The `git restore commit` command allows you to revert the changes made by a specific commit, effectively undoing its impact on your working directory or staging area.
Here's how you can use it:
git restore --source <commit> -- <path>
Replace `<commit>` with the actual commit hash and `<path>` with the file or directory you want to restore.
Understanding `git restore`
What is `git restore`?
The `git restore` command was introduced to enhance the management of your files in the working directory and the staging area. Its main purpose is to restore files or changes from previous commits without altering the commit history. This is particularly useful when you want to discard changes made after a specific commit or revert files affected by errors during development.
Unlike older commands like `git checkout`, which can perform multiple functions (like switching branches), `git restore` focuses specifically on restoring file states. This clarity in functionality helps to streamline operations, especially for new Git users.
Use Cases for `git restore`
Restoring a commit can become vital in several scenarios:
- Mistaken Edits: When you realize that recent changes introduced bugs or errors, restoring a previous commit can save time.
- Collaborative Projects: In a team environment, you might overwrite others' work. Restoring previous commits helps correct this without complicating shared branch histories.
- Version Management: As you develop features, you may want to isolate changes for testing or troubleshooting. Restoring individual files or states allows that flexibility.
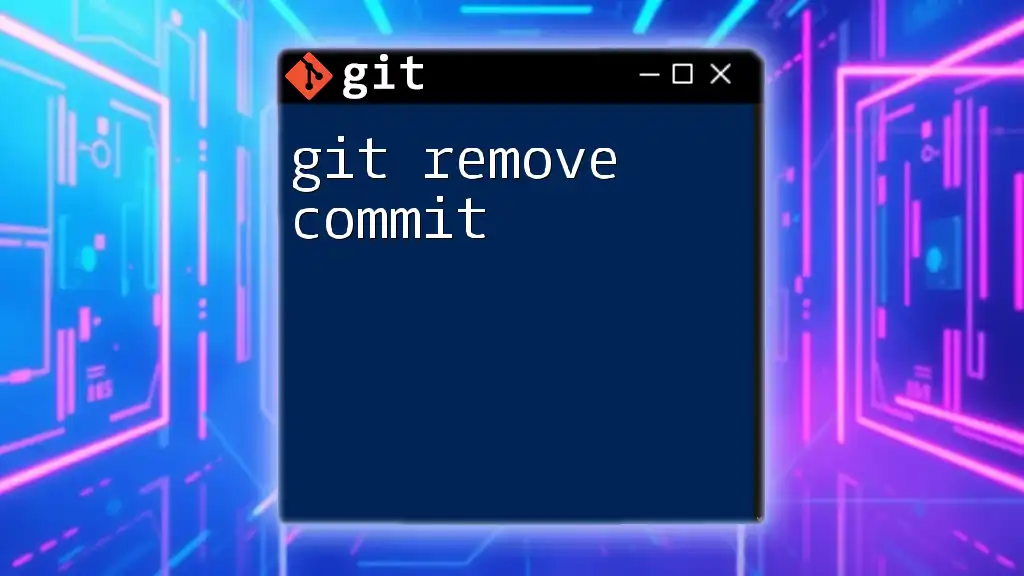
How to Use `git restore commit`
Basic Syntax of `git restore`
The command has a well-defined syntax:
git restore <options> <pathspec>
Here, `<options>` can modify the behavior of the command, while `<pathspec>` refers to the files or directories you intend to restore.
Restoring the Commit
Restoring Changes from a Single Commit
To restore changes from a specific commit, you need the unique commit hash (an alphanumeric identifier for that commit). This is achieved using the following command structure:
git restore --source <commit_hash> -- <file_name>
- `--source <commit_hash>` specifies the commit you're restoring from.
- `<file_name>` refers to the specific file you want to revert to its previous state.
For instance, if your commit hash is `abc1234` and you want to restore a file called `example.txt`, your command would look like this:
git restore --source abc1234 -- example.txt
Restoring Multiple Files
If you need to restore multiple files from the same commit, you can do so by listing them:
git restore --source <commit_hash> -- <file1> <file2> <file3>
This allows you to efficiently handle situations where several files are affected by unwanted changes.
Advanced Options for `git restore`
Using `--staged`
The `--staged` option allows you to restore files directly to the staging area. If you want to revert a file's staged version back to its state in the last commit, you can use:
git restore --staged <file_name>
This command effectively unstages the latest changes made to the file while keeping changes in your working directory, enabling you to individually manage what's ready to be committed.
Using `--worktree`
If you wish to revert changes directly in your working directory without touching the staging area, you can use:
git restore --worktree -- <file_name>
This is especially beneficial if you want to keep your staged changes intact while eliminating errors in your current working copy.
Combining Options
Git allows you to use multiple options in a single command for more complex scenarios. For example:
git restore --source <commit_hash> --staged --worktree -- <file_name>
This command would restore the specified file both in the staging area and working directory from the given commit hash, giving you a robust method to manage your file changes seamlessly.
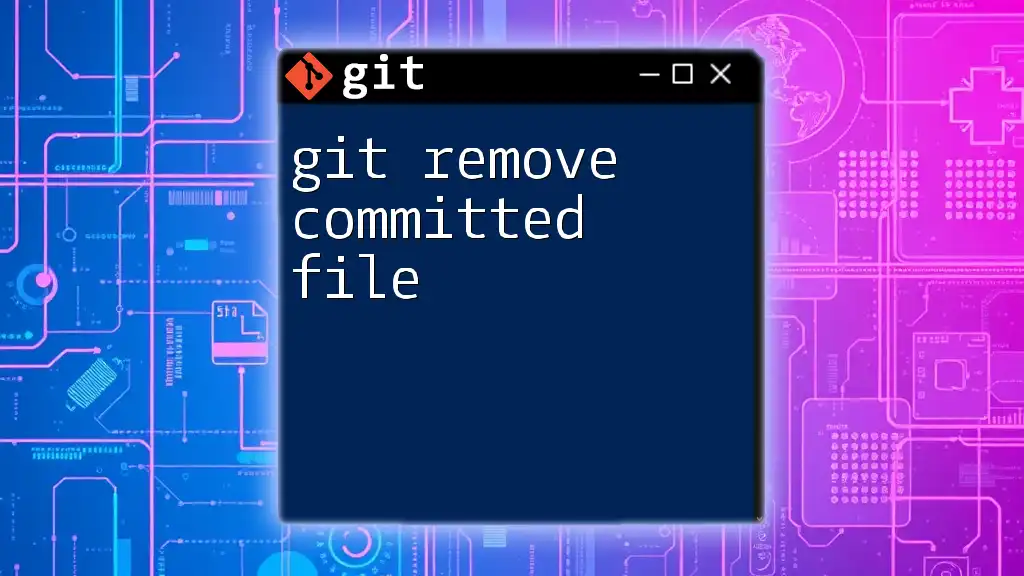
Comparing `git restore` to Other Options
`git checkout`
While `git restore` focuses specifically on restoring files or commits, `git checkout` can accomplish a similar result but also serves various other functions, such as switching branches. Because `git checkout` merges functionalities, it can be less intuitive for beginners.
For example:
git checkout <commit_hash> -- <file_name>
This command also restores a file to a specific commit state, but it can lead to confusion if you’re purely looking to manage file states.
`git revert`
Unlike `git restore`, which rewinds files to a past state without creating a new commit, `git revert` creates a new commit that undoes the changes of a specified commit. This means that the history will reflect the reversal clearly, which is often best practice in collaborative environments as it maintains a complete log of changes.
To revert a commit, you would use:
git revert <commit_hash>
This will create a new commit reversing the changes made by `<commit_hash>`, allowing for clear traceability in the project’s history.
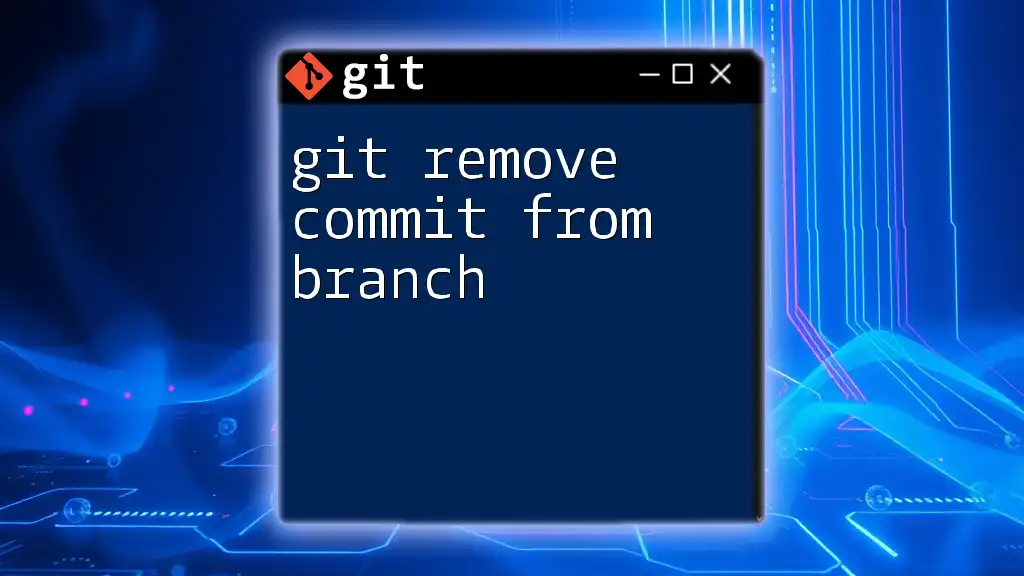
Best Practices for Using `git restore`
Version Control Best Practices
Maintaining a clean commit history is crucial for effective collaboration. Use `git restore` liberally, but always verify what changes will be affected before you execute the command. An organized history allows team members to track changes and minimize conflicts.
Understanding Your Git History
Getting familiar with your project's history will help immensely when choosing which commits to restore. The command:
git log --oneline
will give you a quick overview of your commit history. Each commit is represented with a hash, viewable as a condensed one-liner, making it easy to pinpoint the exact commit you wish to restore from.
Avoiding Common Pitfalls
While `git restore` is powerful, be wary of restoring the wrong files or using incorrect commit hashes. A practice worth adopting is to always create a backup branch before experimenting with restoration commands. A simple command like `git branch backup` can safeguard your current state, ensuring you can revert if necessary.
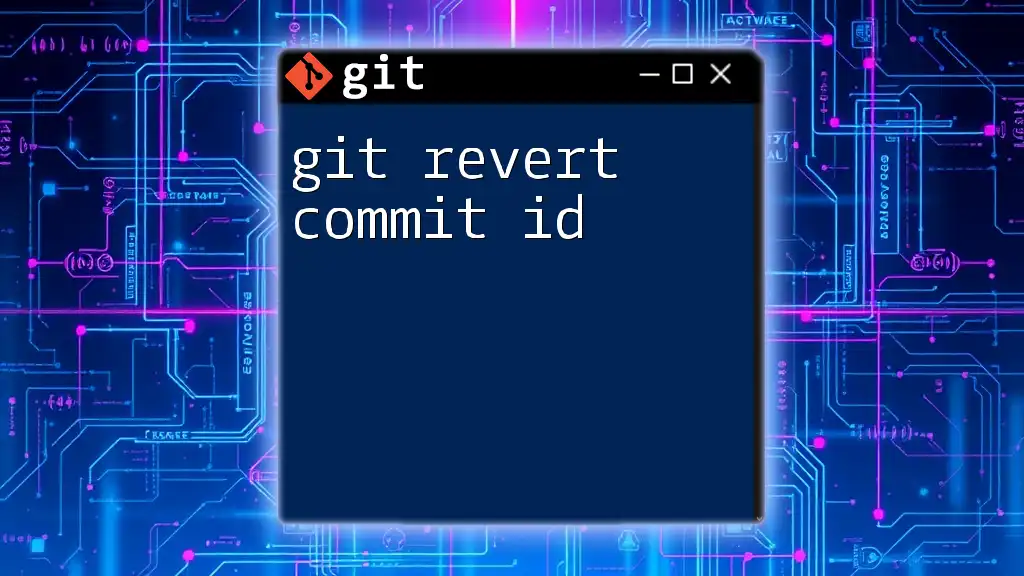
Conclusion
In summary, the `git restore commit` command offers an efficient way to undo mistakes and manage your workflow. By mastering its use, you can navigate through project complexities with greater ease and confidence. Practicing these commands in a safe environment will solidify your understanding and make the command second nature in your development work.
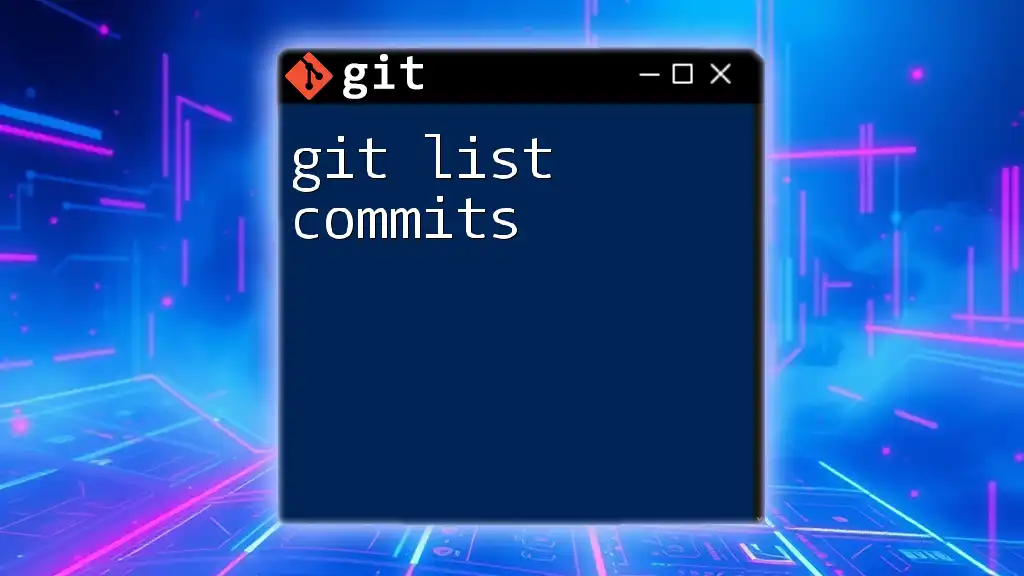
Additional Resources
For further exploration of Git commands, consider visiting the [official Git documentation](https://git-scm.com/doc) and investing time in Git tutorials to strengthen your skills. Effective version control strategies can be found through various online courses and books dedicated to Git best practices, ensuring you not only use commands correctly but also understand their place in successful software development.