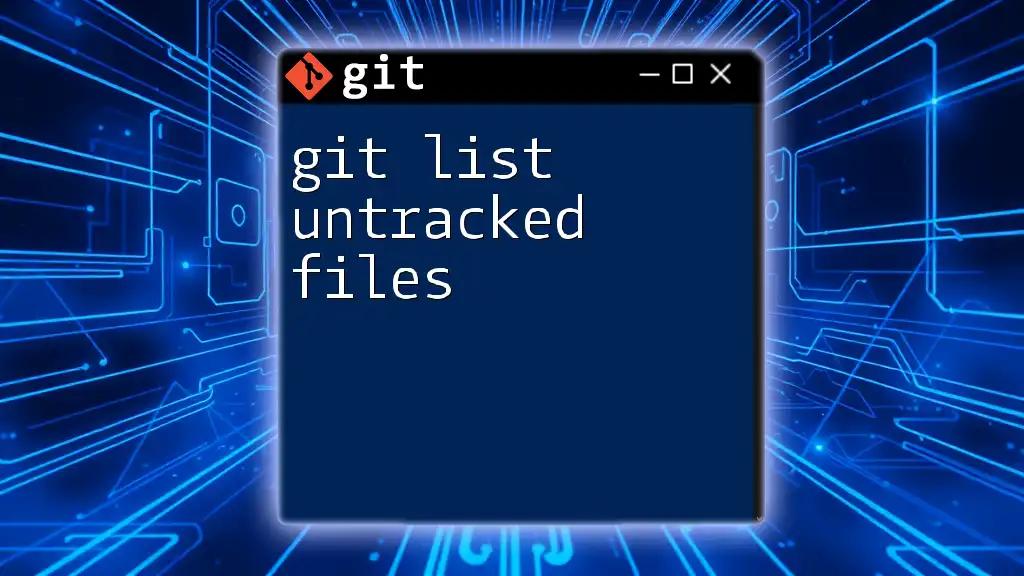
Using `git diff` to View Changes
Understanding `git diff` Command
The `git diff` command allows you to view the differences between various states of your files. This can help you see exactly what you've modified.
Examples of Different Use Cases
To see changes in tracked files since the last commit, you can execute:
git diff
If you want to see the changes in staged files (files ready to be committed), use:
git diff --cached
For a more specific comparison, such as between two commits, you can specify the commits directly:
git diff commit1 commit2
The output of `git diff` will highlight additions and deletions, making it easier for you to review your changes.
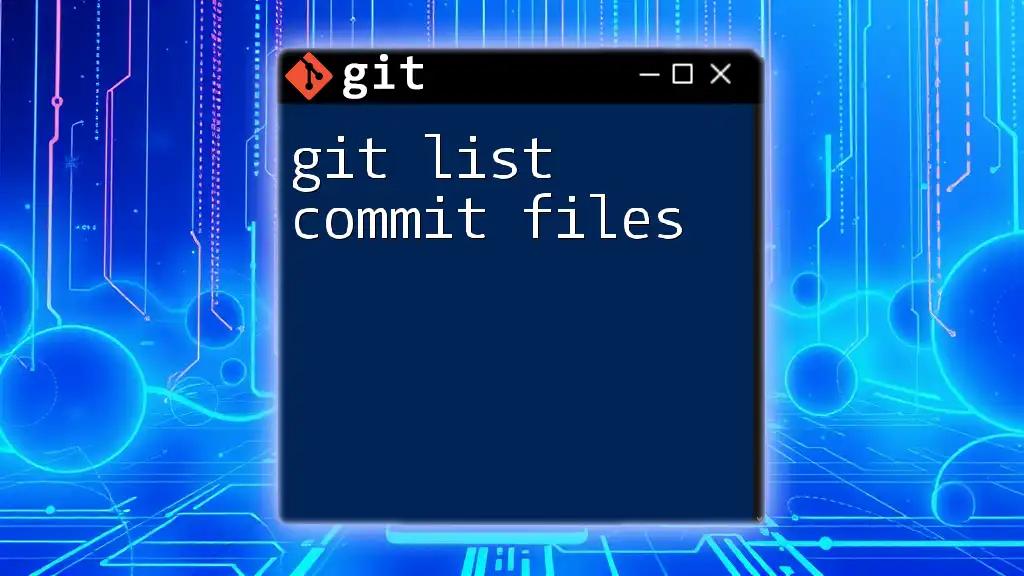
Using `git ls-files` to List All Files
How `git ls-files` Helps
The `git ls-files` command is instrumental in listing files that are part of the version control. It can help you understand the status of your files and manage them more effectively.
Example Usages
To list all tracked files in your repository, you can run:
git ls-files
To filter down to modified files only, use:
git ls-files --modified
You can also utilize other flags like `--others` to show untracked files or `--deleted` to list files that have been deleted.
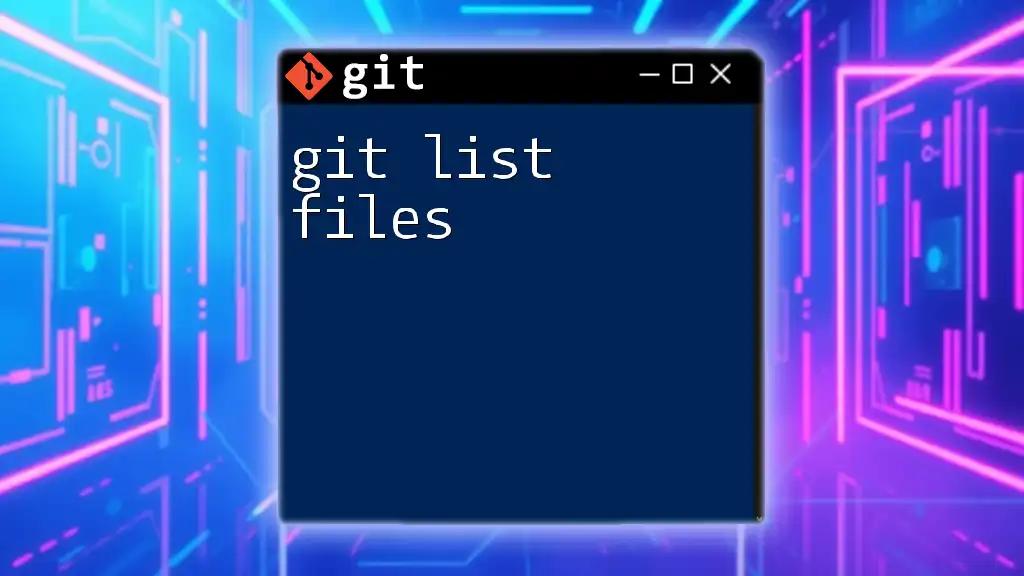
Using `git show` for Specific Changes
Introducing `git show` Command
The `git show` command is incredibly useful for viewing the details of commits, including the files that were changed in a given commit.
Example Usage
To view all the files that have changed in the latest commit, execute:
git show --name-only
This command will display the commit information along with a list of changed files, allowing you to quickly see what has been modified without needing to look through the entire commit details.
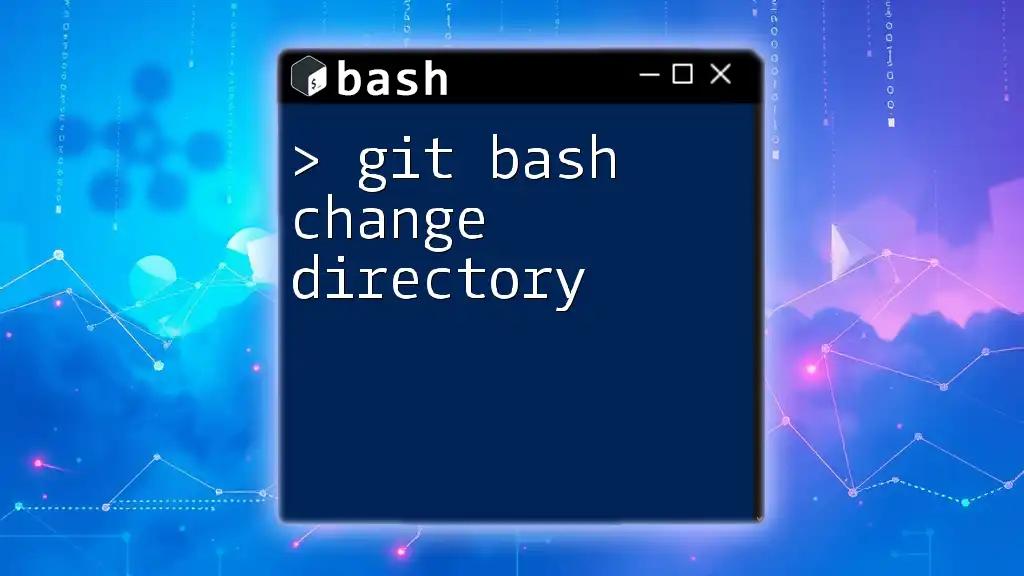
Practical Examples and Scenarios
Common Use Cases for Listing Changed Files
-
Working in a Team
When collaborating with others, regular usage of `git status` and `git diff` will help you see changes made by teammates. This is especially useful before merging branches, as it allows you to assess potential conflicts. -
Debugging a Bug
If you suspect a recent change has introduced a bug, using `git diff` can help you quickly identify the changes. By comparing previous commits with the latest, you can pinpoint the issue and strategize your debugging effort more effectively.
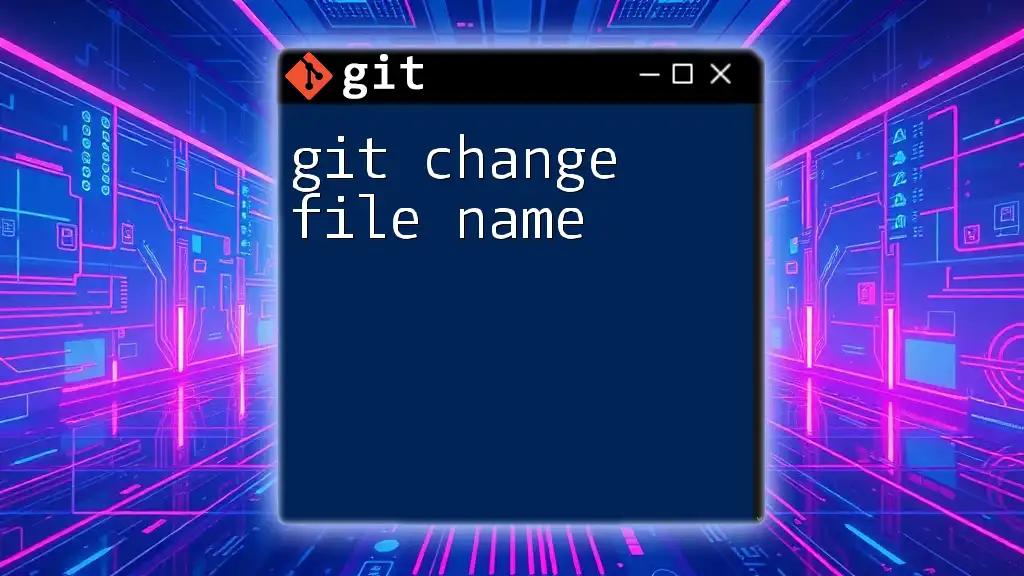
Best Practices for Tracking Changes in Git
Keeping Your Git Clean
To maintain an effective workflow, regularly check your status and commit changes often. This helps prevent a cluttered working directory and avoids overwhelming information during larger development tasks.
Utilizing Git Hooks for Automation
Consider employing Git hooks to automate the process of notifications for changes. For example, you can set up pre-commit hooks to remind you to check the status before finalizing a commit, streamlining your workflow.
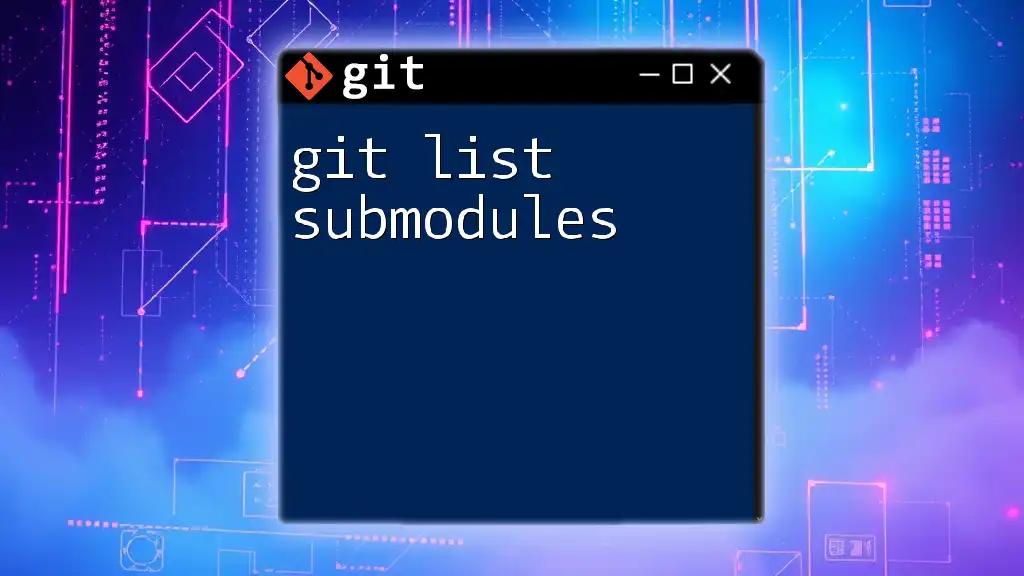
Conclusion
Tracking changed files in Git is crucial for maintaining an efficient and organized workflow. By mastering these commands, you enhance your ability to collaborate effectively, debug issues, and manage your projects more effectively. Be sure to practice using these commands and explore their variations for a deeper understanding.
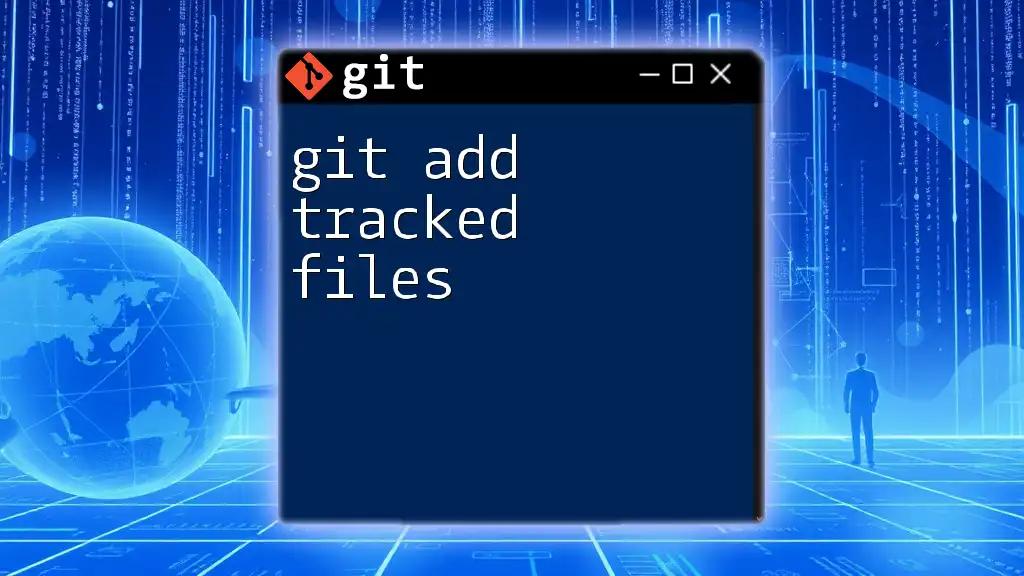
Additional Resources
To further your Git knowledge, refer to:
- The official Git documentation for comprehensive explanations and examples.
- Recommended tools and GUI applications that can simplify working with Git.
- Related articles that delve deeper into version control best practices and strategies.