You can clone a specific commit from a Git repository by first cloning the entire repository and then checking out the desired commit using the following commands:
git clone <repository-url>
cd <repository-directory>
git checkout <commit-hash>
Understanding Commits in Git
What is a Commit?
A commit in Git represents a snapshot of your project at a particular point in time. Each commit has a unique identifier, known as a hash, which allows you to reference changes precisely. Commits play a critical role in tracking modifications to files and maintaining a history of the project's evolution.
How Commits Work
Commits include a few vital components:
- Tree: This represents the state of the files at the time of the commit.
- Parent: Each commit (except the initial commit) points to its predecessor, forming a linked list of history.
- Message: A brief description given by the developer explaining what changes were made.
Understanding these elements will help clarify how to work effectively with commits in your repositories.
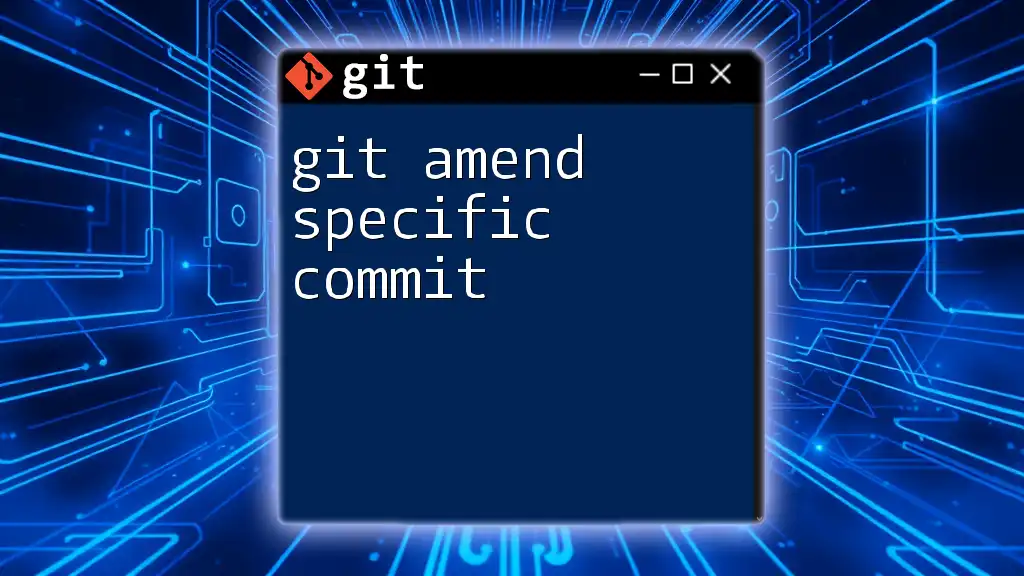
Cloning a Repository
What is Cloning?
Cloning a repository means creating a local copy of a remote project. This operation allows developers to access, modify, and manage the code without impacting the original source or other users.
Basic Clone Command
The fundamental command for cloning a Git repository is:
git clone <repository-url>
When you run this command, Git copies all the files, branches, and commit history from the remote repository to your local machine. It provides a robust groundwork for further collaboration and development.
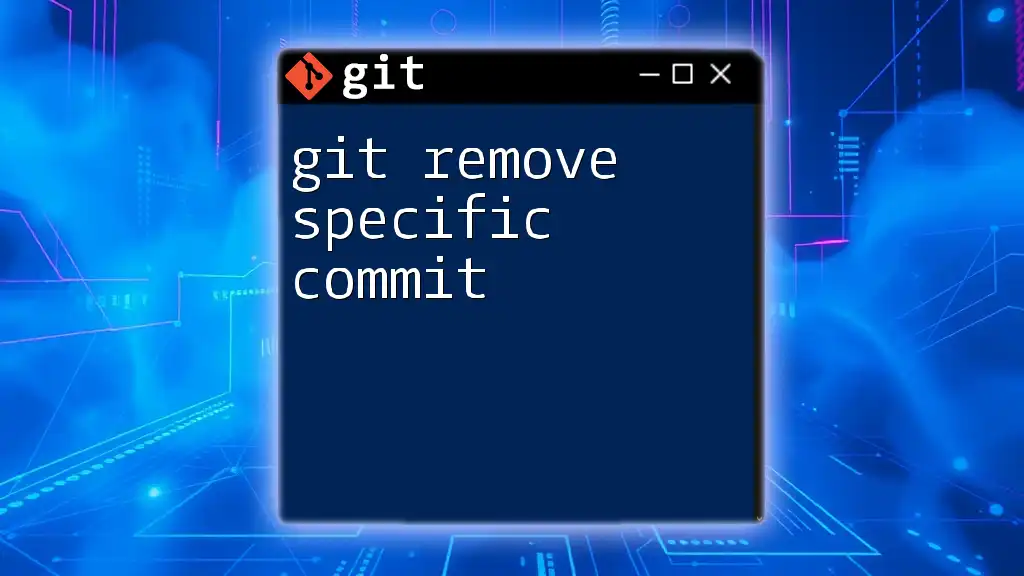
Cloning a Specific Commit
Why Clone a Specific Commit?
There are scenarios where you may want to access the state of your project at a particular commit, such as debugging or reviewing changes. Cloning a specific commit directly is not straightforward; instead, you will clone the full repository and then check out the desired commit. This process provides you a complete context, ensuring you can see all relevant changes.
Step-by-Step Guide to Clone a Specific Commit
Step 1: Clone the Repository
First, you need to clone the entire repository. This ensures you have access to the commit history and necessary data.
git clone <repository-url>
cd <repository-name>
By executing these commands, you obtain a full local copy of the repository, including all commits.
Step 2: Checkout the Specific Commit
Once inside your cloned repository, you can check out the specific commit you’re interested in by using the following command:
git checkout <commit-hash>
Note: When you checkout a specific commit, you enter a detached HEAD state indicating that you are not on any active branch. This is perfectly valid for examining past commits, but remember to create a new branch if you intend to make changes.
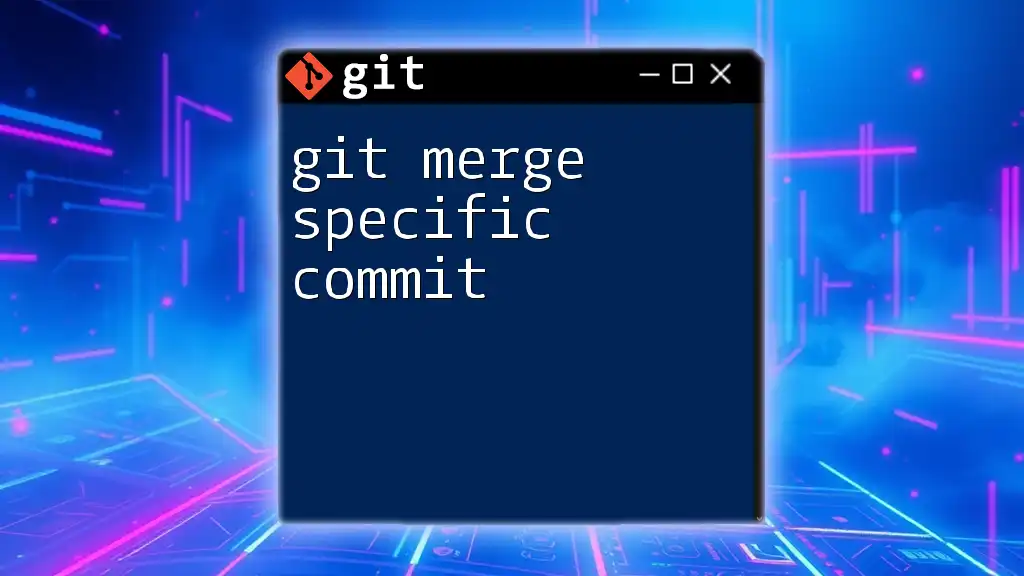
Alternative Approach with Shallow Clone
What is a Shallow Clone?
A shallow clone is a copy of a Git repository that's fetched with a limited history. This means you only get the latest commits—ideal for quick access when the complete history isn't necessary.
Cloning a Specific Commit with Depth
If you're looking to clone a branch along with its latest commit without fully downloading the entire history, you can use the depth option. Here’s how to do it:
git clone --depth 1 --branch <branch-name> <repository-url>
This command will create a shallow clone of the specified branch while limiting the history to just the most recent commit. However, keep in mind that if you need to access older commits, you will have to fetch them later.
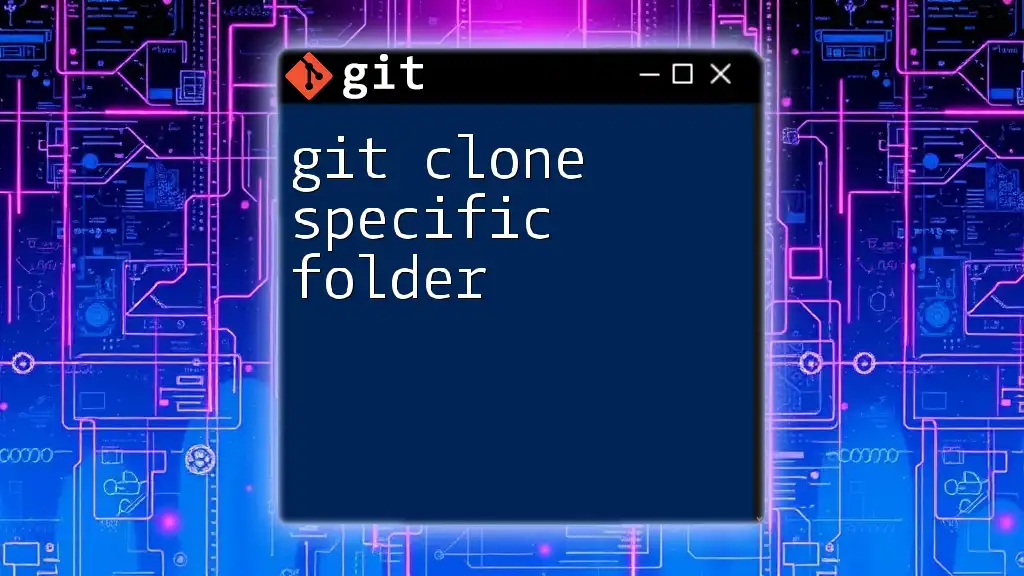
Important Considerations
Detached HEAD State
When you check out a specific commit, you will find yourself in a detached HEAD state. This means you're not on an active branch, which can lead to confusion if you're not prepared for it. If you decide to make modifications in this state, you should create a new branch to retain your changes:
git checkout -b <new-branch-name>
Doing this lets you safeguard your changes while still being able to go back to any branch or commit without losing the newly created work.
Limitations of Cloning Specific Commits
While cloning a specific commit offers advantages, it also has limitations. For one, you may lack the context provided by the full commit history, which may hinder your understanding of subsequent modifications. Furthermore, shallow clones may lead to limitations when trying to push changes back to the remote repository, as you will not have a complete commit history to reference.
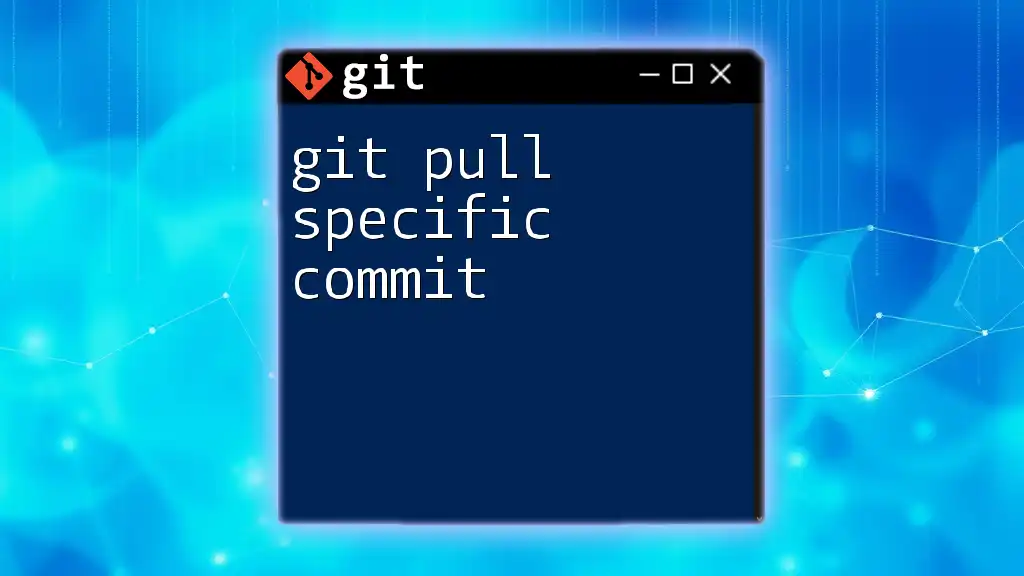
Example Use Cases
Use Case 1: Bug Fixing
Suppose you encounter a bug in your application that you know existed in a previous commit. Cloning the repository and checking out that specific commit allows you to investigate the code as it was during that time, aiding in identifying the source of the issue.
Use Case 2: Learning from History
If you are trying to understand how your project has evolved or learn new coding practices, examining specific commits can be very enlightening. By accessing previous versions of the code, you can gain insights into what changes led to current implementations and, potentially, better methods to achieve similar results.
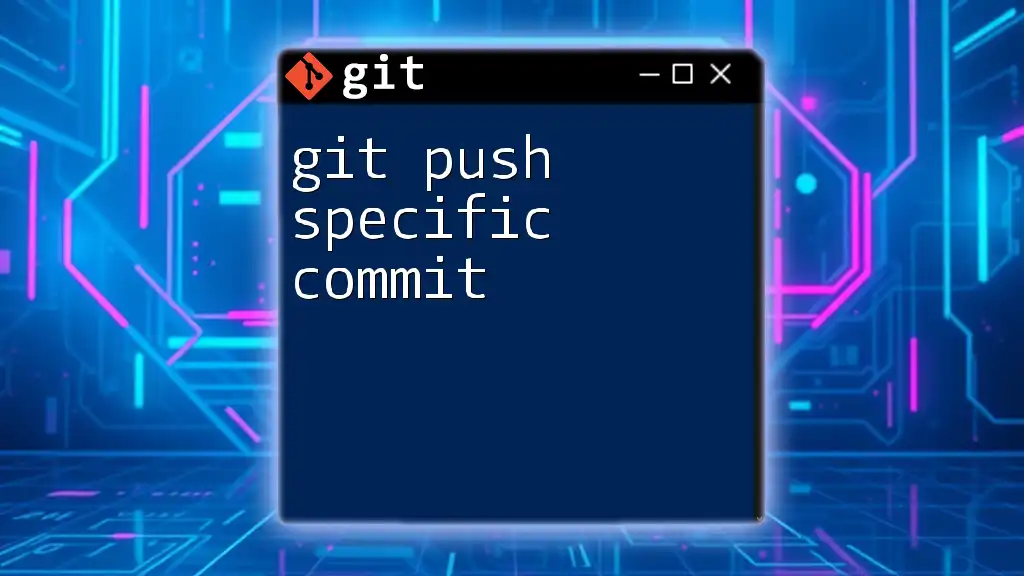
Conclusion
Cloning a specific commit in Git can be an essential technique for various situations, from debugging to code review. By understanding how to clone a repository and checkout particular commits, you position yourself to handle code more efficiently.
Mastering this process will never go out of style, as version control is an integral part of every developer's life. Embrace these techniques and explore the world of Git—there is always something new to learn.