To pull a specific commit in Git, you can use the following command to reset your local branch to that commit while keeping the changes in your working directory:
git fetch origin
git cherry-pick <commit-hash>
Replace `<commit-hash>` with the actual commit identifier you want to pull.
Understanding Git Pull
What is Git Pull?
`git pull` is a command used in Git to synchronize the local repository with its remote counterpart. Essentially, it combines two actions: it fetches changes from a remote repository and merges them into the current branch. This command is vital for maintaining up-to-date code when collaborating with others.
It's important to differentiate `git pull` from other related commands. While `git pull` automatically fetches and merges changes, `git fetch` retrieves updates from a remote repository without merging, leaving you in control of what to do next. On the other hand, `git merge` explicitly combines changes from one branch into another.
When to Use Git Pull
Using `git pull` is crucial in various scenarios, especially in collaborative projects where multiple contributors are making changes. Whenever you want to ensure that your local branch reflects the latest updates made by others, `git pull` is your go-to command. It is also useful for keeping your branches updated before making new commits or starting a new feature.
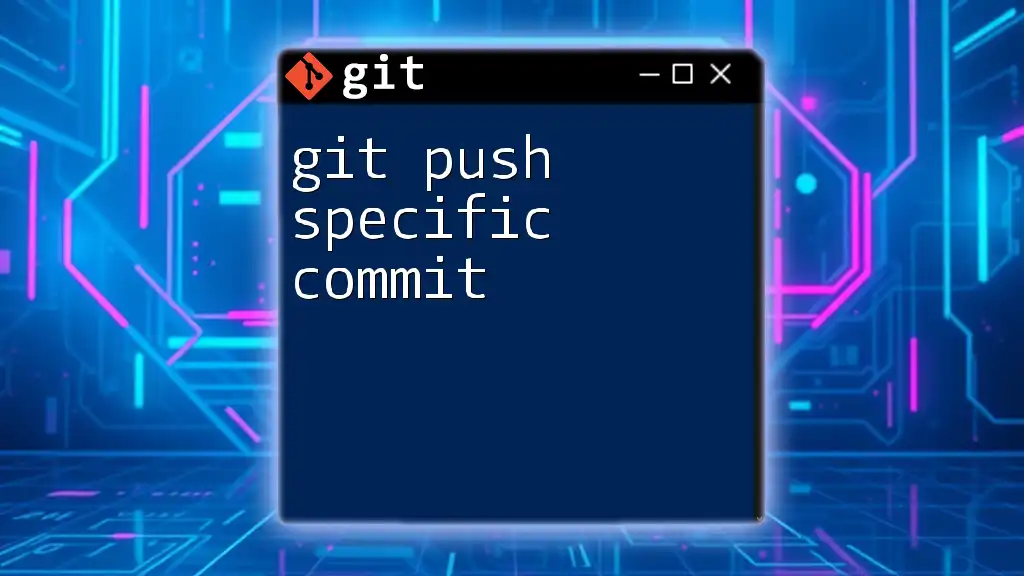
Basics of Commits in Git
Understanding Commits
In Git, a commit is essentially a snapshot of your project's files at a given point in time. Each commit captures the state of the repository and has a unique identifier known as a SHA-1 hash. This hash is critical for referencing commits later.
How to Identify a Specific Commit
To work with specific commits, you need to identify them first. One of the most effective ways to see your project's commit history is by using the `git log` command. This command displays a chronological list of commits, showing their hashes, author information, and commit messages.
To execute this command, you can use:
git log --oneline
This command will give you a concise overview of your commit history, showing each commit’s hash alongside its message.
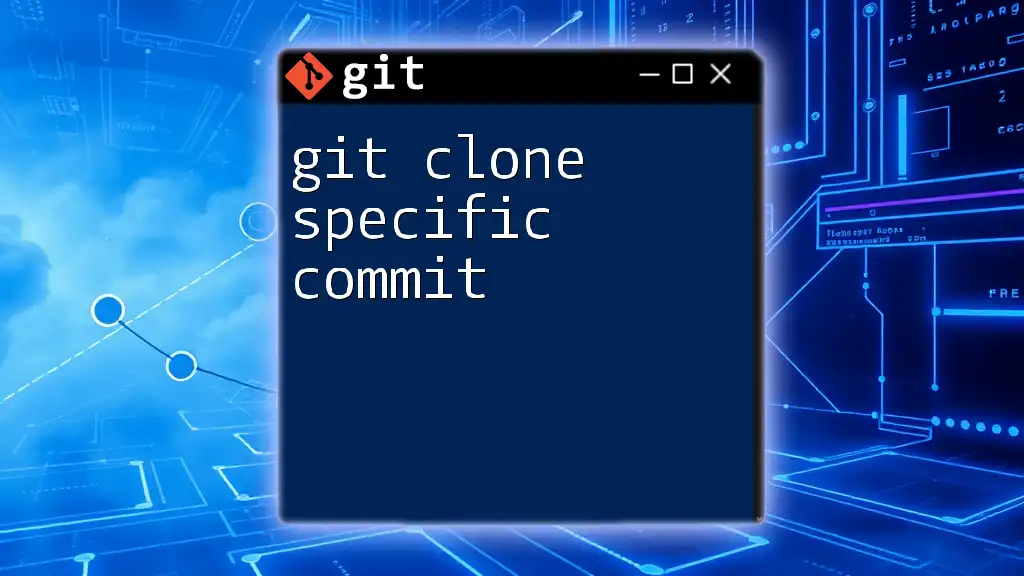
Pulling a Specific Commit
What Does Pulling a Specific Commit Mean?
Pulling a specific commit refers to the process of retrieving a particular change from a remote repository rather than all changes since the last synchronization. This selective approach allows you to focus on specific updates that you find relevant without merging unnecessary changes into your local branch.
How to Pull a Specific Commit
To pull a specific commit, you'll follow a two-step process that includes fetching the commit and then incorporating it into your branch.
The Command Breakdown
-
Fetching a Specific Commit: First, you need to fetch the remote commit using its commit hash. This command will not automatically merge the changes into your branch but will prepare the information you need.
Here’s how to fetch a specific commit:
git fetch origin <commit-hash>
Replace `<commit-hash>` with the actual hash of the commit you wish to pull. This command fetches the commit from the `origin` remote repository.
-
Cherry-Picking the Commit: Next, you'll incorporate the fetched commit into your local branch using the cherry-pick command. Cherry-picking allows you to apply the changes introduced by a specific commit.
Here’s how to perform a cherry-pick:
git cherry-pick <commit-hash>
By executing this command, you effectively make a new commit on your current branch that mirrors the changes made in the specified commit.
Handling Merge Conflicts
When pulling specific commits, you may encounter merge conflicts — situations where changes from the commit conflict with those in your current branch. If this happens, Git will halt the cherry-picking process and prompt you to resolve the conflicts manually.
To resolve merge conflicts, follow these general steps:
-
Identify the Conflicts: Git will mark the conflicting files. You can check which files are affected using:
git status
-
Resolve Conflicts: Open each conflicted file and find the sections marked with conflict identifiers (`<<<<<<<`, `=======`, `>>>>>>>`). Edit the file to resolve the conflict according to the desired changes, and then save the file.
-
Mark as Resolved: Once you've resolved all conflicts, you can mark the changes as resolved:
git add <resolved-file>
-
Complete Cherry-Pick: After resolving the conflicts and staging the changes, you can complete the cherry-pick process by running:
git cherry-pick --continue
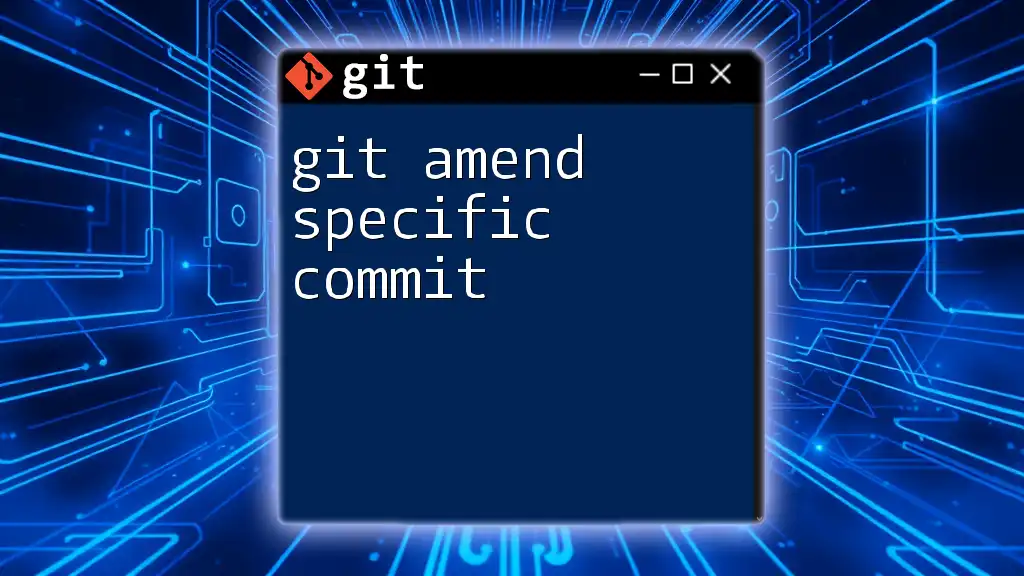
Best Practices for Pulling Specific Commits
When to Consider Pulling a Specific Commit
Pulling a specific commit can be advantageous but only in particular situations. It’s beneficial when you need to apply a bug fix or a feature that was developed independently of the main branch's ongoing changes. However, you should be cautious, as pulling specific commits can lead to an inconsistent project state if not managed correctly.
Avoiding Common Pitfalls
When executing this process, be vigilant about potential errors such as pulling commits that depend on other changes not being merged. This can lead to missing context or associated code. Always ensure that the specific commit you plan to cherry-pick is self-contained and functional.
Keeping Your Branch Clean
After performing operations like cherry-picking, it's essential to keep your branch clean. Consider whether it makes sense to rebase your branch instead of merging if your workflow allows for it. Rebasing can help you maintain a linear project history by applying your changes atop other changes, but it may complicate history if used incorrectly.
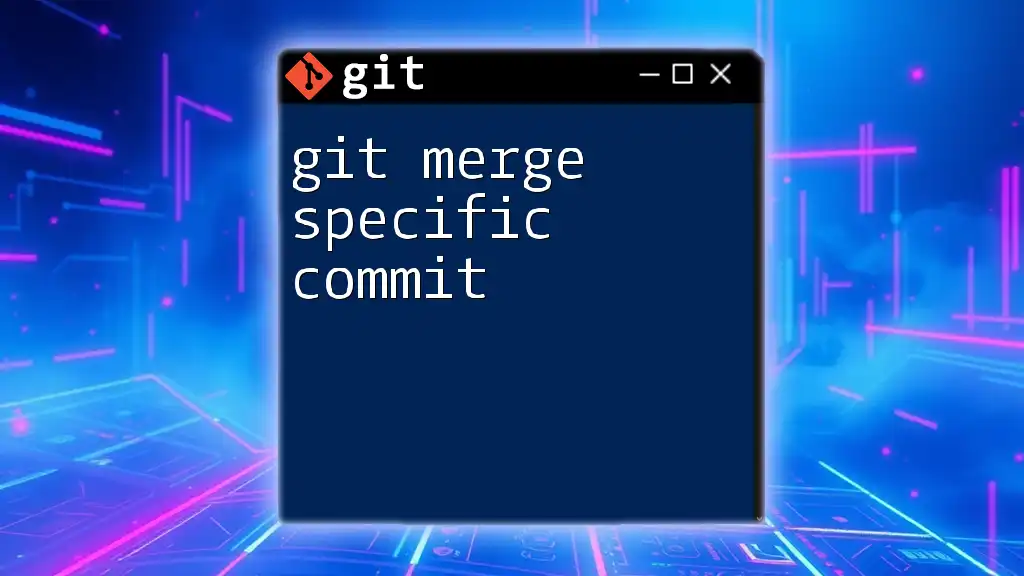
Conclusion
Summary of Key Points
To sum up, pulling a specific commit is a powerful technique that enables developers to apply targeted changes from a shared repository. By understanding how to fetch and cherry-pick commits, you can manage your code effectively and incorporate only the necessary updates into your work.
Further Learning Resources
For those seeking to deepen their comprehension of Git, consider exploring additional resources such as the official Git documentation. Understanding the nuances of version control will significantly enhance your collaborative development experiences.
Call to Action
Now that you have a grasp on how to pull a specific commit, it’s time to put this knowledge into practice. Experiment with these commands in a safe environment and explore the intricacies of Git to become a more proficient developer in your collaborative endeavors!