"Git practice involves regularly using Git commands to reinforce your understanding and mastery of version control in development workflows."
Here’s a basic code snippet to get you started with a few essential Git commands:
# Initialize a new Git repository
git init
# Check the status of your repository
git status
# Add files to the staging area
git add .
# Commit changes with a message
git commit -m "Initial commit"
# Push changes to a remote repository
git push origin main
Understanding Git Basics
What is Git?
Git is a powerful version control system designed to track changes in source code during software development. Unlike other version control systems, Git allows multiple developers to work on a project simultaneously without overwriting each other's changes, making it essential for collaborative projects.
Key Concepts in Git
Repository: A repository (or repo) is where your project files and the entire version history are stored. There are two main types of repositories: local and remote. A local repository is stored on your computer, while a remote repository is hosted on platforms like GitHub or GitLab.
Commit: A commit is a snapshot of your files at a specific point in time. Every time you commit, you create a new version of your project, allowing you to track progress and changes over time.
Branching: Branching allows you to create separate lines of development within a project. Each branch can evolve independently, making it easier to work on new features or fixes without affecting the main codebase.
Merge: Merging is the process of combining changes from different branches. Once you've completed work on a feature branch, you typically merge it back into the main branch (usually called `main` or `master`).
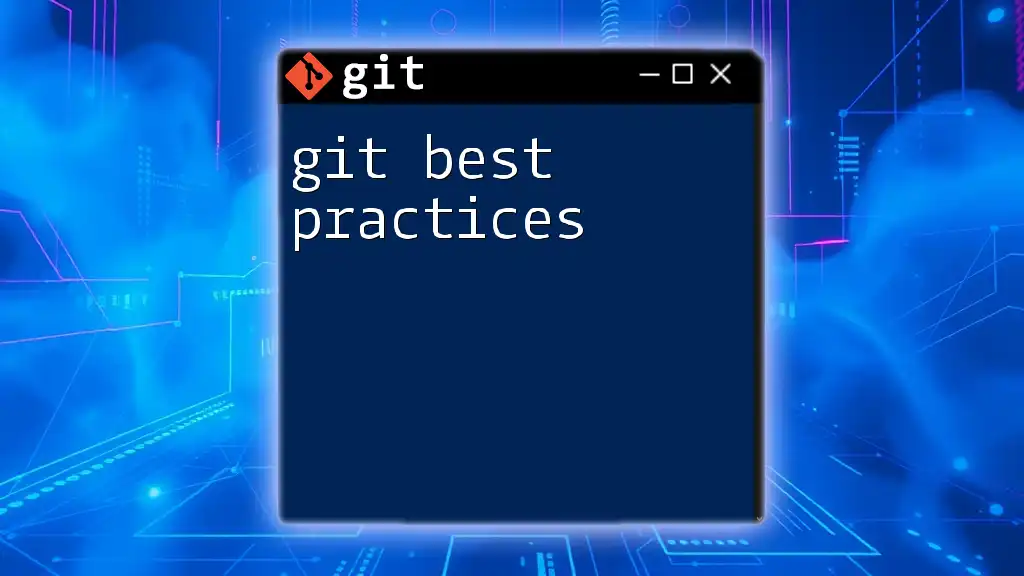
Setting Up Your Git Environment
Installing Git
Git is available for multiple operating systems. Here's how to install it on the most common platforms:
Windows: To install Git on Windows, you can download the installer from the official Git website. Follow the prompts to complete the installation.
macOS: If you're using macOS, the easiest way to install Git is through Homebrew. First, open your terminal and run the following command to install Git:
brew install git
Linux: On Linux systems, Git can typically be installed using your package manager. For example, on Debian-based systems like Ubuntu, you can run:
sudo apt-get install git
Configuring Git
Once Git is installed, you need to configure your username and email. This information will appear in your commits. Use the following commands to set them:
git config --global user.name "Your Name"
git config --global user.email "youremail@example.com"
You can verify your configuration by running:
git config --list
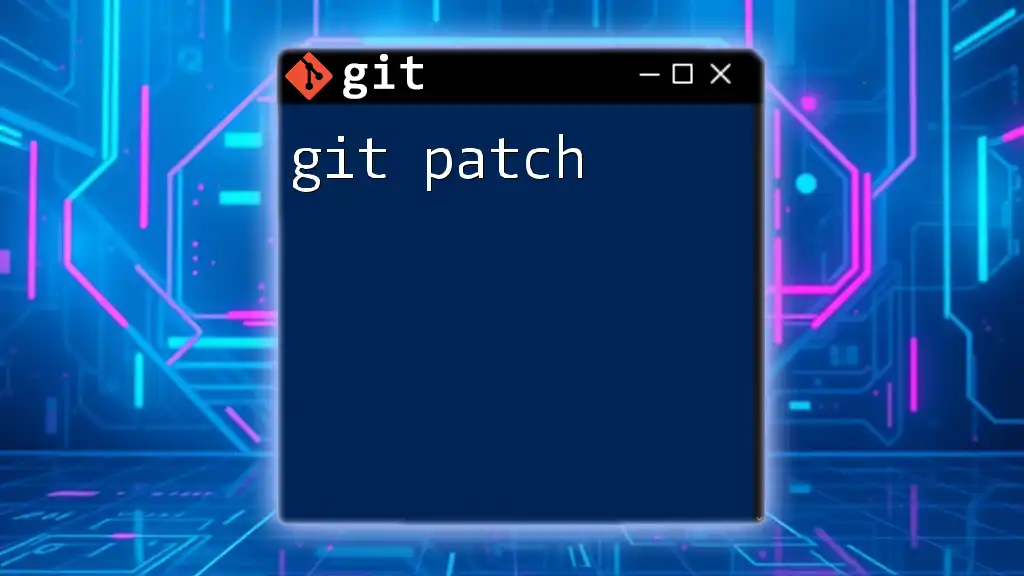
Core Git Commands for Practice
Initializing a Repository
You can start a new Git repository in two primary ways:
Creating a New Repository: To create a new repository, navigate to your desired directory in the terminal and run:
git init my-repo
Cloning an Existing Repository: If you want to work on a project that already exists, you can clone it using:
git clone https://github.com/user/repo.git
Staging and Committing Changes
Staging Files
To stage files (preparing them for a commit), you can use the `git add` command:
git add filename.txt
git add . # This stages all changes in the current directory
Committing Changes
When you're ready to save your staged changes, you commit them with:
git commit -m "Your commit message"
If you need to modify the last commit, use the `--amend` flag:
git commit --amend
Branching and Merging
Creating and Switching Branches
To create a new branch, run:
git branch new-feature
git checkout new-feature
Alternatively, you can create and switch branches in one command:
git checkout -b new-feature
Merging Branches
When you’re ready to merge your changes back to the main branch, first switch to the main branch:
git checkout main
Then merge your feature branch:
git merge new-feature
Viewing Changes
Checking Status of Working Directory
To see the current status of your working directory (which files are staged, unstaged, or untracked), use:
git status
Viewing Commit History
You can review your commit history with:
git log --oneline --graph
This command shows a simplified, visual representation of your commit history.
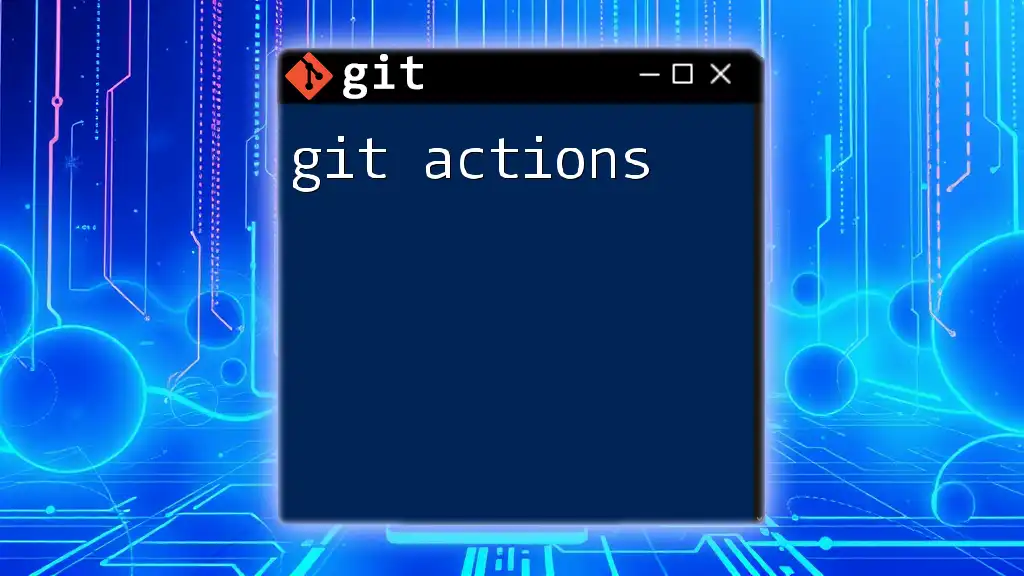
Advanced Git Commands to Master
Undoing Changes
Reverting Commits
If you need to undo a commit, you can use the `git revert` command:
git revert commit_id
This command creates a new commit that undoes the changes made in the specified commit.
Resetting Changes
Git also provides the ability to reset changes, which can be categorized as soft, mixed, or hard resets:
- Soft Reset: Keeps your changes in the staging area.
git reset --soft commit_id
- Mixed Reset: Unstages changes but keeps them in the working directory.
git reset --mixed commit_id
- Hard Reset: Discards all changes and sets the working directory to the specified commit.
git reset --hard commit_id
Collaboration and Remote Repositories
Pushing Changes
To share your local changes with the remote repository, push your commits using:
git push origin main
Pulling Changes
To update your local repository with the latest changes from the remote repository, use:
git pull origin main
Resolving Merge Conflicts
When merging, you might encounter conflicts if two branches have changed the same line. It’s important to resolve these conflicts manually.
Understanding Merge Conflicts: They occur when Git cannot automatically merge changes. You’ll see markers in the affected files indicating where conflicts arise.
Steps to Resolve Conflicts: After resolving conflicts in the files, stage the resolved changes:
git add resolved_file.txt
Then commit the resolution:
git commit -m "Resolved merge conflict"
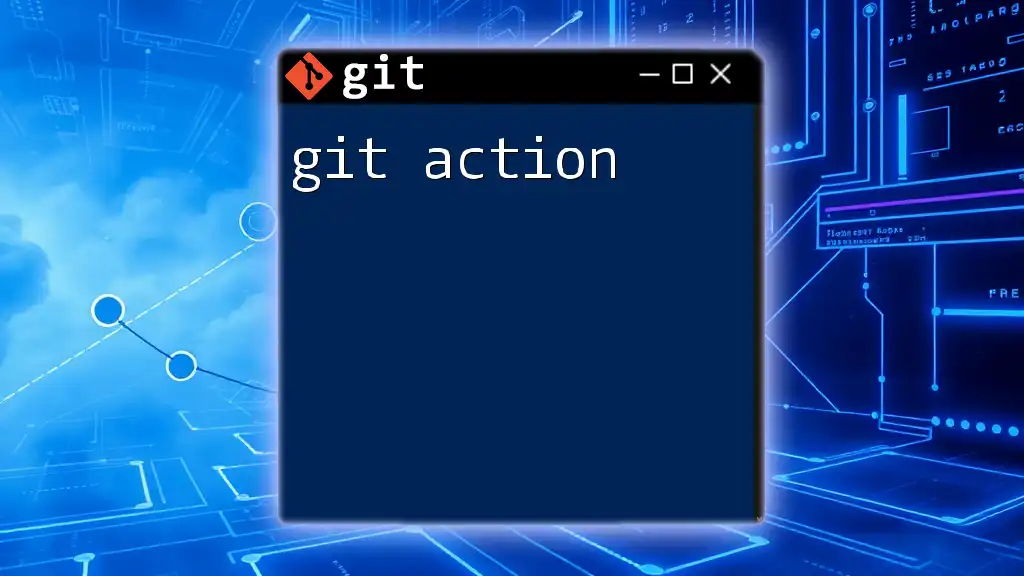
Best Practices for Practicing Git
Regular Commit Practices
Aim to commit often and with meaningful messages. Each commit should represent a logical unit of work, making it easier to understand the project's history.
Collaborating Effectively
Effective collaboration involves communication with team members. Using pull requests not only allows for code review but also keeps everyone on the same page regarding changes.
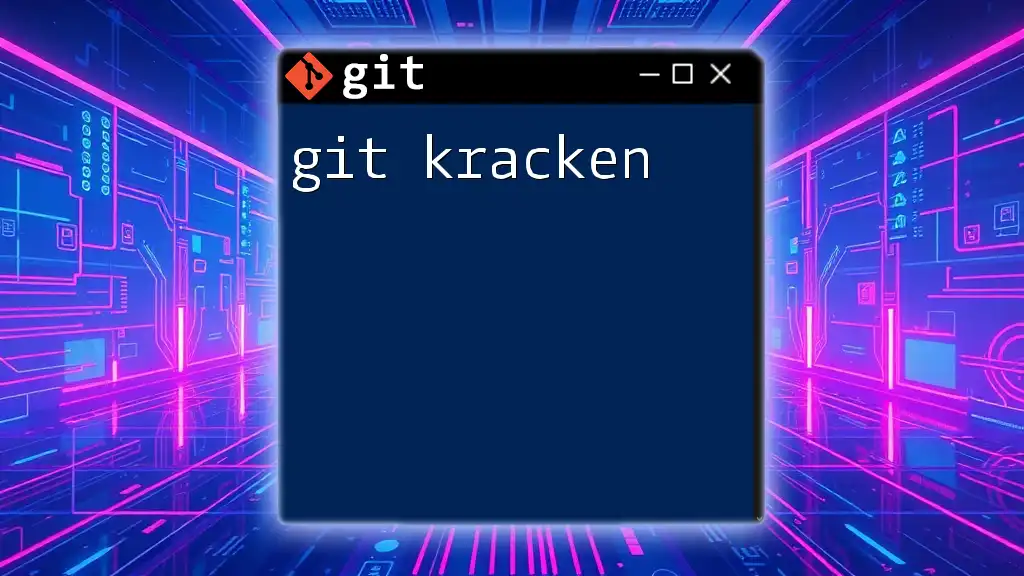
Conclusion
In conclusion, mastering Git requires time and consistent git practice. The commands and practices outlined in this guide will provide a strong foundation to efficiently use Git in your projects. Remember to regularly commit your work, collaborate effectively, and always practice resolving conflicts to enhance your skill set.
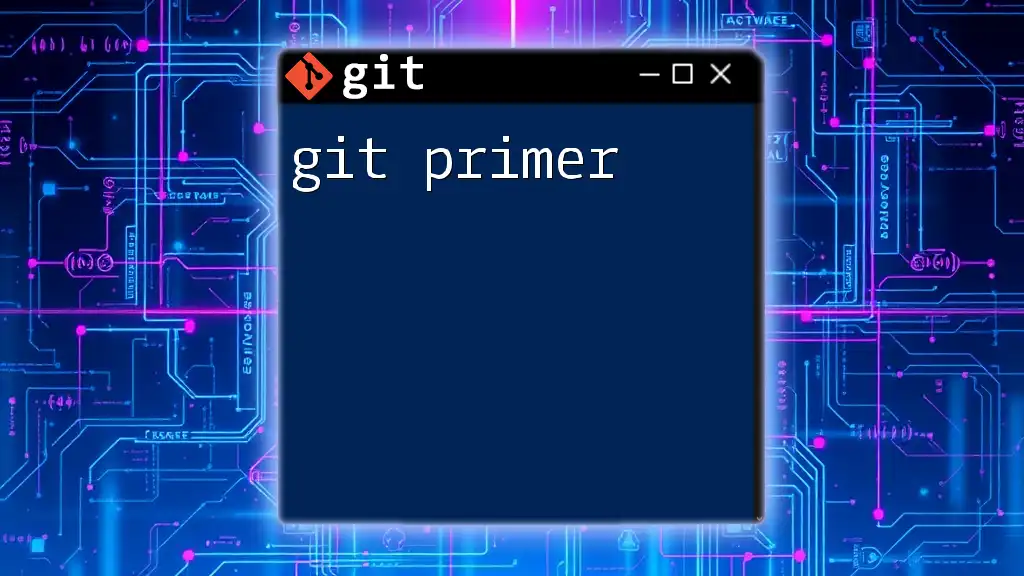
Additional Resources
For those looking to deepen their understanding of Git, consider exploring recommended books, online courses, and valuable tools. The official Git documentation is also a great resource, offering detailed explanations and examples of all Git functions.
Call to Action
Start your Git practice journey today and take your software development skills to the next level!