To check the changes made in your Git repository, you can use the `git status` command to see modified files and the `git diff` command to view the actual changes made to those files.
git status
git diff
What Does “Check Changes” Mean in Git?
In the context of Git, checking changes refers to the process of reviewing the modifications made to your code before committing, pushing, or merging. This crucial aspect of version control allows developers to keep track of their progress, identify errors, and ensure that only the intended changes are recorded in the repository.
When checking changes, you can distinguish between different states of your files:
- Staged Changes: These are files ready to be committed.
- Unstaged Changes: These are modifications that have yet to be added to the staging area.
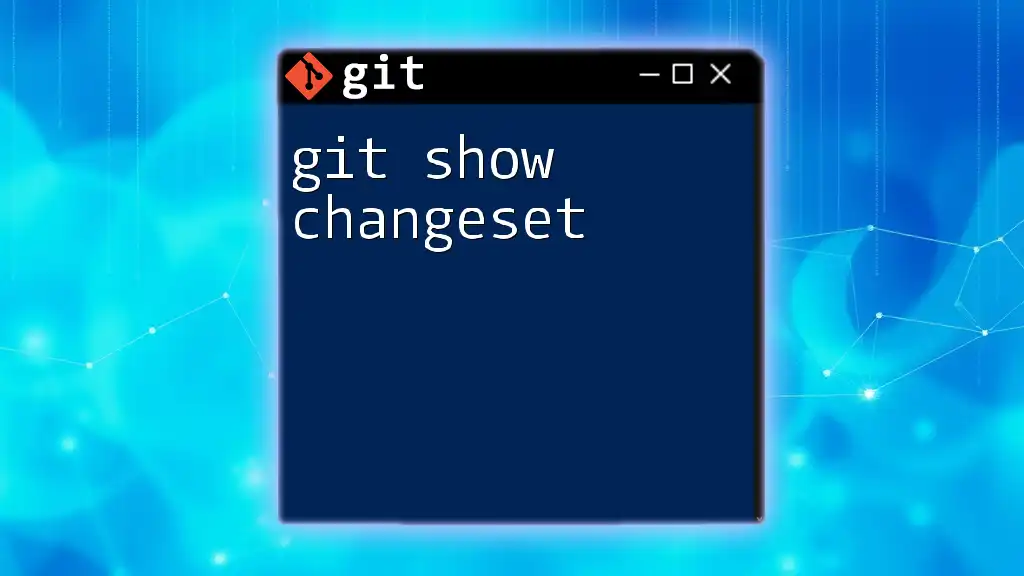
The Importance of Reviewing Changes
Regularly checking changes in Git comes with several key benefits:
- Preventing Errors: By reviewing your changes before committing, you can catch potential mistakes, such as syntax errors or unintended modifications.
- Enhancing Collaboration: When working in teams, checking changes ensures that your contributions align with those of other team members, reducing conflicts and improving coherence.
- Maintaining a Clean Repository: A well-maintained repository is essential for effective collaboration. Regularly checking and cleaning up changes helps keep the project organized and manageable.
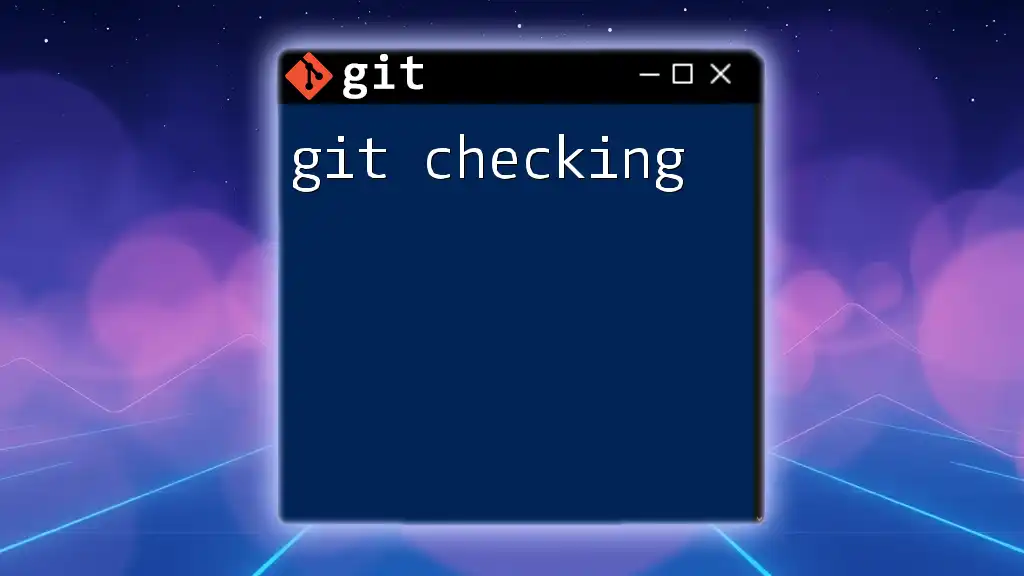
Key Git Commands for Checking Changes
git status
The `git status` command provides a summary of your current branch's state, indicating which files are staged, unstaged, or untracked.
Use Cases: Use this command when you want a quick overview of your project's state before you commit.
Example Command:
git status
Output Explanation: When you run `git status`, you'll receive feedback on the state of your working directory and staging area. Look for these key messages:
- Changes to be committed: Lists files that are staged and ready for the next commit.
- Changes not staged for commit: Shows files that have changes but haven’t yet been staged.
- Untracked files: Displays new files not yet added to version control.
git diff
The `git diff` command lets you see differences between changes in your files. It can display unstaged changes, staged changes, or differences between commits, depending on how you use it.
Unstaged Changes: To see changes you've made but not yet staged, run:
git diff
Staged Changes: To view changes that are in the staging area but not yet committed, use:
git diff --cached
Color-Coding in Output: The output uses color-coding to represent changes:
- Lines starting with `+` are additions (in green).
- Lines starting with `-` are deletions (in red).
git log
The `git log` command allows you to view a chronological history of commits made in your project.
Basic Usage: To see a list of commits, simply run:
git log
With One-Line Output: For a more concise version, you can use:
git log --oneline
Output Explanation: The output lists commits along with their unique hash, the author, date, and commit message. Understanding this information helps you track project progress and review historical changes efficiently.
git show
The `git show` command provides a convenient way to see detailed information about a specific commit.
Use Cases: This command is particularly useful when you want to understand what changes were introduced in a specific commit without browsing through logs.
Example Command:
git show <commit_hash>
Output Breakdown: The output includes the commit message, author information, date, and the actual changes applied in that commit. This clarity helps maintain context when reviewing past modifications.
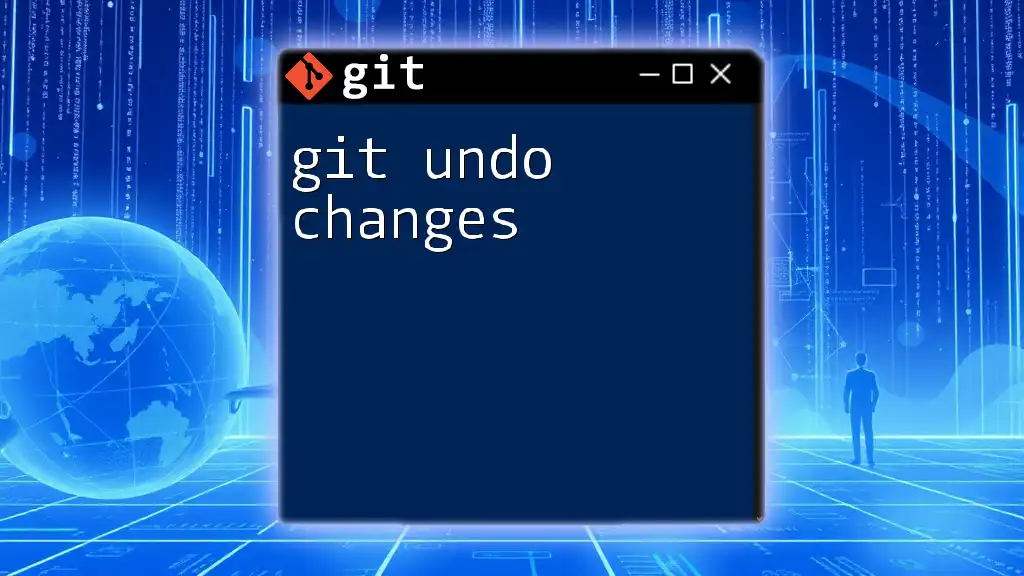
Visualizing Changes with GUI Tools
Introduction to Git GUI Tools
While the command line is powerful, many developers benefit from using graphical user interface (GUI) tools for visualizing changes. These tools simplify the process of checking changes by providing a user-friendly experience.
Example GUI Tools
-
SourceTree: A popular GUI tool that offers a clear view of your repository’s state. It visually indicates staged and unstaged changes, making it easier to manage code effectively.
-
GitKraken: Known for its visually appealing interface, GitKraken provides features such as drag-and-drop functionality for changes and a straightforward way to check changes in the repository.
-
GitHub Desktop: This tool is especially useful for those familiar with GitHub, allowing users to easily manage and visualize their changes without diving deep into the command line.
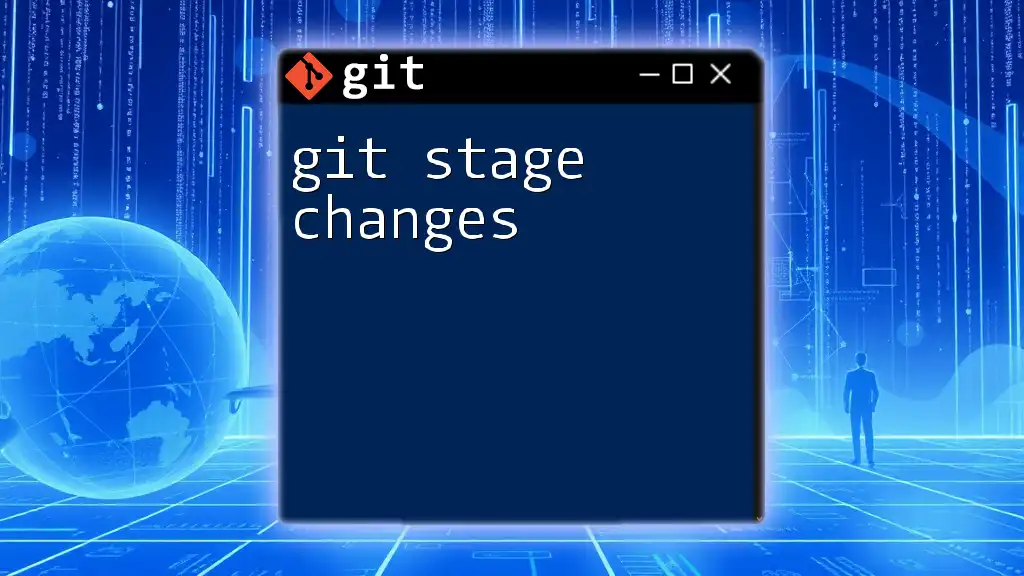
Best Practices for Checking Changes
To make the most of Git’s change-checking capabilities, consider the following best practices:
- Always check your changes before committing: This habit helps ensure you only add the modifications you intend to include.
- Use descriptive messages with `git commit`: Clear commit messages aid future team members (or yourself) in understanding the context of changes.
- Utilize branches to separate features or bugs: By working on separate branches, you can minimize the clutter in the main branch, making it easier to check changes related to a specific feature or fix.
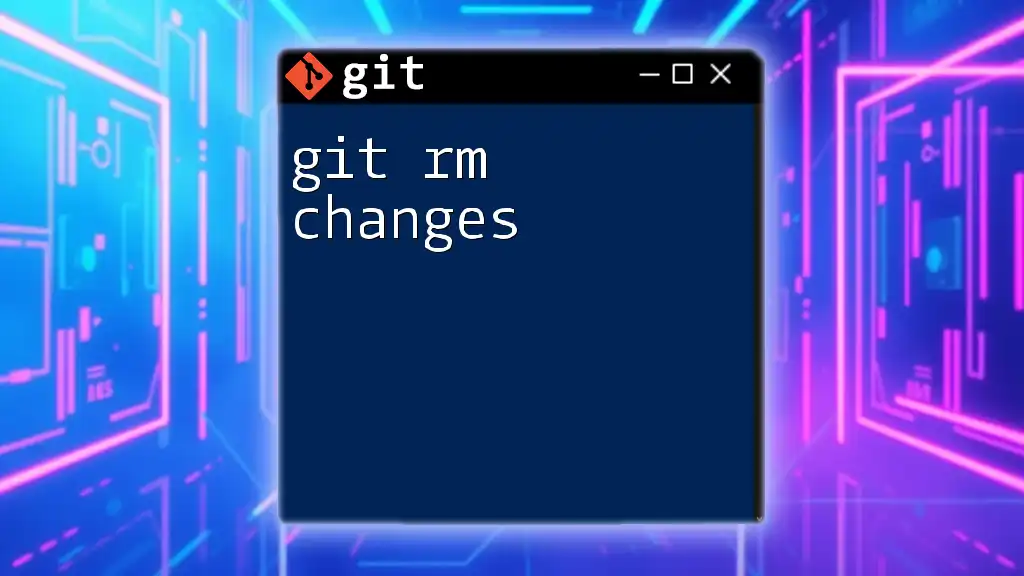
Common Pitfalls When Checking Changes
Understanding potential pitfalls can significantly enhance your workflow:
- Failing to review unstaged changes: Not checking unstaged changes can lead to unintentional commits with errors.
- Ignoring conflicts when merging: Always resolve merge conflicts before finalizing changes to ensure a clean project state.
- Committing too frequently without checking changes first: Frequent commits without review can clutter your project’s history and complicate later analysis.
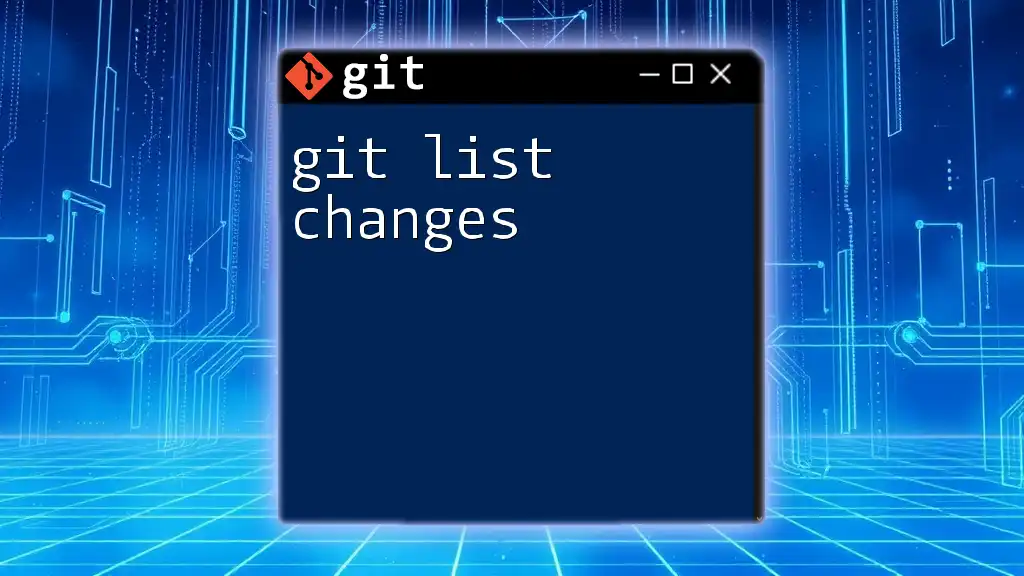
Conclusion
Mastering the process of checking changes in Git is essential for effective version control. By utilizing commands like `git status`, `git diff`, `git log`, and `git show`, you can maintain a clear understanding of your project's state while avoiding common pitfalls. Incorporating these practices into your workflow will not only enhance your coding efficiency but also facilitate smoother collaboration with your team.
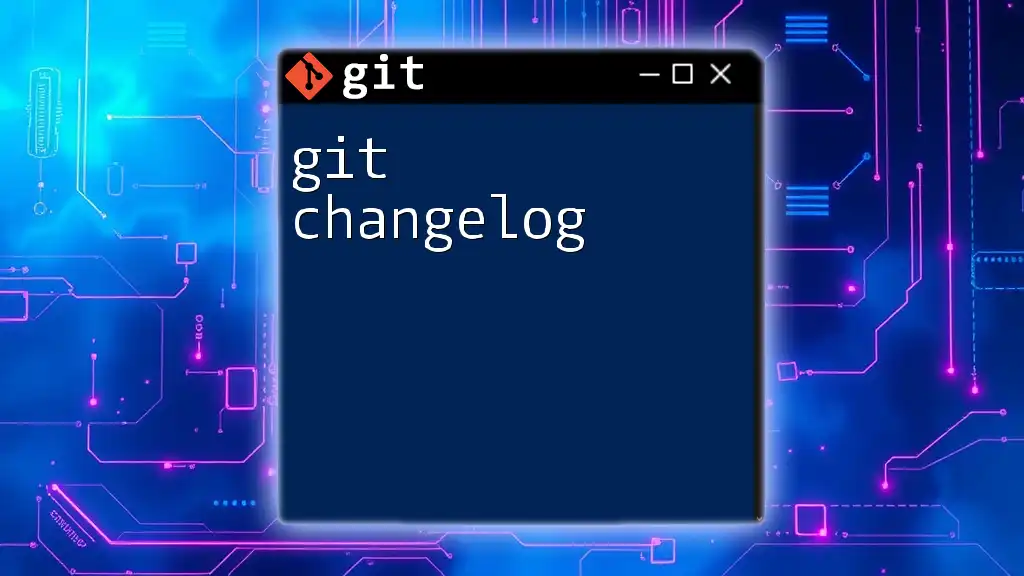
Additional Resources
For further learning, explore the following resources:
- [Official Git Documentation](https://git-scm.com/doc)
- [Recommended Tutorials and Videos on Git](https://git-scm.com/doc/tutorials)
- [Suggested Reading Materials for Advanced Git Usage](https://git-scm.com/book/en/v2)