To push a specific commit to a remote repository in Git, you can use the following command that references the commit hash in a push operation.
git push origin <commit-hash>:branch-name
Understanding Git Commits
What is a Git Commit?
A git commit is essentially a snapshot of your changes in a Git repository. Every time you commit, Git records the current state of your files, along with a message describing what was changed. This allows you to track the evolution of your project over time and revert back to previous states if needed. The structure of a basic commit command is simple:
git commit -m "Your commit message"
In this command, `-m` signifies that you're providing a message inline. Your commit message should be clear and descriptive to explain the changes made.
Identifying Commits
To view your commit history, you can use the `git log` command. This command provides a chronological listing of all past commits, along with their unique hashes and messages. A convenient way to display your commit history in a single line format is as follows:
git log --oneline
Each commit is associated with a unique commit hash. This hash is crucial when you need to reference a specific commit for operations like pushing that commit to a remote repository.
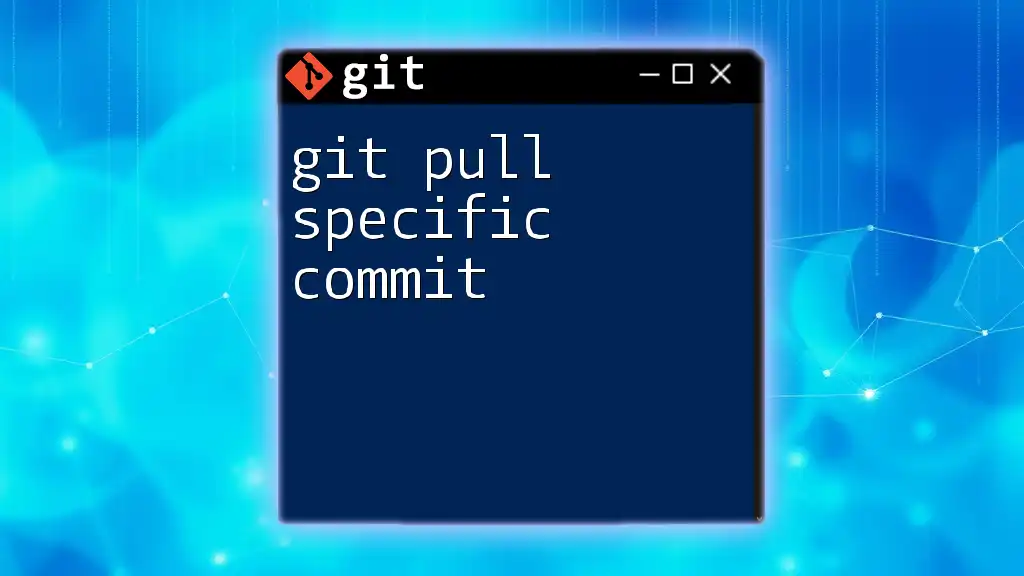
Git Push Basics
What Does Git Push Do?
The `git push` command is the mechanism through which you send your commits from your local repository to a remote repository. When you push, you’re updating the remote repository with your local changes, effectively sharing your work with others.
Push vs. Pull
While `git push` uploads your changes, `git pull` serves the opposite function. It fetches updates from the remote repository and merges them into your local repository. Understanding the distinction between these commands is vital for effective collaboration, ensuring your local environment remains in sync with team updates.
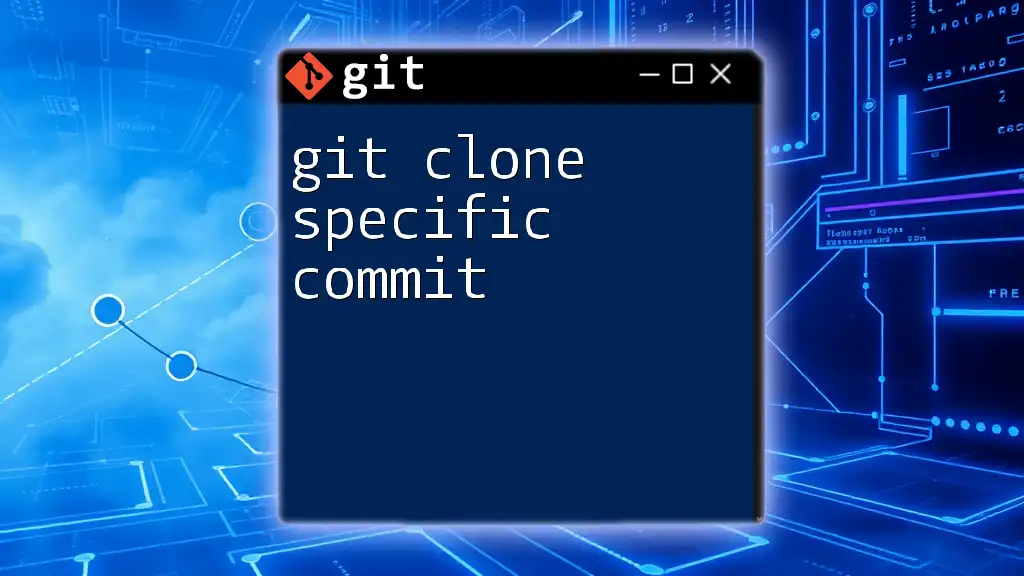
Pushing a Specific Commit
Why Push a Specific Commit?
There are several situations where pushing a specific commit becomes essential. For example, you might want to revert to a previous state, implement cherry-picking, or share incremental updates without affecting the latest changes on your branch. This provides flexibility in managing your version history, focusing only on what’s necessary.
The `git push` Command Syntax
When it comes to pushing a specific commit, typically, you'll need to utilize the command structure:
git push [remote] [commit]:[branch]
Here, `[remote]` usually refers to `origin`, the default name for your remote repository, while `[commit]` refers to the specific commit hash you wish to push. Finally, `[branch]` is the branch in your remote repository to which you wish to push the commit. Understanding this syntax is crucial for effective version control.
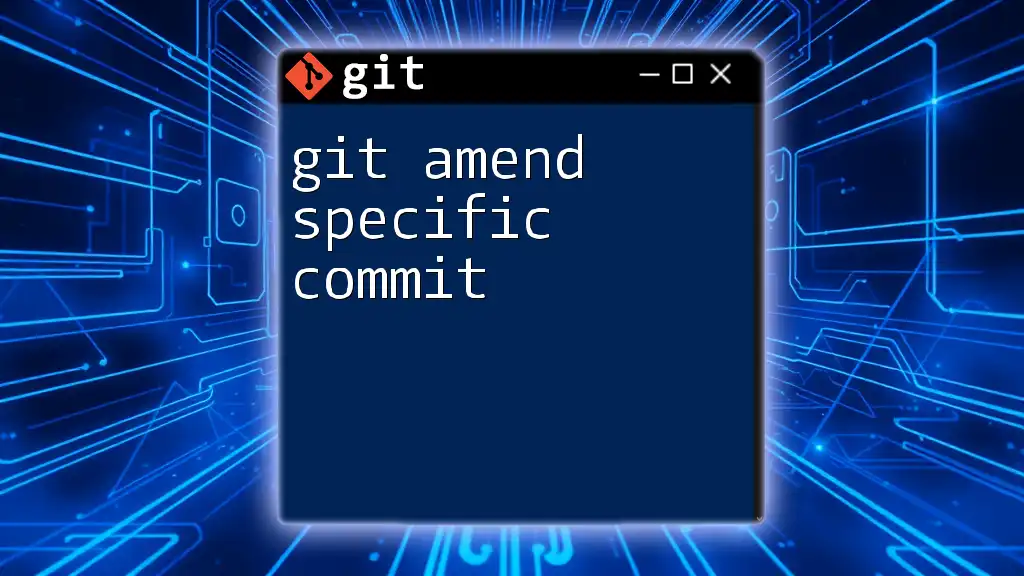
Using Git Cherry-Pick
What is Git Cherry-Pick?
Git cherry-pick is a command that allows you to apply the changes introduced by a specific commit from one branch to another. This is particularly useful if you want to selectively merge changes without merging the entire branch. For instance, if you have a commit in a feature branch that you wish to apply to the main branch, cherry-picking can accomplish this effectively.
How to Cherry-Pick a Commit
Here’s how you can cherry-pick a commit. First, you would identify the commit you need to apply using `git log`, and then you would execute the following command:
git cherry-pick <commit-hash>
Replace `<commit-hash>` with the actual hash of the commit you want to apply. This command takes the changes made in that specific commit and applies them to your current branch. Cherry-picking is a powerful tool when you want to custom-tailor your project's history.
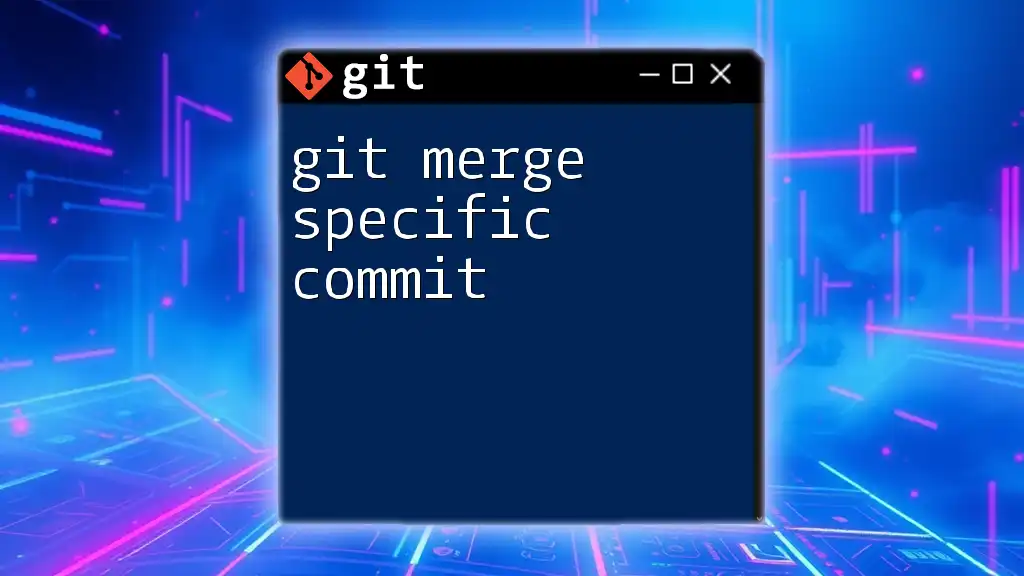
Pushing the Cherry-Picked Commit
Steps to Push the Cherry-Picked Commit
After you have cherry-picked your commit, it’s crucial to push these changes to the remote repository. Here are the steps to follow:
-
Verify the changes made: To ensure that the commit was applied correctly, you can check your current state with:
git status
-
Push the cherry-picked commit: Once verified, the following command will push your changes:
git push origin <branch-name>
Replace `<branch-name>` with the name of the branch you are working on. Executing this command updates the remote repository with the newly cherry-picked commit.
Best Practices for Pushing Specific Commits
It’s essential to adopt best practices when pushing specific commits:
- Keep your commit messages clear: This aids in collaboration and tracking changes within your project.
- Communicate with your team: Inform your team members when you’re pushing specific commits to avoid confusion.
- Review your commit history frequently: Regularly inspecting your Git history helps in recognizing which commits are relevant to push, ensuring a clean and organized repository.
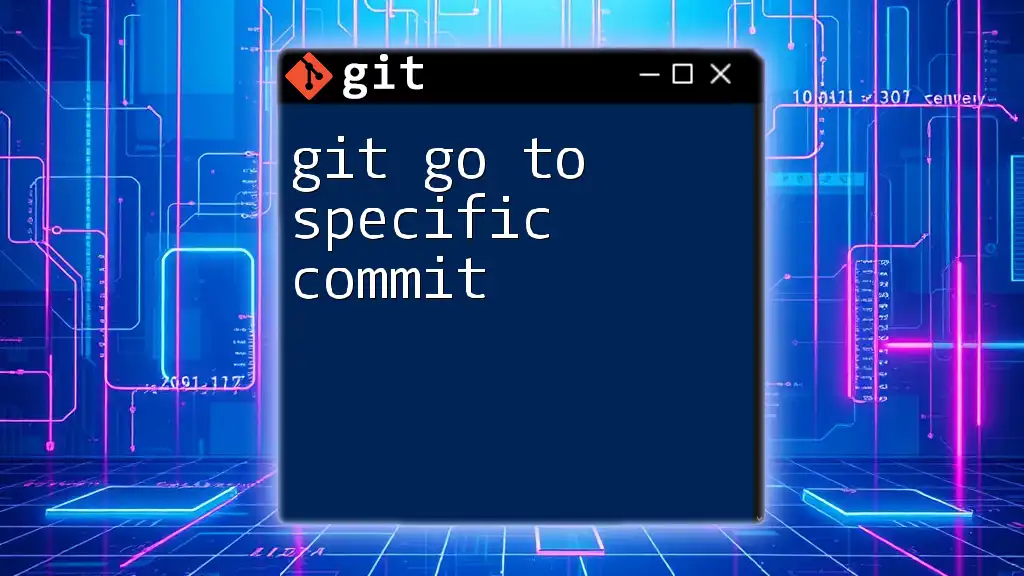
Troubleshooting Common Issues
Common Problems When Pushing Specific Commits
While pushing specific commits, you may encounter some common issues such as conflicts. These occur when the branch you are pushing to has changes that conflict with your commits. Fast-forward issues may also arise, particularly if the remote branch has diverged.
How to Resolve Issues
If you encounter conflicts, Git will notify you. To resolve these conflicts, you can utilize the `git mergetool` command, which opens a visual tool to help you manage the discrepancies.
git mergetool
By resolving conflicts carefully and pushing the corrected state, you ensure your repository remains stable and collaborative efforts continue smoothly.
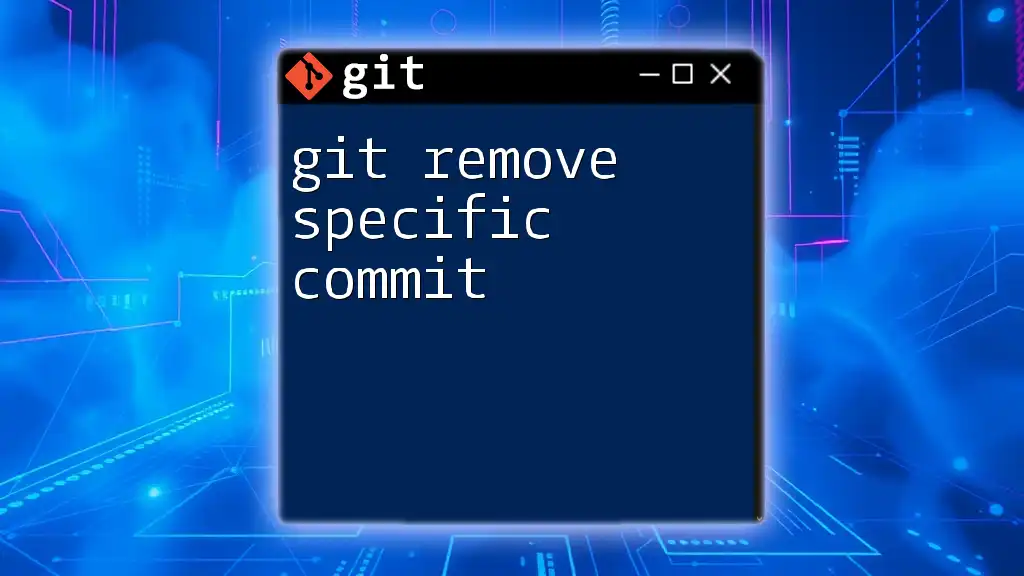
Conclusion
In conclusion, understanding how to git push specific commit opens up a world of possibilities for managing your code changes efficiently. By mastering the commands, such as cherry-picking and resolving conflicts, you can enhance both your individual productivity and your collaborative efforts within a team. Practice these commands often to gain confidence and fluency in your Git usage. Encourage fellow developers to join this journey of mastering Git.