`git clang-format` is a command that uses ClangFormat to automatically format your code before committing changes to ensure consistency in coding style.
Here's a code snippet demonstrating how to use it:
git clang-format --style=file
What is Clang Format?
Clang Format is a powerful tool used for formatting source code. Developed as part of the Clang project, this utility provides a way to define and enforce a consistent coding style across various programming languages, primarily C, C++, Java, JavaScript, Objective-C, and more.
Definition and Purpose
At its core, Clang Format is designed to improve code readability and maintainability by automatically formatting code according to predefined style guidelines. Furthermore, it significantly reduces the discussion and disagreements around code styling, allowing developers to focus on the functionality of their code.
How Clang Format Works
Clang Format operates by parsing the source code and applying formatting rules based on the specified options. Key features include consistent indentation, line spacing, brace positioning, and wrapping styles. For developers, this means the possibility of configuring personal or team-wide coding styles that automatically apply to the code base.
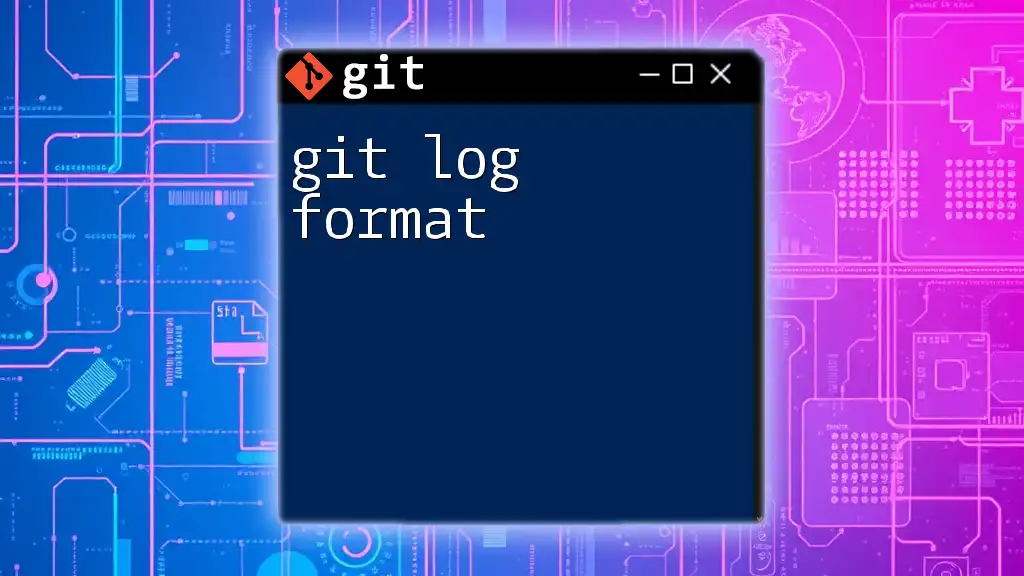
Integrating Clang Format with Git
Why Use Clang Format with Git?
Integrating Clang Format with Git brings several benefits, especially in collaborative software development:
- Consistency: By standardizing coding styles, teams can ensure uniformity across the codebase, leading to easier reading and reviews.
- Conflict Prevention: Automatic formatting significantly reduces the likelihood of style conflicts during merges, streamlining the collaboration process.
Setting Up Clang Format in a Git Repository
To utilize Clang Format in your Git repository, the first step is installation. Here is a general command you can use depending on your operating system.
# Example command for Ubuntu
sudo apt-get install clang-format
Once Clang Format is installed, it's essential to ensure it's ready for use within your Git environment.
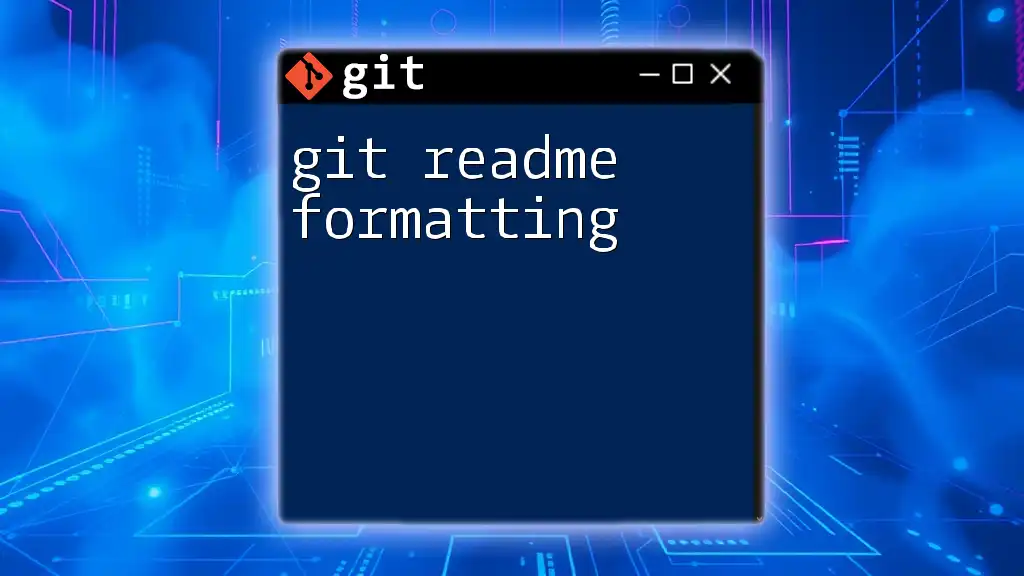
Configuring Clang Format
Creating a .clang-format File
The .clang-format file is a configuration file that defines the style guidelines your code should adhere to. By placing this file in your repository's root or any subdirectory, you establish that formatting style for files within those directories.
Here’s an example of a basic .clang-format file configured to follow Google's coding standards:
# Example .clang-format file for Google style
BasedOnStyle: Google
IndentWidth: 4
ColumnLimit: 120
This file can include numerous style options, allowing teams to customize preferences or select popular styles like LLVM, Mozilla, or WebKit.
Customizing Code Styles
Developers can go beyond standard styles to create custom configurations. For instance, you may want to adjust options such as `SpaceBeforeParens` or `AllowShortBlocksOnASingleLine`. Here's an example of a customized configuration:
# Custom .clang-format configuration
BasedOnStyle: LLVM
IndentWidth: 2
AllowShortBlocksOnASingleLine: Empty
SpacesInParentheses: false
This customization allows teams to align the formatting style even more closely with personal or organizational preferences.
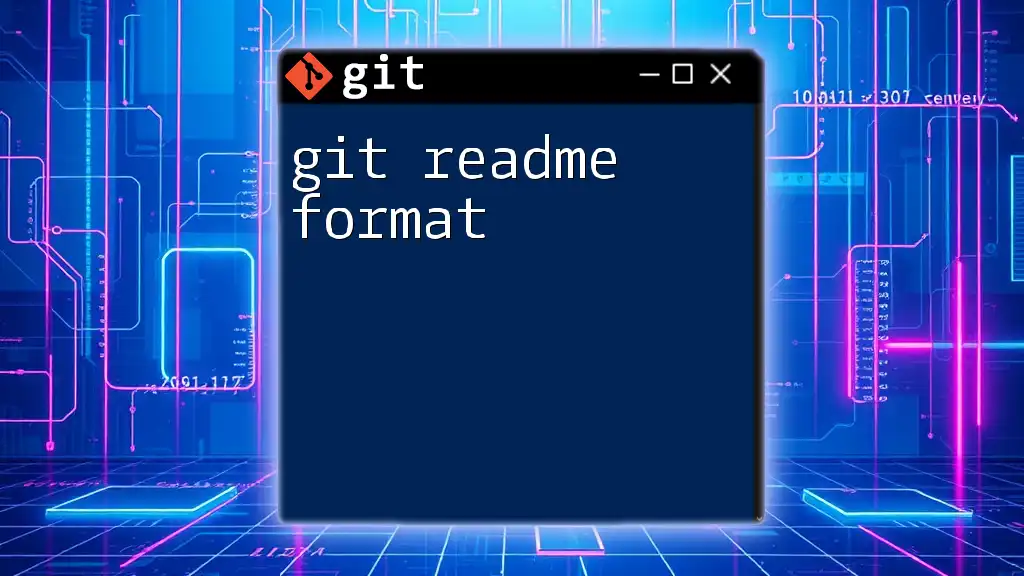
Using Clang Format in the Git Workflow
Formatting Code Before Committing
One practical aspect of using Clang Format is formatting your code quickly before committing it. Here’s how you can format your files efficiently:
clang-format -i path/to/file.cpp
The `-i` flag modifies the file in place, immediately applying the chosen formatting style.
Creating Git Hooks for Automatic Formatting
Git hooks are scripts that trigger during specific events in the Git workflow, making them perfect for ensuring code is formatted consistently. Setting up a pre-commit hook allows developers to run Clang Format automatically before each commit.
Here’s a simple pre-commit hook script you can use:
#!/bin/sh
# Pre-commit hook for formatting changes
files=$(git diff --cached --name-only --diff-filter=ACM | grep '\.[ch]pp$') # Adjust extensions as needed
if [ "$files" != "" ]; then
clang-format -i $files
git add $files
fi
This script checks for staged files that match specific file extensions, formats them using Clang Format, and re-stages the formatted files for a commit, ensuring that all code committed adheres to the specified formatting standards.
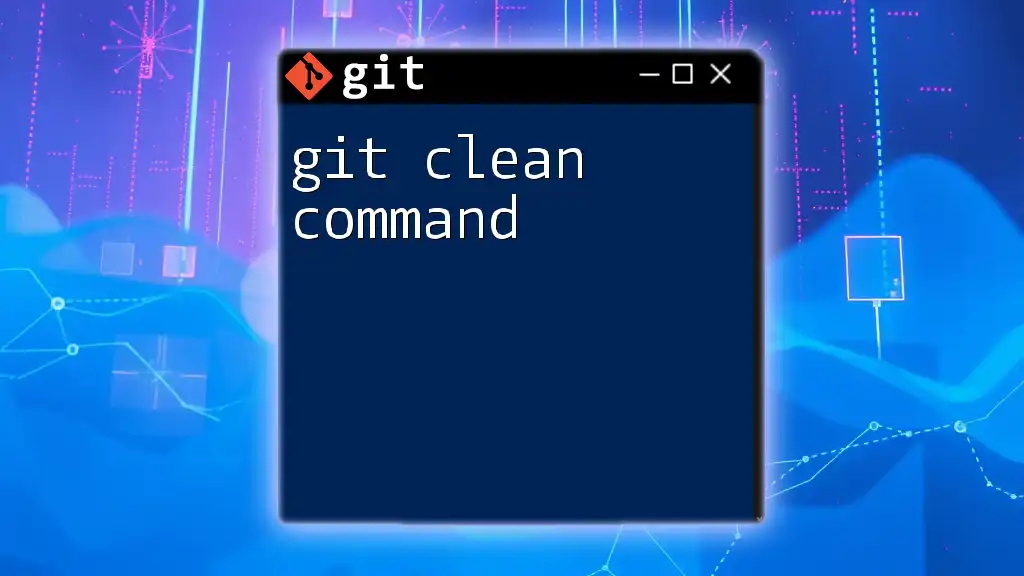
Clang Format Commands and Options
Command Line Usage
Clang Format offers various command-line options to cater to different formatting needs. A basic command to format a file or a set of files is as straightforward as:
# Formatting an entire directory
clang-format -i -style=file path/to/directory/*
The `-style=file` option tells Clang Format to read from the `.clang-format` file located in the specified directory, allowing for seamless integration.
Additional Tips for Effective Use
To leverage Clang Format effectively, consider these practices:
- Regularly run Clang Format before committing changes.
- Encourage team members to use the pre-commit hook to maintain consistency.
- Explore Clang Format’s options by running `clang-format --help` to familiarize yourself with the available commands and configurations.
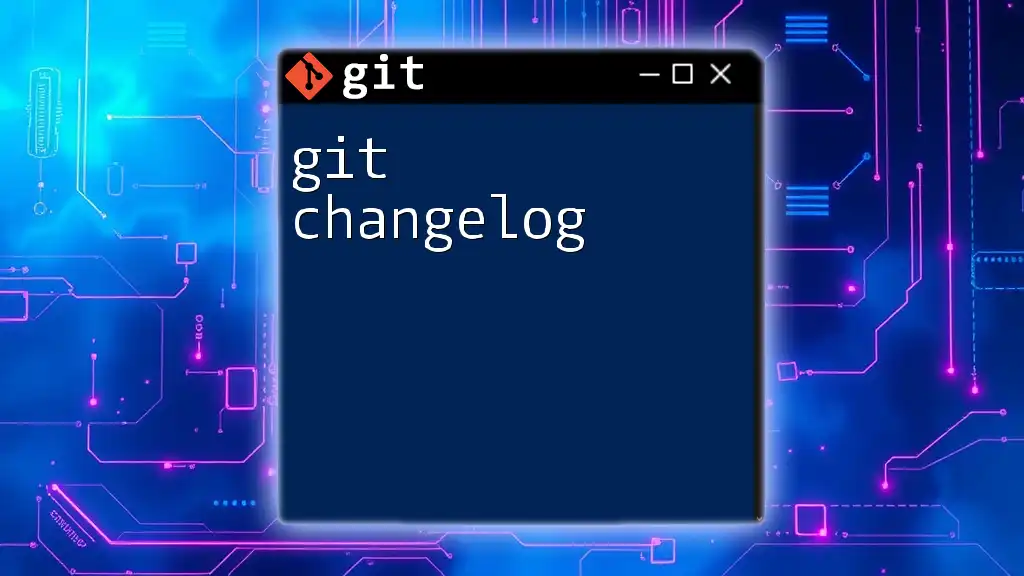
Conclusion
Incorporating git clang format into your development workflow significantly enhances code readability and maintains consistent style within a team. By automating formatting tasks, you can minimize discussions about coding styles, enabling you to focus on writing efficient and clean code. Embrace Clang Format to streamline your coding practices and improve collaboration in projects.
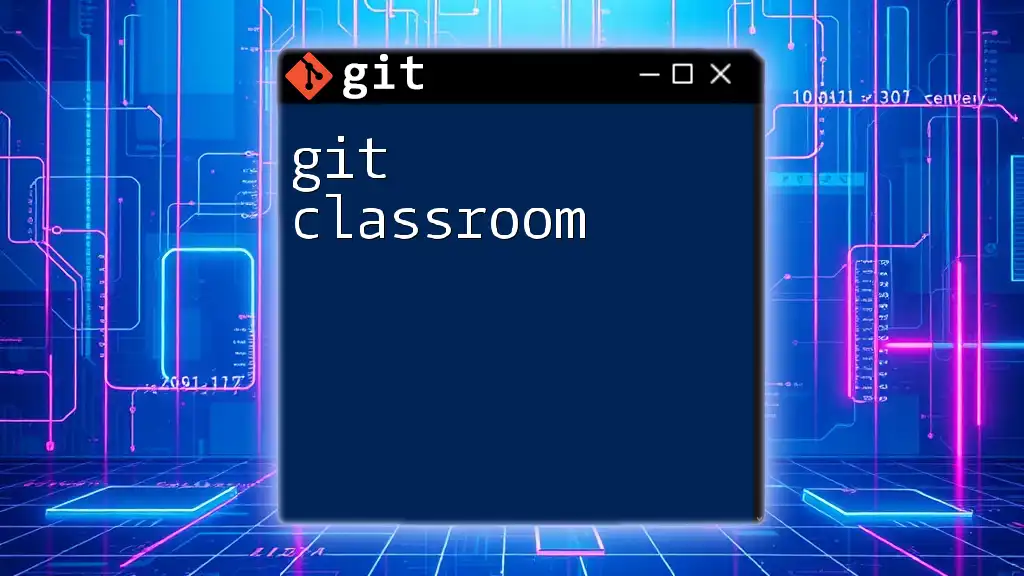
Additional Resources
To deepen your understanding of Clang Format, check out the official documentation, which provides comprehensive explanations and examples. Consider exploring tutorials and engaging with community forums or GitHub repositories dedicated to code formatting, where you can share insights and exchange ideas with fellow developers.
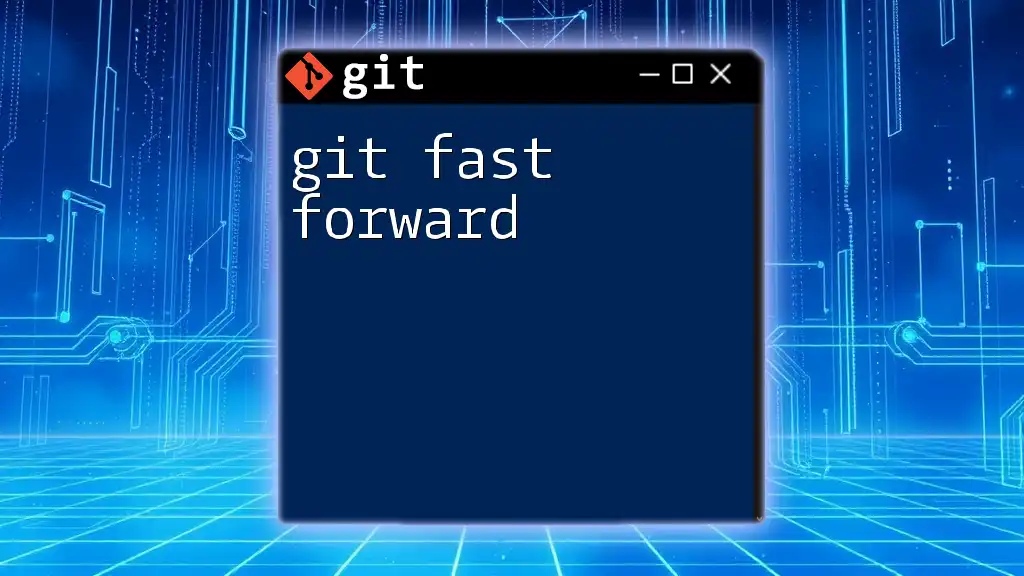
FAQs
What files should I format with Clang Format?
Clang Format is primarily designed for languages such as C, C++, and others supported by Clang. It's common practice to format source files ending with extensions like `.cpp`, `.h`, `.cc`, and `.c`, among others.
Can I integrate Clang Format with other tools?
Yes! Clang Format can be integrated into continuous integration (CI) pipelines, paired with other tools such as linters, ensuring that all code follows the same formatting rules automatically.
Is Clang Format only for C/C++ languages?
While heavily used for C and C++, Clang Format supports various languages, including Java and JavaScript. Its versatility makes it valuable for projects that require standardization across multiple languages.