Git Actions are automated workflows that enable you to streamline tasks in your Git repository directly on GitHub, using straightforward YAML configurations to define specific commands or scripts to run in response to various events.
Here's a simple example of a GitHub Action configuration:
name: CI
on: [push]
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Set up Node.js
uses: actions/setup-node@v2
with:
node-version: '14'
- name: Install dependencies
run: npm install
- name: Run tests
run: npm test
What Are Git Actions?
Git Actions refer to GitHub Actions, a powerful automation tool that allows developers to create custom workflows for software development processes directly within GitHub repositories. This feature enables Continuous Integration (CI) and Continuous Deployment (CD), streamlining various tasks, such as testing, building, and deploying applications.
By using Git Actions, developers can automate mundane tasks and ensure that their applications maintain high standards of quality and efficiency. The automation not only saves time but also reduces the likelihood of human error during repetitive tasks.
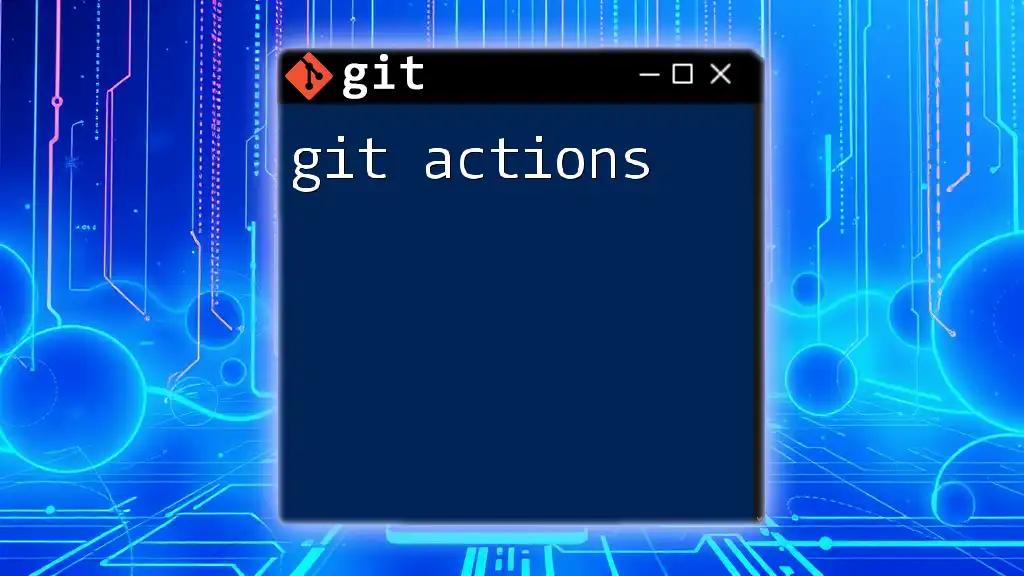
Importance of Git Actions in Modern Development
In the rapidly evolving landscape of software development, efficiency and speed are crucial. Here are key reasons for utilizing Git Actions:
-
Streamlined Processes: Git Actions significantly enhance workflow efficiency by automating routine tasks. This streamlining frees up developers to focus on more critical aspects of development, such as writing code and improving user experience.
-
Integration: Git Actions integrate seamlessly with other tools and services, allowing for a flexible and adaptable workflow. Developers can connect their GitHub repository with CI/CD tools, notifications, and various third-party services, enhancing collaboration and delivering code faster.
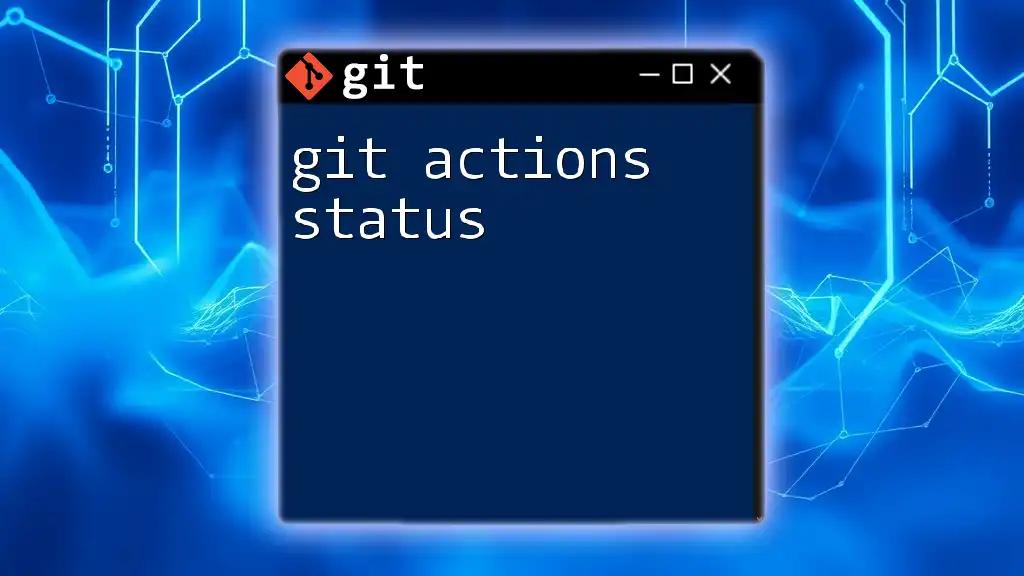
Setting Up Git Actions
Prerequisites
Before diving into Git Actions, ensure you have:
- An active GitHub account to create and manage repositories.
- A basic understanding of Git commands, as this knowledge is essential for crafting workflows effectively.
Creating Your First Workflow
To harness the power of Git Actions, creating a workflow file is essential. This can be done by following these steps:
Steps to Create a Workflow File
- Navigate to your GitHub repository.
- Create a new directory named `.github/workflows`.
- Inside this directory, create a new YAML file (e.g., `ci.yml`).
Here’s an example structure of a simple workflow file:
name: CI
on: [push]
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v2
In this snippet:
- The workflow triggers on every push event.
- It has a single job named build that runs on the latest Ubuntu environment.
- The first step checks out the repository’s code so subsequent steps can act on it.
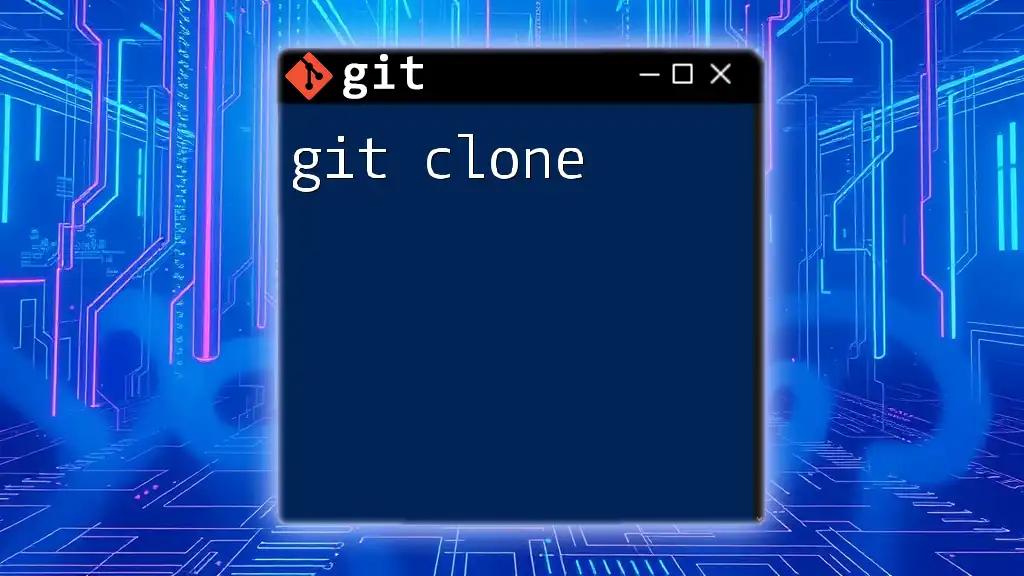
Understanding the Workflow YAML File
Basics of YAML Syntax
Understanding YAML (YAML Ain't Markup Language) syntax is crucial for working effectively with workflow files. YAML is a human-readable data serialization standard that is widely used for configuration files.
Some common YAML elements include:
- Key-Value Pairs: These define specific attributes.
- Indentation: Proper indentation is necessary to define structure and hierarchy.
Components of a Workflow File
Workflows
The first element in a workflow file is the workflows section, where you define the name and settings of your workflow.
Triggers
Triggers determine when your workflow should run. Common triggers include:
- `on: push`: This trigger initiates the workflow whenever code is pushed to the repository.
- `on: pull_request`: This starts the workflow when a pull request is opened, modified, or closed.
Jobs and Steps
A workflow consists of one or more jobs, which run in parallel or sequentially. Each job contains steps where individual commands run.
Here’s an example of how to define jobs and steps:
jobs:
build:
steps:
- name: Install Dependencies
run: npm install
In this snippet:
- The job named build executes a step to install necessary dependencies using the `npm install` command.
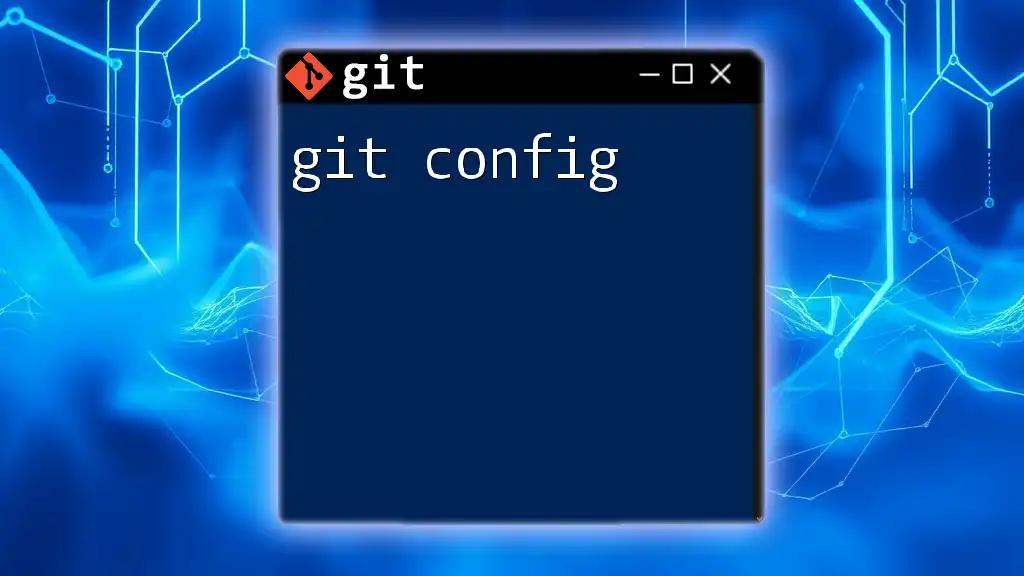
Using GitHub Actions Marketplace
What is the GitHub Actions Marketplace?
The GitHub Actions Marketplace is a repository of community-contributed actions that can be leveraged to enhance your workflows. Instead of building everything from scratch, developers can explore a vast array of pre-built actions that cover a variety of common use cases.
How to Create Custom Actions
While many tasks can be accomplished using pre-built actions, there may be times when a custom action is necessary.
Building Custom Actions
You can create actions in two main ways:
- JavaScript Actions: Ideal for actions that require Node.js runtime.
- Docker Actions: Useful for encapsulating more complex dependencies.
Here’s a brief example of creating a simple JavaScript action:
const core = require('@actions/core');
try {
const name = core.getInput('name');
console.log(`Hello ${name}`);
} catch (error) {
core.setFailed(error.message);
}
This JavaScript action retrieves an input from the workflow and prints a greeting to the console.
Sharing Your Action
Once your custom action is developed, it can be published to the GitHub Actions Marketplace. This involves creating a new repository for your action and providing a `README` file that explains how to use it, along with necessary configurations.
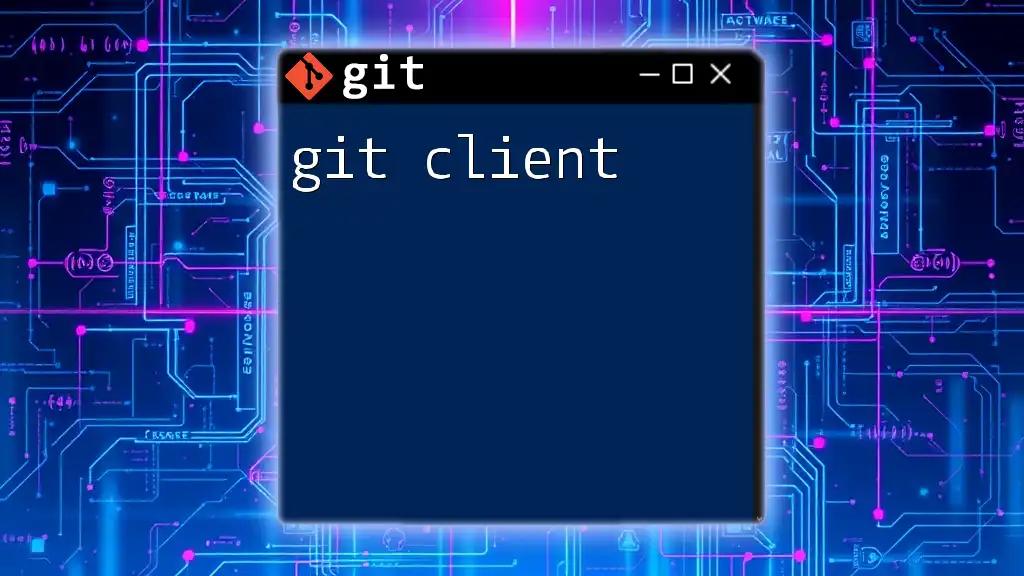
Testing and Debugging Workflows
Common Errors and Solutions
Working with Git Actions can lead to various errors. Understanding these errors is crucial for a smooth development experience. Common issues may include:
- Incorrect syntax in YAML files.
- Missing permissions for certain actions.
Using the Logs
When workflows fail or don’t perform as expected, logs provide valuable insights. You can access workflow logs directly from the GitHub interface:
- Navigate to the Actions tab of your repository.
- Click on the failed workflow run to view detailed logs.
Logs include each step's output and can help identify the root cause of issues.
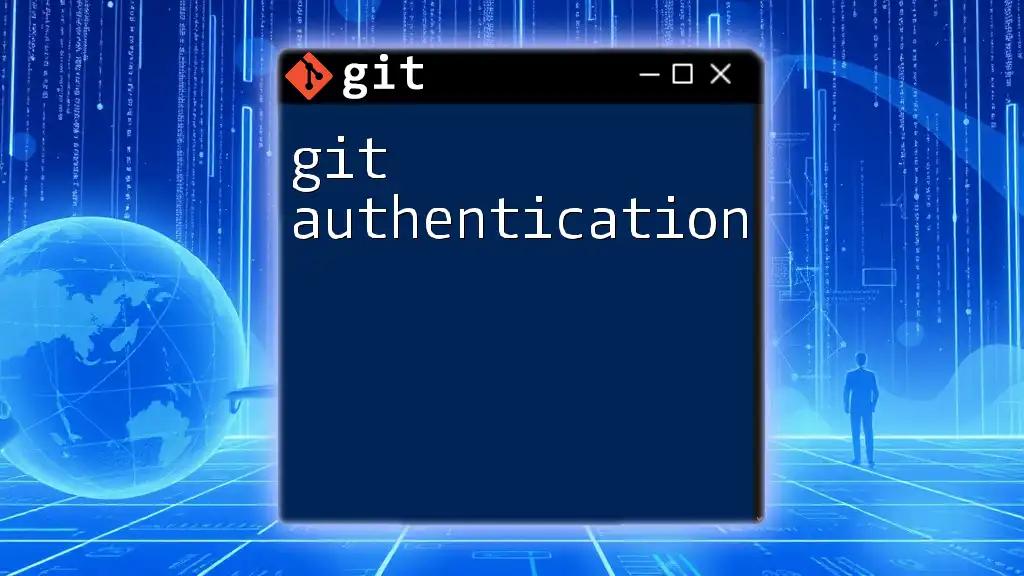
Best Practices for Git Actions
Organizing Workflow Files
Maintaining an organized structure for your workflows aids in scalability and collaboration. Following naming conventions and practicing good organization can significantly enhance readability.
An example of an organized directory structure might look like this:
.github/
workflows/
ci.yml
deploy.yml
Version Control for Workflows
Keeping your workflows updated is essential for maintaining functionality and adapting to changes in your development process. Implementing semantic versioning can help keep track of changes and updates to your actions efficiently.
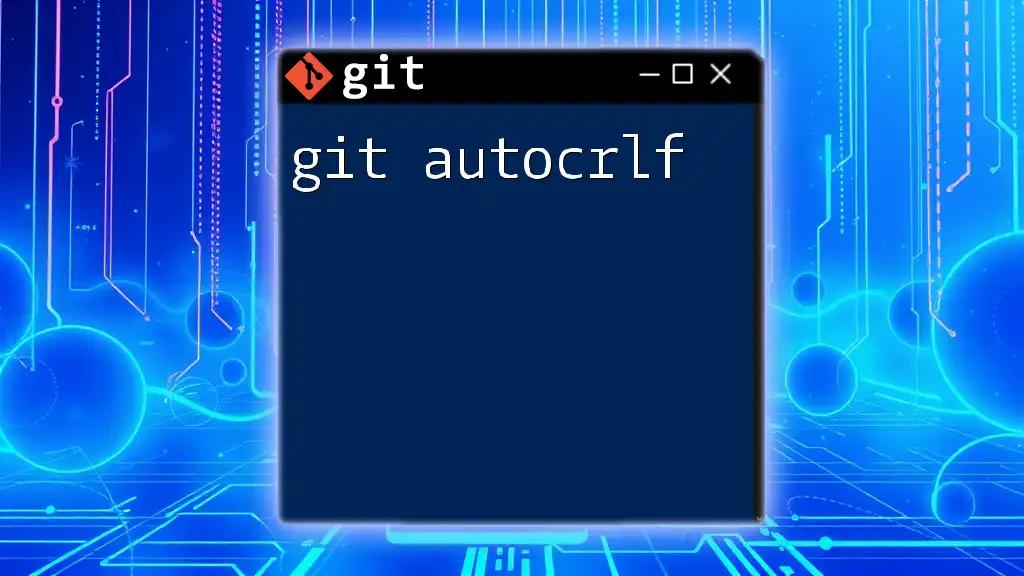
Conclusion
In summary, Git Actions emerge as an invaluable tool in the software development process, enhancing efficiency, improving collaboration, and automating crucial tasks. By understanding their components, learning how to create and utilize actions, and adhering to best practices, developers can leverage Git Actions to transform their development workflows.
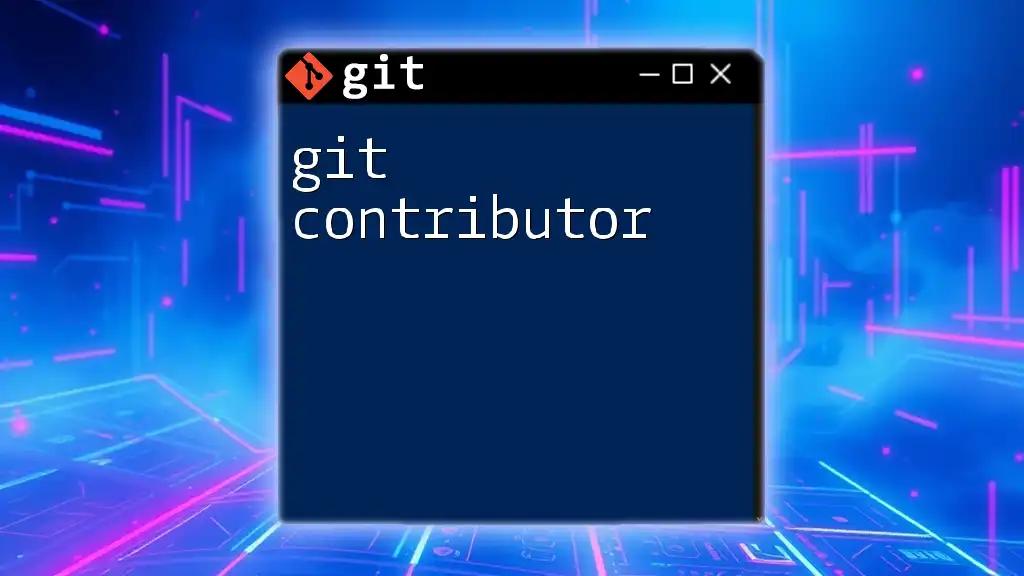
Next Steps
Now that you have a foundational grasp of Git Actions, it's time to experiment with different workflows. Try automating repetitive tasks in your projects or explore creating custom actions that cater to your specific needs.
Additional Resources
For further learning, we recommend exploring the official GitHub documentation on Git Actions, engaging with community forums, and experimenting with tutorials that deepen your understanding of CI/CD practices. By diving deeper into Git Actions, you can significantly boost your efficiency and effectiveness as a developer.