To remove a specific commit from your Git history, you can use the `git reset` command followed by the commit hash, like this:
git reset --hard <commit-hash>
Replace `<commit-hash>` with the hash of the commit you want to remove.
Understanding Commits in Git
What is a Commit?
A commit in Git is a snapshot of your project at a specific point in time. Each commit contains a unique identifier, a commit message describing the changes, and metadata about the author and date of the commit. Commits are crucial in version control because they allow you to track changes, revert to previous states, and collaborate with others while maintaining a detailed history of modifications.
Example: A standard commit message format is:
git commit -m "Fix issue with user authentication"
This logs the changes related to user authentication with a short description.
Why Remove a Commit?
There are various scenarios where you might need to remove a specific commit:
- Accidentally committed sensitive information: If you mistakenly added passwords, API keys, or other sensitive data.
- Correcting mistakes in the commit history: Sometimes, you may find that a commit contains inaccurate information or was made in error.
- Cleaning up a messy commit history: A well-structured commit history is valuable for future reference and can enhance collaboration.
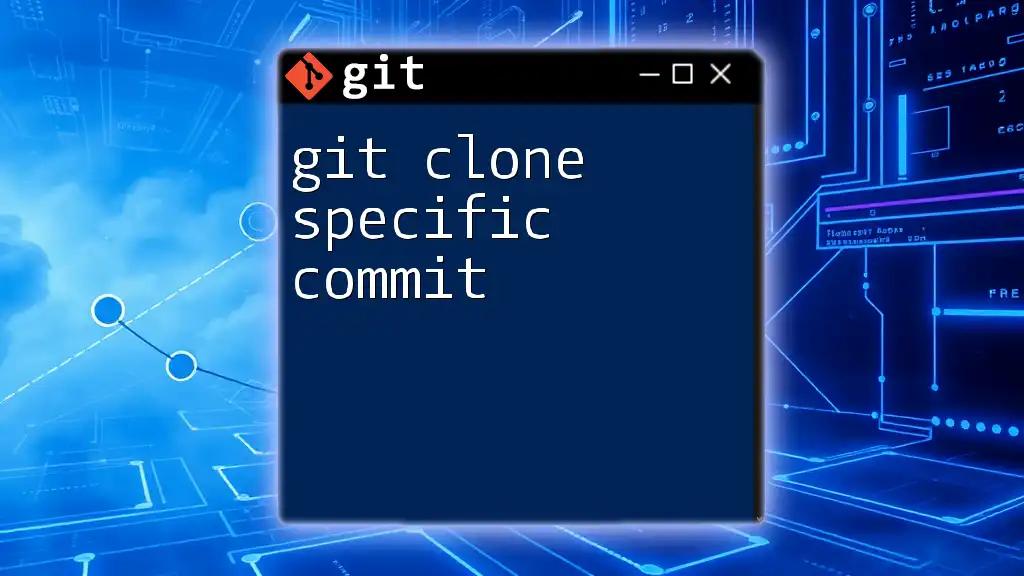
Methods to Remove a Specific Commit
Using `git revert`
What is `git revert`? `git revert` is a command that creates a new commit that undoes the changes made by a specific commit without modifying the project's history. This is often safer than removing a commit because it doesn’t change the existing history, making it ideal for public branches.
How to use `git revert` To use `git revert`, you'll need the commit hash of the commit you want to remove. The syntax is:
git revert <commit_hash>
Example:
-
First, find the commit hash using `git log`:
git log
This will show a list of commits. Locate the commit you want to revert.
-
Then, execute:
git revert abcd1234
After running this command, Git will create a new commit that reverses the changes made by the commit with hash `abcd1234`.
Keep in mind that after reverting, you may need to resolve conflicts if any changes conflict with the current codebase.
Using `git reset`
What is `git reset`? `git reset` is a powerful command used to delete one or more commits from your history. It alters your commit history and can be used in three modes: soft, mixed, and hard.
When to use `git reset`?
- Use `--soft` if you want to keep the changes in your working directory and staging area.
- Use `--mixed` (the default) to keep the changes in your working directory but not staged.
- Use `--hard` if you want to discard all changes permanently and reset your working directory.
How to use `git reset` to remove a commit Here’s how to execute a reset:
git reset --soft <commit_hash>
or
git reset --hard <commit_hash>
Example: To remove the latest commit but keep its changes:
git reset --soft HEAD~1
This command resets the current branch to the previous commit (one commit behind), keeping your changes staged for the next commit.
If you want to discard everything, use:
git reset --hard HEAD~1
Warning: Using `--hard` will permanently delete all changes made in the commit and cannot be undone.
Using `git rebase`
What is `git rebase`? `git rebase` allows you to rewrite commit history by changing, deleting, or altering commits. This is particularly useful for cleanly combining and organizing commits.
How to Interactively Rebase To remove a specific commit, you can use interactive rebase:
git rebase -i <commit_hash>^
This command opens an editor that lists recent commits, starting just before the specified commit.
Example:
-
Run:
git rebase -i abcd1234^
This will open an editor showing a list of commits leading up to `abcd1234`.
-
In the editor, find the commit you wish to remove and change `pick` to `drop`:
drop abcd1234 Remove sensitive data
-
Save and exit the editor. Git will reapply all committed changes except for the dropped commit.
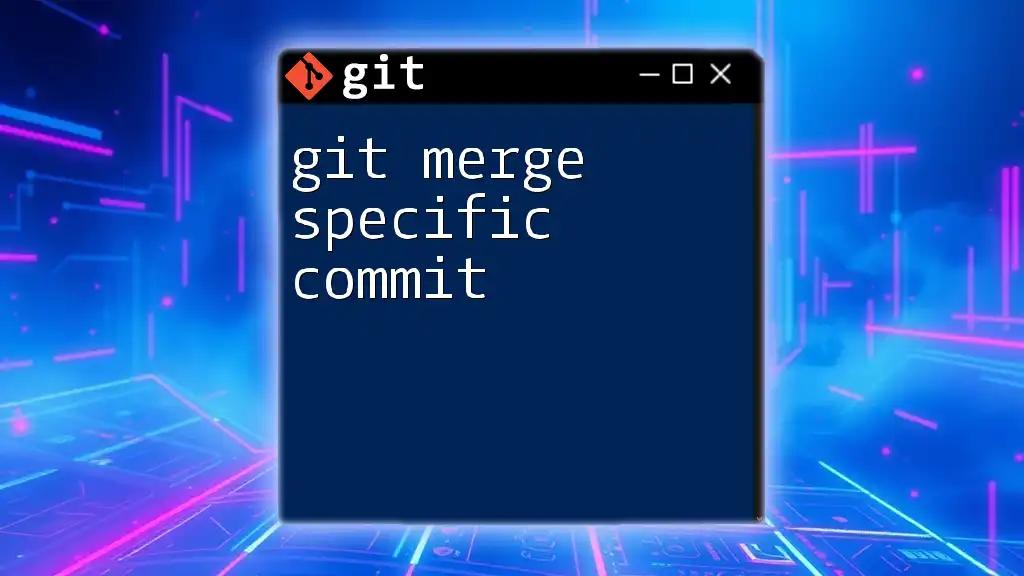
Important Considerations
Work with Caution
Removing commits can be risky, especially in shared repositories. Changing history can disrupt collaborators who have based their work on your commits. Always back up your repository before performing operations that alter commit history.
Alternatives to Removing a Commit
Sometimes it’s better to leave the commit in place, especially if it serves a historical purpose. Instead of removing a commit, consider using branches for experimental changes or simply document the error in the commit history rather than erasing it.
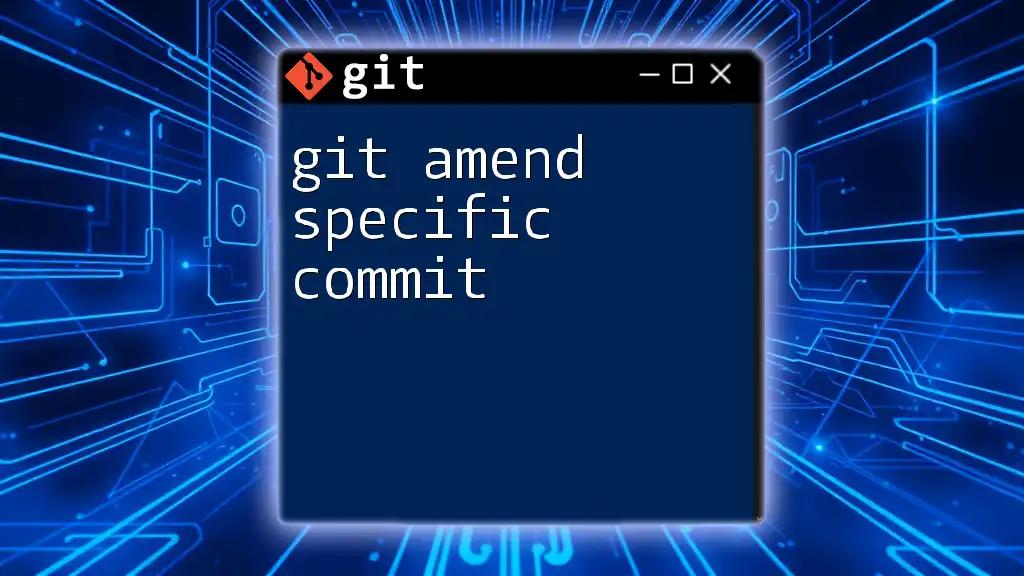
Common Errors and Troubleshooting
Common Mistakes When Removing Commits
A common mistake is misunderstanding the implications of using `git reset` compared to `git revert`. While `git reset` alters the history, `git revert` offers a safer approach without breaking existing history.
How to Resolve Issues
If you experience problems while manipulating the commit history, you can recover lost commits using:
git reflog
This command provides a history of your actions in the repository, allowing you to find lost commits and restore them.
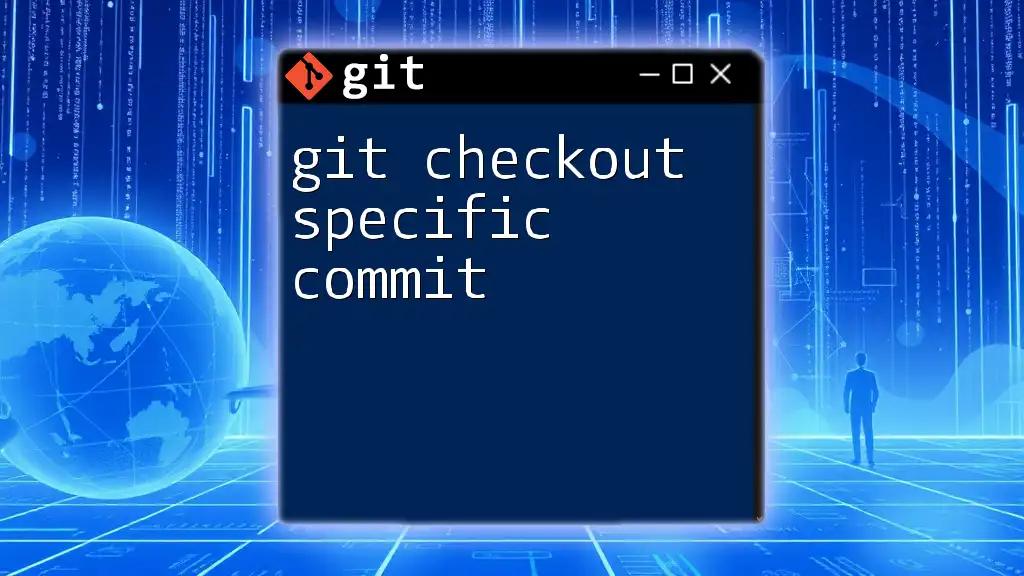
Conclusion
Managing commits effectively is crucial when working with Git. Whether you use `git revert`, `git reset`, or `git rebase`, understanding how to remove a specific commit allows you to maintain a clean and organized project history. Always approach these commands with care, especially in collaborative environments, to ensure the integrity and clarity of your project’s development timeline.
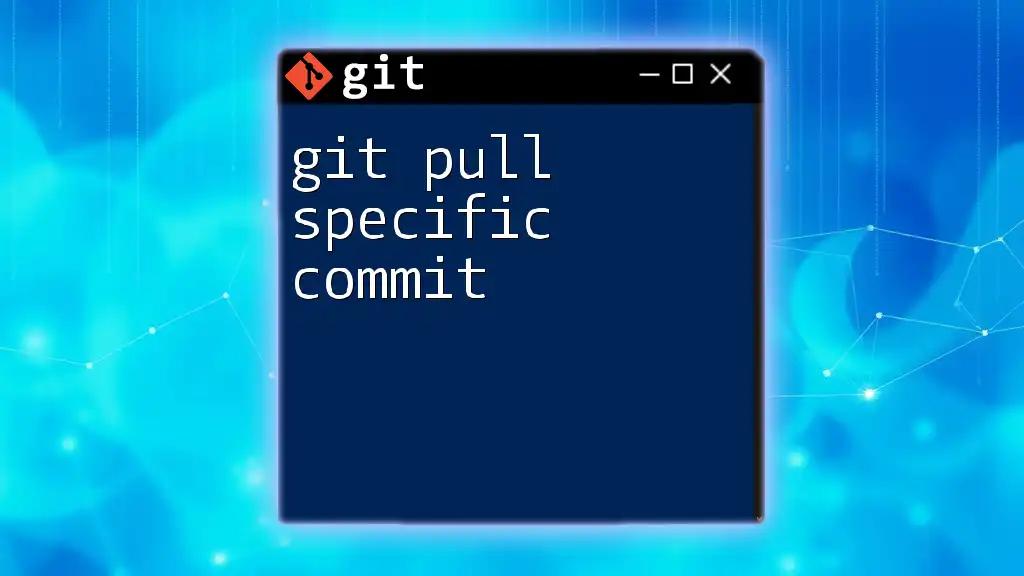
Additional Resources
For further learning, explore the official Git documentation, tools for GUI-based Git management, and various online Git training courses to deepen your understanding and expertise in this essential version control system.