To commit multiple files in Git, simply stage the desired files with `git add` and then execute `git commit` with a relevant message.
git add file1.txt file2.txt file3.txt
git commit -m "Commit message for multiple files"
Understanding Git Commits
What is a Git Commit?
In version control, a commit represents a snapshot of your repository at a specific point in time. Each commit acts as a unique identifier for your code, storing a complete set of changes made since the last commit. When you create a commit, you should include a commit message that describes the changes. A well-crafted commit message enhances clarity and allows collaborators (and your future self) to understand the purpose of the commit without needing to dive into the code.
Why Commit Multiple Files?
Committing multiple files in a single commit is essential for several reasons:
-
Keeping Related Changes Together: Grouping changes that affect the same feature or fix promotes clarity in your project's history. For example, if you modify several files to implement a new feature, committing all those changes as one preserves the context.
-
Streamlining the Development Process: Committing together saves time and allows developers to focus on specific tasks without worrying about scattering their changes across multiple commits.
-
Creating a Clear History of Changes: A clear commit history that reflects logical groupings of changes makes it easier to track progress, revert changes, or identify when specific changes were made.
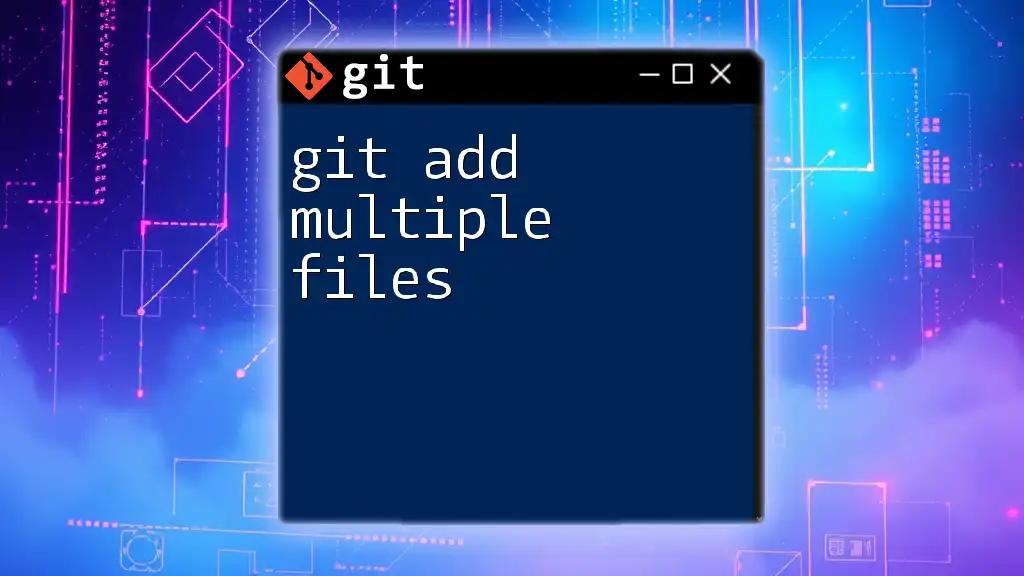
Preparing to Commit Multiple Files
Adding Files to the Staging Area
The staging area acts as a middle ground for changes before they're committed. When you stage files, you're preparing them for inclusion in your next commit.
Command: `git add`
To stage multiple files, you can specify each file individually, like this:
git add file1.txt file2.txt file3.txt
After staging, you should check the status to ensure the desired files are staged correctly:
git status
Using Patterns to Add Files
If you have many files to stage, you can use wildcards to simplify the process. For instance, if you want to add all text files in the current directory, you can use:
git add *.txt
Alternatively, to add all changes across the repository, use:
git add -A
or
git add .
This command stages all modified and deleted files, making it a powerful tool for preparing for your next commit.
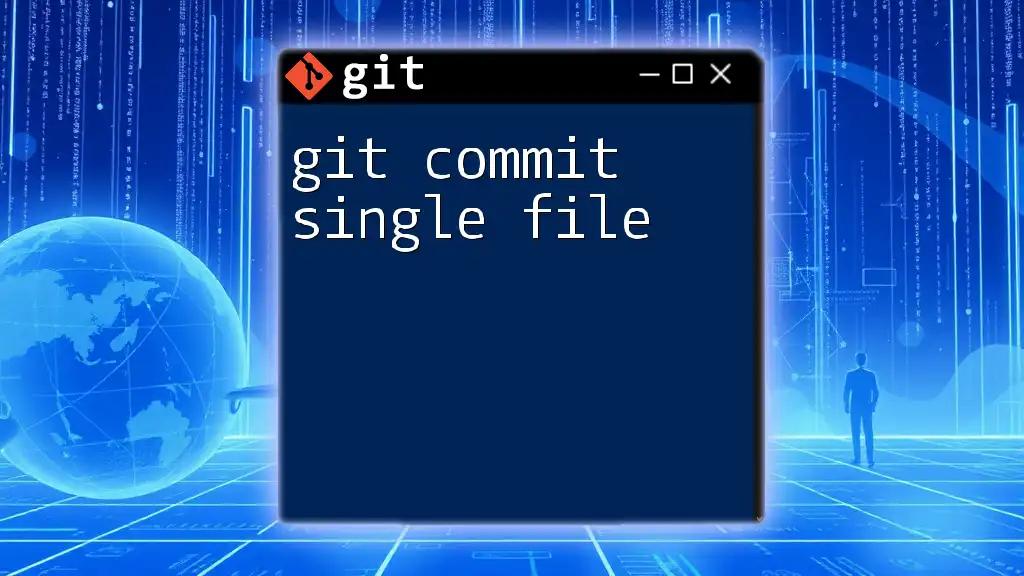
Committing Changes
Making the Commit
Once you've staged your files, you can create a commit that includes all the files you've added.
Command: `git commit`
Use the following command to commit the changes with a meaningful message:
git commit -m "Updated multiple text files"
It’s essential to write concise messages that explain the changes you've made. Clear commit messages are vital for maintaining project clarity and facilitating future collaboration.
Using `git commit -a`
If you prefer to skip the staging step for modified files, you can use the `-a` flag. This will automatically stage all modified files and commit them in one command.
Example:
git commit -a -m "Updated all modified files"
However, keep in mind that this command won't add new files; you must stage newly created files manually.
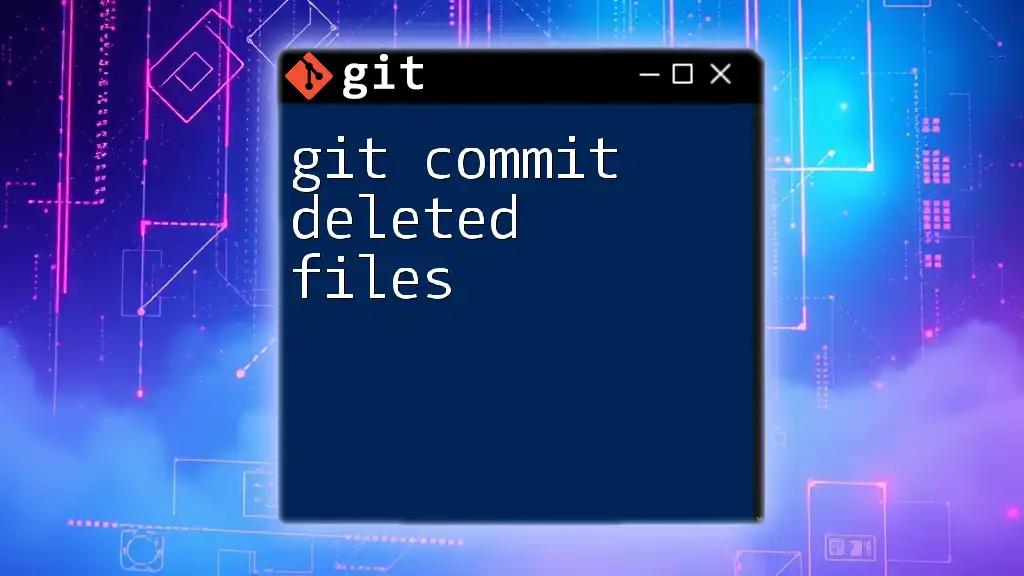
Advanced Techniques
Committing with Interactive Mode
Sometimes, you may want to review changes before you commit. Using the `-v` flag allows you to see a brief overview of the changes alongside your commit message:
git commit -v
This mode is exceptionally helpful when you want to ensure you haven’t missed any critical updates.
Committing with a Patch
For advanced users, `git add -p` allows you to select specific changes to stage and commit, referred to as "patching." This feature is particularly useful when you want to include only part of the changes made to a file.
Example:
git add -p
You'll enter a more interactive mode, allowing you to review each change, and you can choose to stage specific hunks or all.
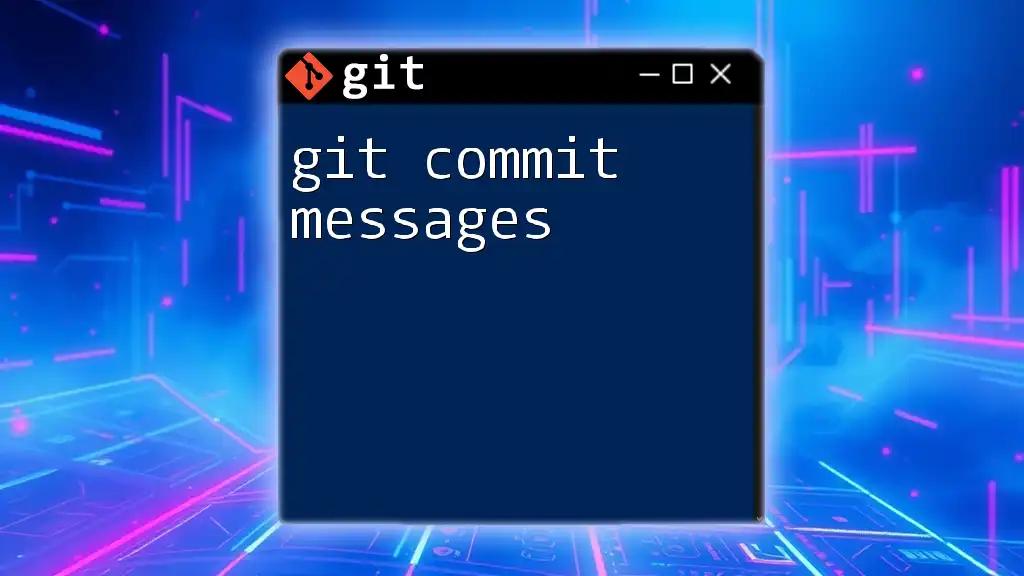
Best Practices for Committing Multiple Files
Keep Related Changes Together
Maintaining logical groupings of changes in a single commit is essential. When you keep related changes together, it provides a clearer and more understandable project history. For instance, if you're adding a new feature, ensure all related files (such as code, tests, and documentation) are included in that commit.
Write Clear Commit Messages
Writing effective commit messages is vital. Here are some best practices for crafting messages:
- Use the imperative mood. For example, write “Fix bug” instead of “Fixed bug.”
- Reference any relevant issue numbers from your project management system (e.g., “Fixes #42”).
- Be concise yet informative. A good structure might include a short summary followed by a more detailed explanation, if necessary.
Review Your Commits
Before committing, always review the changes. The `git diff` command helps you compare staged changes with your last commit to ensure you’re not including unintended edits.
git diff --cached
This command shows you the changes you've staged for commit, allowing you to double-check everything.
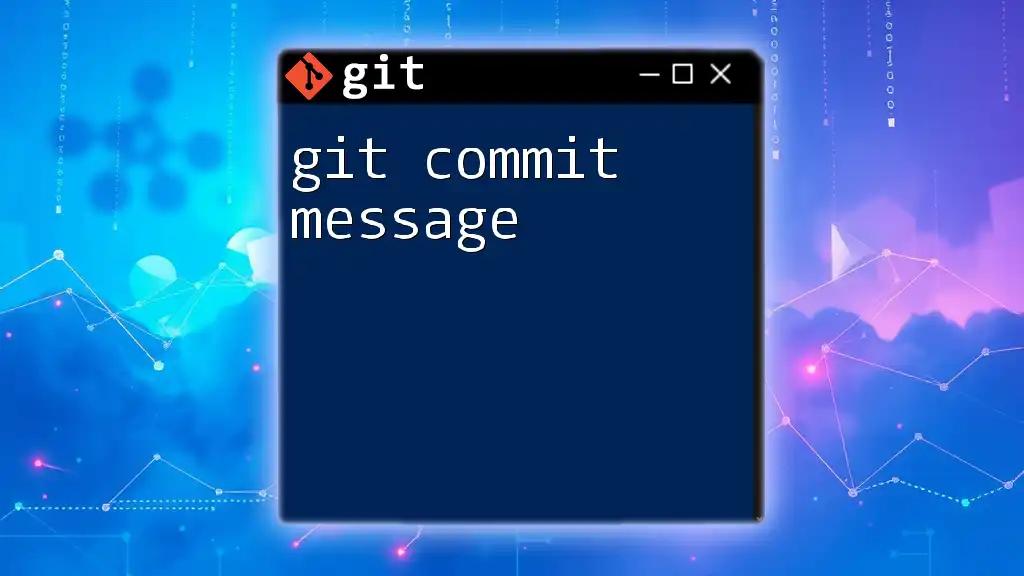
Conclusion
Understanding how to effectively git commit multiple files is a crucial skill for any developer using Git. By mastering the techniques for staging and committing multiple files, you can maintain a clean project history and simplify your workflow. Commit thoughtfully, keep related changes together, and always craft clear commit messages to improve collaboration and project management. A well-organized commit strategy will serve you and your team well in the long run.
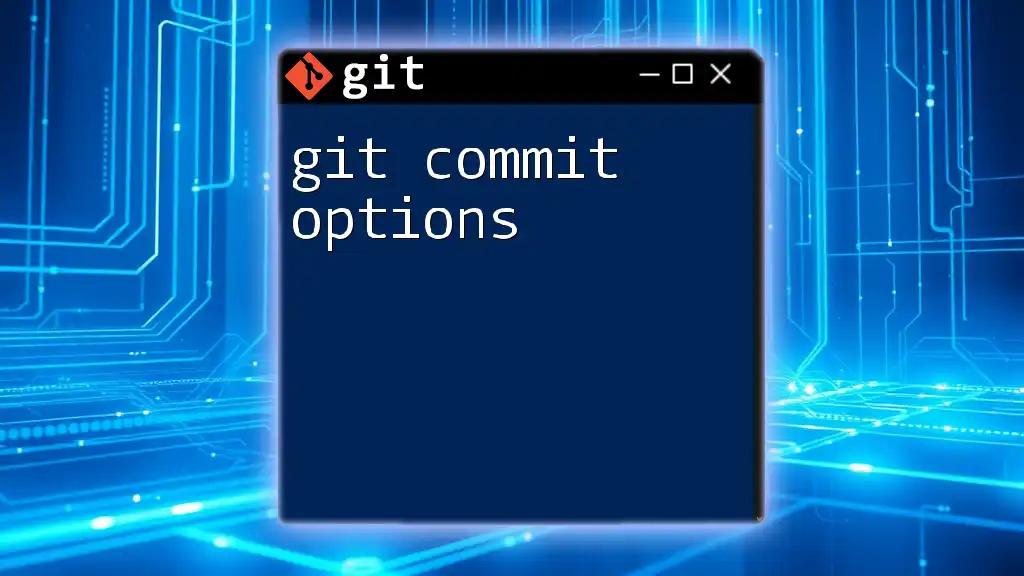
Additional Resources
For those looking to explore Git further, the official documentation is an excellent place to start. Various Git tutorials and courses are also available online to enhance your skills.