The `git show commit` command displays detailed information about a specific commit, including the commit message, author, date, and the changes made to the files.
git show <commit-hash>
Understanding `git show`
What is `git show`?
The `git show` command is a powerful tool in Git that allows users to inspect various types of Git objects. Most commonly, it is used to view the details of commits, but it can also show tags and trees. Understanding this command is crucial for effective version control, as it provides insight into the changes made over time.
General Syntax of `git show`
The general syntax for the `git show` command is as follows:
git show [options] <commit>
In this command, `<commit>` can refer to a specific commit hash, a tag, or a branch name. Various options can be combined with the command to specify how much detail to display or how to format it.
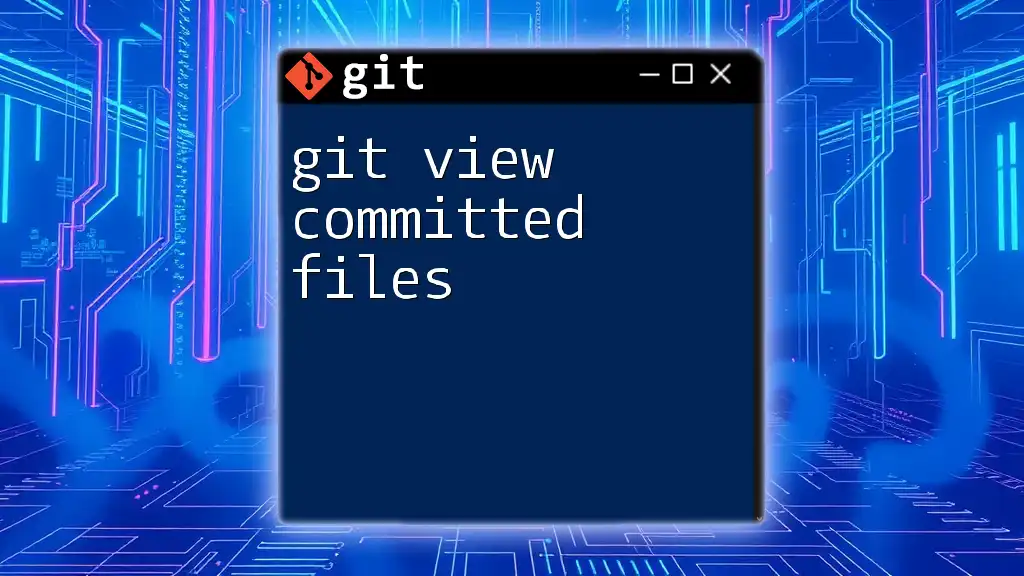
Using `git show commit` in Practice
What Does a Commit Represent?
In Git, a commit represents a snapshot of the project at a certain point in time. Each commit consists of multiple components:
- Commit Hash: A unique SHA-1 identifier for the commit.
- Author: The individual who made the changes.
- Date: When the commit was made.
- Message: A brief description of what changes the commit includes.
How to Use `git show commit`
To see the details of a specific commit, you would use its hash with the `git show` command. Here’s an example:
git show a1b2c3d4
When executed, this command displays:
- The commit hash.
- The author’s name and email.
- The date of the commit.
- The commit message.
- The diff showing the changes made in that commit.
Each part of the output provides crucial context about the changes, making it easier to track project history.
Example Scenarios
Viewing the Latest Commit
To view the most recent commit on your current branch, use the command:
git show HEAD
`HEAD` represents the latest state of your current working directory. This command will show all the details of the last commit made.
Viewing a Specific Commit
If you want to look at a commit that is not necessarily the latest, you can reference its unique hash:
git show <commit-hash>
This allows you to gather details about any point in your project history, essential for debugging or reviewing past changes.
Understanding the Output
Breakdown of Git Show Output
When you run `git show`, the output begins with the commit header which usually includes:
- The commit hash.
- Author details: Format includes name and email.
- Date: Information about when the commit was created.
- Commit message: A short and meaningful description.
This header is followed by the diff section that details the changes made in this commit.
Diff Section
The diff section gives you a visual representation of the changes compared to the previous commit. Here’s how to interpret it:
- Lines prefixed with + indicate additions.
- Lines prefixed with - indicate deletions.
This visual comparison makes it easier to review changes before merging or finalizing updates.
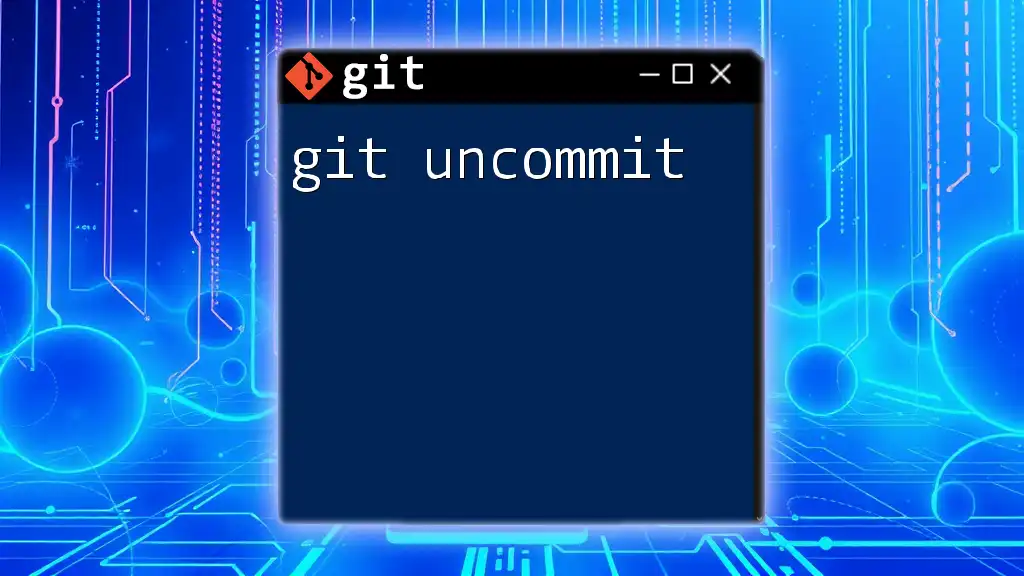
Advanced Usage of `git show`
Customizing Output Format
Using Pretty Print
You may wish to format the output for better readability. The `--pretty` option allows you to customize how you see commit information. Here’s an example:
git show --pretty=format:"%h - %an, %ar : %s"
In this command:
- `%h` shows the abbreviated hash.
- `%an` displays the author name.
- `%ar` shows how long ago the commit was made.
- `%s` reveals the commit message.
This option is valuable for getting a concise view without having to sift through extensive detail.
Combining with Other Options
For additional insights, you can combine `git show` with other options. For instance, if you want to view a summary of the changes along with the actual changes, you could run:
git show --stat <commit-hash>
The `--stat` option provides a summary of which files changed, and how many lines were added or deleted.
Using `git show` with Tags and Branches
You can also use the `git show` command to inspect commits associated with tags and branches. To view a specific tag, you can run:
git show v1.0.0
This command will fetch the commit details related to the specified version tag. Similarly, to see the latest commit made on a particular branch, use:
git show branch-name
This flexibility allows you to explore your project's history thoroughly.
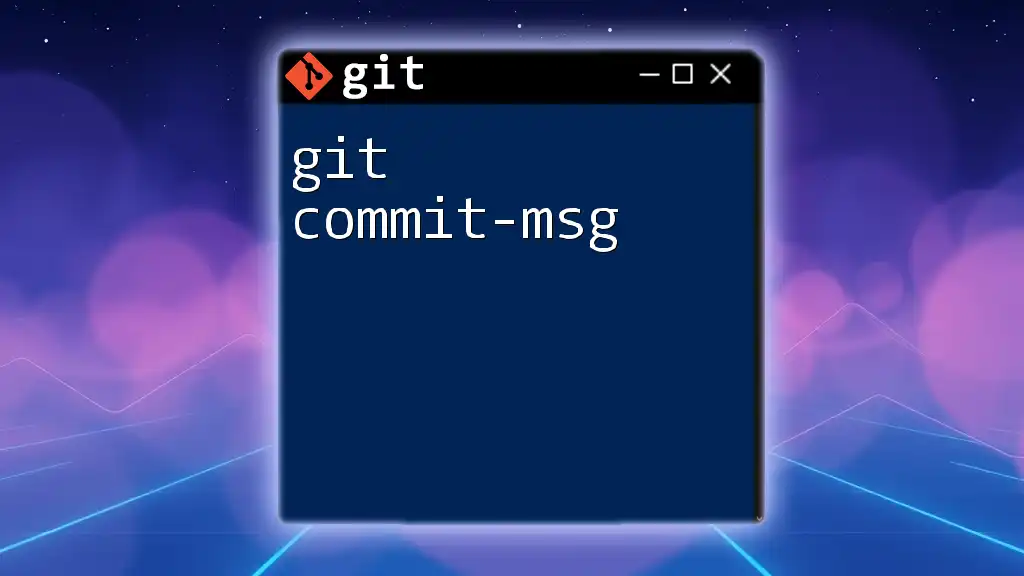
Troubleshooting Common Issues
"Error: fatal: bad revision" message
You may encounter the error:
fatal: bad revision 'your_commit_hash'
This often occurs when the specified commit cannot be found in the repository. Common causes include:
- Typos in the commit hash.
- The commit being located in a different branch.
Double-check the hash and the branch you are currently on when troubleshooting this issue.
Output Too Long to Read
Sometimes the output from `git show` can be extensive and hard to read. If this situation arises, you can use `less` to paginate through the output:
git show <commit-hash> | less
Alternatively, redirecting the output into a text file can help manage particularly verbose outputs.
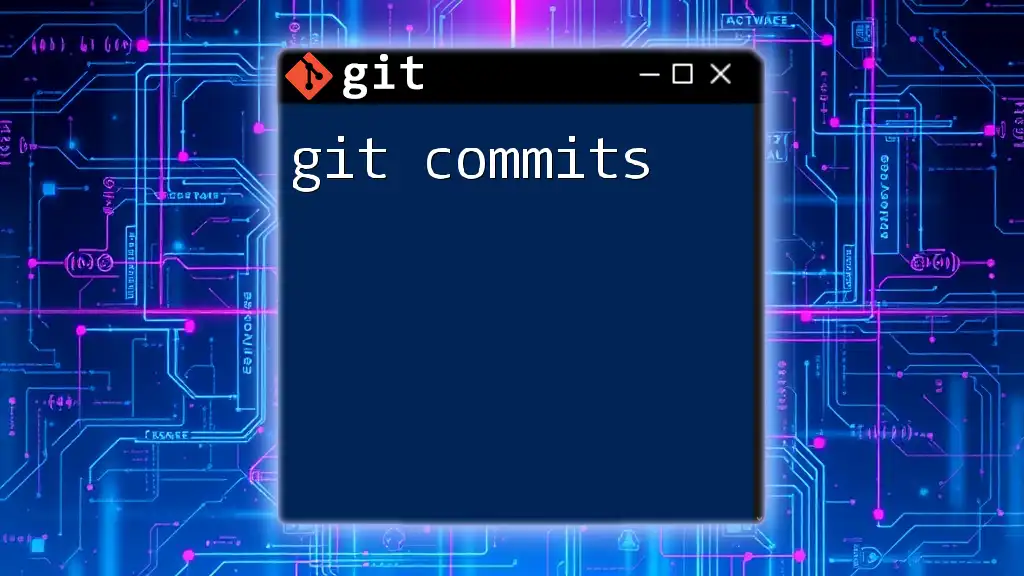
Conclusion
The `git show commit` command serves as a key tool for navigating the history of a Git project. It allows developers to inspect and understand changes over time, facilitating better version control practices. By experimenting with different options and formats, you can gain deeper insights into your project’s evolution.
For ongoing updates on mastering Git commands, consider subscribing to our resources for more tips and practical guides.
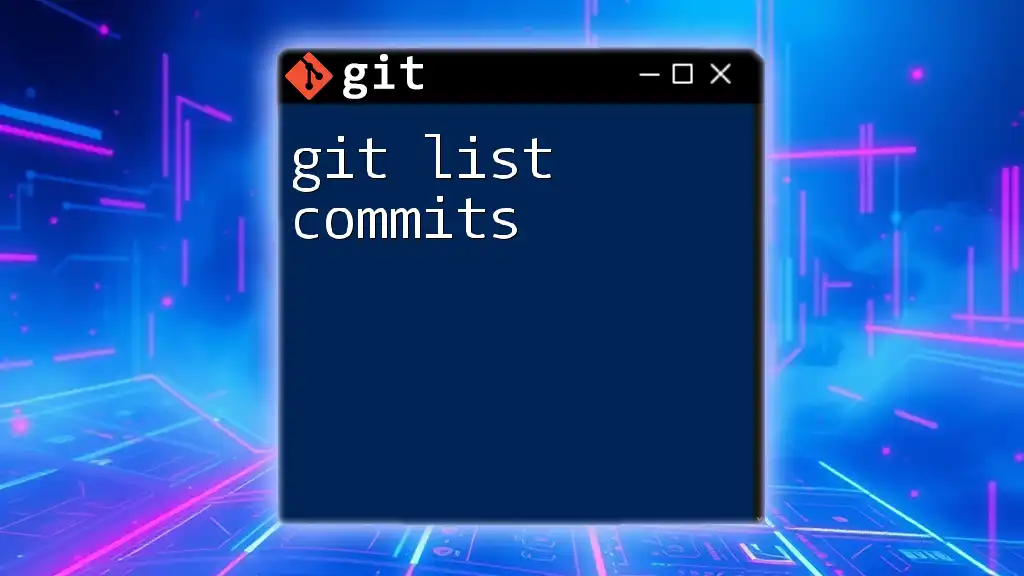
Additional Resources
For further reading, always refer to the [official Git documentation on `git show`](https://git-scm.com/docs/git-show). Additionally, explore recommended books or tutorials to expand your understanding of effective Git usage.