To cancel a merge in Git and revert to the previous state before the merge began, you can use the command `git merge --abort`.
Here's the code snippet in markdown format:
git merge --abort
Understanding Git Merge
What is a Git Merge?
A git merge is the process of combining changes from two different branches into a single branch. It merges the changes made in a source branch into the target branch. This is crucial in collaborative projects where multiple developers are making changes in parallel.
When merges occur, Git creates a new commit that has two parent commits, representing the two branches that were merged together. This ensures that concerted efforts from different contributors can be integrated seamlessly, preserving the individual contributions.
When Do Merges Occur?
Merges typically occur in two scenarios:
- Automatic Merges: These happen when the branches being merged do not have conflicting changes. Git can integrate them without the need for user intervention.
- Conflict Merges: This situation arises when changes made in the two branches conflict with each other. Git will pause the merging process to allow developers to resolve these conflicts before proceeding.
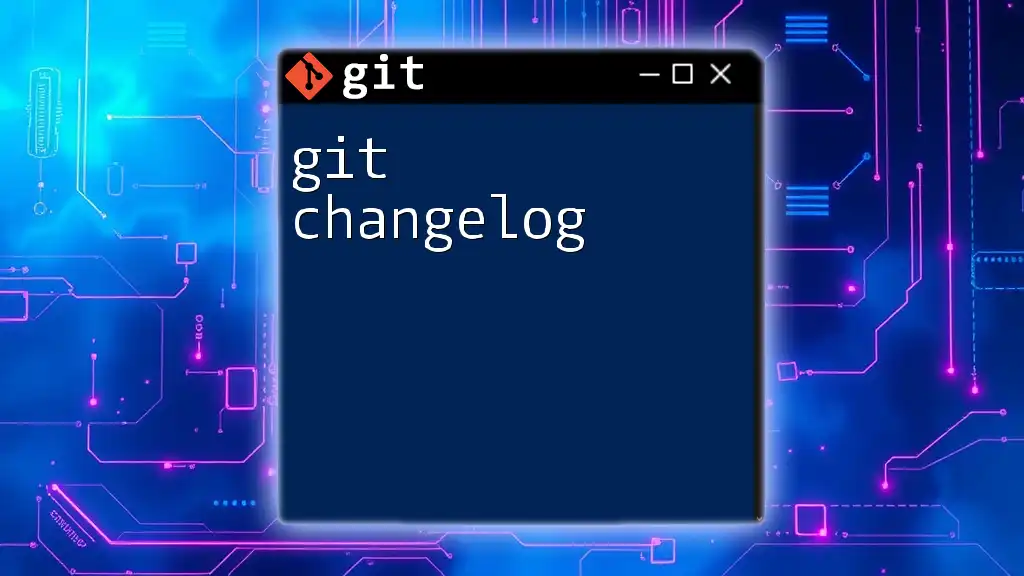
Reasons to Cancel a Git Merge
Common Situations for Cancelling a Merge
There are several reasons why you might want to cancel a git merge:
- Mistaken Merges: You may realize that you have merged the wrong branch into your working branch.
- Introduction of Bugs: If the merged branch contains bugs or untested features, it could be prudent to abort.
- Complex Conflicts: Sometimes, the conflicts to resolve may be too intricate or time-consuming, necessitating a cancellation to reassess your approach.
Consequences of Not Cancelling a Merge
Neglecting to cancel a merge in time can lead to a multitude of complications:
- Downtime in Collaborative Environments: It could affect the workflow of your teammates, especially in a project where timing is of the essence.
- Impact on Project Progress: A hurried merge can lead to delays as the team spends additional time troubleshooting unintended consequences.
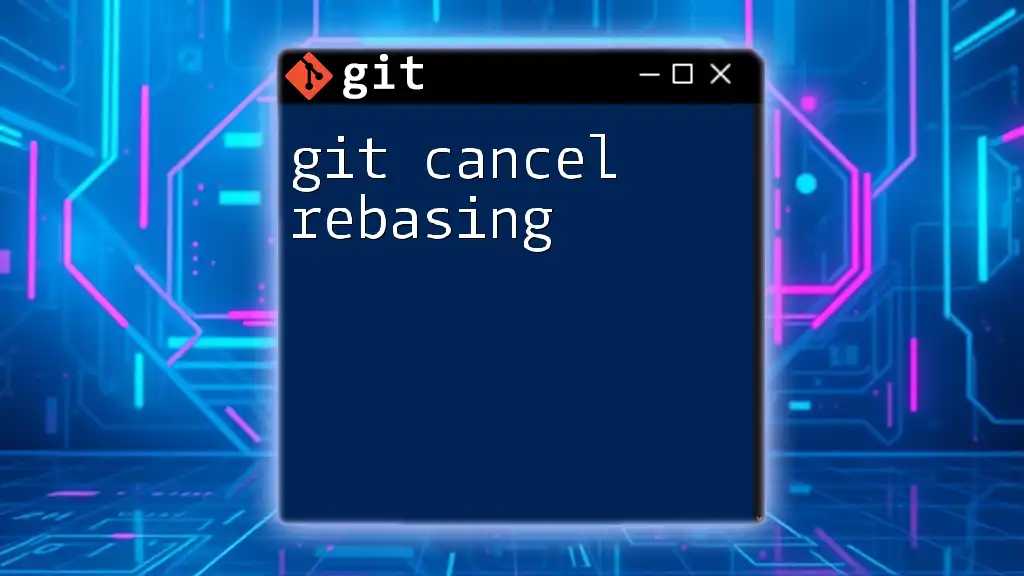
How to Cancel a Git Merge
Using `git merge --abort`
If you find yourself in a situation where a merge needs to be cancelled, one of the simplest solutions is to use the command:
git merge --abort
This command will revert your branch back to the state it was in before the merge attempt was initiated. It effectively undoes the merge process if conflicts were encountered, allowing you to reassess the situation.
Using `git reset`
When a merge has already been completed, you may need to take a more direct approach. This is where `git reset` comes in.
Soft vs Hard Reset
There are two primary types of resets: soft and hard. Understanding their differences is crucial:
- Soft Reset: This moves your HEAD pointer to a previous commit while keeping all changes staged. It's useful when you want to regroup changes after a merge.
- Hard Reset: This option discards changes completely, reverting your working directory back to a specific commit. It's powerful but carries risk as it permanently deletes changes after the specified commit.
Code Snippet for Soft Reset
To execute a soft reset, use the command:
git reset --soft HEAD~1
This command sets the HEAD pointer back by one commit but retains all changes in your staging area. You can now review these changes and decide what to keep or discard.
Code Snippet for Hard Reset
If you're sure about completely doing away with changes made during a merge, the hard reset is your go-to. Be cautious when using it:
git reset --hard HEAD~1
This command erases all uncommitted changes and returns the branch back to the state of the previous commit. Use this option only when you're confident that you no longer need the merged changes.
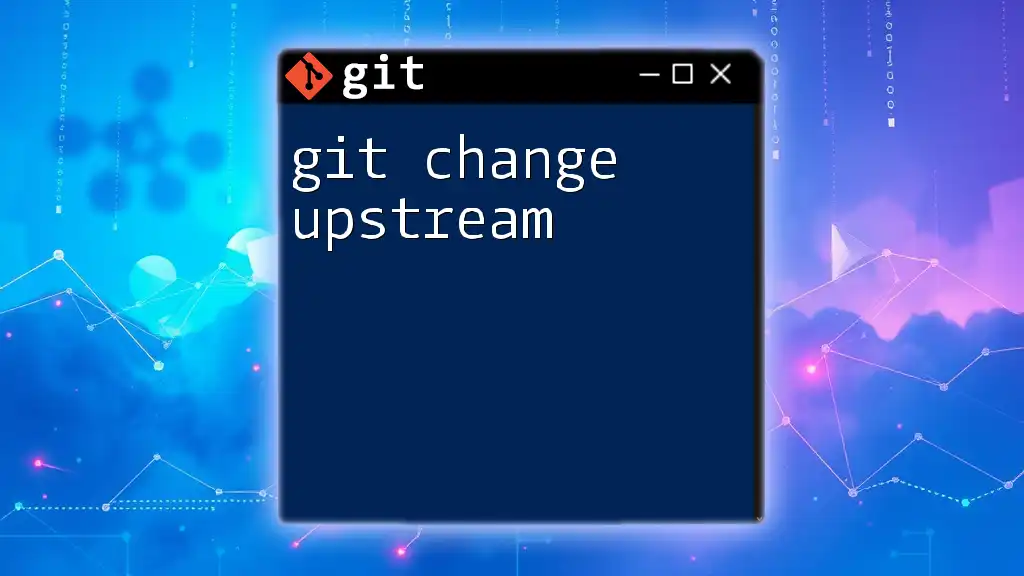
Additional Methods to Cancel Changes during a Merge
Checking Out the Original Branch
Sometimes, it might be simpler to just check out the original branch you were working on:
git checkout <branch_name>
This command allows you to discard any merges or changes made in the current working branch and take you back to the previously established state.
Using `git reflog`
Another handy tool for recovering from complex merge situations is `git reflog`. This command shows a log of where your HEAD pointer has moved over time:
git reflog
Using this command, you can find the commit before the merge and return to it, which is especially useful if you're unsure of how to safely cancel changes.
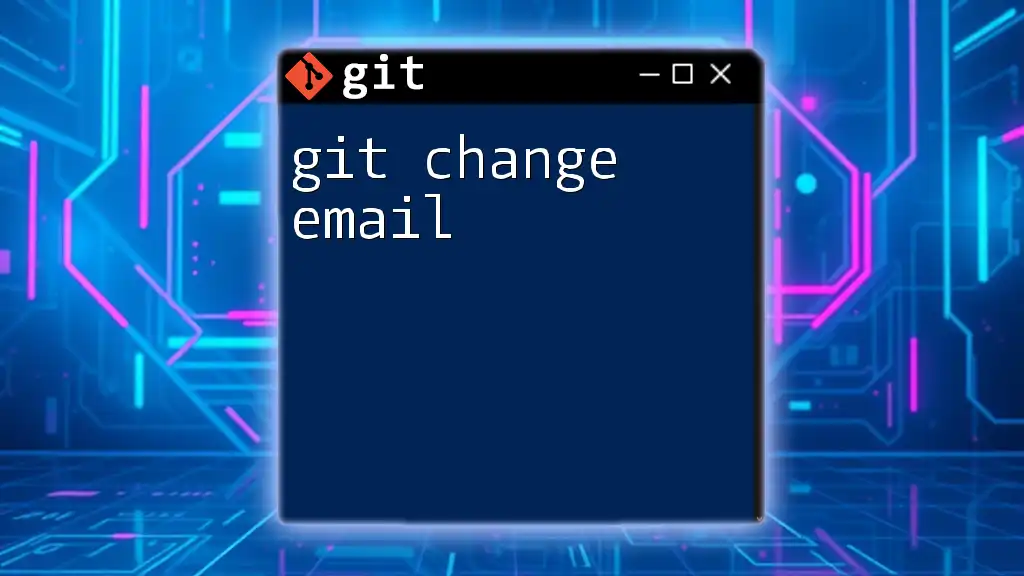
Best Practices After Cancelling a Merge
Review Your Changes
Following a merge cancellation, it's essential to closely review all changes that led to that decision. Use tools that allow you to compare differences between branches effectively. This will help you identify whether you need to try the merge again or pursue a different strategy.
Communicate with Your Team
In collaborative environments, open communication is vital. Inform your teammates about the cancellation, especially if it affects their workflow. Providing insights into why the merge was cancelled can foster better understanding and teamwork moving forward.
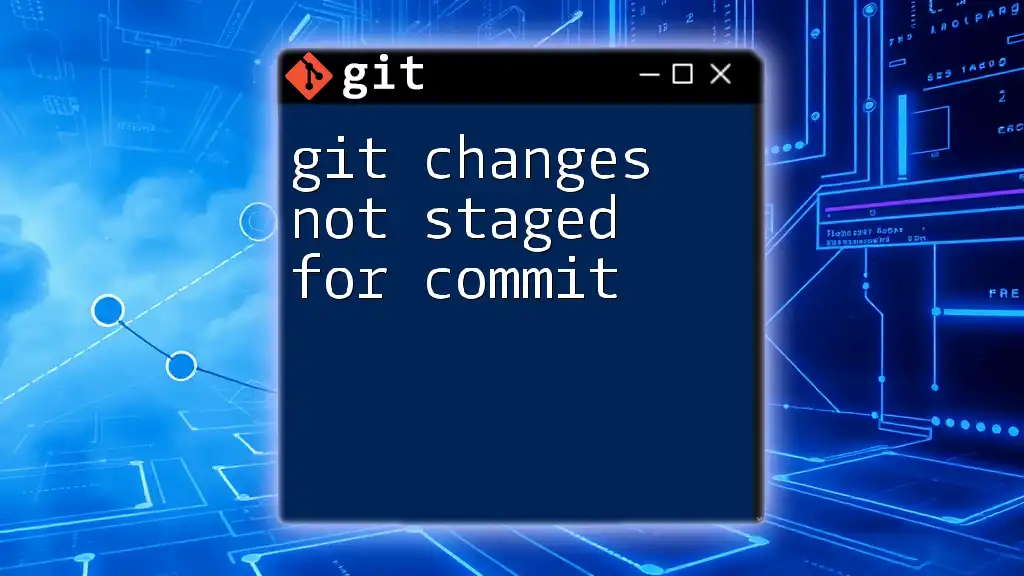
Conclusion
When working with Git, knowing how to cancel a merge is just as important as conducting a successful one. Whether through `git merge --abort`, resets, or other methods, being able to swiftly revert unwanted changes protects the codebase and smooths collaborative workflows.
Practice these commands to make sure you’re prepared for any future merging situations. Remember, the goal of version control is not just to manage code, but to streamline collaboration, which is the hallmark of any successful development project.
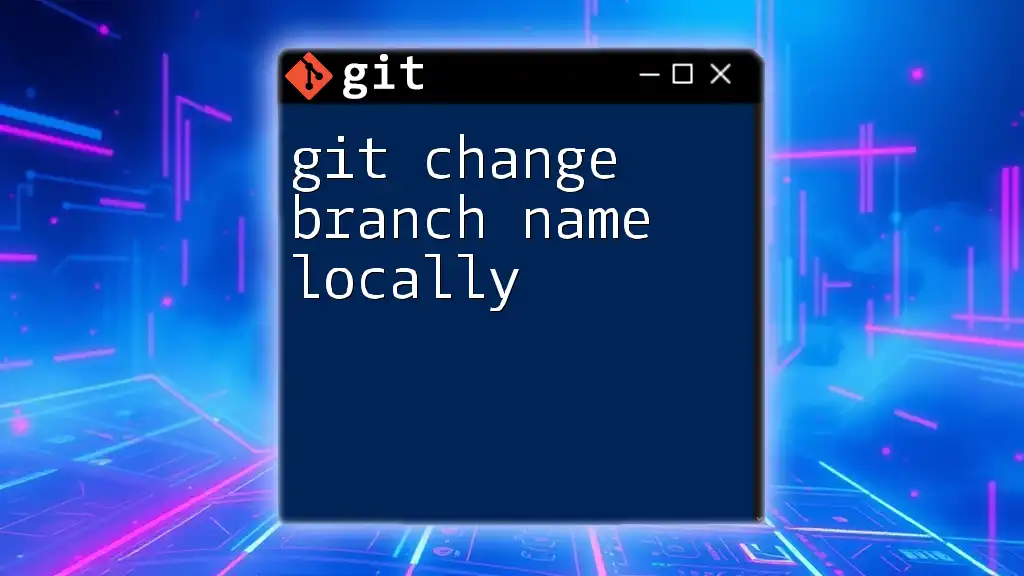
Resources and Further Reading
- For a deeper dive, refer to the [official Git documentation](https://git-scm.com/doc).
- Explore recommended books and courses focused on mastering version control with Git.
- Consider utilizing supplementary tools that enhance code collaboration and version control management.
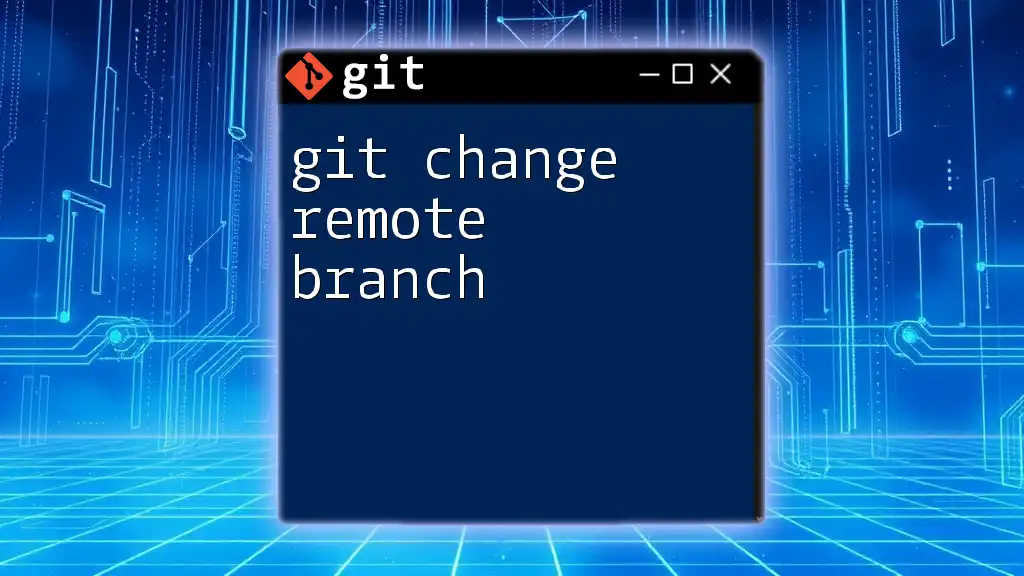
FAQs
Q1: What if I forgot to cancel my merge earlier?
You can still revert to a previous commit using `git reflog` to find the state you need.
Q2: Can I recover changes after a hard reset?
Hard resets permanently remove changes, but if you acted quickly, you may be able to use `git reflog` as a recovery option.
Q3: What if I prefer using a graphical user interface for Git?
Many GUI tools offer functionalities that allow you to cancel merges and manage branches easily, often with intuitive visual cues.
Feel free to share your experiences with cancelling merges or any questions you may have in the comments!