The `git -m commit` command is used to create a new commit with a message that describes the changes made to the repository in a single line.
Here's how to use it:
git commit -m "Your commit message here"
What is a Git Commit?
Definition of a Commit
A commit in Git represents a snapshot of your project at a specific point in time. It is a fundamental building block of Git’s version control system, allowing you to track and manage changes in your files and directories. Every commit you make records not just the changes but also a unique identifier (a hash), the author information, and a timestamp, which collectively create a comprehensive history of your project.
It is crucial to understand that commits are not simply a backup of your work. They encapsulate meaningful changes, serving as milestones in your project’s evolution. This is what makes version control incredibly powerful, especially in collaborative environments where multiple developers are making changes simultaneously.
The Role of the Commit Message
Every commit should be accompanied by a commit message, a brief description of what changes have been made. Commit messages are essential for several reasons:
- Clarity: They provide context, helping team members understand why a specific change was made.
- History Tracking: A good commit message helps you quickly look back through the project history to find the rationale behind decisions and changes.
- Collaboration: When working in a team, well-documented commits reduce confusion and facilitate smooth collaboration.
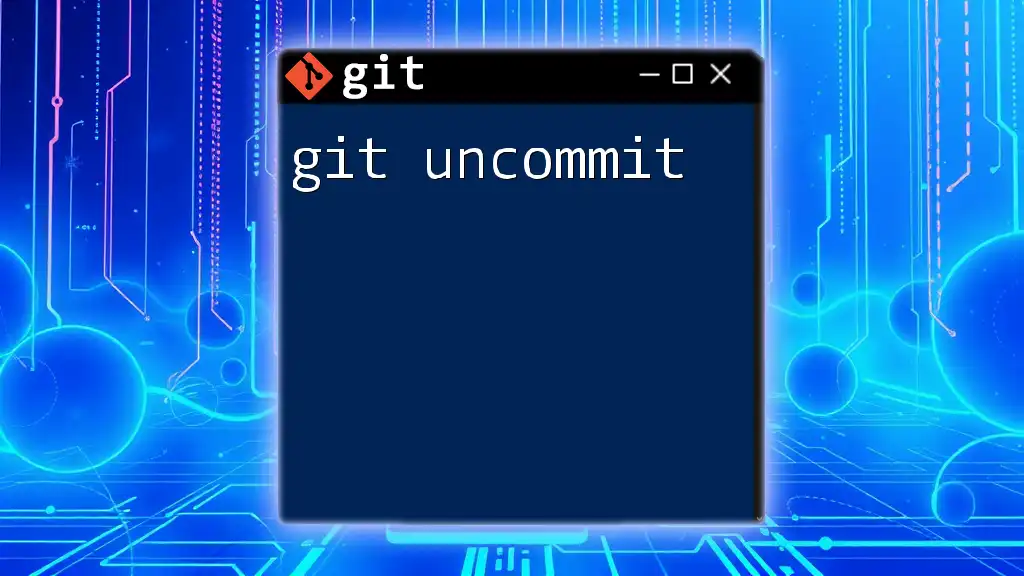
Using the `git commit` Command
Basic Syntax
The command to create a commit in Git is straightforward:
git commit
By default, this command will open an editor for you to write your commit message, allowing for detailed descriptions of changes.
The `-m` Option Explained
The `-m` option stands for "message" and enables you to include the commit message directly within the command, bypassing the need to open an editor. This is especially useful for simple changes or when you want to maintain a quick workflow.
Example of Using `git commit -m`
A simple example of this command would be:
git commit -m "Initial commit"
In this case, you'd be creating a commit with the message “Initial commit,” indicating the project's starting point. Using `-m` streamlines the process, allowing you to commit changes rapidly without additional steps.
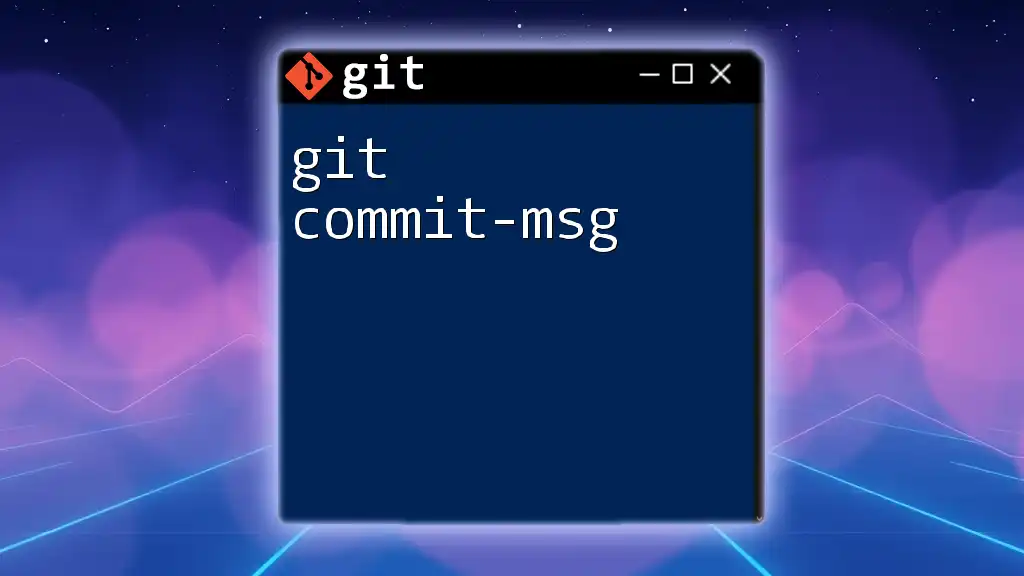
How to Write Effective Commit Messages
Best Practices for Commit Messages
Crafting an effective commit message is vital. Here are some best practices to follow:
- Keep it concise: Aim for a brief, descriptive subject line, ideally under 50 characters. This allows the message to be easily scanned.
- Use the imperative mood: Write messages as if you are giving an order (e.g., "Fix bug" rather than "Fixed bug"). This aligns with the way Git logs messages.
- Provide context when necessary: If your commit requires explanation for clarity, don’t hesitate to include a brief body.
Commit Message Structure
A well-structured commit message generally consists of two parts:
- Subject Line: A single line that summarizes the change.
- Body (if applicable): A detailed explanation of the change, its rationale, and any implications.
Example of a Well-Structured Commit Message
Here’s how to create a clear and informative commit message:
git commit -m "Fix bug in user authentication"
# Optional body
# This commit resolves the issue where users were unable to log in
# when two-factor authentication was enabled.
This format provides clarity to anyone reading the project history.
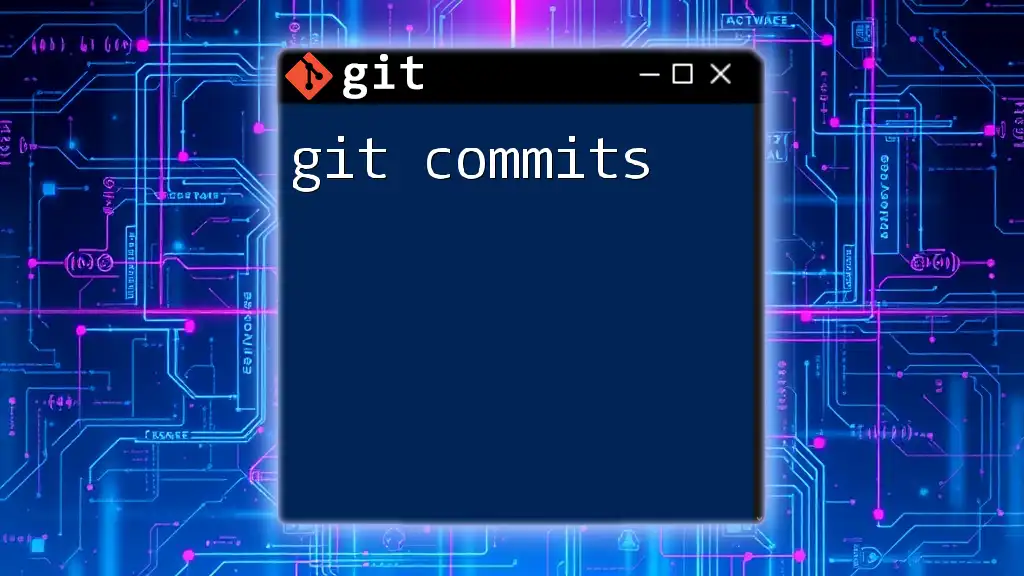
Common Mistakes When Committing
Forgetting to Stage Changes
One common mistake is forgetting to stage your changes before committing. The staging area acts as a buffer between your working directory and the repository, allowing you to decide which changes to include. Always remember to run:
git add .
before committing your changes, ensuring you're capturing what you intend.
Poorly Written Commit Messages
Committing changes without a clear or relevant message can lead to confusion later. Vague messages like "updated files" do not provide insight into what was changed or why. Take the time to write thoughtful messages that reflect the essence of your modifications.
Overusing `-m` Without Context
While the `-m` option is a powerful tool for quick commits, over-reliance on it can lead to poorly documented changes. For complex changes or when multiple related alterations are made, consider using:
git commit
to write a more detailed commit message in your editor.
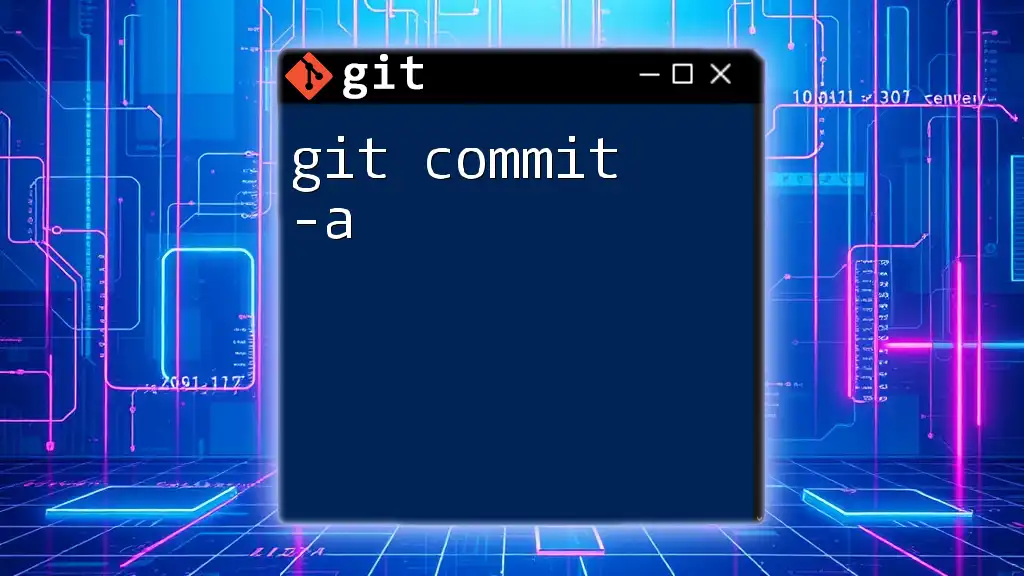
Advanced Usage of Git Commit
Using the `-m` Option in Scripts
The `-m` option can be particularly useful when scripting your Git commands, as it allows for non-interactive commits. For instance, if you are automating a deployment process, you might use:
git add . && git commit -m "Automated deployment on [date]"
This efficiently packages your changes and documentation into a single command.
Combining with Other Git Commands
You can chain multiple Git commands for efficiency. For example:
git add . && git commit -m "Updated project files"
This command first stages all changes and then commits them in one seamless operation, saving time and effort.
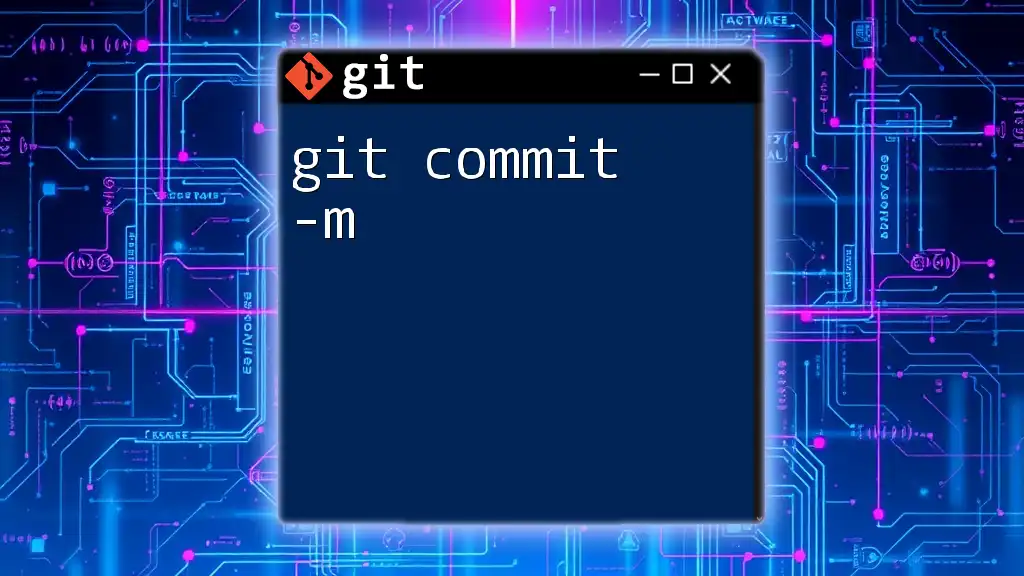
Conclusion
Understanding and effectively utilizing the `git -m commit` command is essential for maintaining a clean and understandable project history. Proper commit messages can greatly enhance collaboration and clarity within your projects. Make committing a significant part of your workflow, and always aim for clarity and context in your messages. Practicing these skills will lead to a more organized and maintainable codebase, benefiting everyone involved in the project.
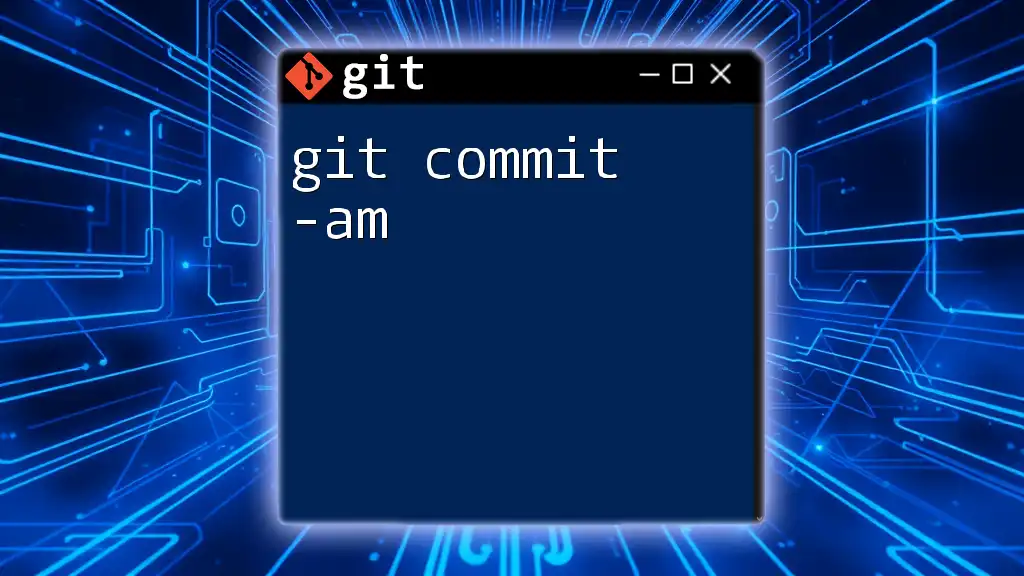
Additional Resources
For further reading and to enhance your Git skills, refer to the official [Git documentation](https://git-scm.com/doc), which provides comprehensive insights into best practices and advanced usage of Git commands. Additionally, numerous online tutorials cover various aspects of Git, catering to beginners and advanced users alike.