In Git, "changes not staged for commit" refers to modifications in your working directory that have not yet been added to the staging area, which can be viewed using the command `git status`.
Here’s a code snippet to check the status:
git status
Understanding Git’s Staging Area
What is the Staging Area?
The staging area in Git is a crucial intermediary between your changes and the final repository history. It acts like a clipboard: you gather your modifications here before officially committing them to your repository. This allows you to control exactly what changes you want to include in your commit.
Staging vs. Committing
To grasp the concept of “git changes not staged for commit,” it’s essential to recognize the difference between staging and committing. Staging means you are preparing specific changes to be saved, whereas committing captures the state of your repository at that moment.
- Staging: Prepares changes.
- Committing: Finalizes and records those changes.
This step-by-step approach helps maintain a clean and organized repository.
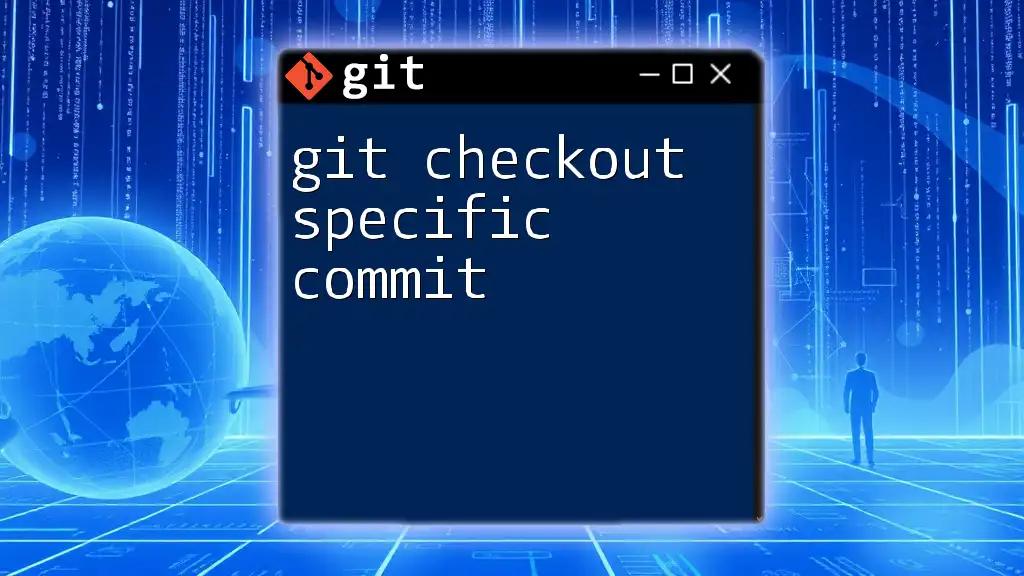
What Does “Changes Not Staged for Commit” Mean?
Definition
When you encounter the message "changes not staged for commit," it indicates that there are modifications in your working directory that have not yet been added to the staging area. In simpler terms, these changes exist in files you have altered since the last commit, but Git has not been instructed to track these updates.
Situations Leading to this Message
This message appears under common scenarios, such as:
- You modified files but didn’t stage them.
- You deleted files or renamed them without updating Git.
When you perform these actions, Git maintains the status quo until you provide explicit instructions to stage the changes.
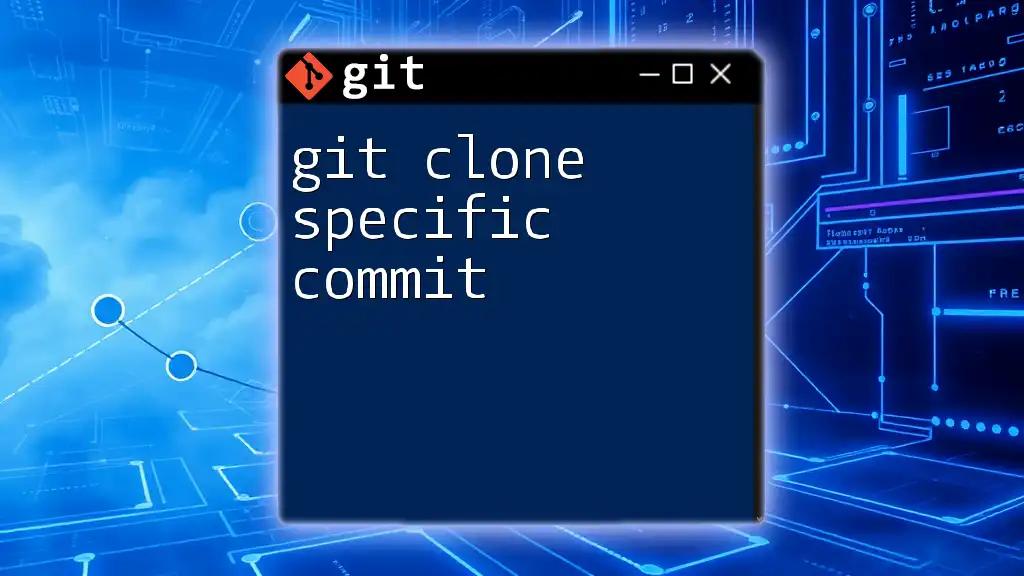
Checking the Status of Your Repository
Using the `git status` Command
To see what changes are not staged for commit, you can use the command `git status`. This command provides a snapshot of your Git repository’s current state.
git status
The output might read:
On branch main
Changes not staged for commit:
modified: file1.txt
deleted: file2.txt
This output clearly highlights files that you have altered but haven’t staged yet.
Understanding Change Types
The status output clearly separates the types of changes that are relevant:
- Modified: Shows files that you have edited since the last commit.
- Deleted: Indicates files that you have removed.
- Added: Refers to any new files that Git hasn't yet begun tracking.
These distinctions are essential for managing your repository effectively.
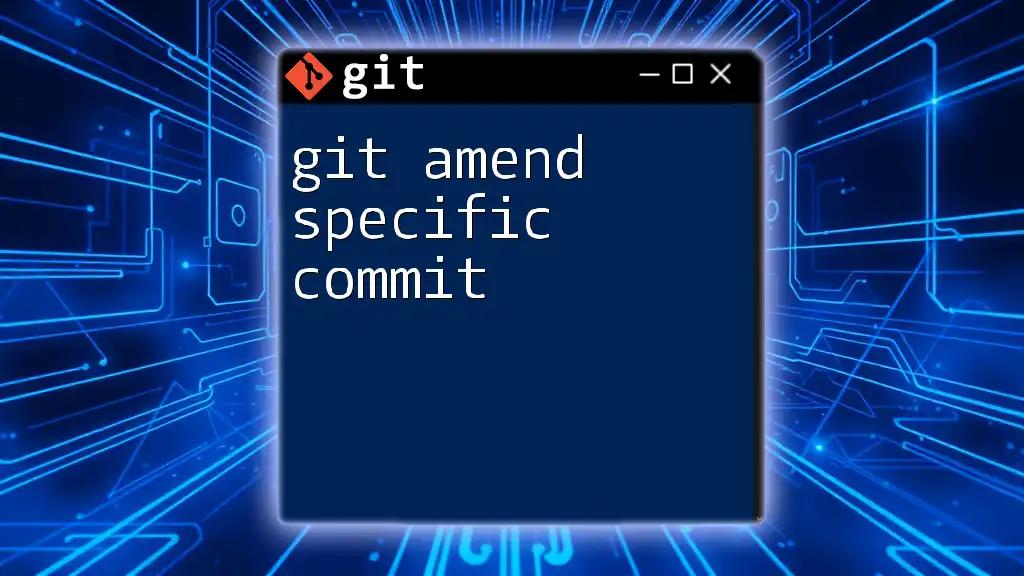
How to Stage Changes
Staging Modified Files
To stage files that you have modified, use the command `git add`, followed by the specific filename.
git add <filename>
For example, if you have made changes to `file1.txt`, run:
git add file1.txt
This command tells Git that you want to include the changes made in `file1.txt` in your next commit.
Staging All Changes
If you have made multiple modifications across several files and want to stage them all at once, use a wildcard to stage everything in your current directory:
git add .
This command effectively stages all changes, including newly created and modified files, which can be very handy when working on larger projects.
Staging Specific Types of Changes
In cases where you want to stage only certain file types (such as `.txt` or `.css` files), you can specify the file type:
git add *.txt
This command will stage all text files in the current directory, saving you time and ensuring you only process relevant changes.
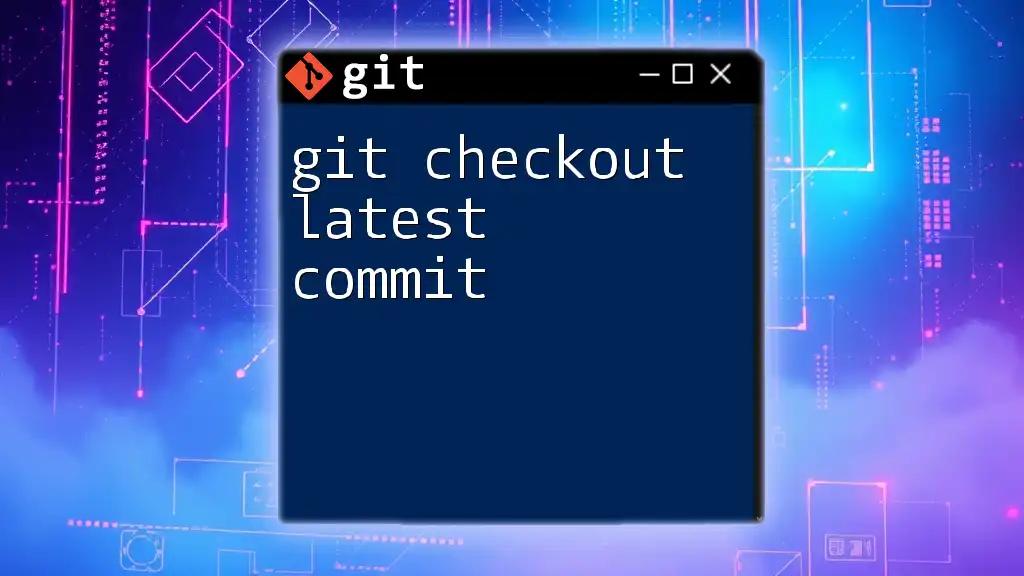
Undoing Changes in the Staging Area
Unstaging Changes
If you accidentally staged the wrong file, you can easily unstage it using the `git reset` command:
git reset <filename>
For example, if you wish to unstage `file1.txt`:
git reset file1.txt
This command will move the specified file back from the staging area to the modified state.
Reverting Otherwise Staged Changes
If you want to discard all changes in a file and restore it to the last committed state, use the `git checkout` command:
git checkout -- <filename>
For instance, if you decide to abandon your changes in `file1.txt`, run:
git checkout -- file1.txt
This command removes unsaved changes, reverting the file back to how it was at the last commit.
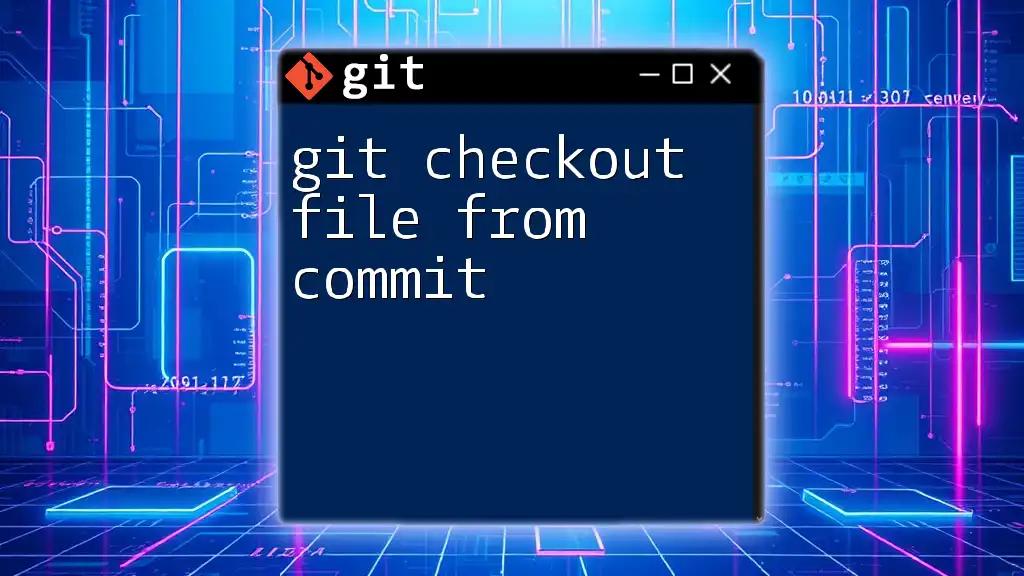
Best Practices
Commit Often
Frequent commits are a best practice that ensures you always have a recorded history of your work. It allows you to trace back your steps easily and helps maintain clarity in collaboration with others.
Use Descriptive Commit Messages
When committing changes, always use meaningful messages that succinctly describe what was done. This practice not only aids your understanding but also facilitates collaboration when others view the commit history.
Review Changes Before Committing
Before finalizing any commit, use the `git diff` command to review what changes you are about to stage and commit:
git diff
This command provides a comprehensive view of the alterations made since the last commit, helping you catch any unintended changes.
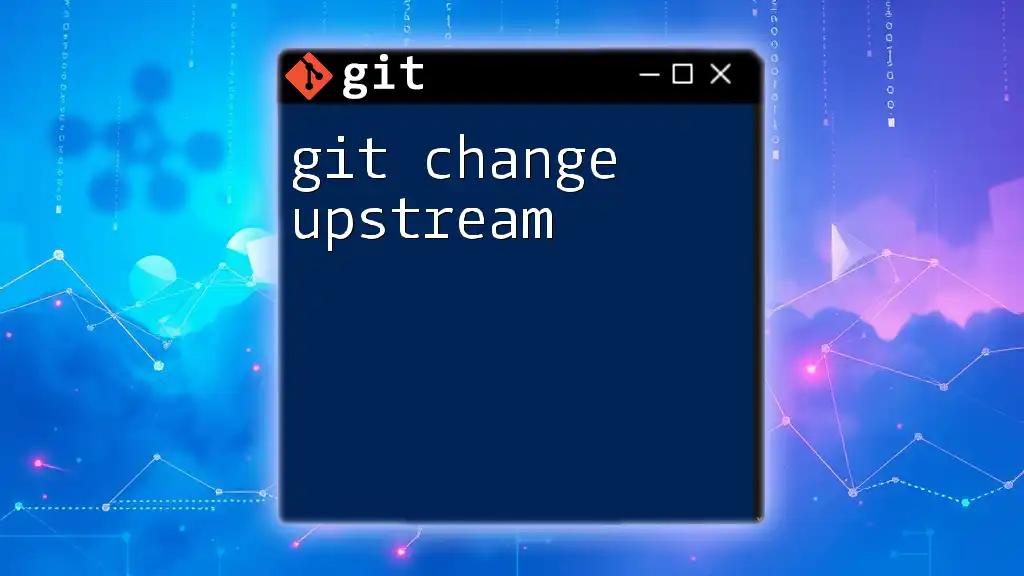
Troubleshooting Common Issues
Accidental Changes Not Staged
A frequent scenario is forgetting to stage modified files before committing. To avoid this, make it a habit to regularly use the `git status` command to double-check the state of your working directory.
Understanding the Risks
Staging incorrect changes can lead to a disorganized commit history and can make future troubleshooting difficult. Always ensure you have staged exactly what you intend to commit.

Conclusion
Understanding "git changes not staged for commit" is essential for effective Git usage. By mastering the staging area and leveraging related commands, you build a strong foundation for managing your projects efficiently. Regular practice of staging and committing effectively will greatly enhance your productivity and confidence in using Git.
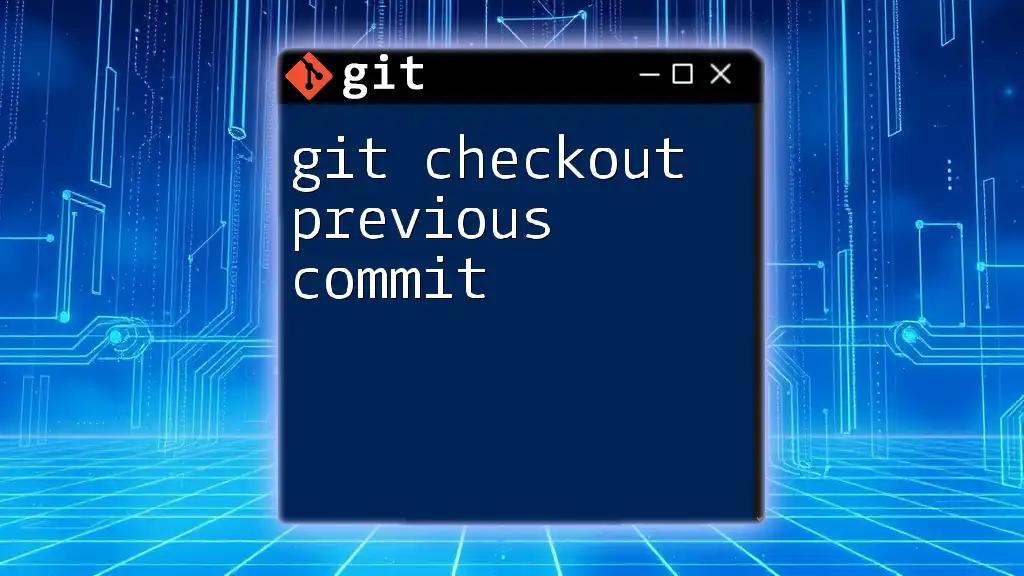
Additional Resources
Useful Git Commands Cheat Sheet
Consider exploring cheat sheets that summarize essential Git commands for quick access and easy reference.
Git Learning Platforms
Look for platforms offering courses and tutorials to help reinforce your learning and confidence in using Git, greatly enhancing this crucial skill in any developer's toolkit.