To list changes in a Git repository, you can use the `git status` command to see the current state of the working directory and staging area, including modified, staged, and untracked files.
git status
Understanding Git Changes
What Are Git Changes?
In the context of Git, changes refer to any modifications made to your files within a repository. This includes added files, modified files, and deleted files. Understanding how to track and list these changes is crucial for effective collaboration, as it allows developers to see who made what changes and when.
Types of Changes in Git
-
Unstaged Changes: These are the modifications you've made but haven't yet marked to be included in your next commit. They exist in your working directory and are visible when you use specific commands to list changes.
-
Staged Changes: Successfully added to the staging area, these changes are ready for committing. The staging area serves as a buffer between the working directory and the repository, allowing you to fine-tune what will be included in your next commit.
-
Commits: A commit represents a snapshot of your files at a certain point in time. Each commit contains a unique ID (hash), author information, and a commit message that explains what changes were made.
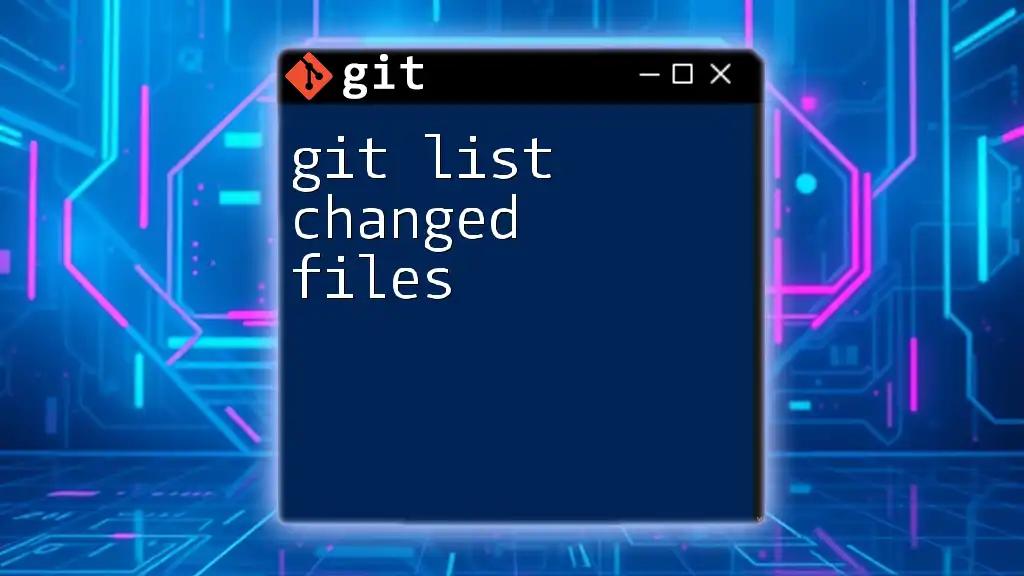
Fundamental Git Commands for Listing Changes
git status
The `git status` command is essential for understanding the current state of your working directory and staging area. It provides a comprehensive summary of modified files, untracked files, and files that are ready to be committed.
Usage:
git status
Example Output:
When you run this command, you may see output similar to the following:
On branch master
Your branch is up to date with 'origin/master'.
Changes not staged for commit:
(use "git add <file>..." to update what will be committed)
modified: file1.txt
deleted: file2.txt
Untracked files:
(use "git add <file>..." to include in what will be committed)
file3.txt
This output clearly indicates which files have been modified, deleted, or are untracked.
git diff
The `git diff` command is used to see the differences between various states of the repository—specifically, changes that are not yet staged for commitment and those that are staged.
Usage: To see unstaged changes:
git diff
To see staged changes:
git diff --cached
Example Output:
The output of a `git diff` command highlights differences, indicating added lines with a `+` and removed lines with a `-`. The context around the changes is also provided to give you insights into what modifications occurred.
git log
The `git log` command presents the commit history of the repository. This command is crucial for tracking changes across the development timeline.
Usage:
git log
Options: To limit the number of displayed commits:
git log -n 5
To show a simplified commit format:
git log --oneline
Example Output:
Running `git log` may yield output like this:
f9c0bd0 (HEAD -> master, origin/master) Fix typo in file1.txt
3e4c56a Add file2.txt
9c23f4b Initial commit
This output provides the commit hash, branch information, commit message, and other relevant details for each commit.

Advanced Commands for Detailed Change Listing
git reflog
`git reflog` is especially useful for tracking where your branches and HEAD have been over time. This command maintains a local history of the actions performed.
Usage:
git reflog
Example Output:
The output of `git reflog` will show entries of HEAD and branch movements, providing timestamps and actions like commits, checkouts, or merges.
git show
The `git show` command displays detailed information about a specific commit, including the changes it introduced.
Usage:
git show <commit-hash>
Example Output:
The output of this command reveals the commit message, author, date, and the specific changes made in that commit. This is handy for getting context on changes without scrolling through the entire file history.
git blame
`git blame` is a powerful command that displays what revision and author last modified each line of a file. This is especially useful for tracking down when a specific change was made and by whom.
Usage:
git blame filename
Example Output:
Running `git blame file1.txt` provides a list of annotations next to each line of the file, showcasing the commit hash, author, and timestamp for each line.
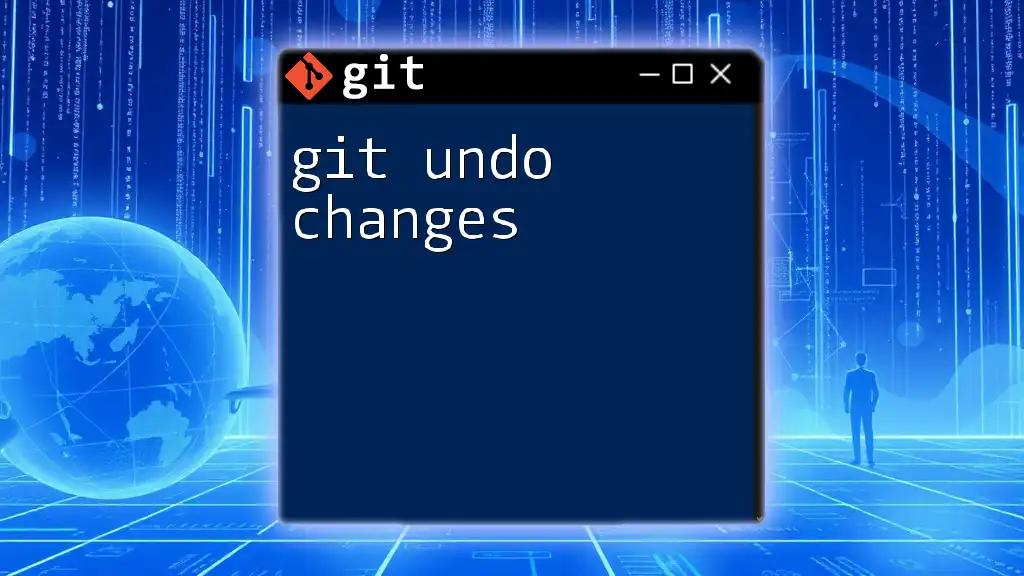
Practical Examples
Listing Changes in a Real-World Scenario
Let’s look at a brief demonstration of listing changes throughout a collaborative project.
1. Initialize a new repository:
git init my-project
cd my-project
2. Create and modify files: Create a new file and make changes:
echo "Hello World" > file1.txt
git add file1.txt
3. Use `git status`, `git diff`, and `git log`:
- Before committing, check the status:
git status
- View the differences:
git diff
- Commit the changes:
git commit -m "Initial commit with file1.txt"
- Review the commit log:
git log
Using these commands, you can effectively track changes as your project evolves.
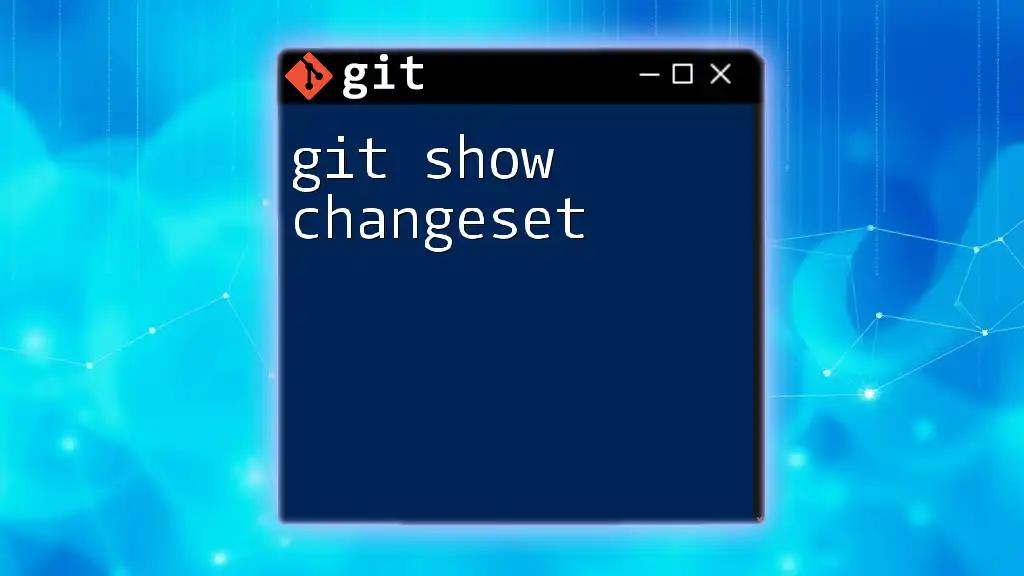
Best Practices for Listing Changes
Regular Monitoring
Regularly checking the status and diffs of your files as you work can significantly enhance your productivity and prevent surprises during the commit stage. Make it a habit to run `git status` before making commits.
Documenting Changes
Maintaining clear and informative commit messages is vital. This not only enhances your change history but also aids your collaborators in understanding the purpose behind your changes.
Collaboration Tips
When working in teams, establish a routine for reviewing changes and communicating about them. Use commands like `git log` and `git blame` to maintain clarity on project development and responsibilities.
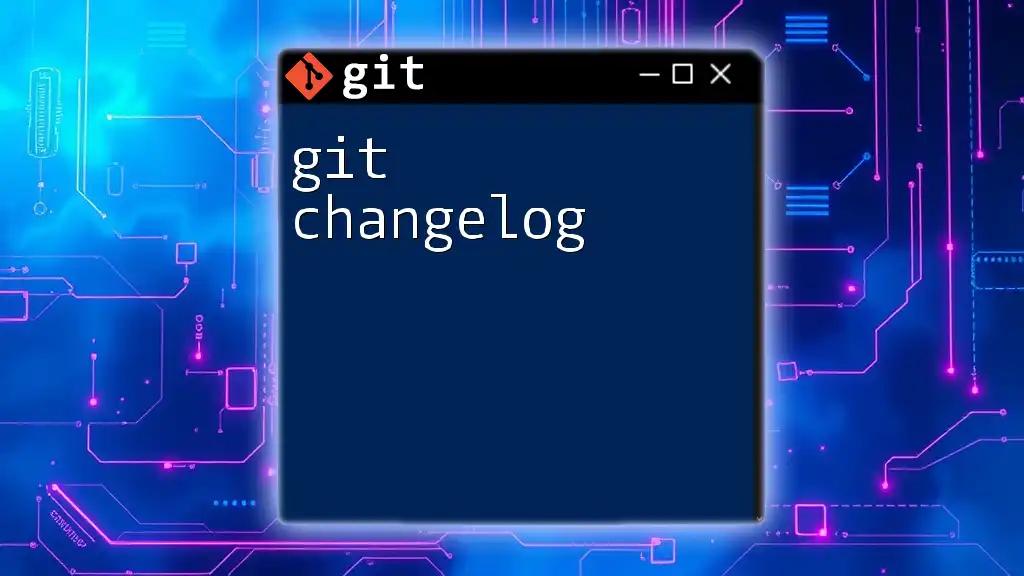
Conclusion
By understanding how to effectively list changes in Git, you empower yourself to manage your projects and collaborate with others more efficiently. Remember to practice these commands regularly, as they will become invaluable tools in your development toolkit. Explore more advanced Git features to further enhance your skills and streamline your workflow.