When you want to include deleted files in your next Git commit, you can stage the changes using the `git add` command followed by the `-u` option to track file deletions.
git add -u
git commit -m "Removed unnecessary files"
Understanding File Deletion in Git
What Happens When You Delete a File?
When a file is deleted in a Git-controlled directory, it's essential to understand how this action interacts with both the working directory and the staging area. The working directory is where the actual files reside, while the staging area acts as an intermediary where changes are gathered before being committed to the repository.
When you delete a file directly from the working directory (using your OS's file manager), Git is not immediately aware of this change. This creates a disconnect that can lead to confusion and inconsistencies if not managed properly. In contrast, using the command `git rm` informs Git about the deletion and stages this change, making it ready for a commit.
Types of Deletion
There are primarily two methods for deleting files within Git:
- Deleting through the file system: This requires additional steps to stage the deletion, which can lead to mistakes if not carefully followed.
- Using `git rm`: This command not only removes the file from your directory but also stages the change, making it clear that the file has been intentionally deleted.
Why You Might Want to Commit Deleted Files
Committing deleted files is an important practice in version control for several reasons. When working on collaborative projects, keeping information transparent is vital. Committing deletions ensures that all team members are aware of changes to the file structure, maintaining project integrity and minimizing confusion about the current state of the codebase.
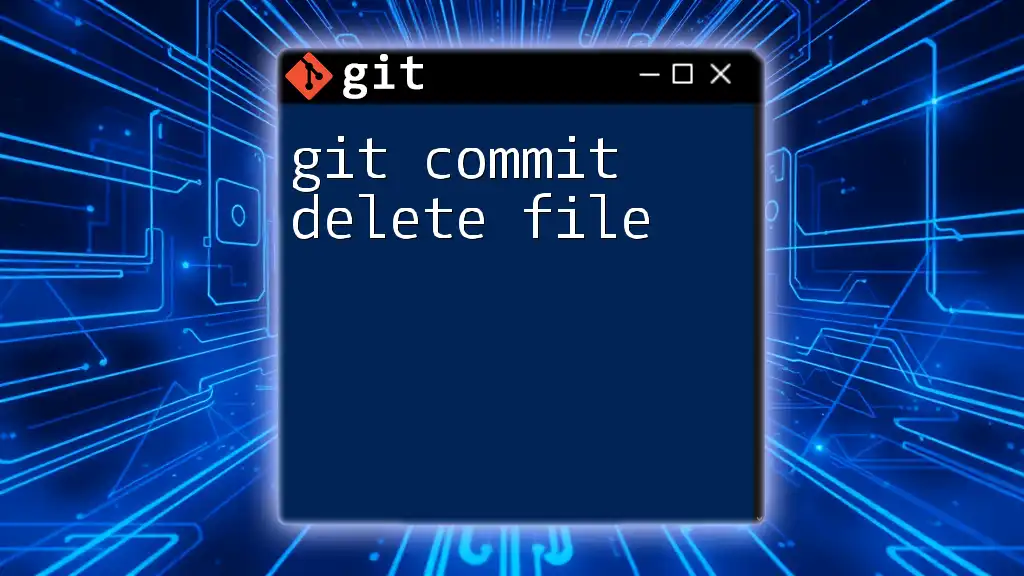
The Git Command: `git rm`
What is `git rm`?
The command `git rm` is specifically designed to remove files from both your working directory and the staging area in Git. This command is fundamental when you want Git to track deletions efficiently.
Basic Syntax and Examples
To use the command, the basic syntax is straightforward:
git rm <file>
For example, if you want to remove an outdated file named `old_file.txt`, you can execute the command:
git rm old_file.txt
By using `git rm`, the file is removed from your working directory and stakeholders can see the deletion staged and ready to be committed.
Additional Options with `git rm`
You can enhance the functionality of `git rm` with additional options:
- Using the `-r` flag: This flag allows you to delete directories and their contents recursively.
git rm -r folder_name
- Using the `--cached` flag: This option will remove the file from the staging area while keeping it in your working directory. This is particularly useful if you want the file to remain locally while removing it from the version control.
git rm --cached file_to_keep.txt
Each option can cater to specific needs, demonstrating the versatility of the `git rm` command.
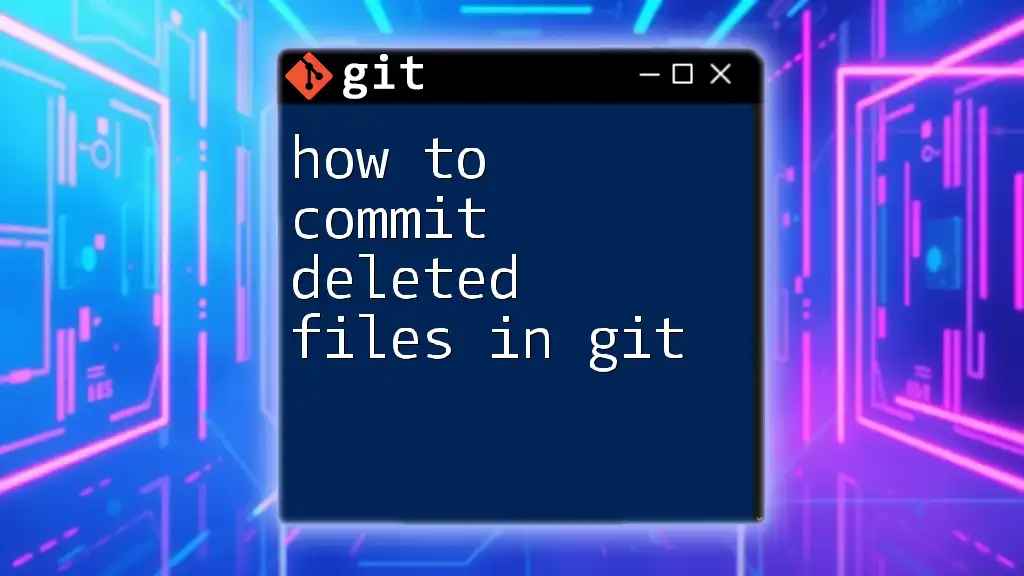
Committing Deleted Files
The Commit Process
After you've used `git rm` to delete files, you need to commit these changes to the repository for them to take effect. This is done with the `git commit` command, which captures your staged changes in a new commit, keeping the project history comprehensive.
Syntax and Examples
To commit your changes after deletion, you may use the following command:
git commit -m "Removed old_file.txt"
In this command, the `-m` flag allows you to write a concise commit message that describes the change. It’s crucial to keep your messages clear and meaningful so that team members can understand the context of the deletion. Good commit messages enhance the project's overall maintainability.
Best Practices for Commit Messages When Deleting Files
A well-crafted commit message when deleting files is essential. It should provide enough context for why the file was deleted. For instance, instead of a vague message like "deleted files," use something descriptive such as "Removed deprecated API documentation to clean up repository." This provides clarity and aids in future debugging or updates.
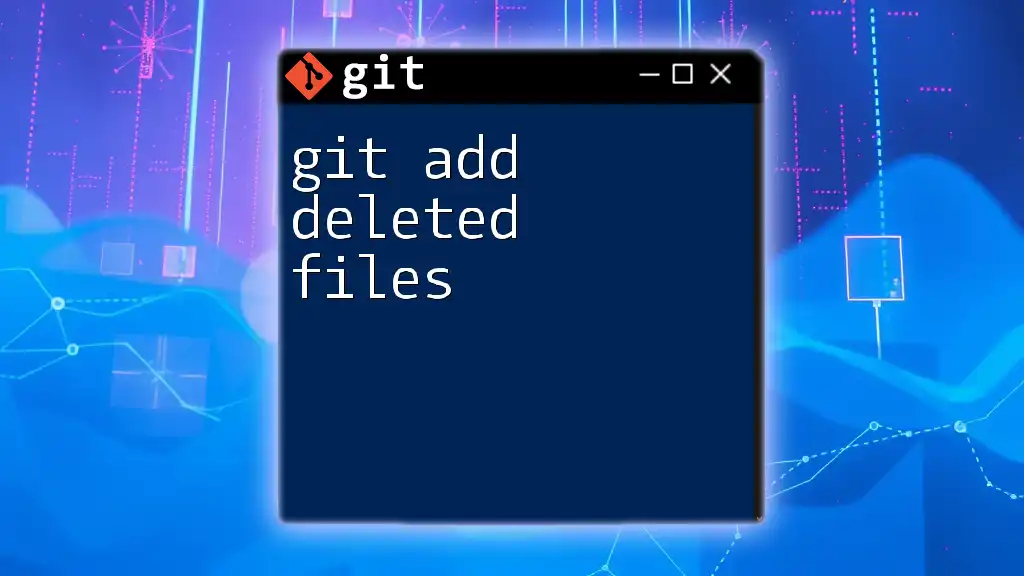
Recovering Deleted Files in Git
How to Restore Deleted Files
If you've deleted a file but need to recover it, Git provides mechanisms to handle this. One way is to use the `git checkout` command to restore a file to its state before deletion.
git checkout HEAD^ -- deleted_file.txt
In this command, `HEAD^` refers to the state of the repository just one commit prior. Thus, this command retrieves the version of the file as it existed before it was deleted.
Alternatives for Recovery
Another way to find and restore deleted files is through the use of `git reflog`. This command allows you to look back at the history of your commits, including those before the deletion occurred.
git reflog
From the reflog, you can locate the commit hash of the way the repository was before the deletion and use it to check out the deleted file or even restore the entire commit.
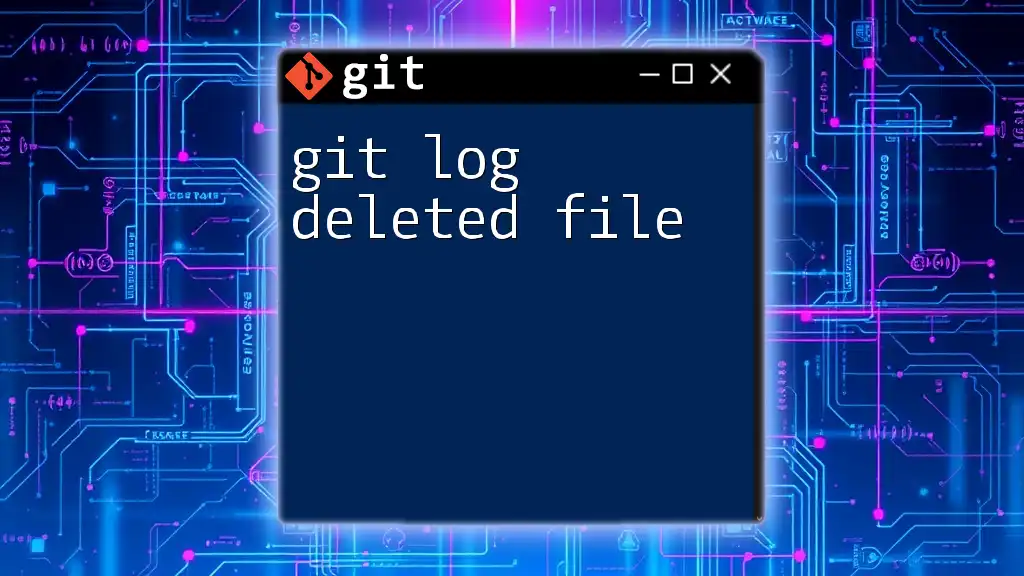
Common Pitfalls When Committing Deleted Files
Forgetting to Stage Changes
A common mistake is forgetting to stage the deletion before committing. When you delete a file directly in the file system, Git does not automatically stage this change, which means your commit will not include the deletion. This oversight can lead to inconsistencies in project history.
Commit Mismanagement
Users sometimes find themselves committing previous states unwittingly. This situation often arises due to a lack of awareness of the current working directory. It’s crucial to stay mindful of which files are staged and which are not.
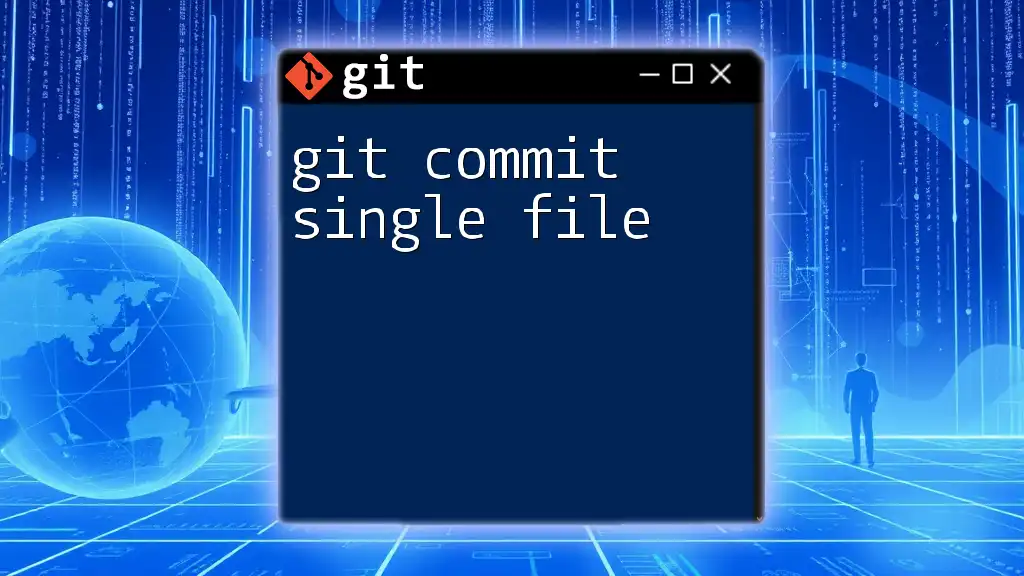
Conclusion
Understanding how to manage deleted files with Git involves more than just using commands; it requires a practice of maintaining clarity and integrity in your projects. By mastering commands like `git rm` and adhering to best practices for commit messages, you’ll significantly enhance your version control proficiency. The ability to recover files and recognize potential pitfalls further solidifies your command over Git, making version control a more manageable aspect of your development workflow.
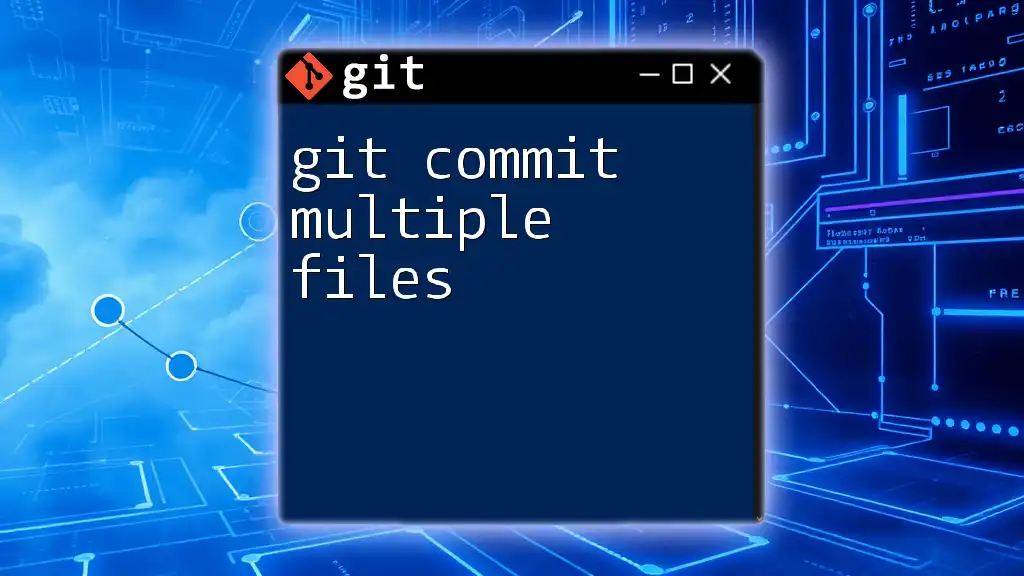
Additional Resources
For anyone looking to deepen their understanding of Git, refer to the official Git documentation for comprehensive guidelines and examples. Additionally, exploring books and tutorials on Git can provide even greater insights and practical experience with version control.