The `git pull` command fetches changes from a remote repository and merges them into your current branch, while `git commit` saves your changes to the local repository with a specified message.
git pull origin main
git commit -m "Your commit message here"
What is `git pull`?
`git pull` is a command that simplifies the process of fetching and integrating changes from a remote repository into your local working directory. When working on a collaborative project, it’s important to regularly synchronize your local changes with those made by your teammates to ensure that you are all on the same page and integrated in the latest updates.
The two primary operations that `git pull` performs are fetching and merging. It first fetches the latest changes from the remote repository and then merges them into your current local branch. Understanding how `git pull` works is crucial for effective collaboration, especially in larger teams.
Why Use `git pull`?
Using `git pull` helps maintain a coherent workflow. Here are a few key reasons why it is essential:
- Synchronizing with Remote Repositories: Regularly pulling changes ensures you are working on the most up-to-date version of the project, reducing the chances of running into conflicts later on.
- Team Collaboration: It enables you to see what modifications others have made, which fosters better collaboration and communication.
- Minimizing Merge Conflicts: Performing frequent pulls limits the number of changes that need to be reconciled if you need to sync your work with the latest updates, ultimately resulting in fewer and easier conflicts to resolve.
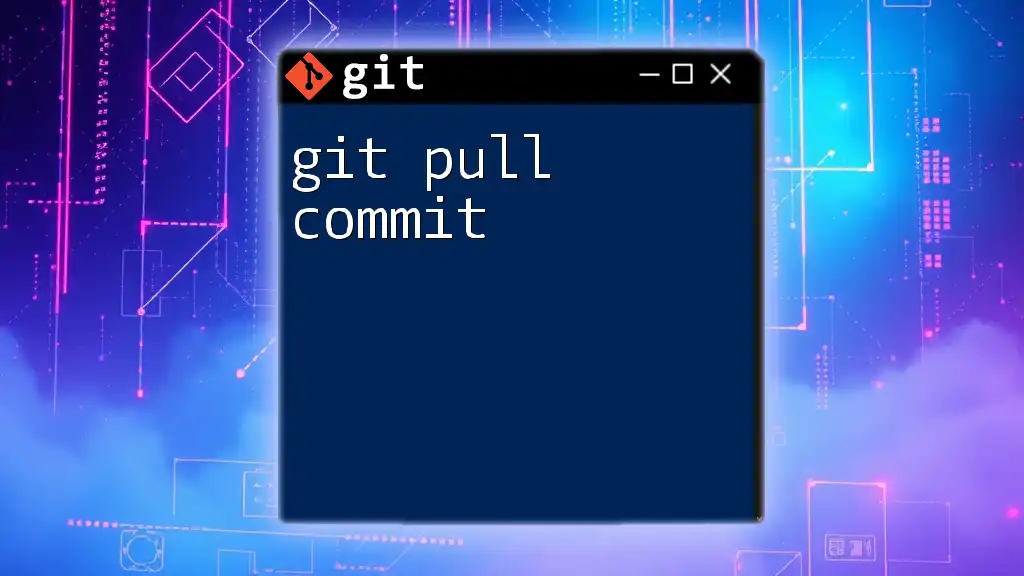
How to Use `git pull`
To execute a pull command, you can use the basic syntax:
git pull [options] [<repository> [<refspec>...]]
Examples of `git pull`
-
Pulling from the default remote repository:
git pull
This command will fetch and merge changes from the current branch's remote counterpart (usually `origin/master`).
-
Pulling from a specific branch:
git pull origin feature-branch
This command allows you to pull updates from `feature-branch` in the `origin` repository. It’s useful when you're working in a team where multiple branches are frequently updated.
Common Options for `git pull`
- `--rebase`: This option re-applies your local changes on top of the fetched branch, which can result in a cleaner project history and help prevent unnecessary merge commits.
- `--all`: This option allows you to pull updates from all remote branches, which could be useful for maintaining a broad overview of the repository.
- `--quiet`: This reduces output messages, creating a cleaner console window, which may be desirable during automated scripts.
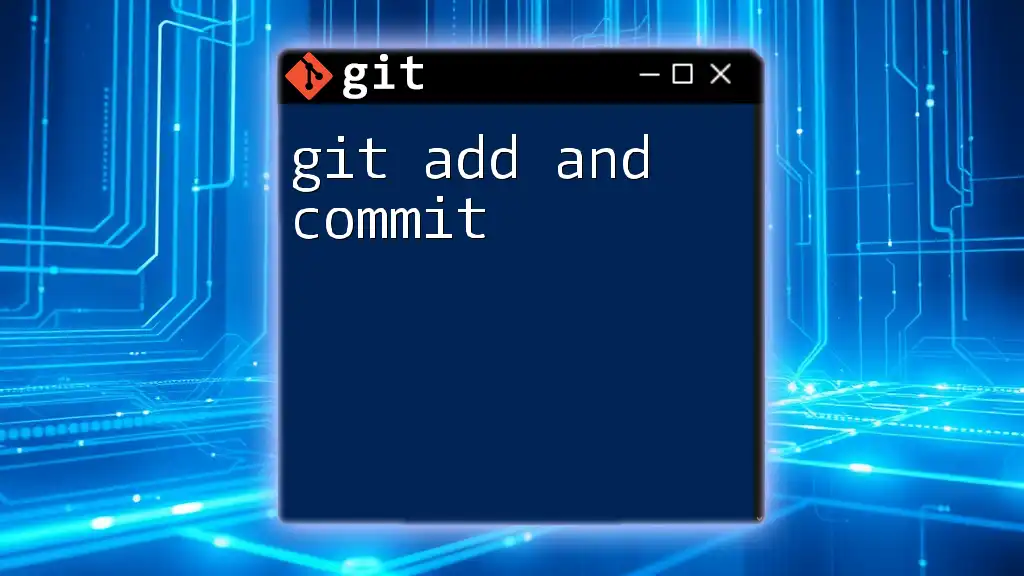
What is `git commit`?
A git commit is a snapshot of your changes that serves as a key part of your Git workflow. It records the current state of your repository so you can track your project's history over time. Each commit includes a commit message that captures the essence of the changes made, aiding in understanding the project's evolution.
Why Use `git commit`?
The act of committing is central not just for documenting your work but also for ensuring accountability and collaboration. Here are reasons why it's vital:
- Capturing Project History: Each commit offers a memory of what was changed and why, helping you and your team trace back steps when needed.
- Facilitating Collaboration: Commit history allows other team members to see what has been done and what has yet to be accomplished.
- Logical Project Progression: Commits enable a structured way to present changes, making it easier to manage and review a project.
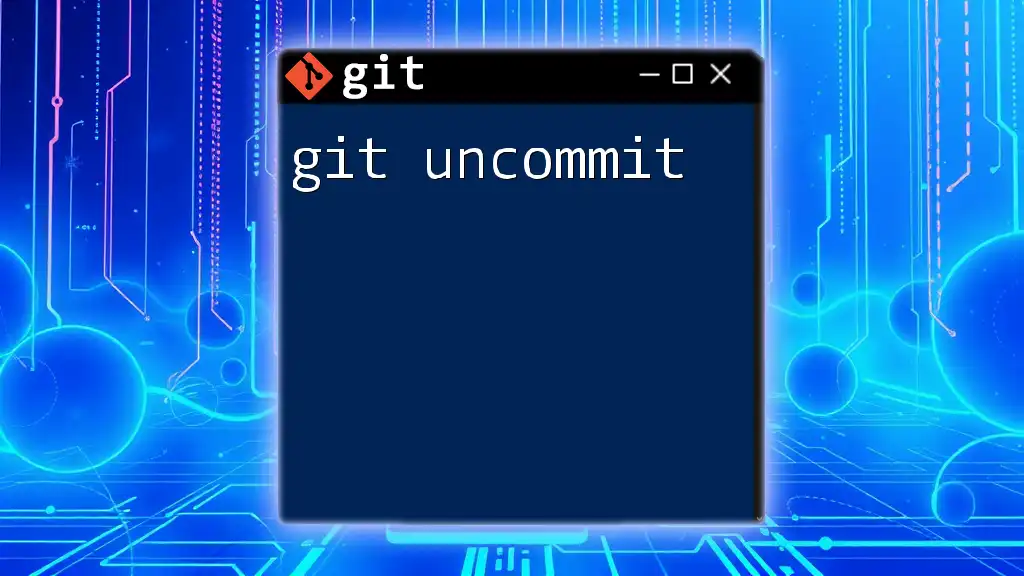
How to Use `git commit`
To commit changes, you can use the following syntax:
git commit -m "Your commit message"
Examples of `git commit`
-
Committing changes with a message:
git commit -m "Fixed bug in user login"
This captures the changes staged in the index with a linked description providing context.
-
Committing all changes:
git commit -a -m "Updated user profile page"
The `-a` flag tells Git to automatically stage files that have been modified and deleted before committing.
Crafting Effective Commit Messages
An effective commit message is crucial for maintaining a clear history. Here are some tips for constructing meaningful commit messages:
- Structure: Use the imperative mood, which makes messages more active and clear (e.g., "Add feature" rather than "Added feature").
- Brevity and Clarity: Be concise yet informative. A well-written commit message can save time for your future self and your teammates, enabling quick understanding of changes.
- Reference Issue Numbers: If applicable, link to issue numbers in your commit messages to provide additional contextual information.
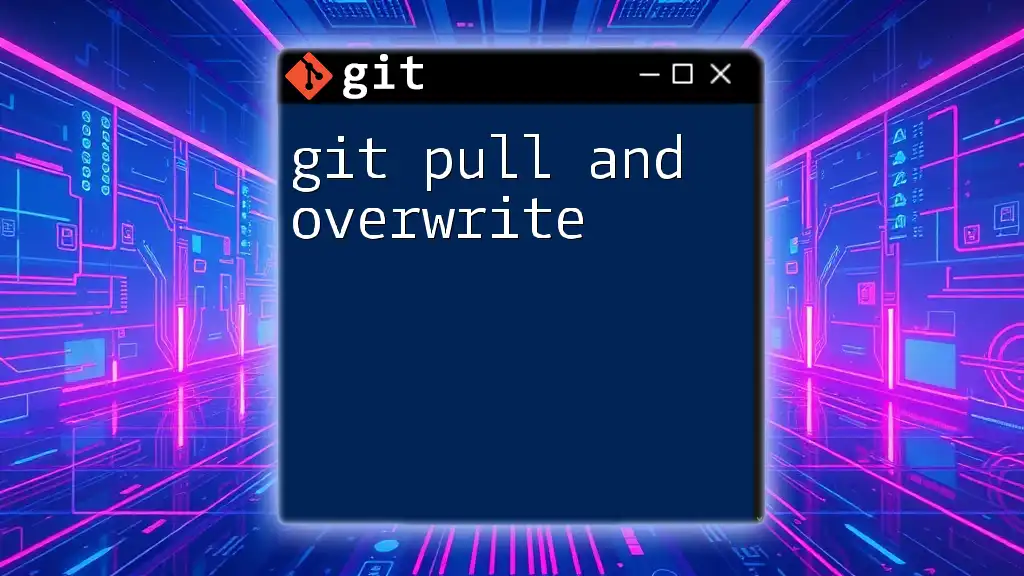
Combining `git pull` and `git commit`
In a collaborative environment, it’s often important to pull changes before making your local commits. Here’s a typical workflow:
- Make local changes to your files.
- Execute `git pull` to fetch the latest changes from the remote repository.
- Resolve any conflicts that arise during this pull.
- Stage your changes using `git add`.
- Finally, commit your changes using `git commit` along with a descriptive message.
This sequence ensures that your changes are made on top of the most recent version of the project, which decreases potential for conflicts and maintains a smooth workflow.
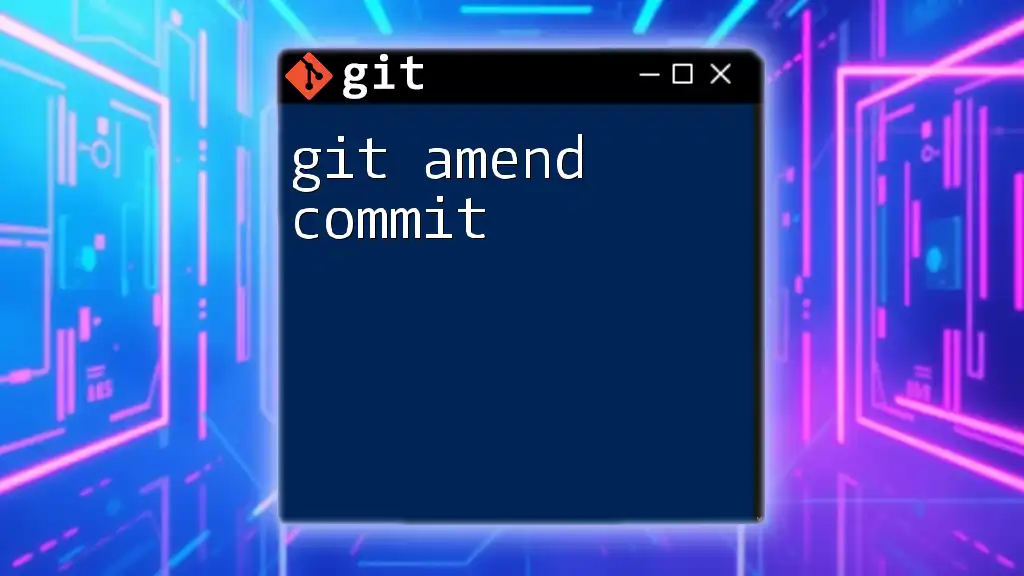
Troubleshooting Common Issues
You may encounter some common issues while using `git pull` and `git commit`. Here's how to handle them:
-
Addressing conflicts after `git pull`: If there are conflicts when pulling, Git will pause the merge and allow you to resolve conflicts manually. Use `git status` to review files with conflicts and edit them to make decisions about which changes to preserve. Once resolved, stage the changes and commit them.
-
Commit errors: Occasionally, you might receive messages that indicate an issue when attempting to commit. Understanding the context of these messages can help you troubleshoot quickly. For instance, if you forget to stage changes, Git will inform you that there’s nothing to commit. Always check your status with `git status`.
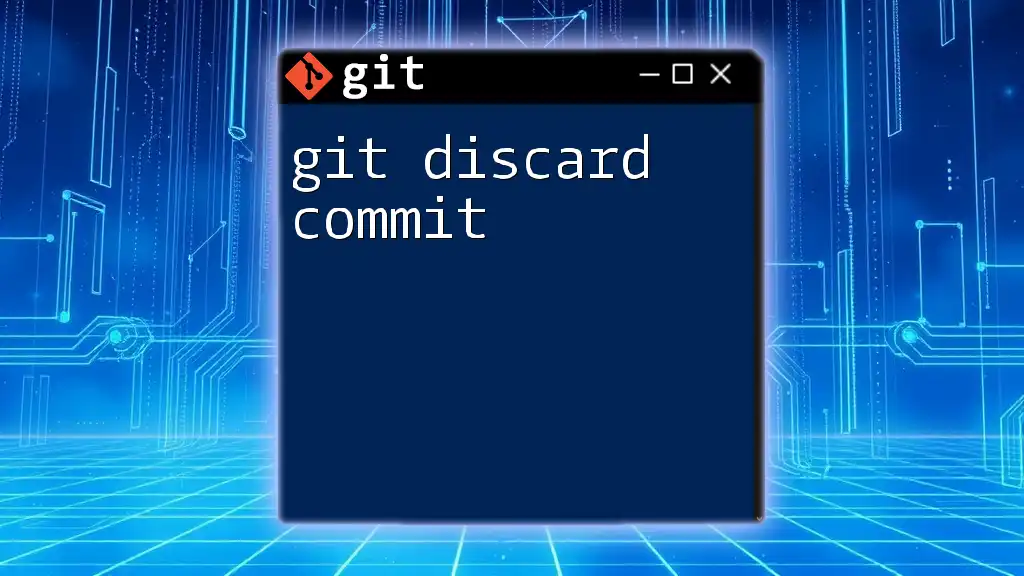
Conclusion
Mastering git pull and commit is fundamental for any developer working with Git. By regularly utilizing these commands, you'll ensure effective communication with your team and maintain a clean project history. Practice the nuances of these commands to refine your skills and enhance your workflow, and don't hesitate to share your experiences or questions as you engage with Git in your projects!
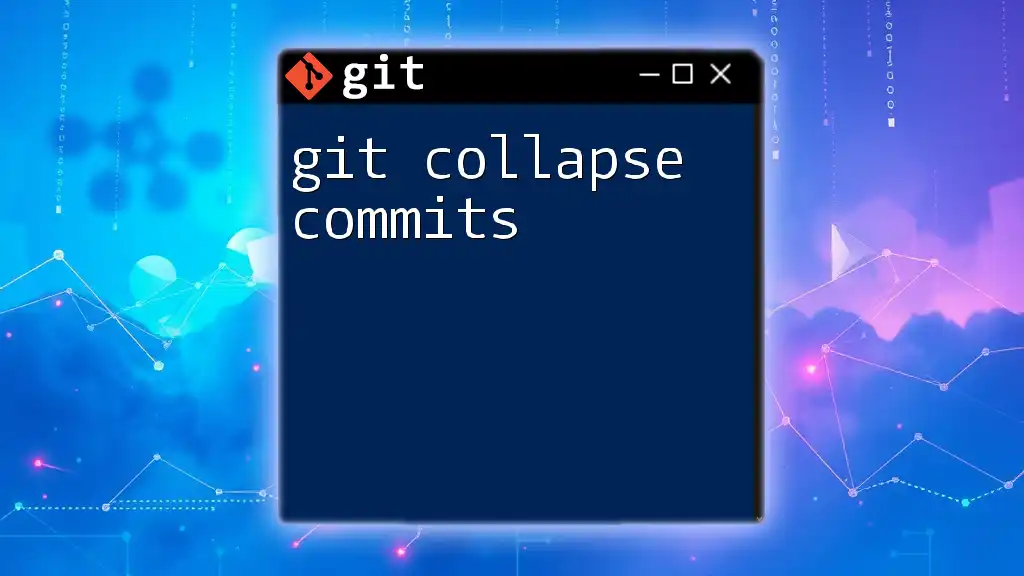
Additional Resources
To further enhance your skills, consider delving into the official Git documentation to explore more on these commands. Additional tutorials and online Git playgrounds for practice can also provide invaluable hands-on experience.