The `git commit -m` command is used to create a new commit with a concise message describing the changes made, allowing for clear version history in your Git repository.
git commit -m "Add README file with project instructions"
What is `git commit`?
The `git commit` command is a fundamental part of using Git for version control. It serves the purpose of capturing a snapshot of the changes made to the files in your repository. When you commit changes, you are essentially creating a new point in the project history that can be referred back to later.
Every commit is stored in the repository's history and can include a message that describes the changes made. This helps both you and others understand what happened at that particular point in time.
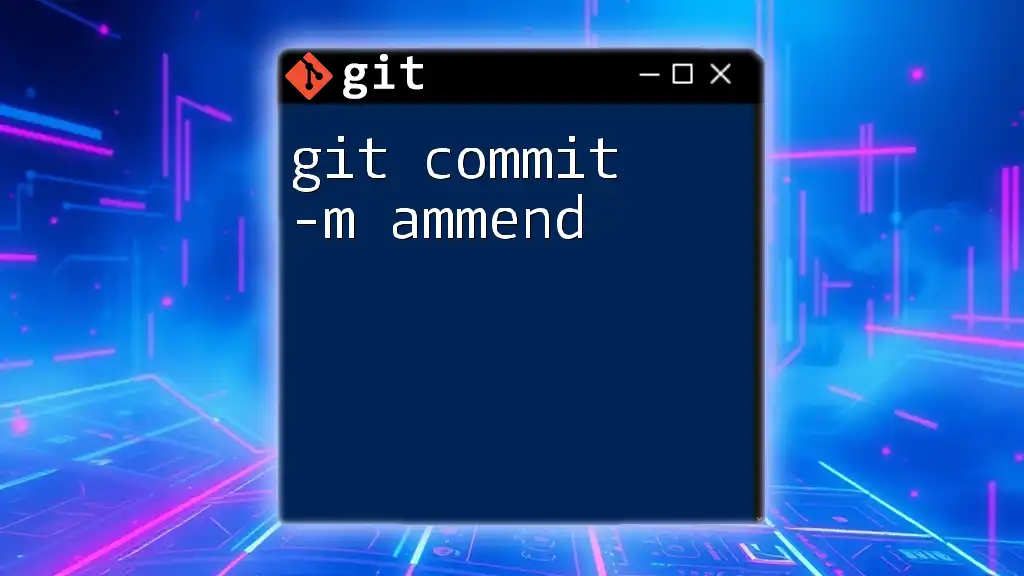
Why Use the `-m` Option?
The `-m` option, short for "message," allows you to include a commit message inline when you commit your changes. Utilizing the `-m` option simplifies the commit process by allowing you to save your changes without launching a text editor. This option is particularly helpful for users who prefer working within a command-line interface and want to avoid the interruption of switching contexts.
The main advantages of using `-m` include:
- Speed: Quickly committing with a message saves time.
- Simplicity: Reduces the steps required to commit files, making it easier for new users to learn.
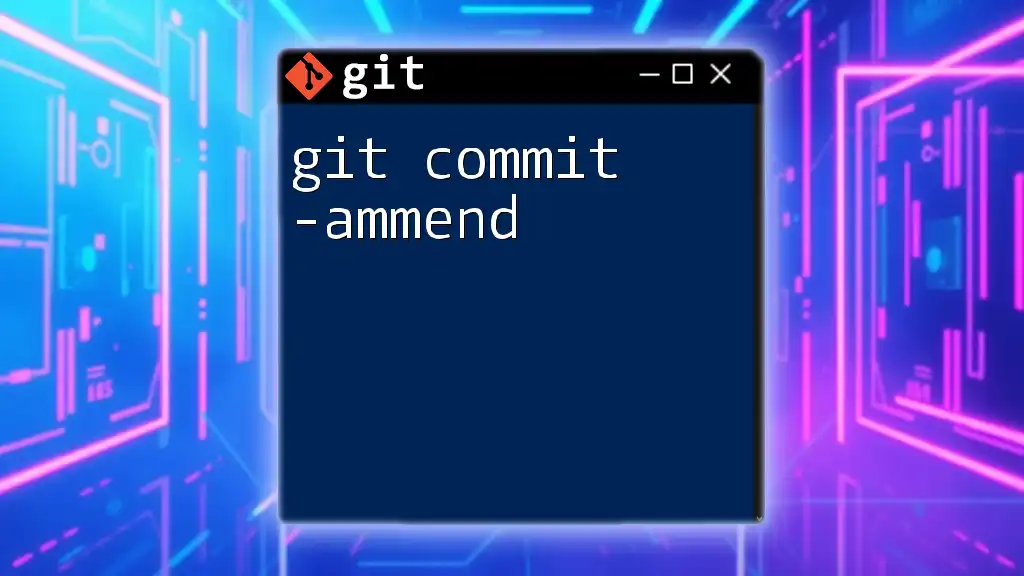
Basic Syntax of the `git commit -m` Command
To use the `git commit -m` command, the syntax is straightforward:
git commit -m "Your commit message here"
This command can be broken down into several components:
- `git`: The command-line interface for Git.
- `commit`: The action to save your staged changes.
- `-m`: The option that allows you to write your commit message directly.
- `"Your commit message here"`: The text that provides context about what changes were made.
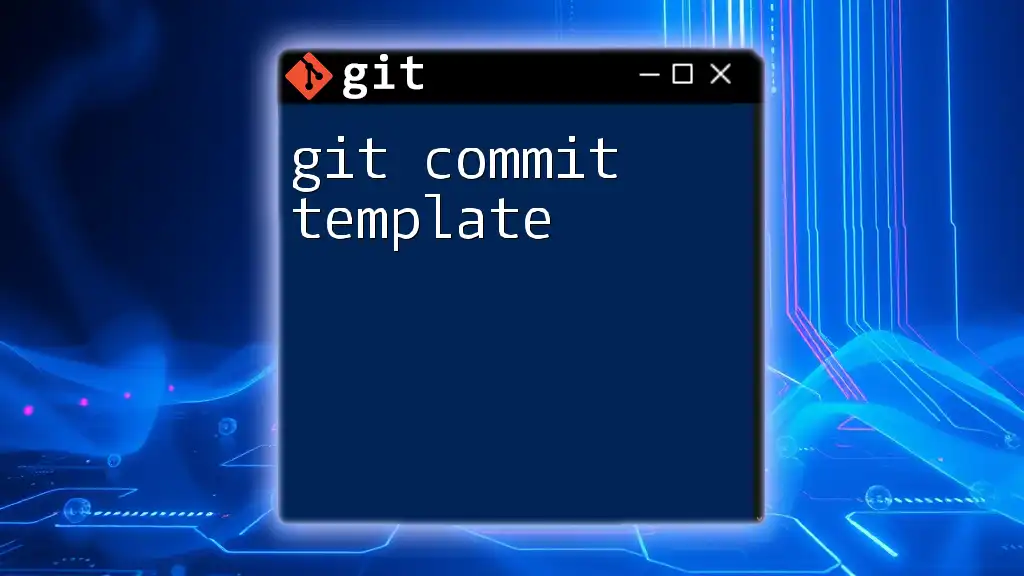
Crafting Effective Commit Messages
Importance of Commit Messages
Commit messages play a crucial role in collaboration and code review processes. Clear and thoughtful messages provide context for changes, making it easier for teammates and reviewers to understand the rationale behind modifications. A well-documented project history can significantly improve maintainability.
Components of a Good Commit Message
Crafting an effective commit message typically involves several components:
-
What to include:
- A brief summary (typically 50 characters or less) that encapsulates what the change is about.
- A detailed explanation in the body of the message, if the changes are complex or require further clarification.
-
Length and clarity:
- Strive for conciseness in the summary.
- If you write a body, consider keeping it around 72 characters per line for readability.
Common Practices
Following certain common practices can enhance the quality of your commit messages:
- Use the imperative mood, such as "Add feature" or "Fix error." This approach gives your messages a clear and actionable tone.
- Reference issue numbers where applicable, for instance, "Closes #123," to provide additional context for the changes.
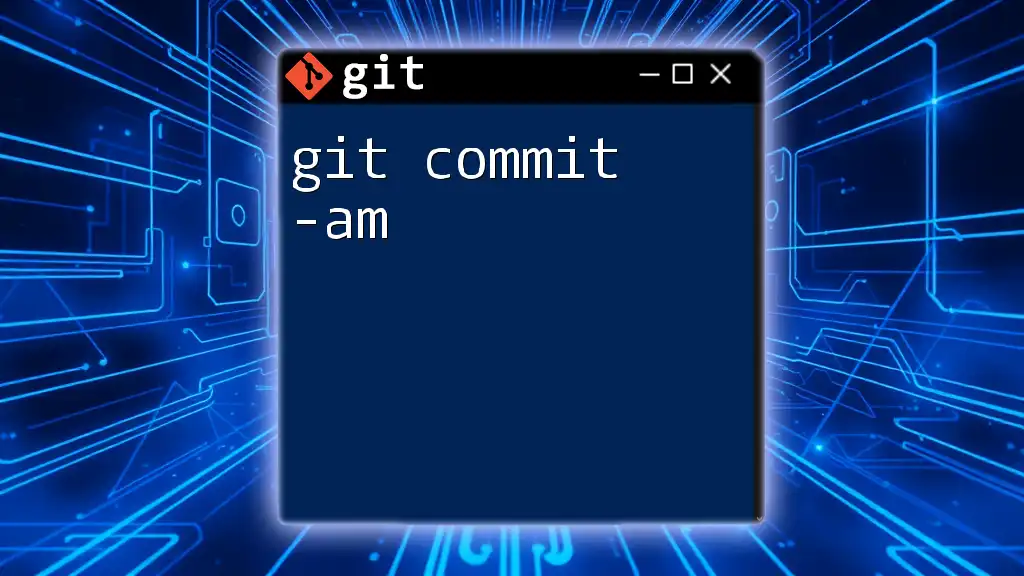
Examples of `git commit -m` Command
Basic Example
For a straightforward example, consider updating a README file to better explain project setup instructions:
git add .
git commit -m "Update README with installation instructions"
In this case, you staged all changes using `git add .`, which prepares everything for the commit, and then provided a clear, informative message that describes the change being made.
Example with Multiple Commits
When working on a project, developers often make multiple changes that need to be committed incrementally. Here’s how that might look:
git add file1.txt
git commit -m "Add file1 and update file structure"
git add file2.txt
git commit -m "Create file2 and add initial content"
This sequence illustrates making multiple commits with specific descriptions, aiding future reference in understanding why each change was made.
Example Referencing Issues
Referencing issues in your commit messages helps in tracking progress related to specific tasks or bugs. An example can be formatted like so:
git add bugfix.py
git commit -m "Fix login issue (Closes #42)"
This message indicates the direct link between the commit and an issue tracked in a project management tool, providing clarity on what was resolved.
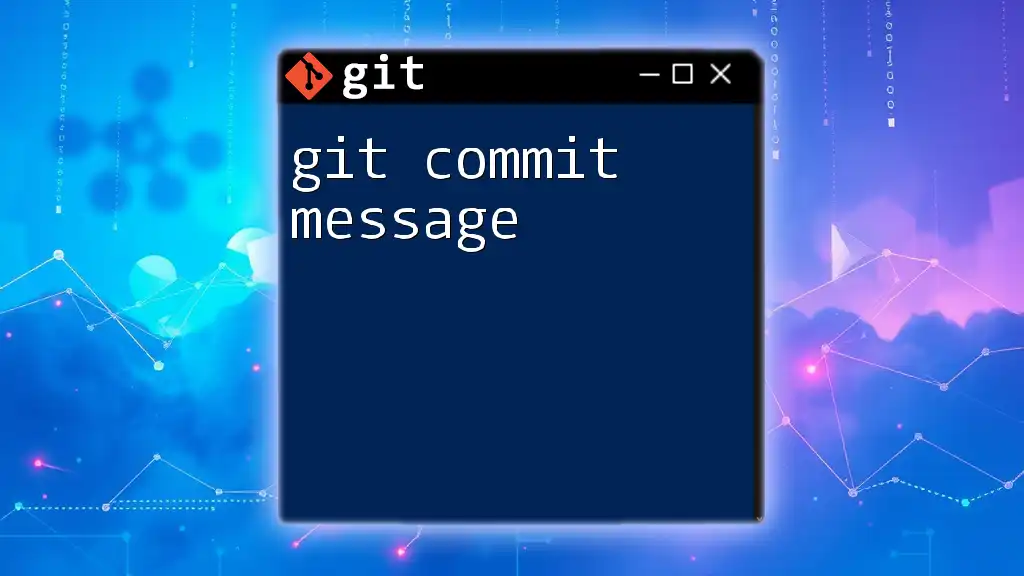
Common Mistakes to Avoid
Not Using Commit Messages
One significant pitfall is failing to include commit messages altogether. This can leave your project history unclear and untraceable, making it difficult for anyone reviewing the commits to understand what changes were made and why.
Vague Commit Messages
Avoid vague phrases like "Fixed stuff" or "Changes made.” Such messages are unhelpful and hinder collaboration by leaving others guessing about the nature of the revisions.
Overly Verbose Commit Messages
While it’s important to be detailed, overly lengthy messages can become cumbersome. Strive for clarity and conciseness to ensure messages are easy to digest.
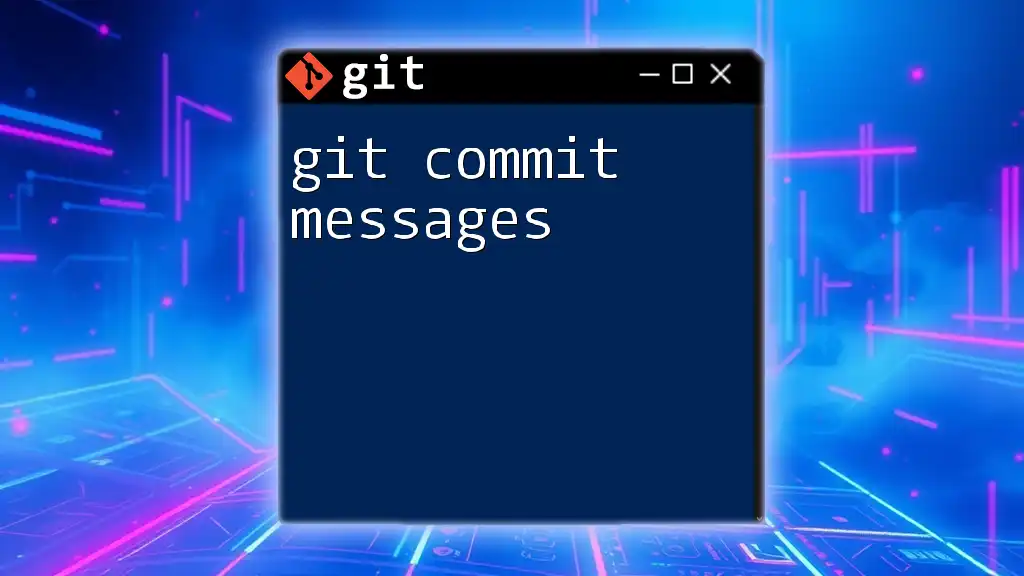
Checking Your Commit History
Using `git log`
To view your commit history, utilize the command:
git log
The output will list recent commits alongside their messages, allowing you to see and understand your project’s progression over time.
Filter Commit History
Git also provides features to filter your commit history. For example, if you want to look for commits that mention "bugfix," you can use:
git log --grep="bugfix"
This will return a list of commits that match your search criteria, making it easier to navigate through potentially vast sets of project history.
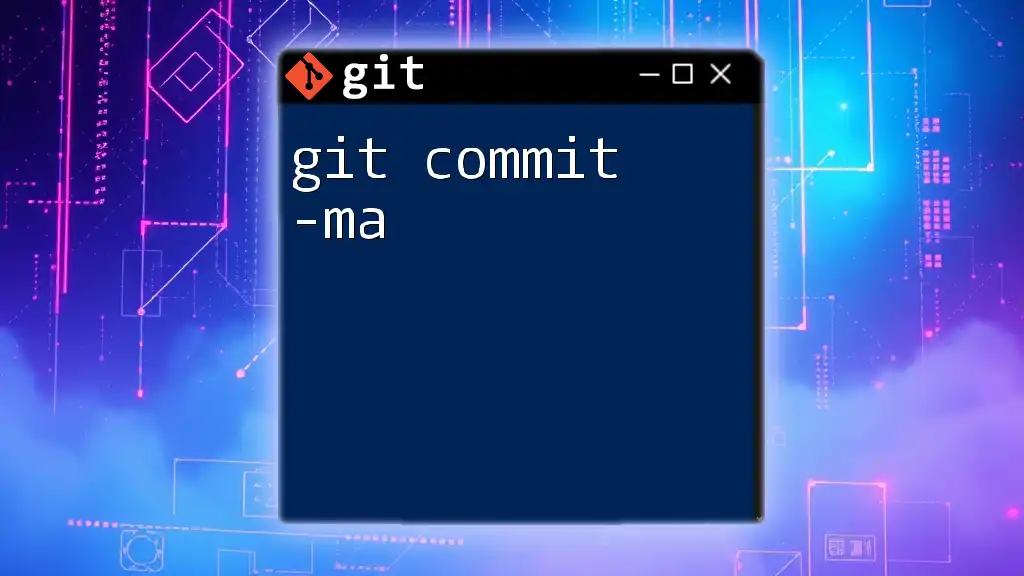
Conclusion
In summary, the `git commit -m` command is an essential tool in your Git workflow for managing version control effectively. By crafting meaningful commit messages, you not only document your changes clearly but also facilitate better collaboration and code reviews. Practice writing concise, informative messages and contribute to the overall quality and maintainability of your projects. Dive deeper into Git usage to enhance your development skills and maximize your workflow’s efficiency!
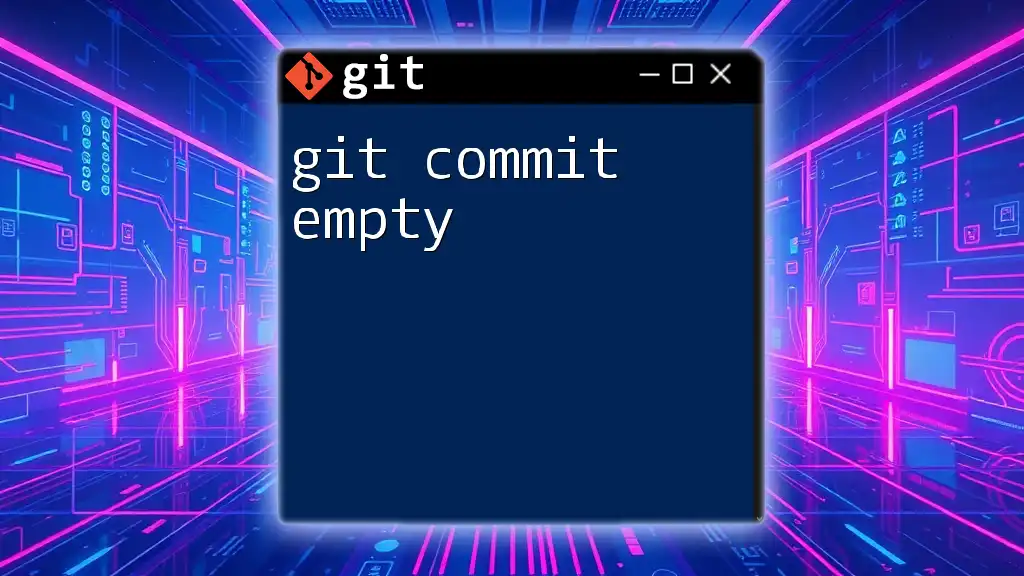
Further Reading and Resources
To continue your journey with Git, consider checking out the official Git documentation, which offers comprehensive guidance. Additionally, various online courses are available to deepen your understanding of Git, and community forums can provide further insights and support. Engaging with these resources will bolster your ability to use Git effectively in both individual projects and collaborative environments.