To remove the most recent commit in Git while keeping the changes in your working directory, use the command `git reset --soft HEAD~1`.
git reset --soft HEAD~1
Understanding Git Commits
What is a Commit?
A commit in Git is a snapshot of your project's files at a particular point in time. Each commit serves as a record, encompassing not just the changes made to the files, but also the author, timestamp, and a unique identifier known as the commit hash. This structure allows developers to track changes over time effectively.
For example, creating a commit can be done using the following command:
git commit -m "Your commit message here"
Why You Might Need to Remove a Commit
There are several reasons why you may need to remove one commit from your Git history. These can include fixing mistakes in the code, removing sensitive information, or adjusting the project structure as requirements evolve. However, it’s essential to consider that removing a commit can lead to loss of history, especially if the commit has already been shared with others. Thus, understanding the implications of modifying commit history is paramount.
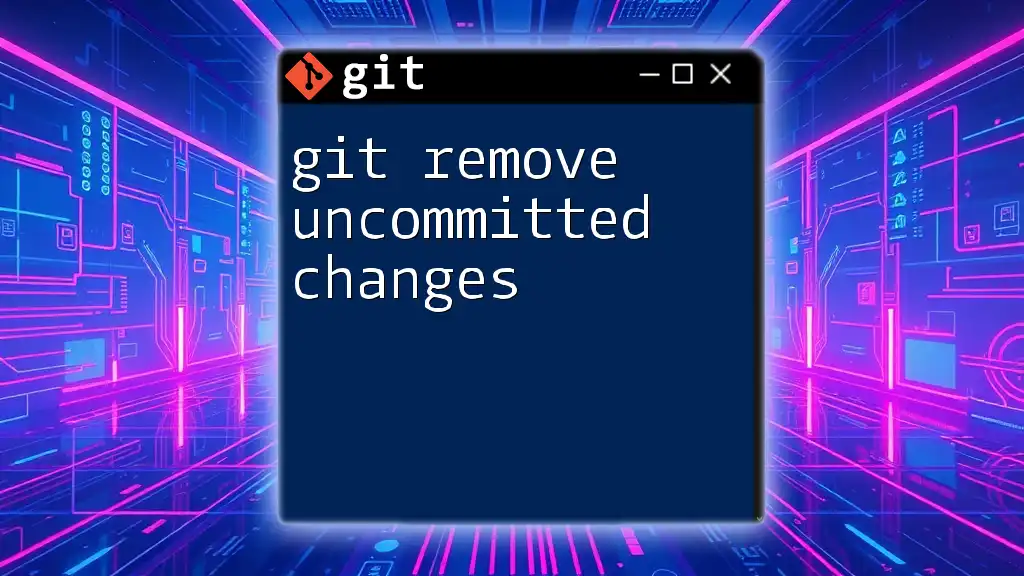
Approaches to Remove a Commit
Choosing the Right Method
Choosing the correct method to remove one commit heavily depends on your context—specifically, whether the commit has been pushed to a shared repository or not. Some methods can rewrite history, while others can add new records to negate previous changes safely.
Using `git reset`
Overview of `git reset`
The `git reset` command is a powerful tool that allows you to move your current HEAD and, optionally, the index and working directory to a specified state. There are three main modes to consider: soft, mixed, and hard.
- Soft (`--soft`): Keeps all changes in the staging area.
- Mixed (default): Keeps changes in your working directory but removes them from staging.
- Hard (`--hard`): Discards all changes completely.
Removing the Most Recent Commit
If you need to remove one commit, particularly the most recent one, you can use:
git reset --hard HEAD~1
This command will remove the latest commit and all changes associated with it from both your history and the working directory. Be cautious with `--hard`, as it will eliminate all uncommitted changes without warning.
Using `git revert`
Overview of `git revert`
Unlike `git reset`, the `git revert` command creates a new commit that undoes the changes made by a previous commit. This method is often considered safer because it preserves project history, making it suitable for shared repositories.
To remove one commit using revert, you can run:
git revert HEAD
This command will generate a new commit that effectively negates the changes of the most recent commit. This approach maintains a clear project history while allowing the removal of undesired changes.
Understanding the Revert Process
The `git revert` command is beneficial when collaborating with a team, as it avoids complications associated with rewriting history. When you revert a commit, the original commit remains in the history, maintaining context and document integrity.
Alternative: Interactive Rebase
What is Interactive Rebase?
An interactive rebase is a technique that allows you to edit commit history in a more granular way. It’s particularly useful for cleaning up your commit history before pushing changes to a shared repository.
Steps to Perform an Interactive Rebase
To remove one commit using interactive rebase, initiate the process by using:
git rebase -i HEAD~3
This command opens the last three commits in your default text editor. You can see the commits listed alongside the command options.
Inside the editor, you can remove a commit by deleting the line or replacing pick with drop for the specific commit you want to omit. Save and close the editor to execute the rebase.
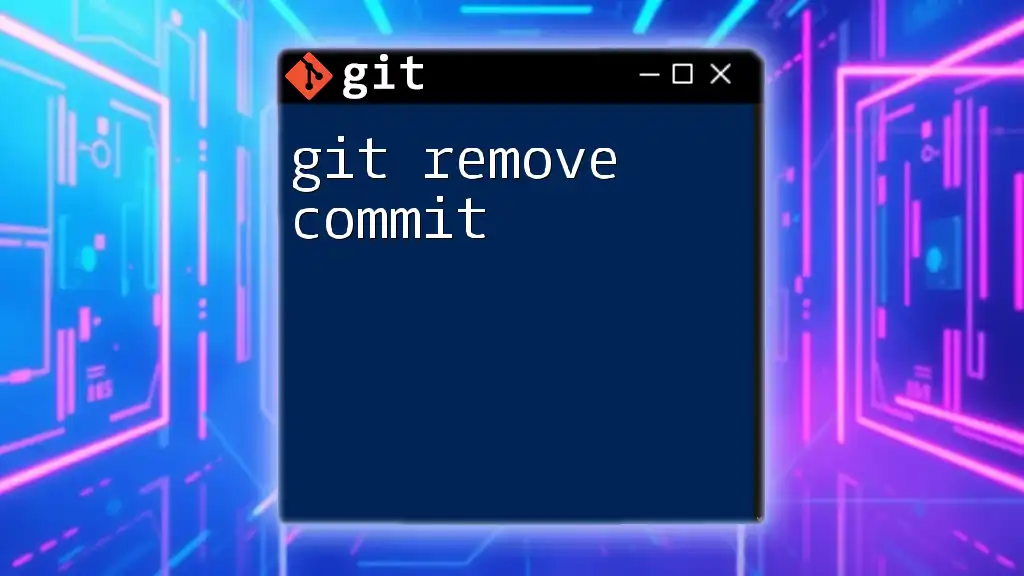
Potential Challenges
Dealing with Conflicts
It’s not uncommon to encounter merge conflicts after removing a commit. If this happens, Git will notify you, and you'll need to resolve these conflicts before continuing. You can manually edit the conflicting files, then stage the resolved files using:
git add <filename>
Once all conflicts are resolved, you can complete the rebase process with:
git rebase --continue
Impact on Collaborators
When you remove one commit that has been pushed to a shared repository, it can create significant challenges for your collaborators. They may encounter issues when trying to pull or push changes. It's advisable to communicate with your team before making such changes and, if possible, revert instead of reset to avoid disrupting the develop workflow.
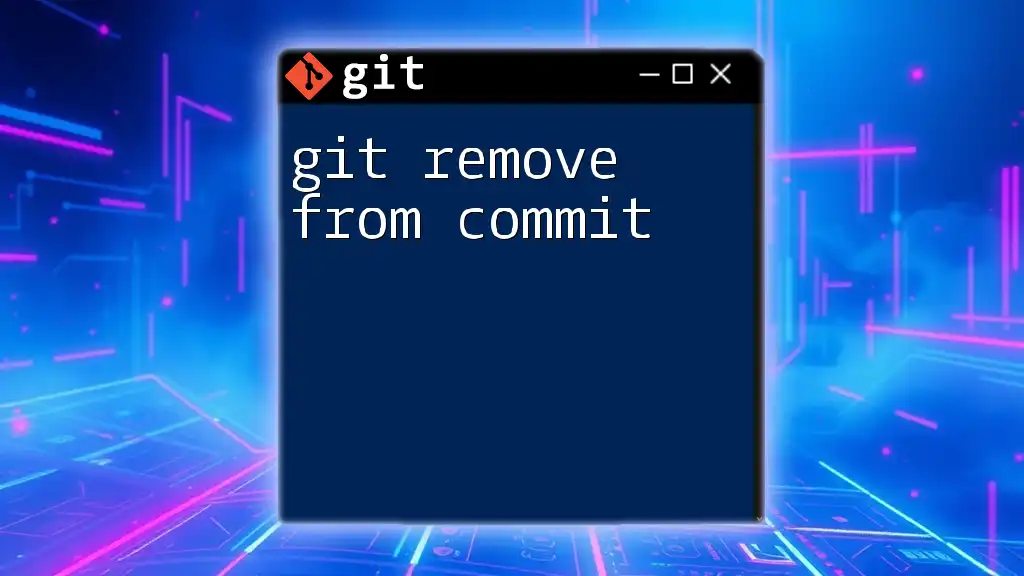
Conclusion
In summary, understanding how to remove one commit in Git is a fundamental skill that can enhance your version control practices. Whether you opt for `git reset`, `git revert`, or interactive rebase, each method has its merits and associated risks. Always proceed with caution, especially when modifying history in a shared environment. Practicing these commands in a safe setting will increase your confidence and proficiency when managing your Git repositories.
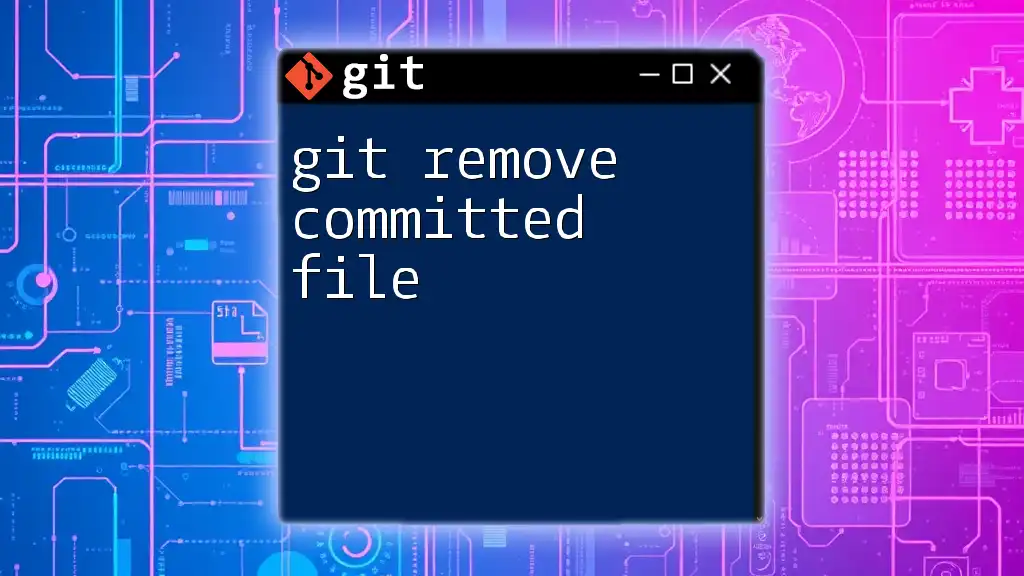
Additional Resources
For further reading, please refer to the official Git documentation [here](https://git-scm.com/doc). Exploring Git GUI tools can also be beneficial for beginners, as they provide visual representations of your Git history. Lastly, consider putting your skills to the test with hands-on exercises that reinforce what you've learned about manipulating commits in Git.