To overwrite local changes and sync your Git repository with the remote version, use the command `git fetch` followed by `git reset --hard origin/main` after replacing `main` with your branch of interest.
git fetch origin
git reset --hard origin/main
Understanding `git pull`
What is `git pull`?
The `git pull` command is essential for collaborating on projects with Git. It essentially fetches changes from a remote repository and merges those changes into your local branch. By default, `git pull` performs two actions: it first invokes `git fetch` to update your remote tracking branches, and then it executes the `git merge` command to unite the fetched changes with your current branch.
Keeping your local repository synchronized with the remote is crucial for collaborative workflows, helping you ensure that you are working on the latest codebase.
Why Would You Need to Overwrite Local Changes?
Sometimes, you may find it necessary to overwrite your local changes. This can occur in several scenarios:
- Team Updates: If your teammates have made substantial changes that are essential for the project's success, and you want to ensure that your local version aligns with the main repository.
- Resetting Progress: If you realize that your local changes are experimental or flawed, you may wish to discard them.
- Fixing Mistakes: If you've made incorrect changes that you need to revert, overwriting local modifications can save time.
However, it’s essential to understand the risks associated with overwriting local changes, as doing so can lead to loss of work that has not been committed.
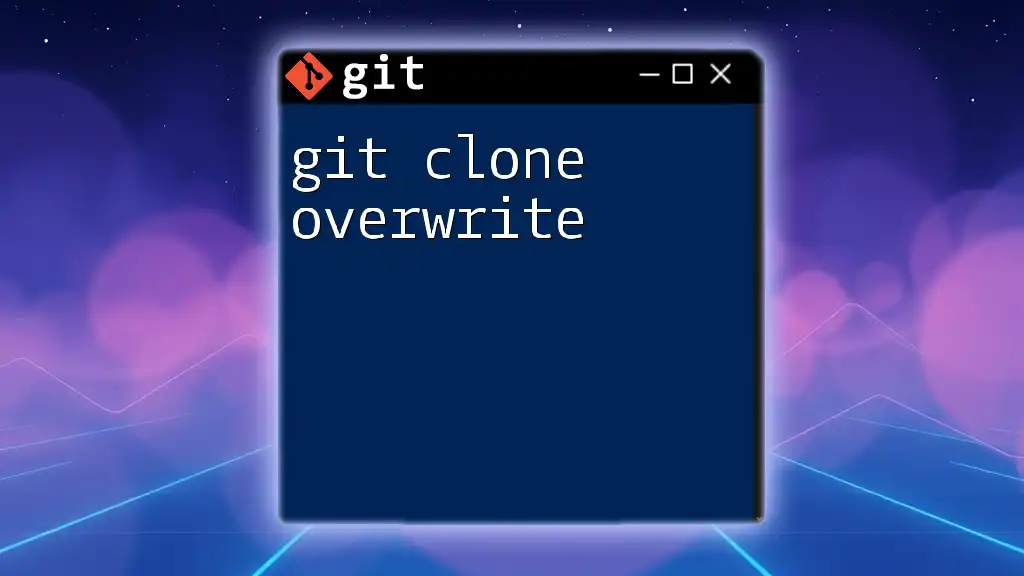
How to Use `git pull`
Basic Syntax and Usage
The basic syntax for `git pull` looks like this:
git pull [options] [<repository> [<refspec>...]]
A typical example to update your `main` branch from `origin` is:
git pull origin main
This command effectively brings the latest changes from the remote `main` branch into your local branch, merging any modifications in the process.
Pulling Changes without Overwriting
To execute a `git pull` without the risk of losing your local changes, just run:
git pull origin main
By default, this command will attempt to merge changes with your current branch, preserving any local modifications that do not conflict with the incoming changes.
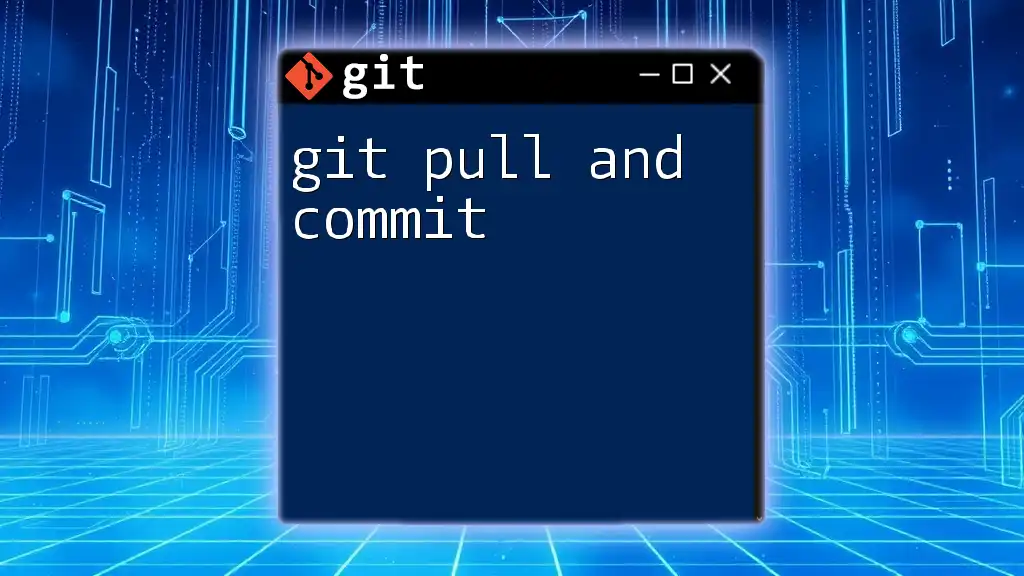
Overwriting Local Changes with `git pull`
The `--rebase` Option
Sometimes, you might want to rearrange your commits after pulling in updates. The `--rebase` option achieves this by placing your local commits on top of the fetched changes:
git pull --rebase origin main
This command brings in changes from the remote `main` branch and re-applies your local commits on top, resulting in a cleaner, linear commit history. This can avoid the messy merge commits that can arise without it.
Force Overwrite with `git reset`
Understanding `git reset`
The `git reset` command is used to reset your local branch to a particular state. It offers three modes: soft, mixed, and hard. When you intend to overwrite your local changes, a hard reset is typically what you need, as it discards all local changes.
Steps to Force Overwrite with `git reset`
Step 1: If you have any uncommitted changes that you want to save, stash them. This step is essential to avoid losing any work. You can stash your changes using the following command:
git stash
Step 2: Next, you can perform a hard reset to match the remote branch. Here’s how you can do it:
git fetch origin
git reset --hard origin/main
This sequence of commands first fetches the latest changes from the `origin` repository and then resets your local `main` branch to the state of the remote `main`, effectively discarding all local changes.
Warning: Data Loss
Using commands that overwrite changes carries a significant risk. Always ensure that you have a backup or have committed any essential work before proceeding with operations like `git reset --hard`. If you overwrite changes unintentionally, you might lose significant progress that cannot be recovered.
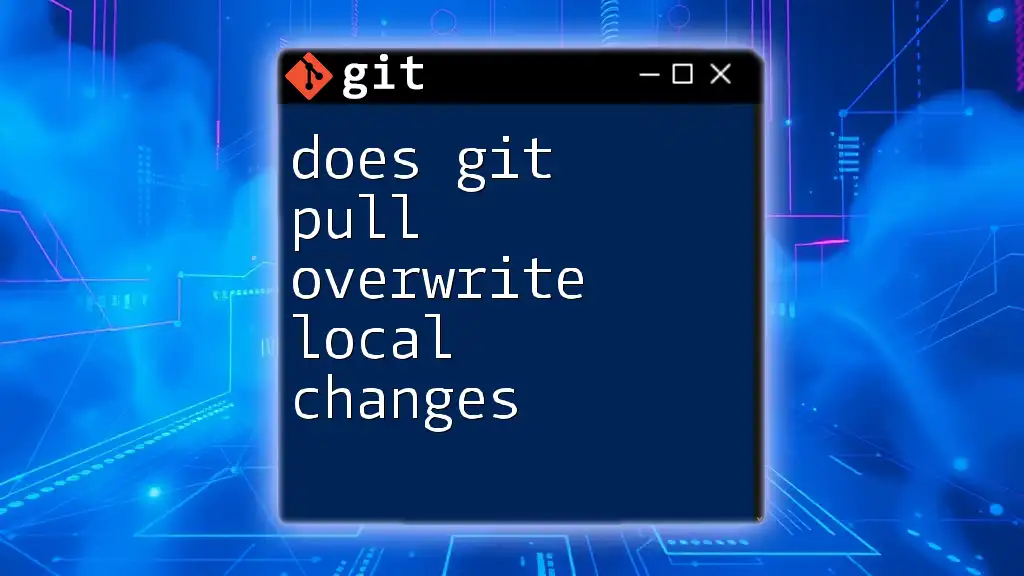
Best Practices for Using `git pull` and Overwriting
Communication Within a Team
Strong communication is paramount when working in a team setting. Establish clear protocols for when team members need to pull changes, ensuring everyone is aligned on project updates. This coordination can minimize the need for overwriting local changes.
Regularly Commit Changes
Commit your changes frequently and before pulling from remote sources. Regular commits create a safety net, allowing for easier management and rollback in case any unwanted changes are introduced during a pull.
Use of Branches
Consider using feature branches for development rather than continually working directly on the main branch. To create a feature branch, utilize:
git checkout -b feature-branch
This approach helps isolate your work and decreases the risk of overwriting precious changes when performing a `git pull`.
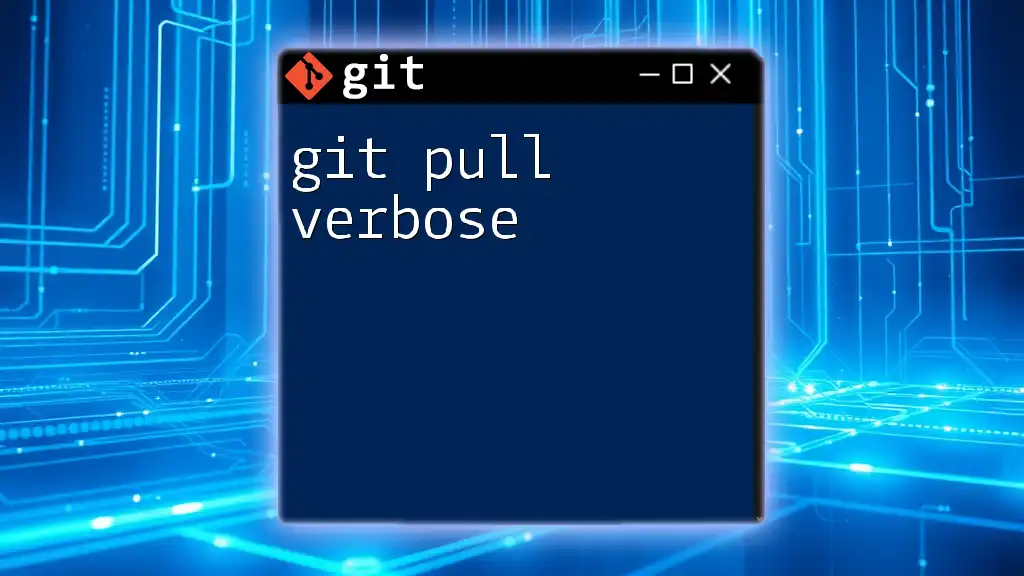
Troubleshooting Common Issues
Merge Conflicts Explained
Merge conflicts may arise when your local changes clash with changes from the remote repository during a pull. Understanding how to resolve merge conflicts is crucial for maintaining a smooth workflow. Git will mark the conflicting sections in your files, allowing you to manually resolve the discrepancies before committing your final code.
What to Do if You Pull the Wrong Branch
If you inadvertently pull changes from the wrong branch, don’t panic. You can revert to safe commit points utilizing the `git reflog` command, which tracks all your recent actions. Here’s how to recover lost commits:
git reflog
Use the output to identify the correct commit to reset or checkout.
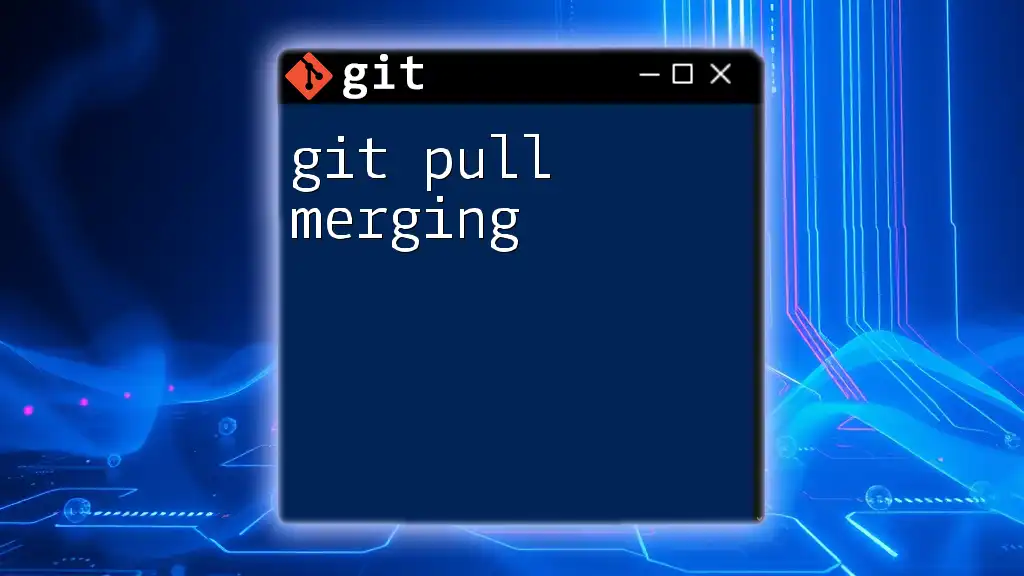
Conclusion
In summary, mastering the `git pull and overwrite` process is vital for effective collaboration and version control. Understanding the implications and risks of these commands ensures you can manage your codebase efficiently. Regularly practicing and engaging in healthy team communication will help streamline your Git workflows, empowering you to navigate version control like a pro. Explore additional resources or tutorials to further enhance your Git skills and stay ahead in your development journey.