When a `git push` is taking forever, it often indicates that the repository is large, your network connection is slow, or the remote server is experiencing high traffic or performance issues.
Here’s a simple command to push your changes:
git push origin main
Understanding Git Push
What is Git Push?
The `git push` command is fundamental to the Git workflow, enabling you to transfer your local repository's changes to a remote one. Essentially, it updates the remote repository with new commits from your local branches, allowing collaboration and version control to occur seamlessly.
The syntax for `git push` is straightforward:
git push <remote> <branch>
Here, `<remote>` is typically something like `origin`, and `<branch>` refers to the branch you're pushing changes to, such as `main` or `develop`.
How Git Works Under the Hood
Understanding what happens during a push operation can shed light on why your `git push` might be taking forever. When you initiate a push, Git compares the state of your local branch with the remote one. It determines which objects (commits, trees, and blobs) need to be sent and facilitates their transfer. The larger and more complex these objects are, the longer the push operation may take.
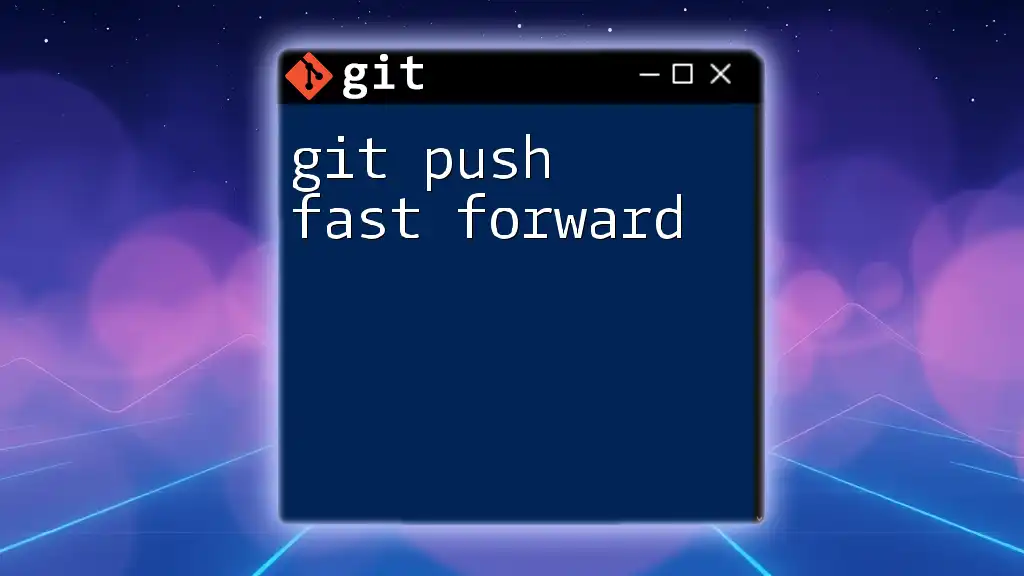
Common Reasons for Slow Git Push
Large Repositories
One significant factor for a slow `git push` is the size of your repository. When a repository contains many files, especially large ones, the time it takes to synchronize changes can skyrocket. Take note of projects like the Linux kernel, which, despite their usefulness, are often cumbersome due to their size.
To mitigate this issue, you can implement a few strategies:
- Use of `.gitignore`: By ignoring unnecessary files and folders that do not need to be versioned, you can significantly reduce the repository size.
- Splitting Large Repositories: Sometimes large monolithic codebases can benefit from being broken up into smaller repositories. This approach, known as microservices architecture, allows for faster pushes among smaller teams.
Data Transfer Overhead
The performance of your network often determines the speed of your `git push` operations. If you're pushing from a location with limited bandwidth, or if your connection is unstable, the output can lag. Additionally, geographical distance from the remote server can introduce latency.
Here are some tips to optimize data transfer:
- Use a faster internet connection: If you are consistently facing slow pushes, consider upgrading your internet service provider.
- Use SSH over HTTPS: SSH is often faster than HTTPS for Git operations and provides secure authentication.
Unoptimized Repo History
An unoptimized repository history can contribute to slower push times. Every commit you make contributes to the overall history of the branch, and a bloated history can slow the comparison process Git undertakes during a push.
To optimize your repo history:
- Squash Commits: By squashing commits, you can reduce the number of objects being pushed. Use the interactive rebase command:
git rebase -i HEAD~n
Here, replace `n` with the number of commits you want to squash.
- Regularly Clean Up Branches: Deleting merged or obsolete branches can lead to a cleaner and faster repository.
Large Binary Files
Pushing large binary files can dramatically impact the speed of a `git push`. By default, Git is optimized for text files rather than binaries. To manage large files, consider using Git LFS (Large File Storage), which allows you to store large files outside the main repository while keeping pointers in Git.
To implement Git LFS, follow these steps:
git lfs install
git lfs track "*.psd"
git add .gitattributes
This will help manage and optimize the transfer of large file types.
Remote Server Performance
Sometimes the performance of the remote server can be a bottleneck for your `git push`. If the server has limited CPU or RAM resources, this could slow down the response time. Server issues, especially if shared with multiple users, might hinder performance further.
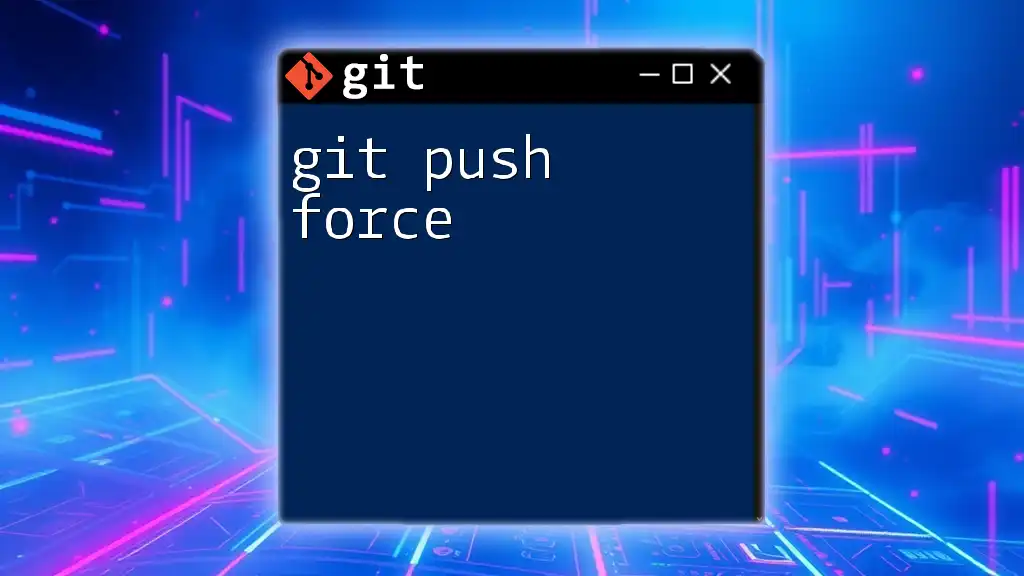
Troubleshooting Slow Git Push
Analyzing Push Performance
To determine the specific reasons for your slow `git push`, consider enabling verbose output:
git config --global core.verbose true
This command will provide detailed feedback during your push operation, helping you identify if the delay is associated with network issues, repository size, or something else.
Tools for Monitoring Git Performance
Several tools can assist in evaluating your Git performance and repository efficiency. Two of the most effective are:
-
Git Sizer: Offers insight into the size of the repository and identifies potential problems.
git-sizer
-
Git Fame: Analyzes the contributions made by team members and can spotlight areas for improvement.
git fame
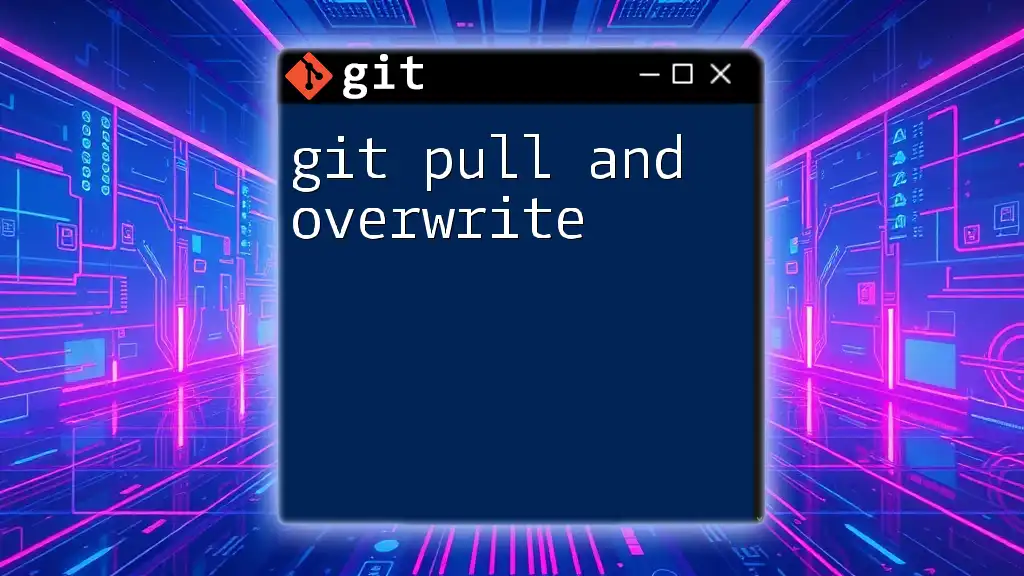
Best Practices for Faster Git Push
General Tips
Keeping your repository clean and easily navigable is essential for improved performance. Make it a habit to conduct a `git pull` before every push. This practice minimizes discrepancies between your local and remote repositories and ensures a smoother push experience.
Using Smaller Commits
Making smaller, logical commits aids in performance. Instead of pushing numerous changes in a single commit, break your work into atomic pieces that are easier to handle. This not only improves push speed but also simplifies collaboration with your team.
Considerations for Team Collaboration
Communication is crucial among team members to prevent large pushes that could lead to conflicts and delays. Establishing branch naming conventions can also alleviate confusion, making it easier for everyone to know what changes are being worked on.
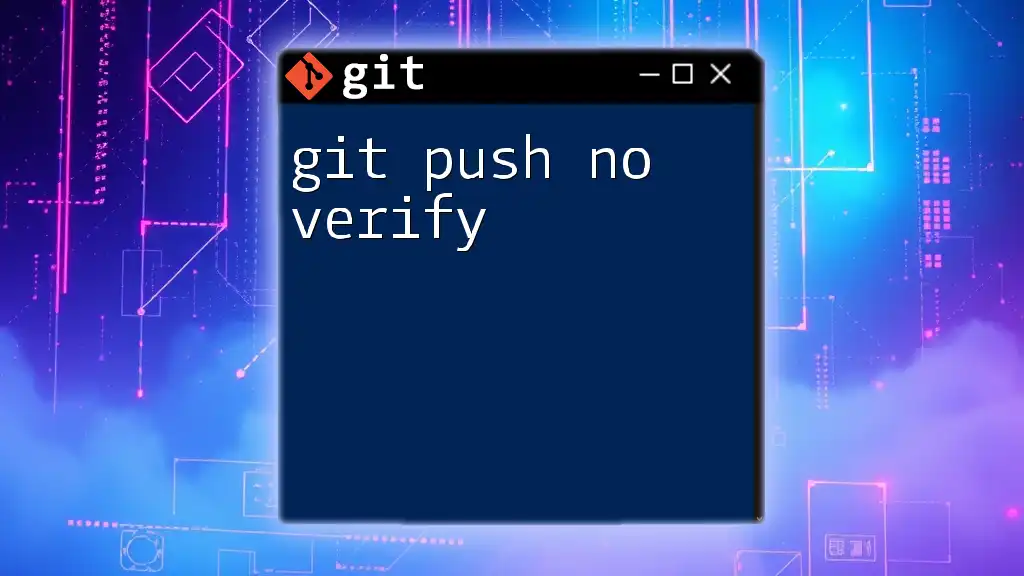
Conclusion
In summary, tackling the issue of a `git push` that is taking forever involves understanding the underlying mechanics of Git and identifying specific pitfalls in your workflow. Regularly monitoring repo health, optimizing file management, and adopting strong collaboration practices can go a long way in ensuring smoother and faster push operations. By incorporating these insights, you can not only improve your own productivity but also foster a more efficient collaborative environment for your team.
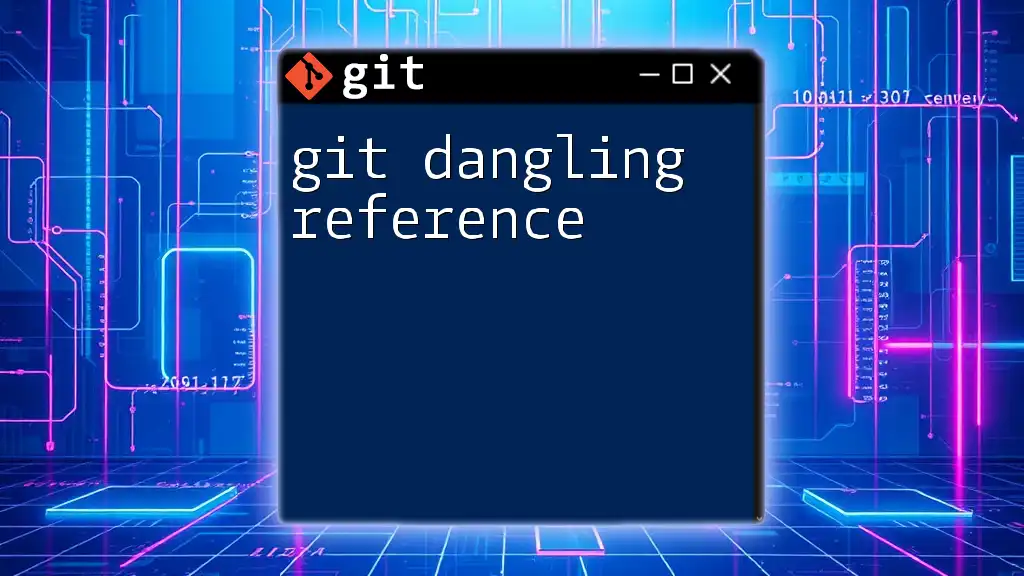
Additional Resources
For further reading on Git best practices and advanced techniques, refer to official Git documentation and stay updated with the latest developments in version control. Subscribing to newsletters or forums specializing in Git can also provide ongoing insights and help you stay ahead in your Git journey.