The command `git log` displays a list of all commits in the repository along with their hashes, authors, dates, and commit messages.
Here's how to use it:
git log
What are Git Commits?
In Git, a commit serves as a snapshot of your project at a specific point in time. Each time you make a commit, you not only save your changes but also create a unique reference for that set of changes, which can be helpful for tracking the history and evolution of your project.
Commits are significant in maintaining a clear and efficient collaboration workflow, allowing multiple contributors to work on the same project without overwriting each other's efforts. Being able to list commits is crucial for auditing changes, reviewing contributions, and debugging issues that may arise as a project evolves.
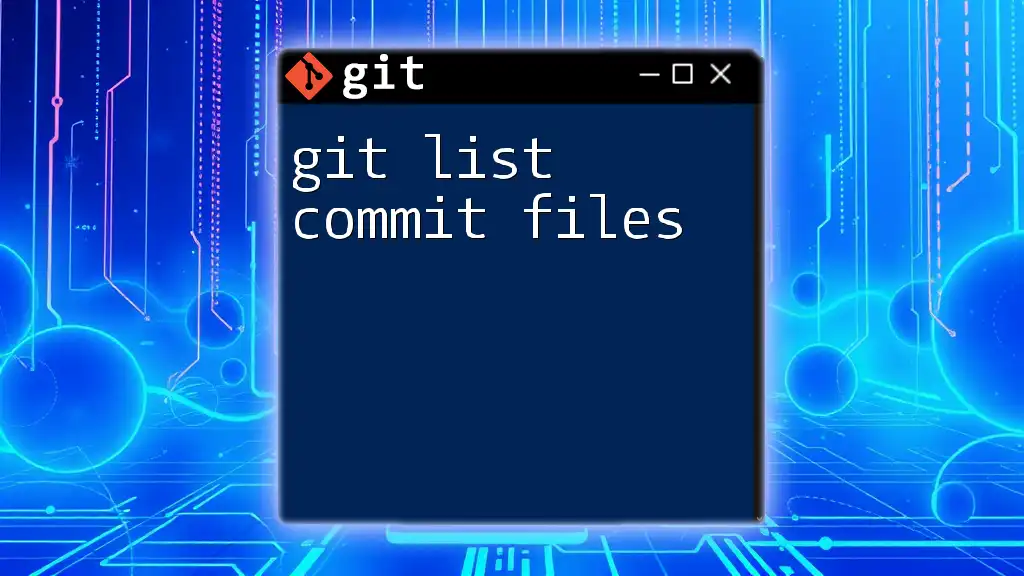
Why List Commits?
Listing commits is essential for several scenarios:
- Auditing: By reviewing the commit history, you can ensure that all changes made to the project are documented and purposeful.
- Collaboration: Knowing who made changes and why can facilitate better communication within a team.
- Debugging: Tracking down when a particular change was made that introduced a problem can significantly speed up the troubleshooting process.
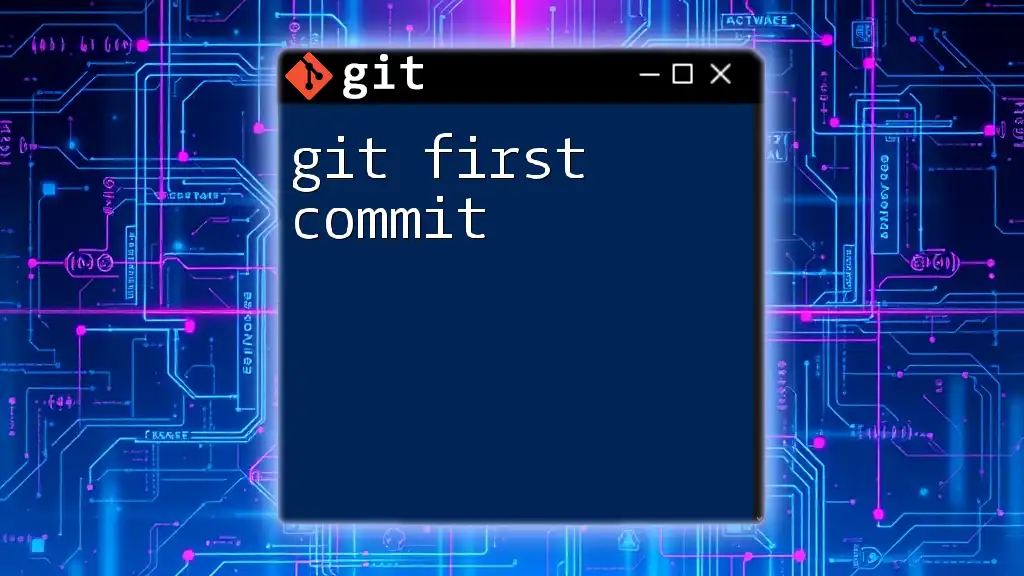
Understanding Git Log Command
What is the Git Log Command?
The `git log` command is your primary tool for listing commits in a Git repository. It provides a detailed view of the commit history, allowing you to see each commit's metadata, which includes the commit hash, author name, date, and commit message.
Basic Usage of `git log`
To view the commit history, simply execute:
git log
The output will be a list of all commits made in the current branch, starting from the most recent. Each entry includes vital details:
- Commit Hash: A unique identifier for the commit.
- Author: Who made the changes.
- Date: When the changes were made.
- Commit Message: A brief description of what changes were made.
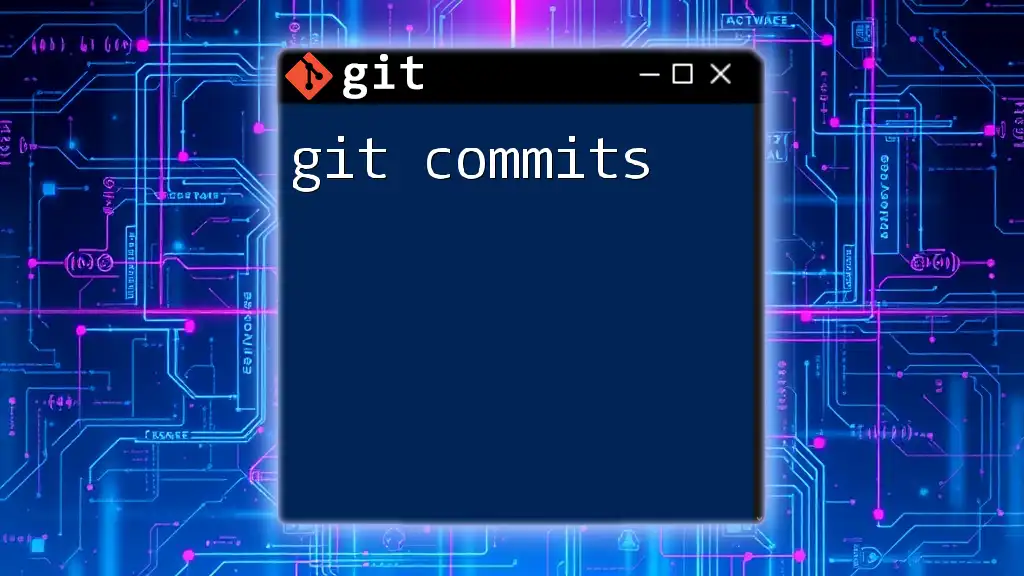
Filtering Commits with Options
Displaying a Limited Number of Commits
Sometimes, you may only want to see the most recent commits. Use the `-n` option to limit the output:
git log -n 5
This command displays only the last five commits, keeping the output concise and manageable.
Displaying Commit History for Specific Files
To examine the commit history of a particular file, you can specify the file path in your command:
git log -- path/to/file.txt
This command will list only those commits that made changes to `file.txt`, which is especially useful for debugging or reviewing changes specific to a component of your project.
Filtering Commits by Date
You can also narrow down your commit list based on dates using the `--since` and `--until` options:
git log --since="2023-01-01"
git log --until="2023-02-01"
These commands will show commits that occurred after January 1, 2023, or before February 1, 2023, respectively. Combining these options can provide focused results:
git log --since="2023-01-01" --until="2023-01-31" -n 10
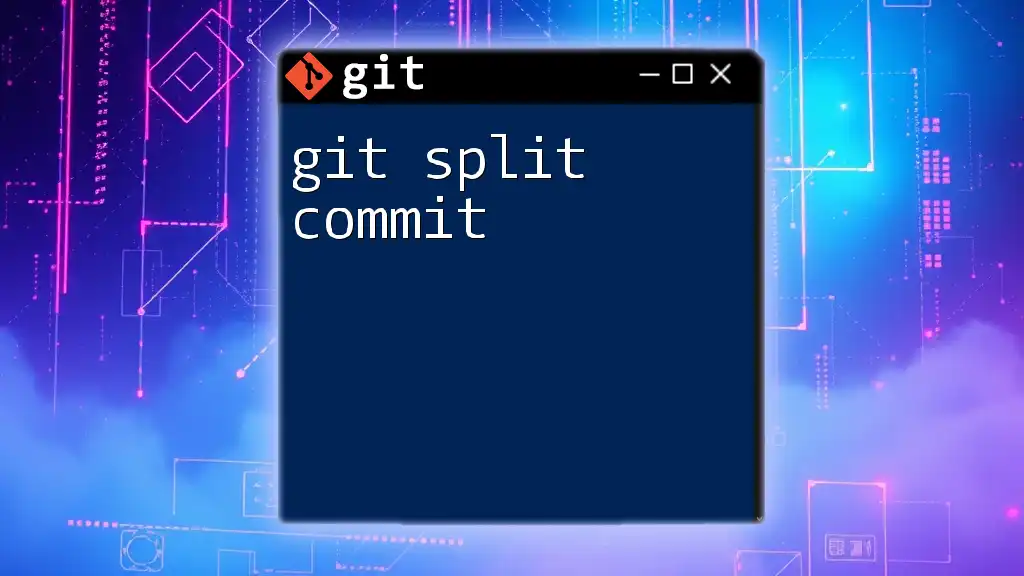
Formatting the Output
Pretty Printing Commits
Git allows you to customize the output format of the log, making it easier to read or export. Here are a couple of useful formats:
git log --pretty=oneline
The `--pretty=oneline` command will display each commit on a single line, which is useful for a quick overview. Alternatively, you can format it more descriptively:
git log --pretty=format:"%h - %an, %ar : %s"
In this format:
- `%h` represents the abbreviated commit hash,
- `%an` displays the author's name,
- `%ar` shows the relative date (e.g., "2 weeks ago"),
- `%s` is the commit message.
Exporting Commits to a File
For documentation or analysis, you may want to save the commit logs to a file:
git log > commit_history.txt
This command will write all commit details into `commit_history.txt`, allowing you to share or review it later.
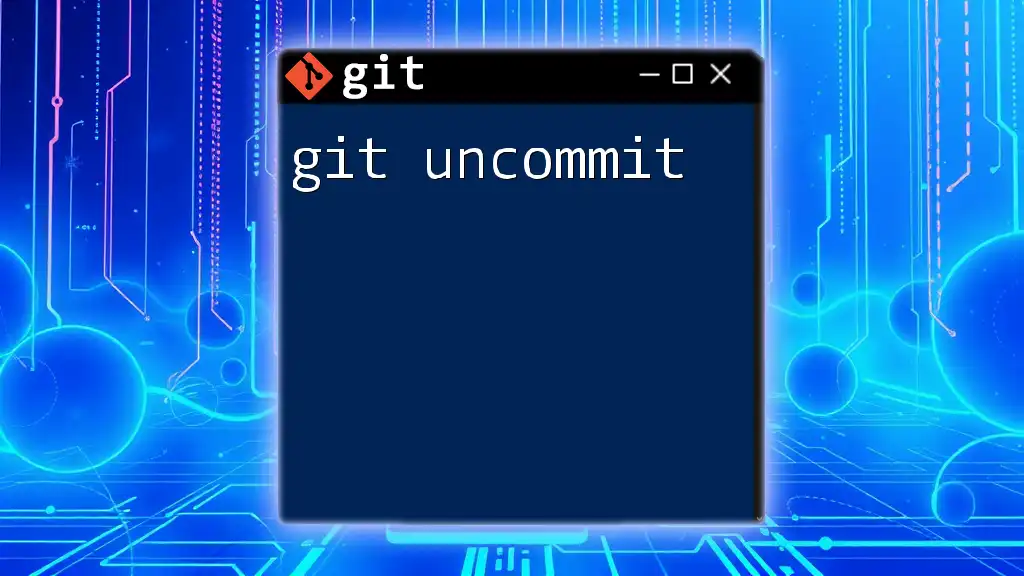
Advanced Commit Viewing
Viewing Commit Differences
To understand how a file has changed between commits, the `git diff` command becomes invaluable. You can compare the differences between two specific commits with:
git diff COMMIT_HASH_1 COMMIT_HASH_2
This shows you exactly what changed from one commit to another, which can be helpful for in-depth reviews.
Interactive Mode
For a more visual representation of your commit history, consider using `--graph` and `--all` flags:
git log --graph --all --decorate
This command creates a visual representation of your branches and merges, making it easier to see the project's development over time.
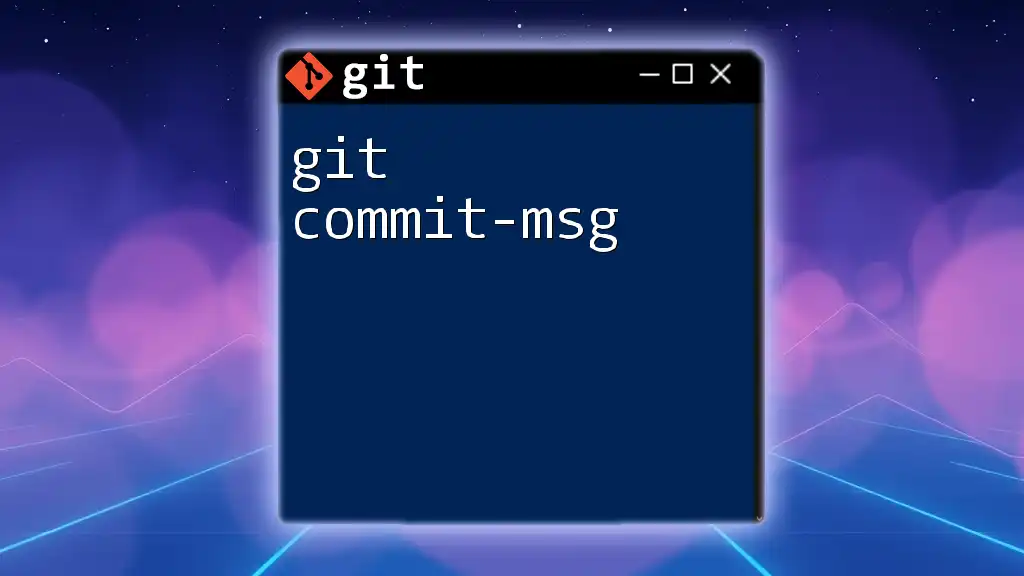
Conclusion
In conclusion, mastering the ability to list commits is a foundational skill in Git. Whether you are tracking changes, auditing contributions, or debugging code, knowing how to effectively use the `git log` command and its various options will greatly enhance your version control experience.
As you delve deeper into Git, I encourage you to explore these commands in your own projects. Practice will help you become proficient in managing and navigating your commit history effectively.
Keep refining your Git skills, and don’t hesitate to reach out or join in on classes that dive into the intricacies of Git commands for a more comprehensive understanding!