When creating commits in Git, it's essential to write clear, descriptive messages that convey the purpose of the changes and follow best practices to maintain a clean project history.
Here's a simple example of a Git commit command with a best practice message:
git commit -m "Fix typo in documentation for installation instructions"
Understanding Git Commits
What is a Git Commit?
A Git commit is a snapshot of your changes that is saved in the repository. Each commit serves as a record of alterations made to the codebase. This is a fundamental aspect of version control systems, allowing developers to rollback changes, track progress, and collaborate effectively.
Why Commits Matter
Properly executed commits can significantly enhance the maintainability and usability of your codebase. Good commit practices help in creating a clear history of changes, making it easier to understand why modifications were made. When reviewing code or debugging issues, a clean commit history can save time and reduce frustration.
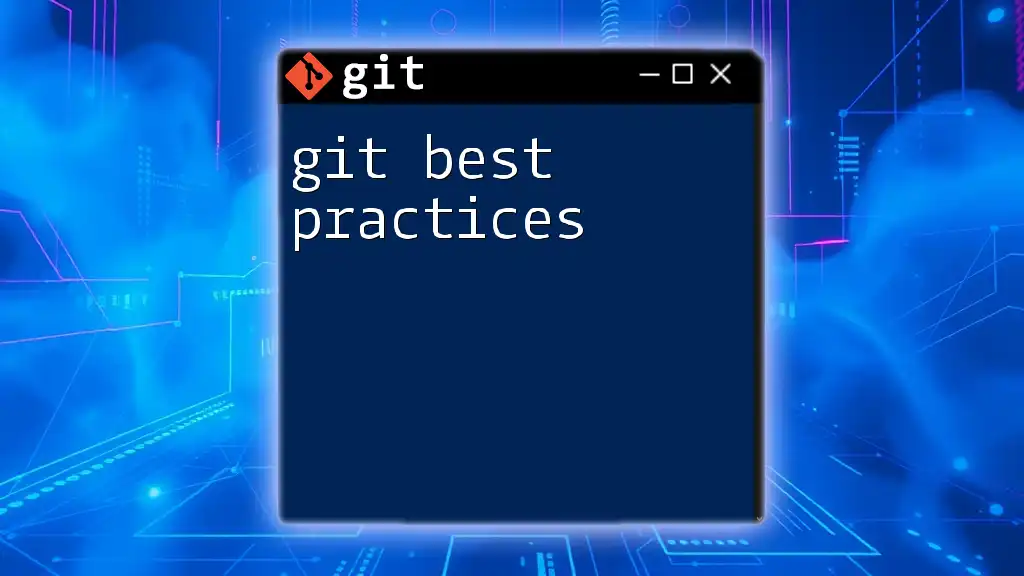
Best Practices for Git Commits
Use Clear and Descriptive Commit Messages
Writing clear and descriptive commit messages is crucial for effective communication among team members. A well-crafted commit message serves as a summary of what changes were made and why.
Example Commit Message:
Fix: Correct infinite loop in data processing
This message tells the reader that a bug was fixed and provides a high-level overview of the change.
Follow a Consistent Format
Consistency in commit message formatting helps maintain clarity throughout the project. Using the imperative mood is a common practice in commit messages.
Example of Imperative Style:
Add user authentication feature
This format makes it easy for anyone reviewing commit history to understand what the code is doing at first glance.
Message Structure
A well-structured commit message typically consists of three parts: subject, body, and footer.
-
Subject Line: This should be a brief summary, ideally not exceeding 50 characters. It encapsulates the essence of the changes.
-
Body: The body provides a detailed explanation of the changes and their rationale. It is recommended to wrap text at 72 characters to improve readability.
-
Footer: Includes any references to issues, tickets, and breaking changes, helping to connect the commit to specific project management tasks.
Example Commit Message:
Refactor user profile handling
Simplify the user profile loading process by eliminating redundant API calls. This change improves response time and reduces server load.
Closes #154, #157
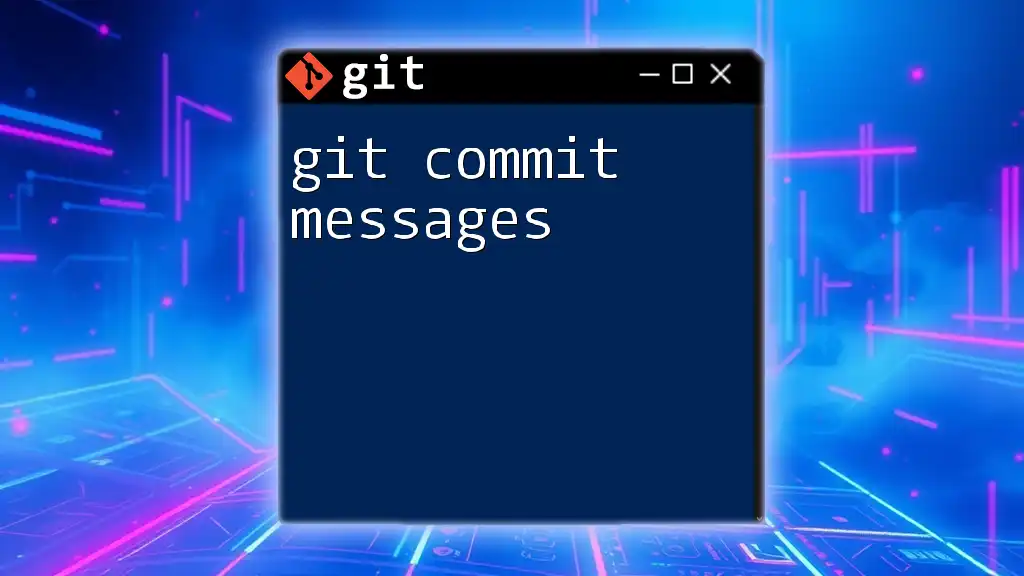
Keeping Commits Small and Focused
Why Small Commits are Beneficial
Making small and focused commits is advantageous for managing your project's history. Small commits allow for easier reviews because they usually contain one identifiable change. This minimizes the risk of introducing large-scale issues and simplifies the rollback of changes when necessary.
Techniques for Effective Commits
-
Atomic Commits: This principle stipulates that each commit should include changes related to a single task or feature. This keeps the project organized and makes any future fixes simpler because you can address changes without affecting other functionality.
-
Use Feature Branching: Creating separate branches for features or bug fixes allows you to isolate your work, making it easier to manage and review. Once the feature is complete and verified, it can be merged back to the main branch.
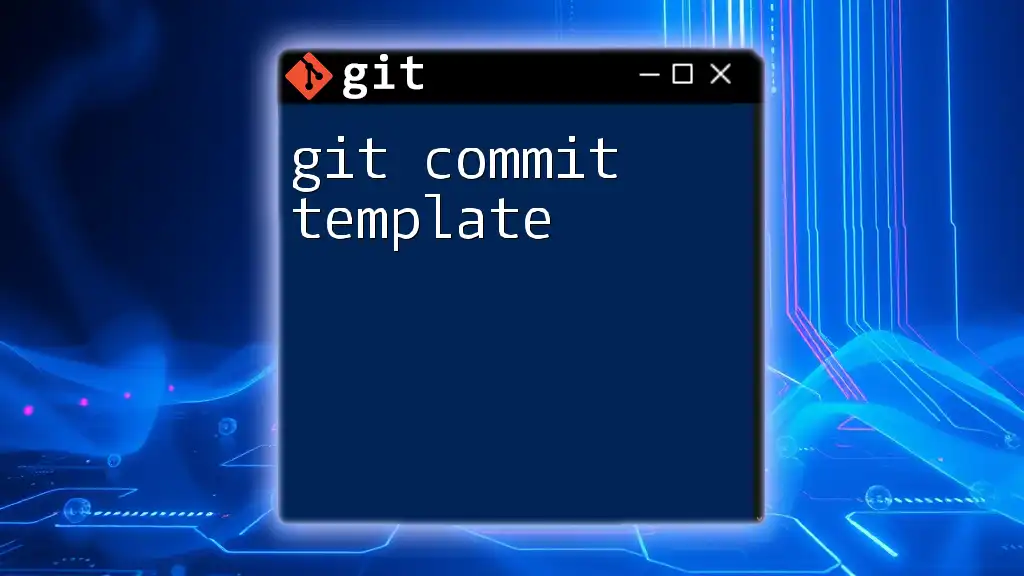
When to Commit: Timing and Frequency
The Right Moments to Commit
Identifying the ideal moments to commit is essential. Commits should be made after completing a task, fixing a bug, or implementing a feature. This ensures that each commit represents a distinct change or accomplishment.
Avoiding Over-committing
While it’s crucial to commit regularly, over-committing can clutter your history with trivial changes. Aim for meaningful commits rather than committing for the sake of committing. This balance maintains clarity in your project’s history.
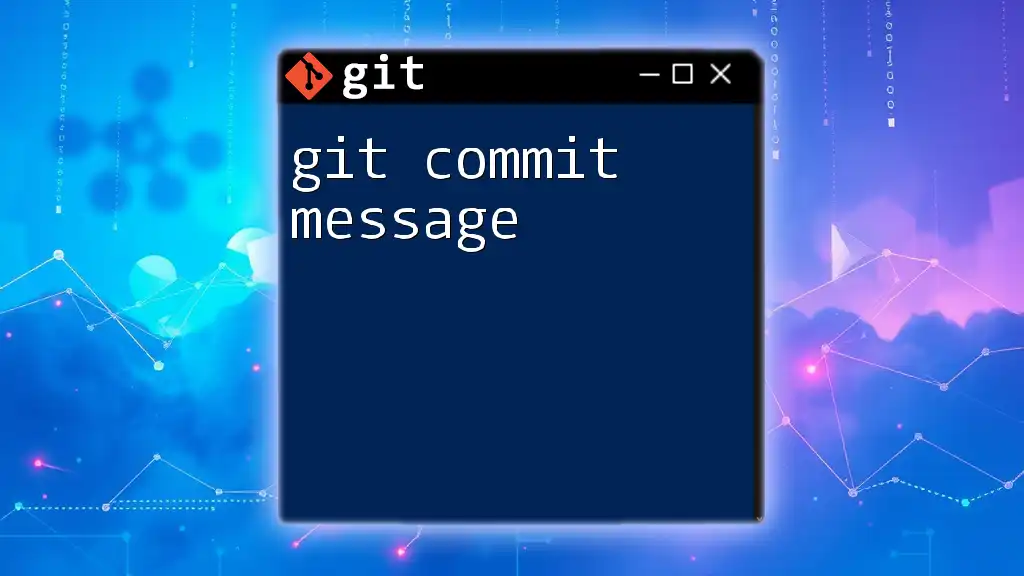
Utilizing Git Commit Hooks
What Are Commit Hooks?
Commit hooks are scripts that Git executes before or after events such as commits or merges. They allow developers to enforce certain rules or checks, improving code quality and consistency.
Setting Up Hooks
A common use for commit hooks is to enforce style guidelines or prevent erroneous commits.
Example: Pre-commit Hook
#!/bin/sh
# Check for trailing whitespace
if git diff-index --check --cached HEAD --; then
echo "Commit contains trailing whitespace!"
exit 1
fi
This script checks for any trailing whitespace in the committed files, helping to maintain a clean codebase.
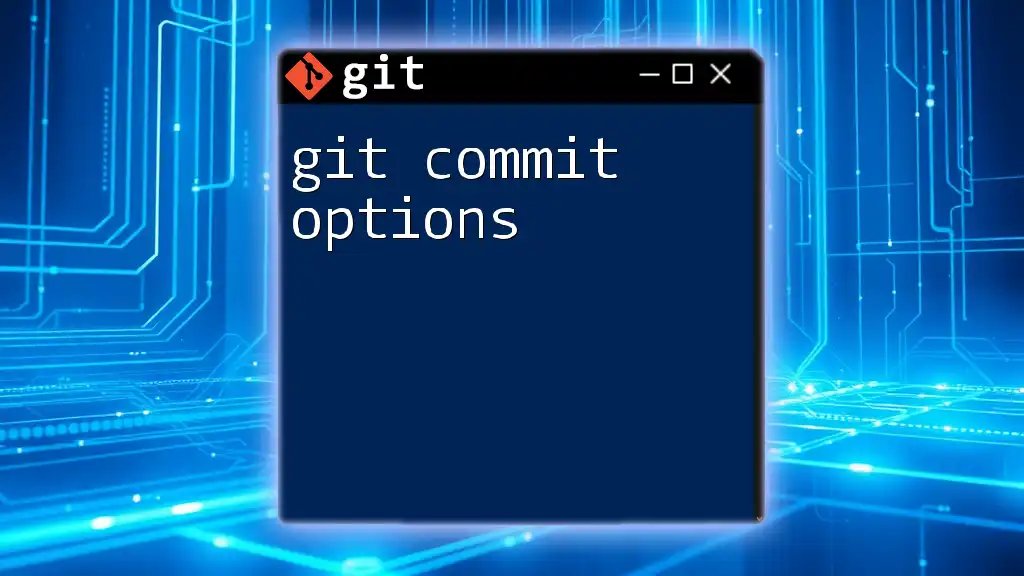
Tools to Enhance Commit Workflow
Git GUIs and Integrated Development Environments (IDEs)
Utilizing Git GUIs and IDEs such as GitKraken, Sourcetree, or Visual Studio Code can simplify the committing process. These tools often provide visual representations of branches, changes, and commit histories, making it easier to manage your repository.
Linting and Formatting Tools
Incorporate tools like ESLint or Prettier to maintain code quality before committing. These tools ensure that code adheres to defined standards and formatting rules, thereby reducing the likelihood of style-related issues during code reviews.
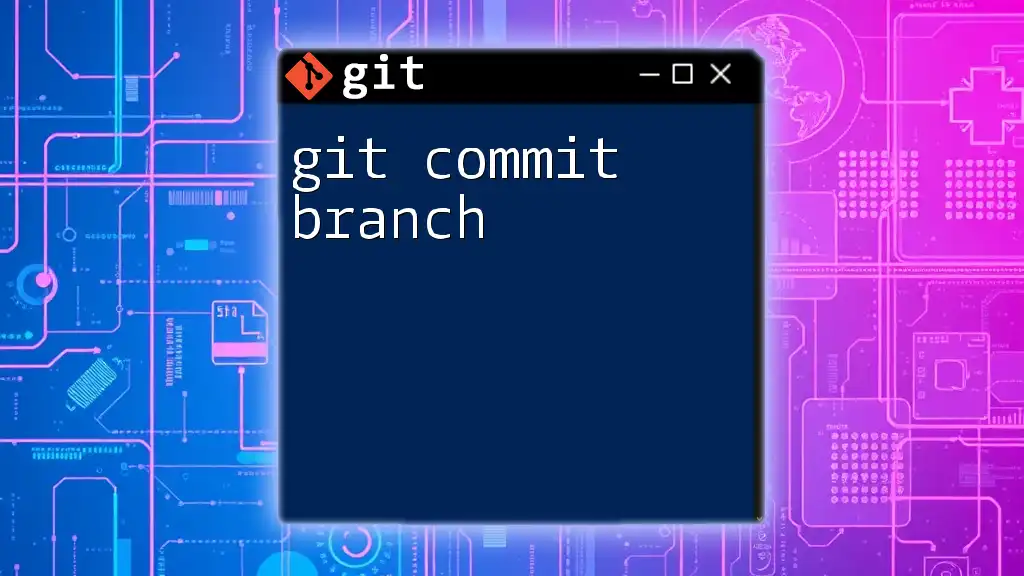
Effectively Using Amend and Squash
Using git commit --amend
When you need to make minor adjustments to the last commit, utilize the `git commit --amend` command. This allows you to modify the previous commit without cluttering your history with additional commits for minor changes.
Squashing Commits for a Clean History
Squashing commits during a rebase can clean up your commit history. This process consolidates multiple commits into a single cohesive commit that captures the essence of the changes made.
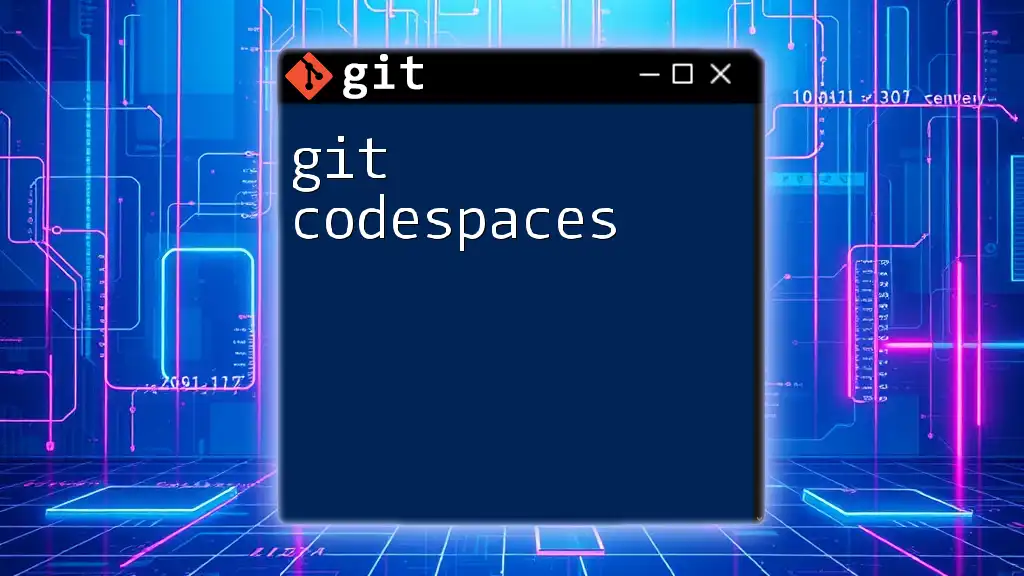
Conclusion
In summary, adhering to git commit best practices not only enhances the quality of your codebase but also improves teamwork and collaboration. By focusing on clear commit messages, keeping commits small, utilizing hooks, and leveraging tools, you will foster a cleaner and more efficient development process.
Adopting these practices will ultimately lead to a more manageable and understandable project, making it easier for both current collaborators and future contributors to engage with your code.
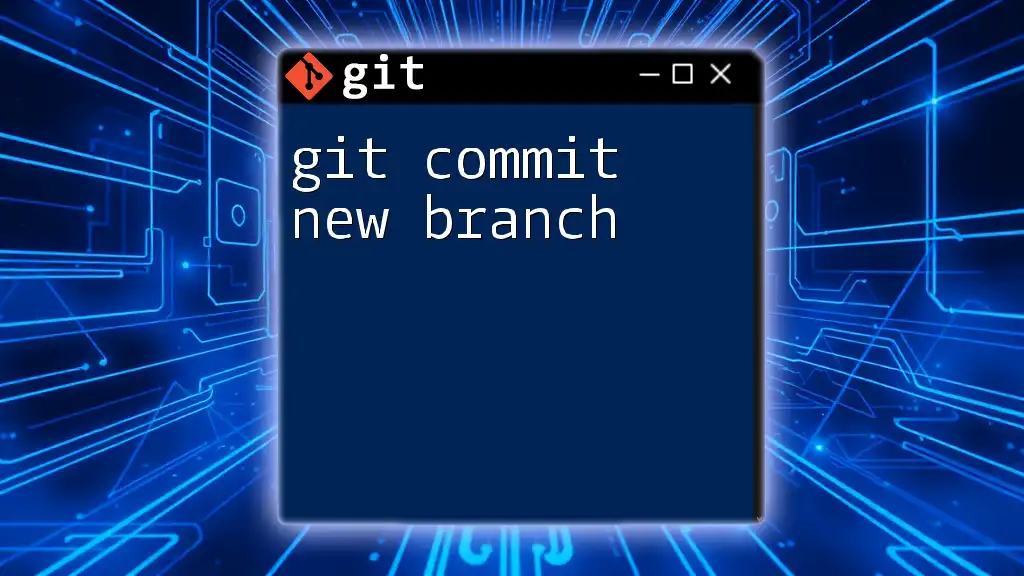
Further Resources
To bolster your understanding, consider exploring books focused on Git, along with documentation and articles that delve deeper into these best practices. Keep an eye out for tools and plugins that can enhance your Git experience, making it as streamlined as possible.