"Git authentication failed" typically occurs when your credentials are incorrect or missing, preventing access to a remote repository.
git clone https://github.com/username/repository.git
# If prompted for a username and password, ensure they are correct or use a personal access token.
Understanding Git Authentication
What is Git Authentication?
Git is a widely used version control system that allows developers to manage and track changes to their code repositories. One vital aspect of using Git is authentication, which ensures that only authorized users can access and change a repository's contents. Proper authentication processes help protect sensitive code from unauthorized access and maintain project integrity.
Common Authentication Methods
HTTPS Authentication
When using HTTPS to clone or push to repositories, authentication typically requires a username and password. However, since 2021, many platforms, including GitHub, have shifted to using Personal Access Tokens (PATs) as a more secure alternative. These tokens serve as a substitute for passwords, providing a way to specify granular permissions.
SSH Authentication
SSH (Secure Shell) is another method used for Git authentication. It relies on a pair of keys—public and private—to establish a secure connection. The private key remains on the user’s machine, while the public key is added to the user’s profile on the Git service (like GitHub). SSH is generally preferred over HTTPS due to its enhanced security and the ability to authenticate without entering credentials repeatedly.
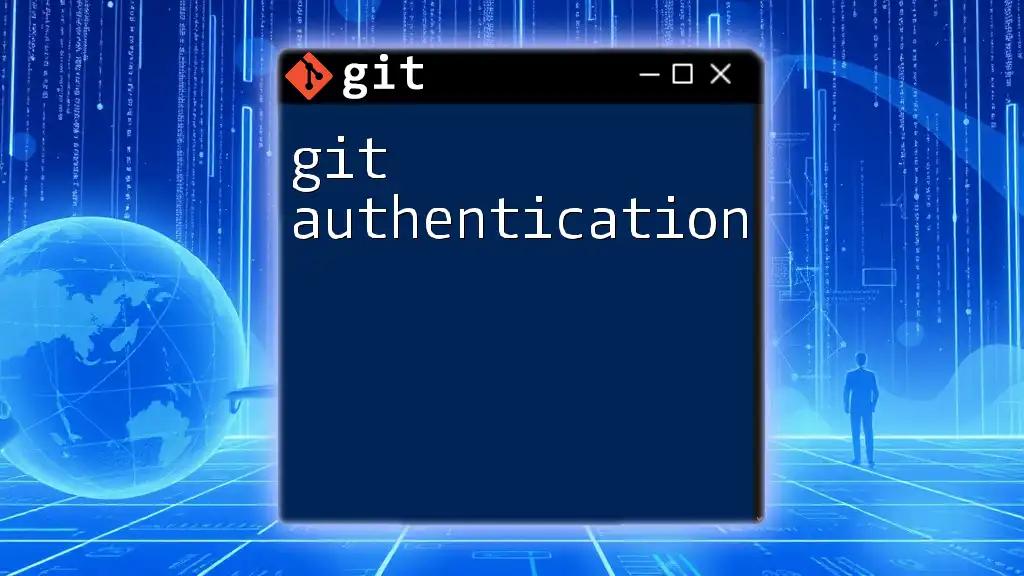
Causes of Authentication Failed Errors
Incorrect Credentials
One of the most common reasons for a git authentication failed error is the use of incorrect credentials. This could be an outright mistake in entering your username or password. An example error message you might encounter is:
remote: Invalid username or password.
fatal: Authentication failed for 'https://github.com/username/repo.git/'
Expired or Revoked Access Tokens
Another frequent cause of authentication issues is using an expired or revoked Personal Access Token (PAT). PATs are time-sensitive; if they are not renewed or if they've had their access revoked by the user or a system administrator, attempts to push or pull changes will result in authentication errors.
SSH Key Issues
When using SSH, misconfigured or missing SSH keys can lead to authentication failures. The most common error message in this scenario is:
Permission denied (publickey).
fatal: Could not read from remote repository.
This typically indicates that either the SSH key has not been created, or it has not been added to the SSH agent or the Git service (e.g., GitHub).
Network Issues
Sometimes, network issues can also contribute to authentication failures. Firewalls, proxy settings, or network configurations may interfere with the connection to the remote repository, resulting in a failure to authenticate.
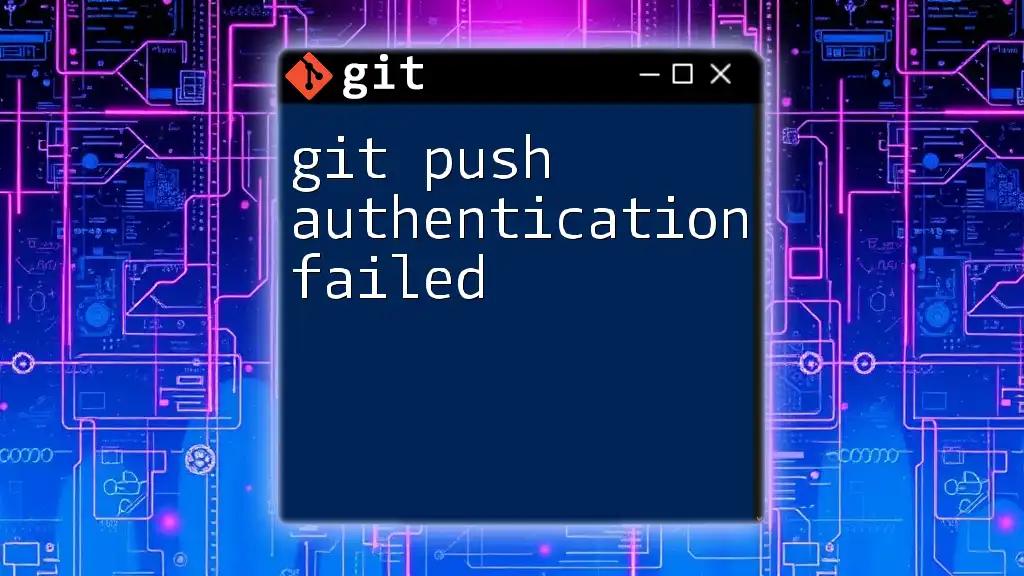
Troubleshooting Git Authentication Errors
Step-by-Step Guide for HTTPS Issues
Checking Credentials
Start by verifying your credentials. If you are using HTTPS for cloning or pushing, double-check that your username and password (or PAT) are correct. If you enter the wrong password or token, you will encounter the authentication failure message mentioned earlier. To clone a repository, you might use:
git clone https://github.com/username/repo.git
If you need to update existing credentials, consider re-cloning or changing the remote URL.
Using Personal Access Tokens
If you're still facing issues, consider creating a new Personal Access Token. This can be done in your Git service profile settings. Once generated, update your remote URL to incorporate the new PAT. For example:
git remote set-url origin https://<username>:<PAT>@github.com/username/repo.git
Remember to replace `<username>` and `<PAT>` with your actual username and the generated token.
Solutions for SSH Issues
Testing SSH Connection
If you're using SSH, the first step is to test if your SSH key is correctly configured. You can check this by running the following command:
ssh -T git@github.com
If your connection is established successfully, you'll receive a welcome message. If there's a problem with the key, this command will produce an error.
Generating a New SSH Key
If there is an issue with your SSH key, you might have to generate a new key. To do this, run:
ssh-keygen -t rsa -b 4096 -C "your_email@example.com"
This command creates a new SSH key using your email as a label.
Adding SSH Key to SSH Agent
Once the SSH key is generated, ensure it's added to the SSH agent. Run the following commands:
eval "$(ssh-agent -s)"
ssh-add ~/.ssh/id_rsa
This sets up the SSH agent and adds your private key for authentication.
Adding SSH Key to GitHub
After you generate an SSH key, you need to add the public key to your GitHub account. This is done in the SSH and GPG keys settings under your account profile. Copy the content of your public key (usually located in `~/.ssh/id_rsa.pub`) into the GitHub settings.
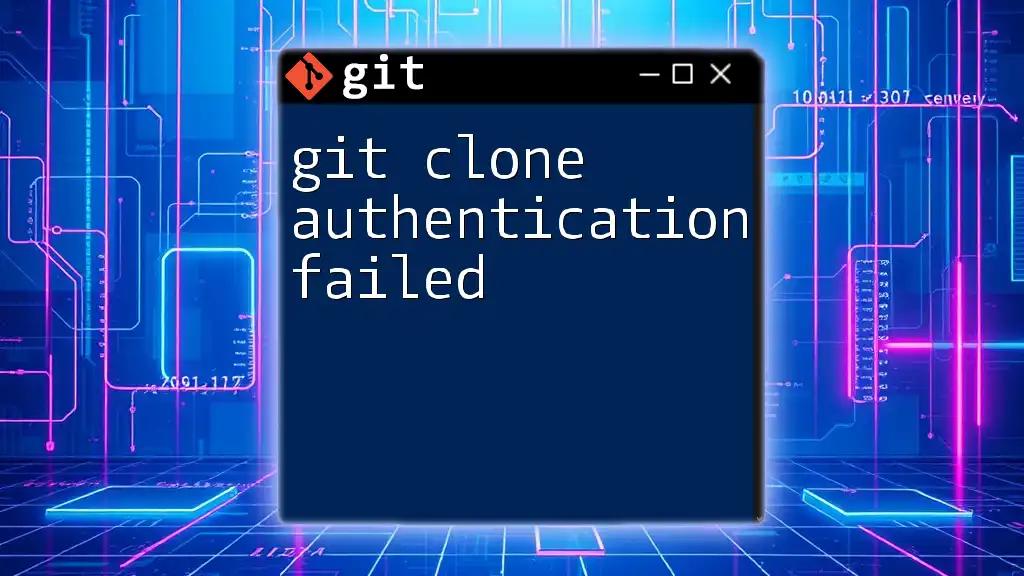
Preventing Future Authentication Issues
Best Practices for Credential Management
To prevent future git authentication failed errors, managing credentials effectively is crucial. Utilizing credential managers like the Git Credential Manager can automate the process of storing your credentials securely. Avoid hardcoding tokens or passwords in code.
Regularly Updating Tokens and Keys
Regularly monitor your PATs and SSH keys. Tokens should be rotated periodically, and old, unused tokens should be revoked promptly. This helps maintain a higher level of security.
Diagnosing Authentication Problems Early
Be proactive about diagnosing potential authentication problems. Familiarize yourself with tools and commands that can help you monitor SSH or HTTPS connections, such as checking your authentication status or viewing stored credentials.
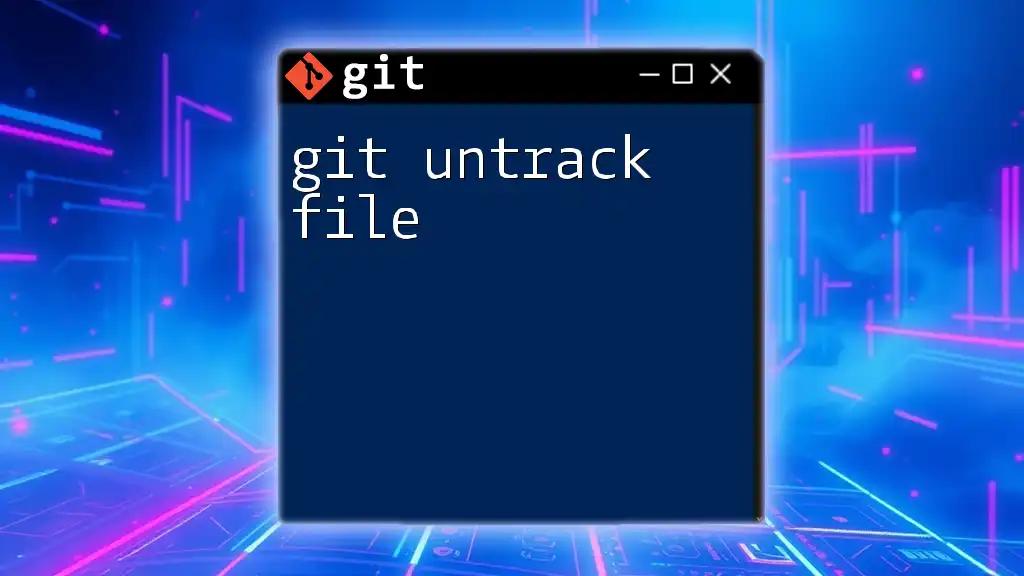
Conclusion
In summary, understanding the common causes of git authentication failed errors and how to troubleshoot them can significantly improve your workflow with Git. Whether facing credential issues, token expirations, or SSH key misconfigurations, following the outlined steps will help you regain access quickly. Embrace continuous learning with Git, and don't hesitate to seek additional resources for deeper insights and strategies. Happy coding!