The "git push authentication failed" error typically occurs when your credentials are incorrect or not recognized by the remote repository, often due to a missing or misconfigured SSH key or credentials manager.
Here's a simple command to verify your current remote URL configuration in case it needs to be updated:
git remote -v
Understanding Git Push Authentication
What is Git Push?
The `git push` command is a way to upload your local changes to a remote repository. When you make changes to your code locally—whether by adding new files, modifying existing ones, or creating additional commits—you use `git push` to sync these changes with the remote repository (like GitHub, GitLab, or Bitbucket). It's essential because it allows your collaborators to access your latest updates and ensures that your work is backed up.
Why Authentication Matters in Git
Authentication in Git is crucial because it secures access to your repositories. When you perform actions like `git push`, Git verifies your identity to ensure that you have permission to modify the repository. This prevents unauthorized access and helps maintain the integrity of the codebase.
Different types of authentication methods are commonly used:
- SSH Keys: A secure way to authenticate without having to enter a password every time.
- HTTPS: Requires a username and password for accessing the repository, which can be managed through various credential helpers.
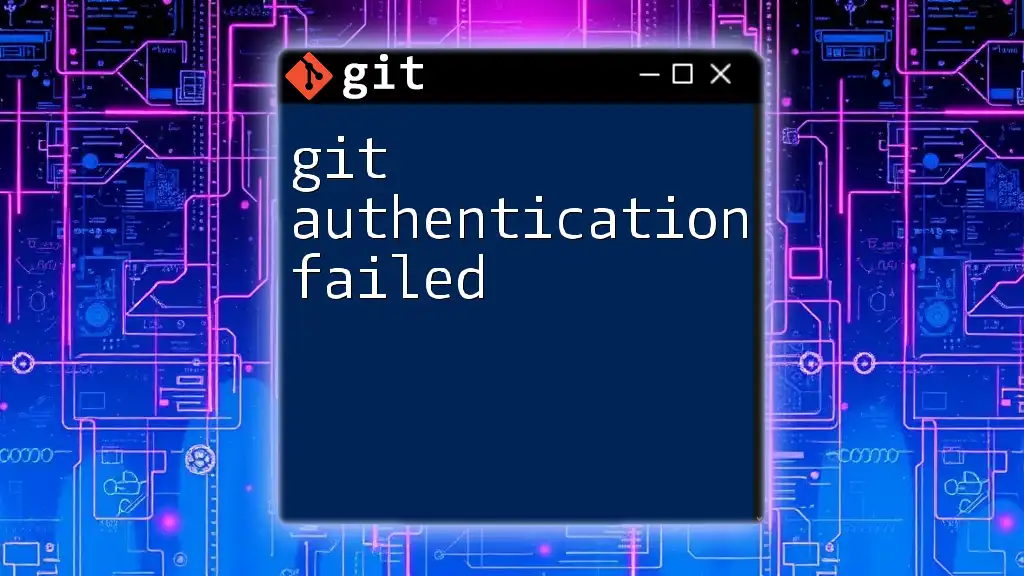
Common Causes of Git Push Authentication Failed Errors
Incorrect Credentials
One of the most prominent reasons for the "git push authentication failed" error is the submission of incorrect credentials. This can happen because of simple mistakes such as typing errors in your username or password. For example, mixing up letters, using the wrong password case, or even being logged into a different account can lead to issues.
SSH Key Issues
SSH keys provide a secure way to access your Git repositories without the need for username/password authentication. If your SSH key is missing or not configured correctly, you will encounter authentication errors.
To resolve this, you may need to generate a new SSH key and add it to your Git provider. You can create one using the following command:
ssh-keygen -t rsa -b 4096 -C "your_email@example.com"
After generating your SSH key, ensure you add it to your SSH agent and upload the public key to your repository settings.
Two-Factor Authentication (2FA) Complications
Two-Factor Authentication is an added layer of security that prompts users for a second form of verification. When enabled, standard password authentication for `git push` commands will not work as it requires an extra verification step.
To overcome this, you should use Personal Access Tokens instead of your regular password. This token acts as a secure key to authenticate your Git operations. Check your Git service provider's documentation for instructions on generating a Personal Access Token.
Repository Permissions
Sometimes, authentication errors can result from insufficient permissions to push to the repository. If you don't have the appropriate access rights, Git will return a "permission denied" error.
It’s critical to verify that you have the necessary permissions on the repository. For instance, on GitHub, you can check your permission settings in the repository's "Settings" tab under "Manage Access."
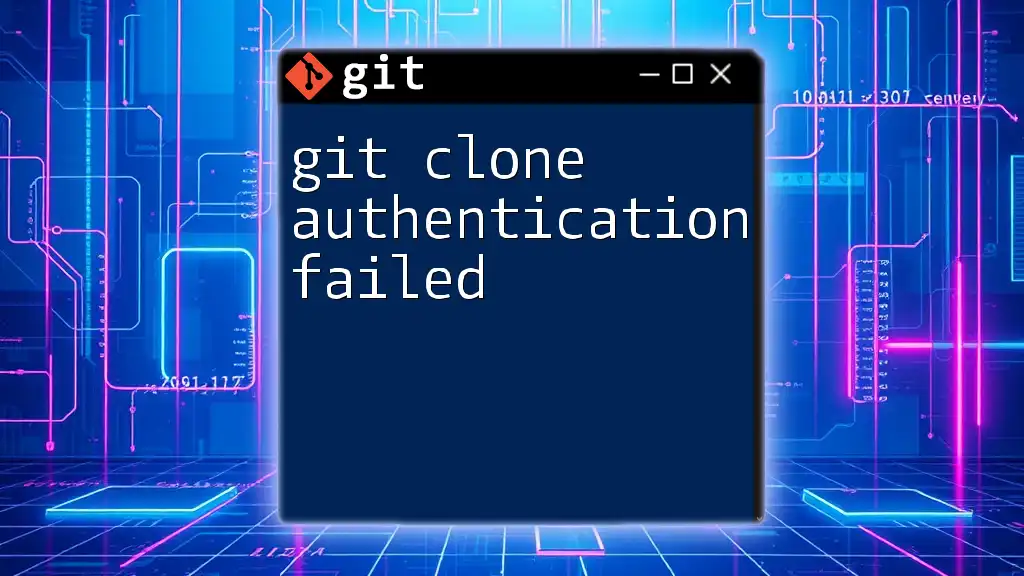
Diagnosing the Issue
Reading the Error Message
When you encounter the "git push authentication failed" error, the first step is to pay close attention to the error message. It often contains specific phrases that can help identify the core issue. Phrases like "permission denied," "bad credentials," or "no matching key" provide valuable clues.
Debugging Tips
For SSH-specific issues, you can get more detailed logs by using:
GIT_SSH_COMMAND='ssh -vvv' git push
This command will enable verbose logging, which can help you understand where the authentication process is failing.
Another key point to diagnose issues is to check your remote URL configuration. You can list your remote repositories by executing:
git remote -v
This command will show you the remotes associated with your local repository and can help ensure you're pushing to the correct URL.
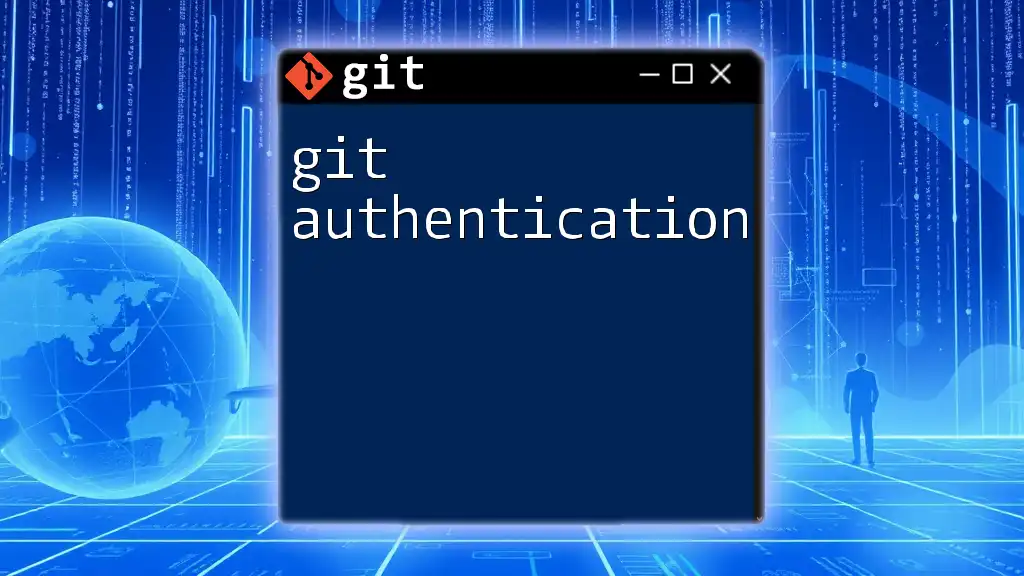
Solutions to Fix Git Push Authentication Issues
Resetting Your Credentials
If you suspect that your saved credentials are incorrect, clearing them might help. Git can cache your credentials, and sometimes this cache needs to be refreshed. You can clear saved credentials with:
git credential reject
This will prompt Git to ask for your credentials again during the next push, allowing you to input them anew.
Updating or Replacing SSH Keys
If you're dealing with SSH key issues, it’s essential to verify your existing keys. If you don't have the right key set up or if it has been changed, you’ll need to replace or add a new one using `ssh-add`:
ssh-add ~/.ssh/id_rsa
This command adds your private SSH key to the SSH agent, ensuring it is used for authentication.
Creating and Using Personal Access Tokens
For HTTPS connections with 2FA enabled, creating and using a Personal Access Token is a necessity. To create a token on platforms like GitHub:
- Go to Settings in your profile.
- Navigate to Developer settings.
- Select Personal access tokens and Generate new token.
- Choose the scopes or permissions you want to provide the token.
Once you’ve created the token, you can use it as your password when performing `git push`. It’s essential to keep this token secure, like a password.
Checking and Modifying Repository Permissions
If you suspect permission issues, navigating to the repository settings on the Git hosting service can provide clarity. Check the "Manage Access" area to confirm your user role and permissions. If you lack the necessary permissions, you might need to:
- Request access from the repository owner.
- Modify your role if applicable.
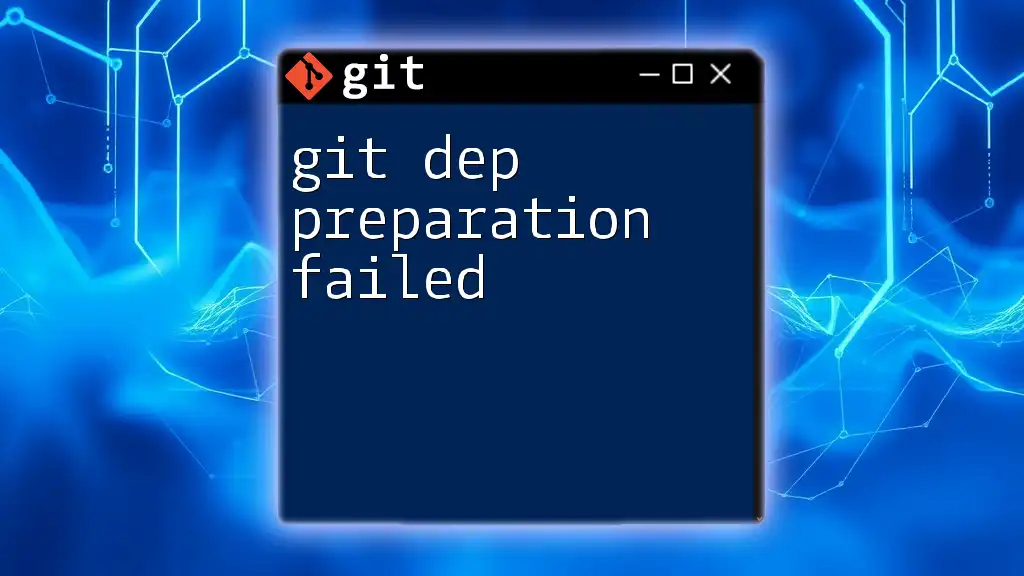
Best Practices for Avoiding Authentication Issues in the Future
Use SSH Instead of HTTPS
Consider adopting SSH authentication for your Git operations. It’s not only more secure but also eliminates the need to enter your username and password frequently. Setting up SSH keys is a one-time process that pays off significantly in convenience.
Keeping Credentials Secure
Securing your passwords and authentication tokens is vital. Utilizing a password manager can help manage your credentials effectively, ensuring you don't lose track of them or use insecure practices when storing them.
Using Git Configuration Settings
Git allows you to manage how your credentials are stored with configuration settings. For instance, you can use the command below to store your credentials securely:
git config --global credential.helper store
This command will enable Git to remember your credentials securely.
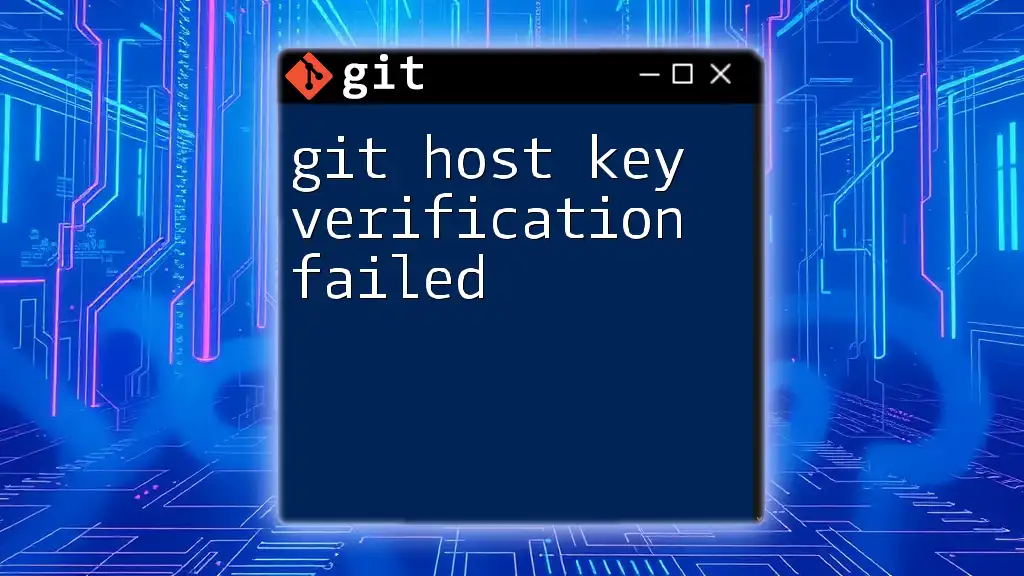
Conclusion
In summary, the "git push authentication failed" error can stem from a variety of issues, including incorrect credentials, SSH key problems, two-factor authentication complications, and even permission issues. By understanding these potential pitfalls and the solutions provided, you can effectively troubleshoot authentication errors and streamline your Git experience. Remember to adopt best practices, like using SSH and managing your credentials securely, to prevent these issues from arising in the future.