The `git commit -m` command is used to create a new commit in your Git repository with a brief, descriptive message summarizing the changes made.
Here's an example of how to use it:
git commit -m "Fix typo in README file"
What is `git commit`?
Definition and Purpose
The `git commit` command is a fundamental component of the Git version control system. It allows users to take a snapshot of the changes made to their codebase. Each commit serves as a checkpoint in the project’s history, allowing developers to track their progress or revert to earlier states if necessary. When you commit changes, you’re solidifying a specific aspect of your project, ensuring that you can effectively manage and share your work with others.
The Role of Commit Messages
Commit messages are a critical part of every commit. They provide context to the changes made, making it easier for collaborators (and your future self) to understand why changes were implemented. A well-crafted commit message can clarify the intent behind the changes, making the overall development process more transparent and manageable.
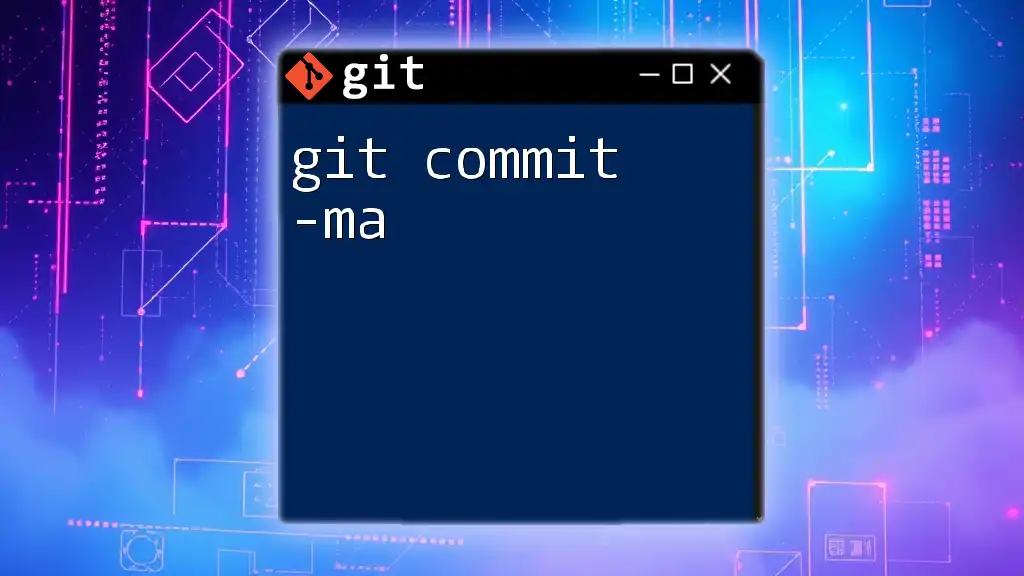
Understanding the `-m` Flag
Explanation of the `-m` Option
The `-m` flag in the `git commit` command stands for "message." This option allows you to provide a commit message directly within the command line, eliminating the need to open a text editor to enter your message. By using `git commit -m`, you simplify the commit process and can quickly document your reasons for making changes without interrupting your workflow.
Syntax and Usage
The general syntax of the command is as follows:
git commit -m "Your commit message here"
This structure conveys to Git that you want to create a commit that includes the staged changes, along with a specific message describing those changes.
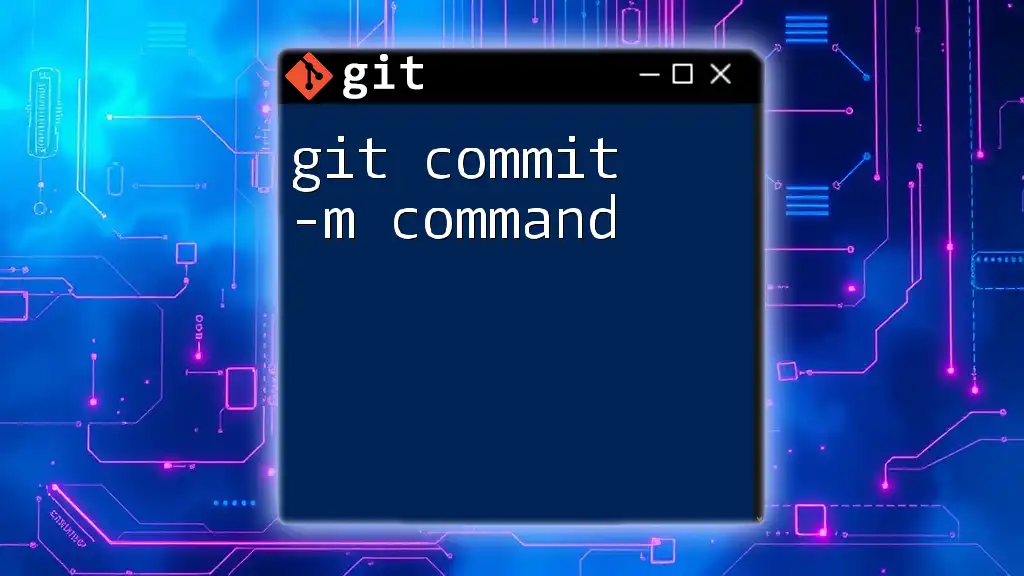
Writing Effective Commit Messages
Best Practices for Commit Messages
Writing effective commit messages is crucial for maintaining a clear project history. Here are some key tips:
- Be concise yet descriptive: Aim for a message that is specific but not overly lengthy. A good balance ensures that readers immediately grasp the purpose of the commit.
- Use the imperative mood: Start your message with a verb in the imperative form, such as "Fix," "Add," or "Update." This style is consistent with the way Git summarizes changes, making it easier for others to read.
Examples:
- Good message: `Fix typo in README.md documentation`
- Bad message: `Fixed a typo in the documentation`
Types of Commit Messages
Different types of commits can warrant different styles of messages. It’s useful to categorize your changes to make the history easier to navigate:
- Feature additions: Commit messages should clearly announce new features. Example:
git commit -m "Add user authentication feature"
- Bug fixes: Be explicit about what was fixed. Example:
git commit -m "Fix crash on login failure"
- Documentation updates: Indicate updates made to documentation with clarity. Example:
git commit -m "Update license information in documentation"
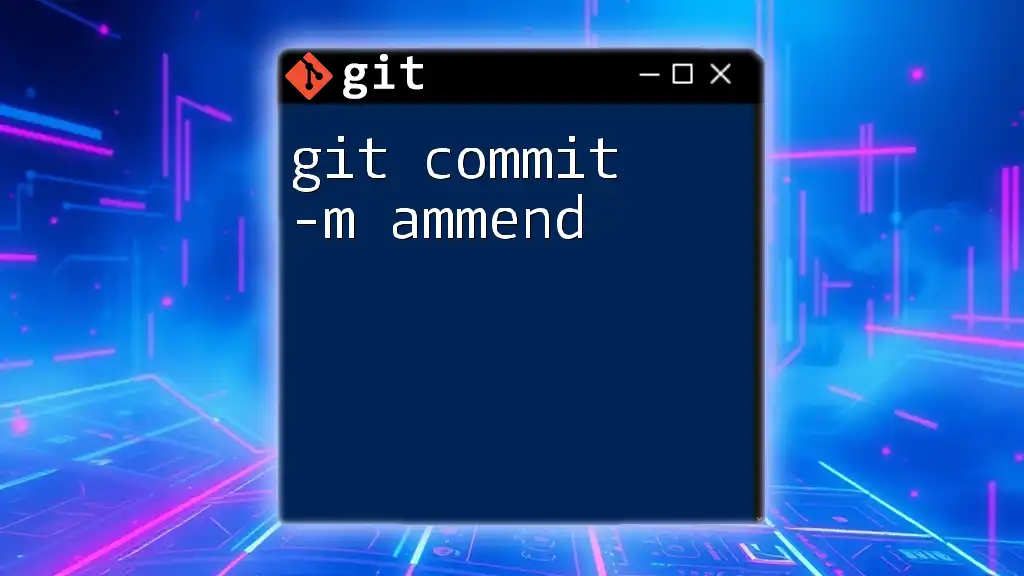
Common Scenarios Using `git commit -m`
Committing Changes After Modifications
When you modify a file and want to commit those changes, ensure you first stage the file. Here’s a step-by-step process:
-
Modify a file, for instance, `file.txt`:
echo "New content" >> file.txt
-
Stage the changes:
git add file.txt
-
Commit the changes with a message:
git commit -m "Add new content to file.txt"
Staging Multiple Files for a Single Commit
Sometimes you may make changes across multiple files and want to group those changes in a single commit. This can be accomplished easily by staging all modified files together:
git add file1.txt file2.txt
git commit -m "Update file1 and file2 with new information"
Amending Commit Messages
If you realize that you need to modify the message of your most recent commit, Git allows you to do so using the `--amend` option. This can be particularly useful for correcting typos or adding additional context:
git commit --amend -m "Correct last commit message"
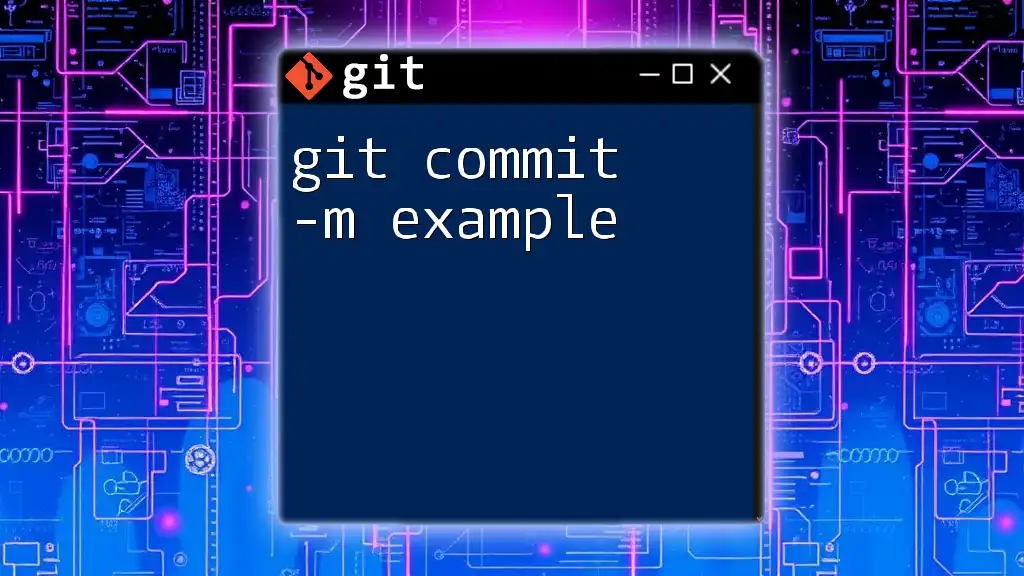
Troubleshooting Common Issues with `git commit -m`
Missing Staged Changes
One common issue developers face is attempting to commit without staging any changes. In such cases, Git will notify you that there are no changes to commit. To resolve this, ensure you have staged the desired files first using `git add`.
Writing Commit Messages with Special Characters
When crafting commit messages that include special characters, such as quotes, proper syntax is necessary to ensure they are interpreted correctly. This can be done as follows:
git commit -m "Implement feature \"X\" for better user experience"
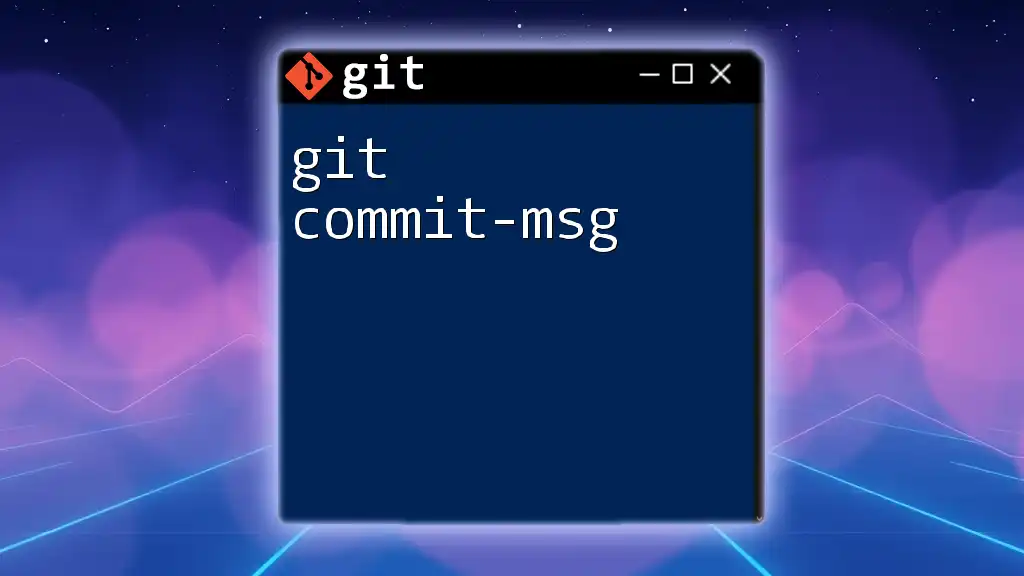
Integration with Other Git Commands
`git add` and `git commit`
Understanding the relationship between `git add` and `git commit` is essential for effective Git usage. The `git add` command stages changes, while `git commit` finalizes those changes. A typical workflow might look like this:
git add .
git commit -m "Stage all modified files"
Viewing Commit History
After making several commits, you may want to review your commit history to see how your project has evolved or to track down specific changes. You can use:
git log --oneline
This command provides a summarized view of your commit history, showing each commit's hash and message.
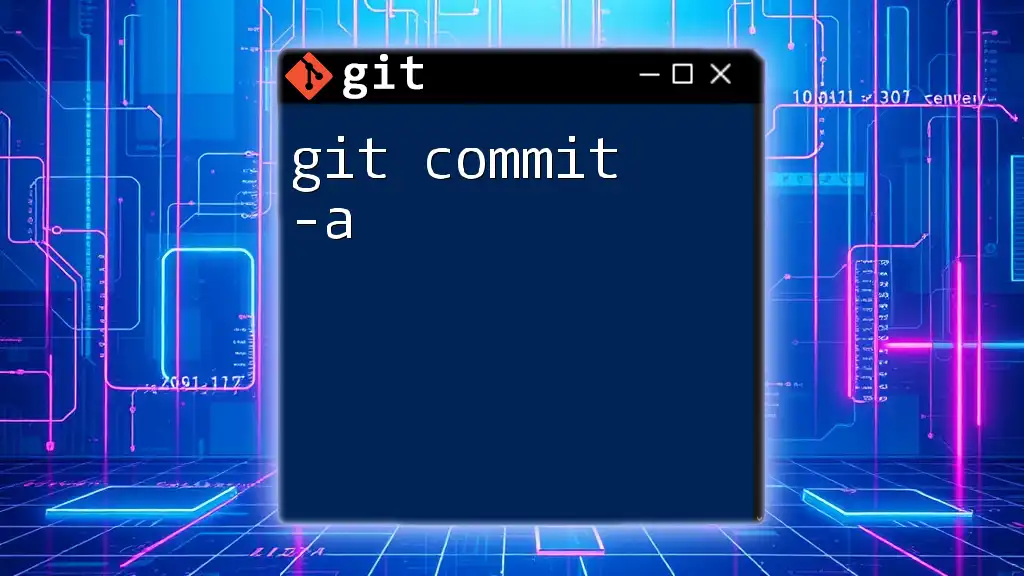
Conclusion
Understanding and effectively using `git commit -m` is vital for anyone working in a collaborative coding environment. By crafting clear and concise commit messages, you not only document your work but also enhance team communication and project tracking. Embrace best practices, and remember, every commit is a piece of your development journey!
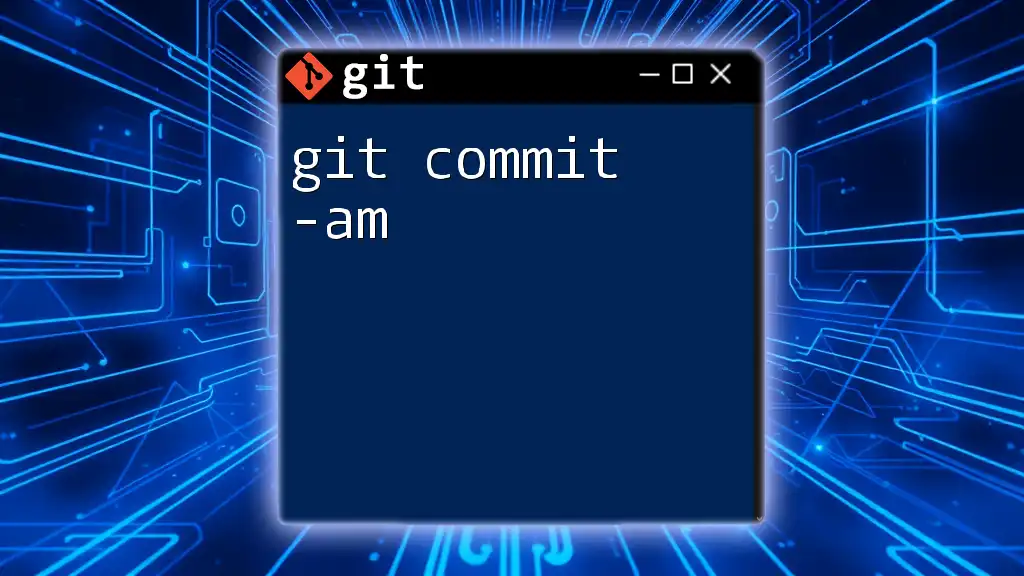
Additional Resources
For those eager to expand their knowledge further, consider diving into the official [Git documentation](https://git-scm.com/doc) and exploring various tutorials available online. Mastery of Git enhances your collaboration capabilities and contributes to the success of your software projects.