To undo a remote commit in Git, you can use the command to reset your local branch and then force-push it to the remote repository.
git reset HEAD~1 && git push origin your-branch-name --force
Understanding Git Commits
What is a Git Commit?
A Git commit represents a snapshot of your code at a specific point in time. Each commit contains a unique ID, a commit message, and metadata such as the author and the timestamp. By creating consistent commits, you maintain a permanent history of your project, enabling you to track changes and revert to previous states if necessary.
Why You Might Need to Undo a Commit
Many reasons can lead to the need to undo a commit. Common scenarios include mistakenly committing incorrect code, inadvertently including sensitive information, or needing to revert a feature that isn't fully developed. In collaborative environments, it's especially crucial to manage how changes are communicated and reverted since they can impact the work of others.
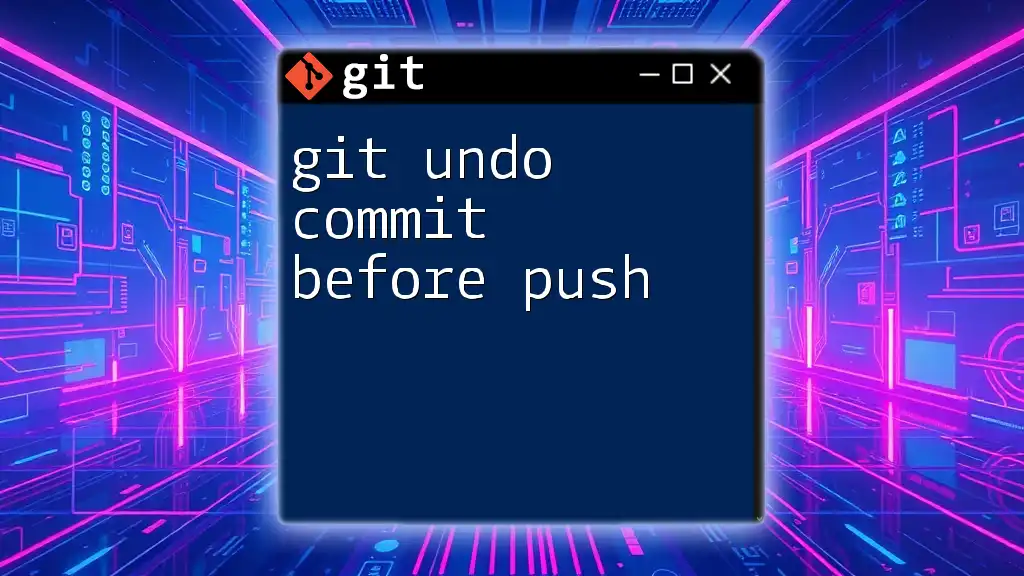
Basics of Remote Repositories
What is a Remote Repository?
A remote repository is a version of your project that is hosted on the internet or a network, commonly on platforms like GitHub, GitLab, or Bitbucket. Unlike your local repository, a remote repository allows multiple users to collaborate and maintain a consistent version of the project.
How To Connect to a Remote Repository
To link your local repository to a remote one, use the `git remote add` command. Here's an example of how to set this up:
git remote add origin https://github.com/username/repo.git
This command establishes a remote named `origin` for your local repo, enabling you to push and collaborate on code with your team.
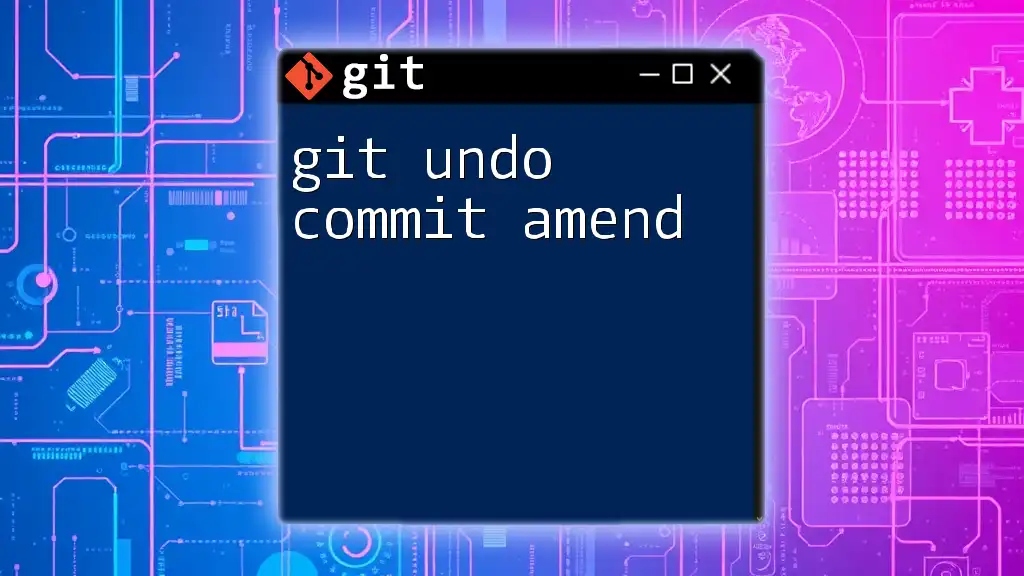
Undoing the Latest Commit on Remote
Step 1: Identifying the Commit to Undo
Before you can undo a commit, you need to identify the specific commit you wish to revert. You can view your commit history by executing:
git log
When you run this command, you’ll see a list of past commits in reverse chronological order. Each entry includes the commit hash, author, date, and commit message, allowing you to pinpoint which commit to undo.
Step 2: Undoing the Commit Locally
Once you have identified the commit hash, you can choose how to undo it. You have two primary methods: soft reset and hard reset.
Soft Reset vs Hard Reset
-
Soft Reset: This option keeps your changes staged, allowing you to modify and recommit them if necessary. To perform a soft reset, use the command:
git reset --soft HEAD~1
-
Hard Reset: This option discards all changes in the last commit, reverting your working directory to match the previous commit. This is used when you're confident you don’t want to keep those changes:
git reset --hard HEAD~1
Step 3: Forcing the Change to the Remote Repository
After the local changes are made, you’ll need to push those updates to the remote repository. In Git, if you previously pushed the commit you are now undoing, you will have to force push the changes to overwrite the remote history. This can be done with:
git push origin HEAD --force
Caution: Force-pushing rewrites the commit history on the remote, potentially affecting other collaborators' work. It is vital to communicate with your team before taking this action to avoid confusion and conflicts.
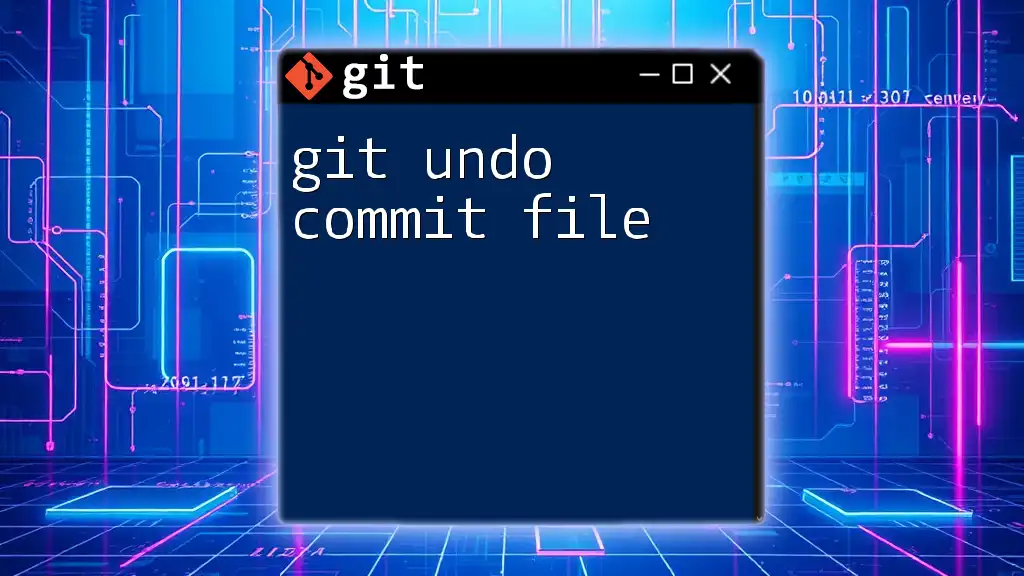
Undoing Older Commits
Using Revert Command
If you need to undo an older commit without altering the commit history, the `git revert` command is your best option. This command creates a new commit that effectively "undoes" the changes made in a specified commit.
For example:
git revert <commit-hash>
This approach has the advantage of maintaining the integrity of the commit history, which is particularly important in a collaborative project. If you are reverting a merge commit, be sure to understand how the `revert` command works in that context, as it can be slightly more complex.
Pushing the Reverted Changes to Remote
Once you have created the revert commit, you can push it to the remote repository using:
git push origin main
This command updates the remote repository with the new changes while keeping the history intact, which is vital for transparent collaboration.
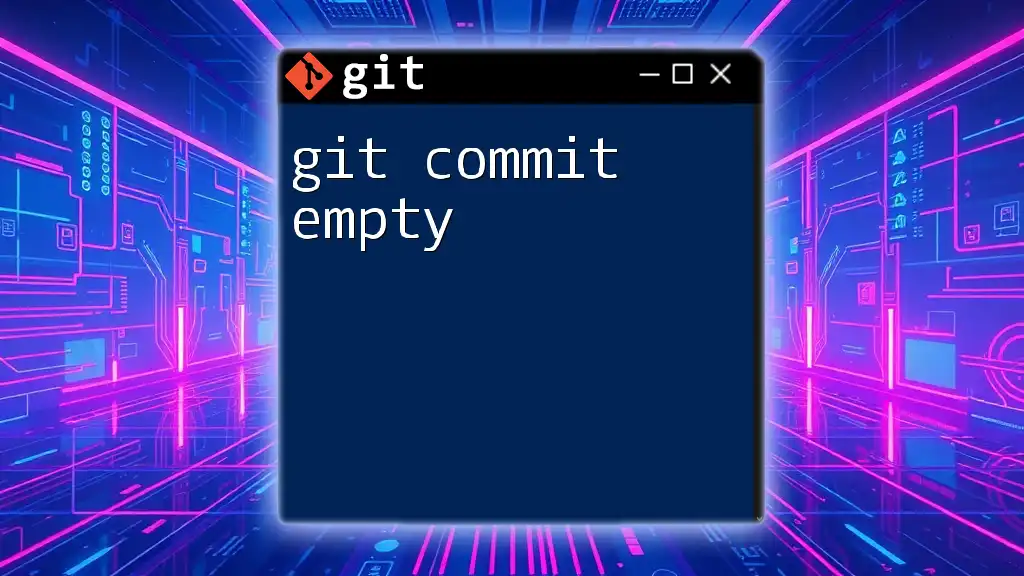
Best Practices for Undoing Commits
Communicate with Your Team
Communication is key when it comes to managing changes in a collaborative environment. Make sure to notify your team members about the changes you are making, particularly about any force pushes. This can help avoid confusion and ensure everyone is aligned.
Regular Backups and Branching
To promote an organized workflow, consider using branches for feature development. By developing in separate branches, you can isolate your changes and avoid cluttering the main branch. This strategy allows for testing and quality assurance before merging into the main codebase.
Testing Before Committing
Before committing, always review your changes using:
git diff
This command shows you the differences between your working directory and your last commit, providing an opportunity to catch mistakes before they become part of the commit history.
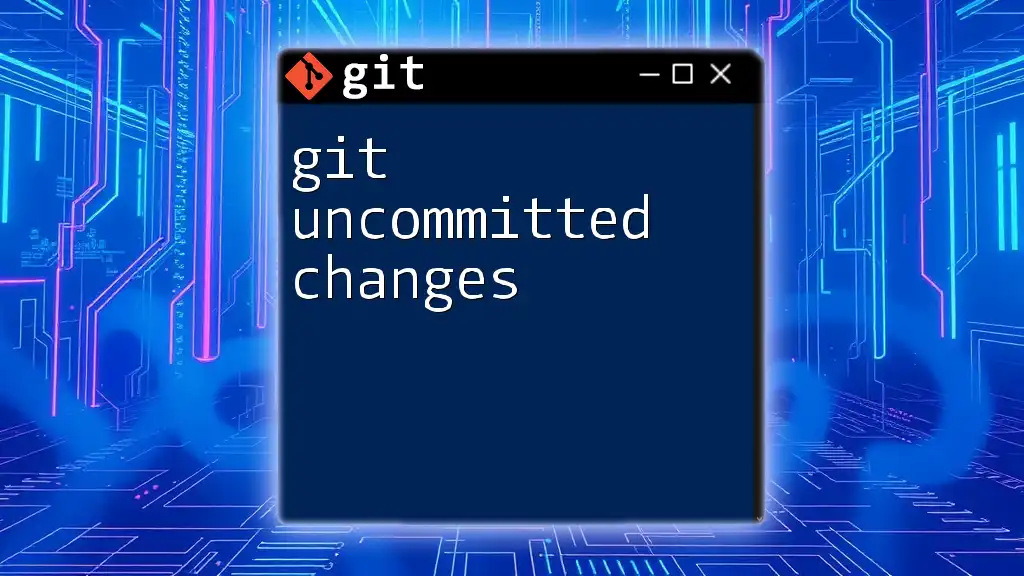
Conclusion
Understanding how to effectively undo commits in remote repositories is a crucial skill for anyone using Git. Whether you’re reverting a mistaken commit or dealing with unexpected changes, knowing the appropriate commands and their implications can save time and maintain project integrity. Practice these commands in a safe environment, and communicate openly with your team to ensure smooth collaboration.
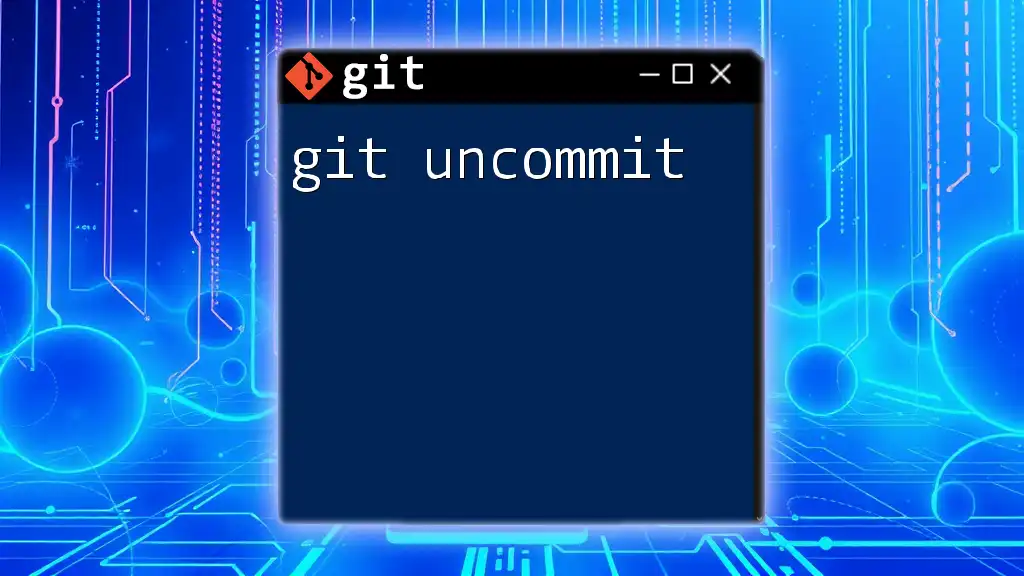
Additional Resources
For further reading, consider exploring the official Git documentation for a more in-depth understanding of Git commands. Additionally, numerous online courses and books can help you master Git. Don’t hesitate to reach out if you have any queries or require further clarification on undoing commits in remote repositories.
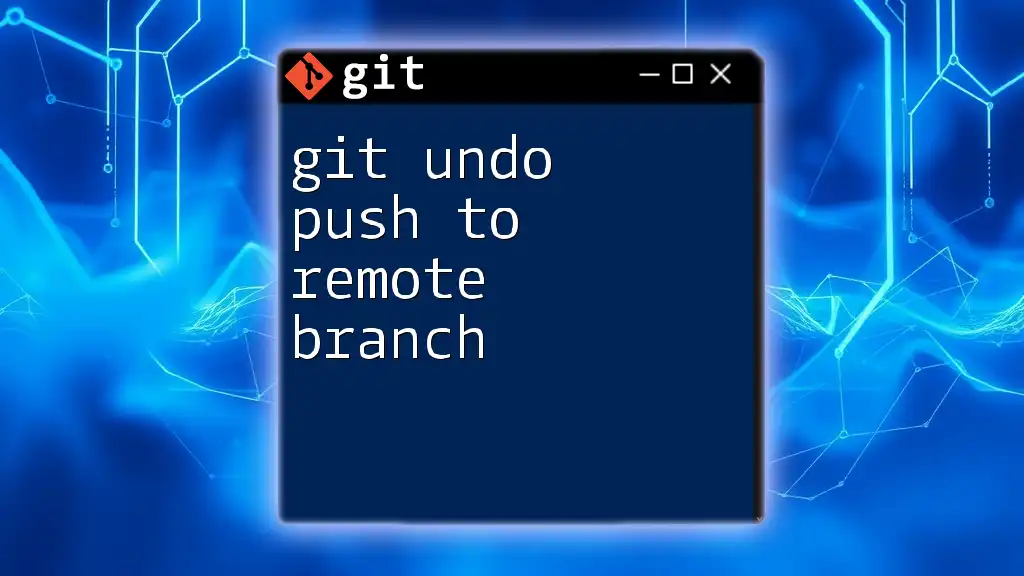
Code Snippets Reference
Here’s a quick summary of the key commands discussed in this article for easy reference:
git remote add origin https://github.com/username/repo.git
git log
git reset --soft HEAD~1
git reset --hard HEAD~1
git push origin HEAD --force
git revert <commit-hash>
git push origin main
git diff
Mastering these commands will empower you to manage your version control effectively!