To change a file name in Git, use the `git mv` command followed by the current file name and the new file name.
git mv old_filename.txt new_filename.txt
Understanding the Git Workflow
What is Git?
Git is a powerful version control system that allows developers to track changes in their files and collaborate effectively. With features like branching and merging, Git supports teams in managing their codebases, ensuring that multiple developers can work simultaneously without overwriting each other's changes. Understanding Git is crucial for modern software development, as it enhances productivity and maintains project history.
Understanding File Tracking
Git tracks changes at the file level, recording the history of modifications made over time. When you alter a file, add new content, or rename it, Git registers these changes and permits you to revert to earlier versions if needed. Renaming files is an essential part of maintaining organized and scalable repositories. Using the correct methods to rename files ensures they remain tracked consistently and can be worked with effectively.
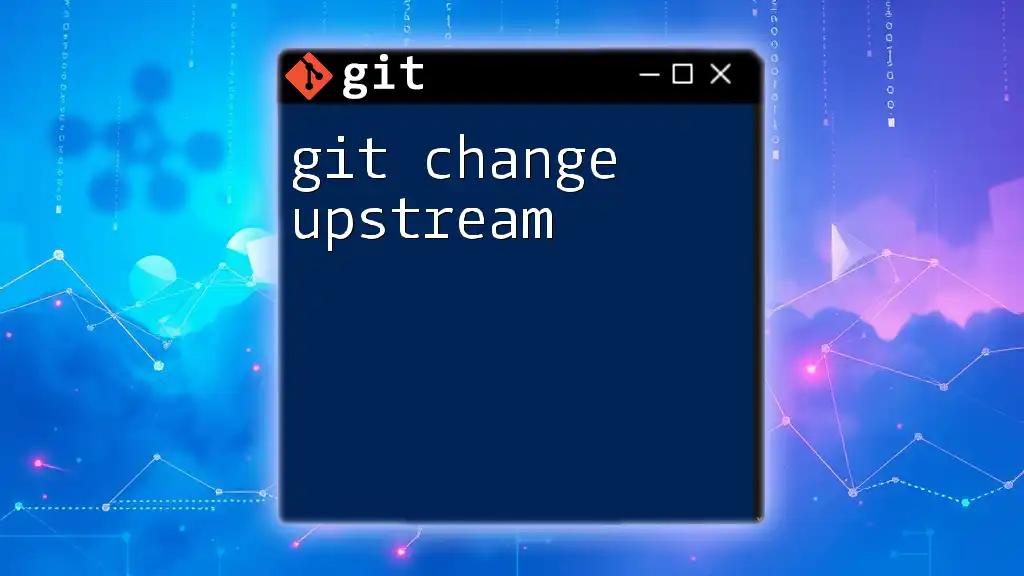
The Basics of Renaming Files in Git
Using the Rename Command
To change a file name in Git, the most straightforward approach is to use the `git mv` command. This command serves as an explicit instruction to rename files within the version control system.
Example Usage:
git mv <old_filename> <new_filename>
This command effectively tells Git to both rename the file and stage the change for the next commit. When executed, it keeps the file's history intact, connecting the new filename to the old one. This seamless transition is one of the primary benefits of using `git mv` instead of manually renaming the file and adding an additional step to stage the removed file.
Manual Renaming and Git's Tracking
Alternatively, you can rename a file using standard filesystem commands. For example, in a Unix-like environment, you might use the `mv` command:
mv <old_filename> <new_filename>
git add <new_filename>
git rm <old_filename>
While this method works, it requires you to run multiple commands to accomplish what `git mv` does in one step. Additionally, using this method could lead to discrepancies in Git's tracking, as the system doesn't automatically link the old and new filenames unless explicitly staged and committed.
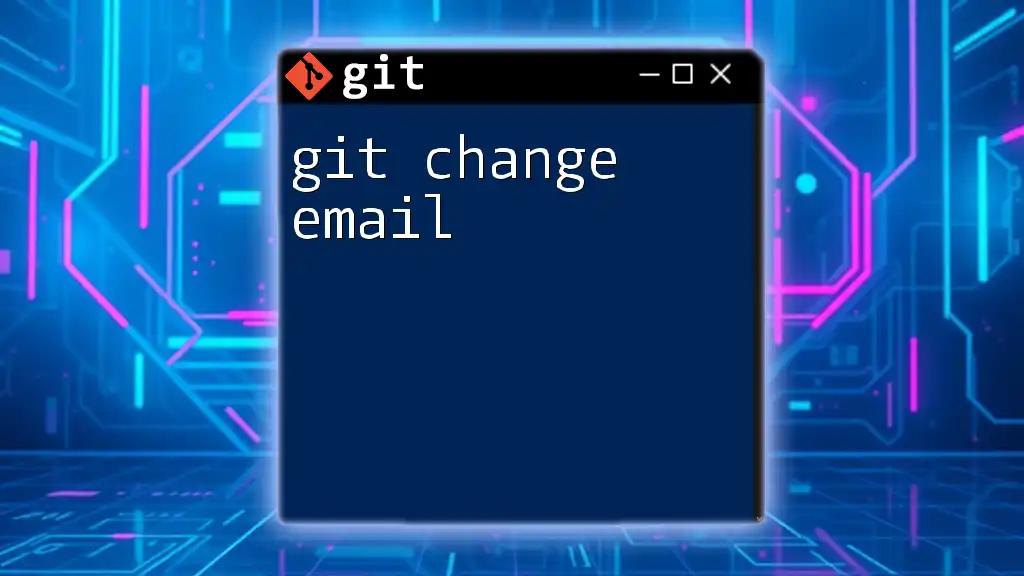
Committing the Renaming Changes
How to Commit File Renames
Once you have renamed a file, committing the change is vital. Committing creates a snapshot of your current changes, allowing you to revert to any stage in your project later.
Code for Committing Renames:
git commit -m "Rename <old_filename> to <new_filename>"
A meaningful commit message is essential, as it provides context for your changes. Good commit messages offer clarity on the purpose of the rename, which can be extremely helpful for collaborators reviewing your project history.
Viewing the Rename in Git History
Git maintains a detailed history, allowing you to track changes, including file renames. To view the history of a renamed file, you can use:
git log --follow <new_filename>
The `--follow` flag is crucial here; it ensures that Git follows the file's history across renames. This feature enables developers to understand the evolution of a file, even after it has been renamed.
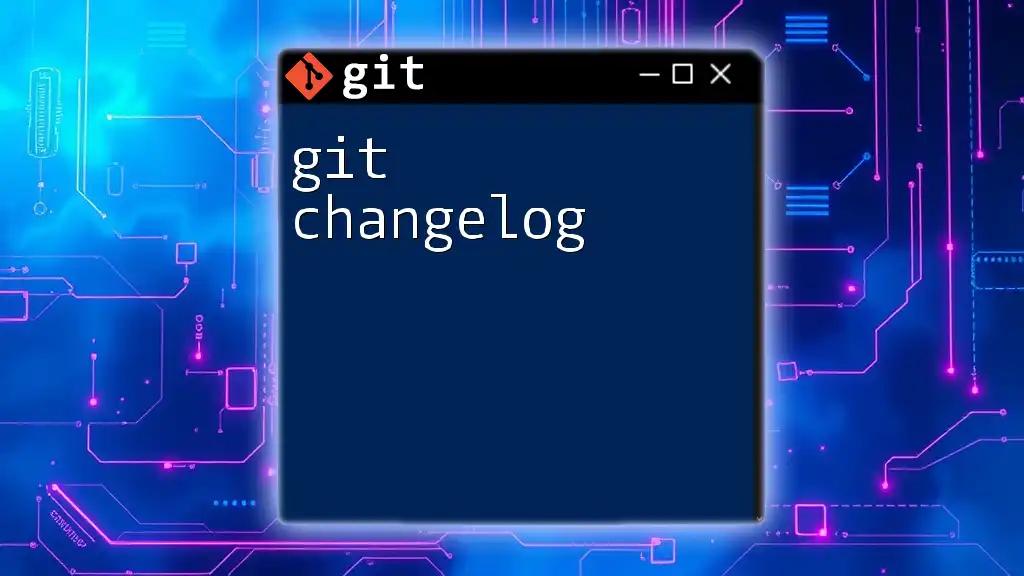
Best Practices for Renaming Files
Consistency in Naming Conventions
When renaming files, maintaining a consistent naming convention is crucial. Consistency enhances readability and makes it easier for you and your collaborators to navigate the codebase. Establishing and adhering to a naming standard improves project organization and can ultimately lead to improved productivity.
Avoiding Common Pitfalls
Renaming files can lead to mistakes if not done carefully. Common issues include forgetting to commit the changes, resulting in confusion over whether the file was renamed successfully. It’s also essential to be cautious of merge conflicts, especially if multiple branches have modified the same files. Always check for outstanding commits before renaming, and communicate with your team regarding major changes.
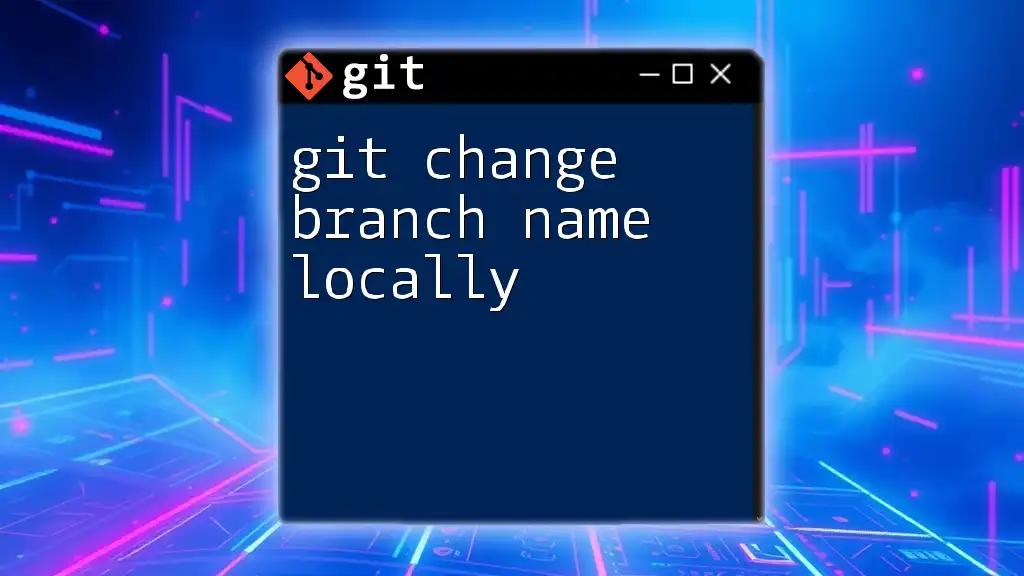
Advanced Renaming Techniques
Renaming Files in a Branch
If you're working in a branch, renaming files might affect other team members. When merging branches where the renamed files exist, Git will handle most renaming conflicts automatically. However, it’s vital to communicate any changes to your team and ensure that they’re aware of the new file structure.
Renaming Multiple Files
Renaming multiple files can be achieved efficiently using a series of `git mv` commands. For example:
git mv <old_filename1> <new_filename1>
git mv <old_filename2> <new_filename2>
Batch renaming can simplify processes, especially when managing a large number of files. Furthermore, you can use wildcards to rename files that share a common pattern, making organizational changes swift and uncomplicated.
Renaming Without Affecting History
In scenarios where preserving the original file history is critical, using `git mv` is the best method. However, if you must rename files and eliminate their history for privacy or legal reasons, consider using Git's `filter-branch` command with caution — it's a more advanced technique and may be best suited for those familiar with Git's inner workings.
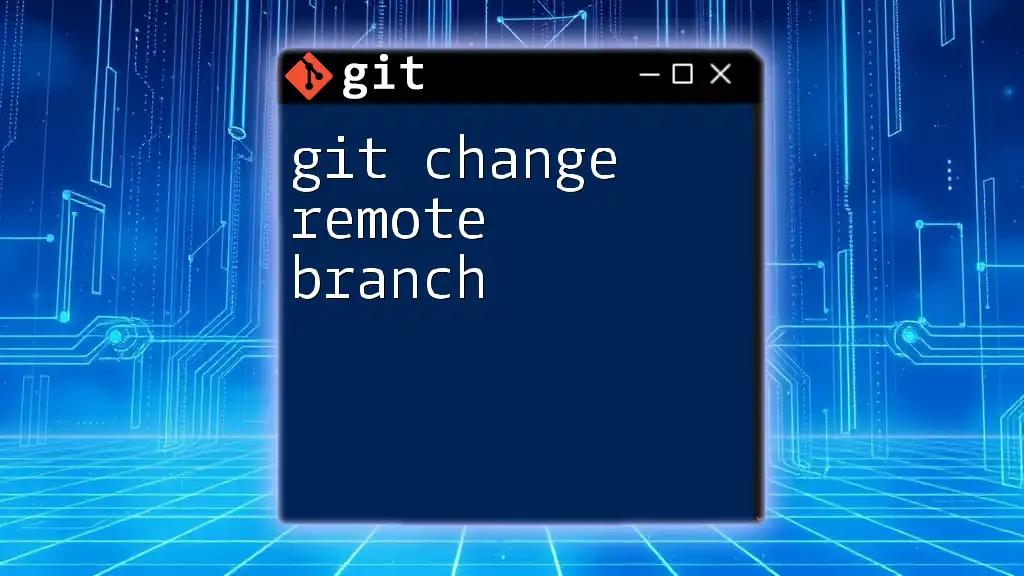
Conclusion
Renaming files in Git is not only a common task but also an essential skill for maintaining clear and organized codebases. By leveraging commands like `git mv`, understanding the commit process, and adhering to best practices, you can ensure a smooth workflow. Whether you're working solo or as part of a team, knowing how to effectively handle file renaming will streamline your version control efforts.
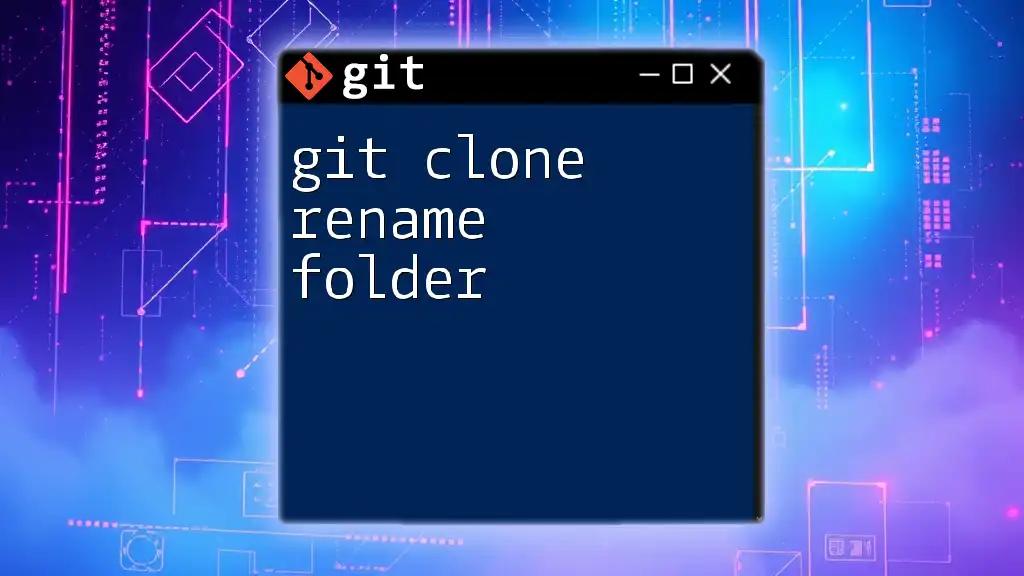
Additional Resources
Consider exploring additional tutorials, documentation, and community discussions related to Git. Engaging with such resources enhances your knowledge and can provide insights into more advanced Git functionalities. Don’t hesitate to reach out to community forums when you have questions or require assistance.
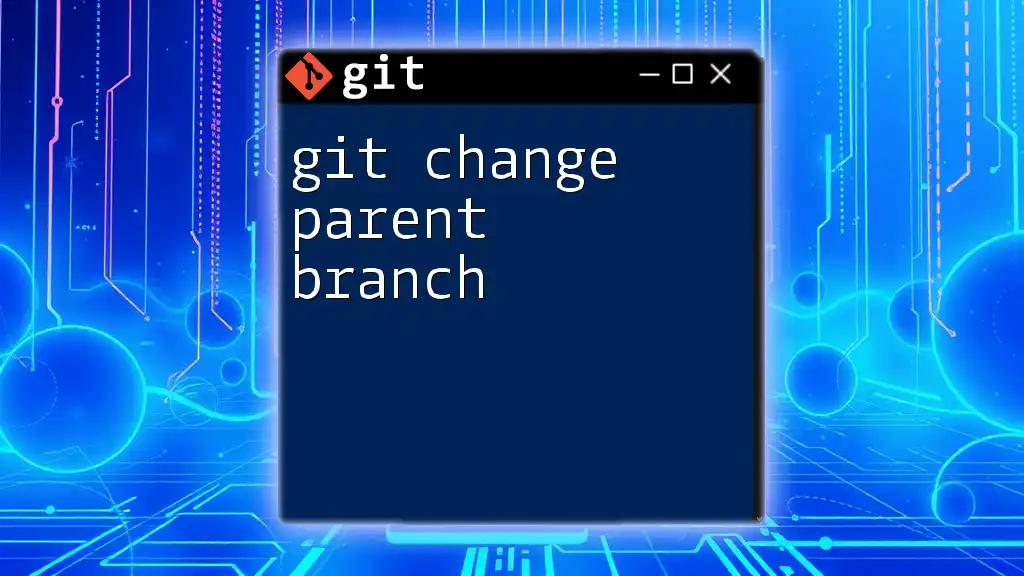
Call to Action
Share your experiences and tips regarding renaming files in Git! If you found this guide helpful, subscribe for more insights on mastering Git and version control. Your feedback and engagement contribute to enriching the Git learning community!