To commit a single file in Git, use the `git commit` command along with the `-m` flag to include a commit message and specify the file you want to commit.
git commit -m "Your commit message" path/to/your/file.txt
Understanding Git Commits
What Does "Committing" Involve?
Committing in Git involves saving changes in your project permanently. Each commit creates a historical snapshot of your project's files at a particular point in time, allowing you to track and revert changes when necessary. Unlike simply saving a document on your computer, which just updates the file, a commit captures the entire state of your repository, enabling collaboration and effective project management.
Reasons to Commit a Single File
When working with version control, committing a single file rather than multiple files at once can be immensely beneficial. It enhances the clarity and focus of your commit, thereby making it easier to understand the progress of development. This practice also streamlines collaboration with teammates; when you make clear, directed commits, it becomes easier for others to perform code reviews and track the evolution of specific features or bug fixes. Additionally, smaller, more focused commits may simplify debugging and rollbacks, should issues arise.
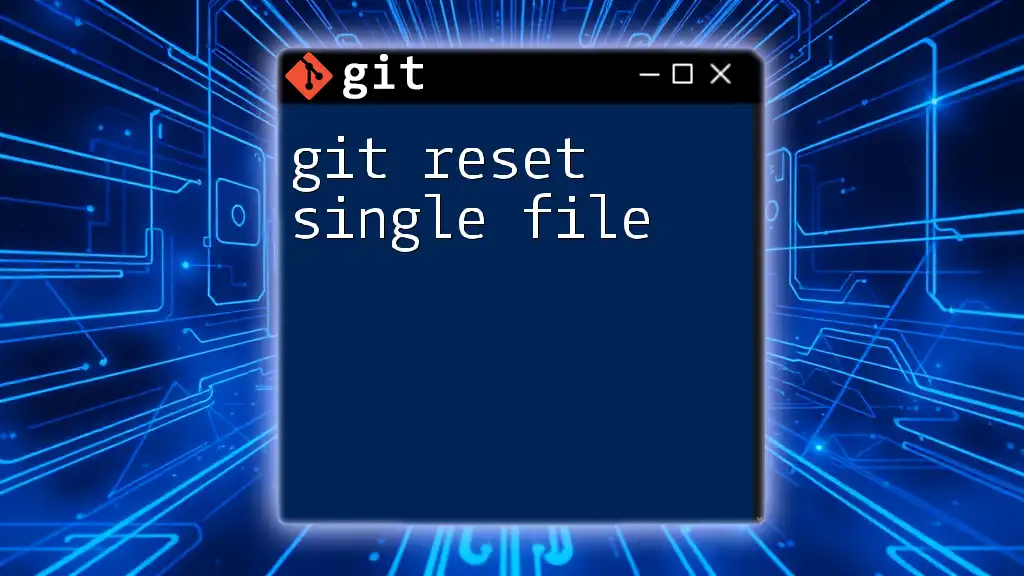
Preparing for a Single File Commit
Making Changes to Your File
Before you can commit, you first need to make changes to the particular file you are focusing on. Editing a file is as simple as opening it in your preferred code editor, making the necessary modifications, and saving those changes. It's essential to choose a code editor that integrates well with Git, such as VSCode, Sublime Text, or Atom, to streamline your workflow.
Checking the Status of Your Repository
After making your changes, it's a good habit to check the status of your repository. You can do this using the `git status` command. This command is vital as it informs you of the current state of your working directory and staging area. For example:
git status
The output will detail modified, new, or deleted files, giving you a clear insight into what has changed since your last commit.
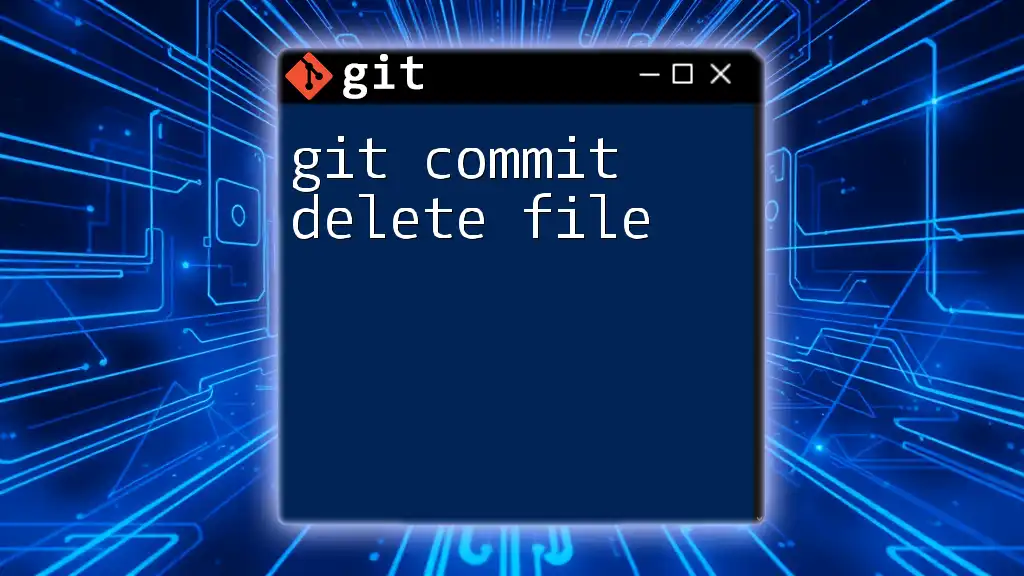
Committing a Single File
Staging the File
To commit a specific file, you need to stage it first. Staging is the process of preparing your changes for commit. You can stage a single file by using the `git add` command followed by the path to the file. For example:
git add path/to/your-file.txt
After staging the file, you can verify that it has been staged by running `git status` again. You should see that your file is now in the "Changes to be committed" section.
Committing the Staged File
Now that you have staged your file, you are ready to commit. The `git commit` command is used for this purpose. It allows you to create a snapshot of your file and includes a message that describes the changes made. The syntax is simple:
git commit -m "Your commit message describing the change"
Writing an effective commit message is crucial. Your message should be clear and concise, allowing others (and yourself) to understand the purpose of the change later. Aim for an imperative mood (e.g., "Fix bug in user login") to convey the action taken.
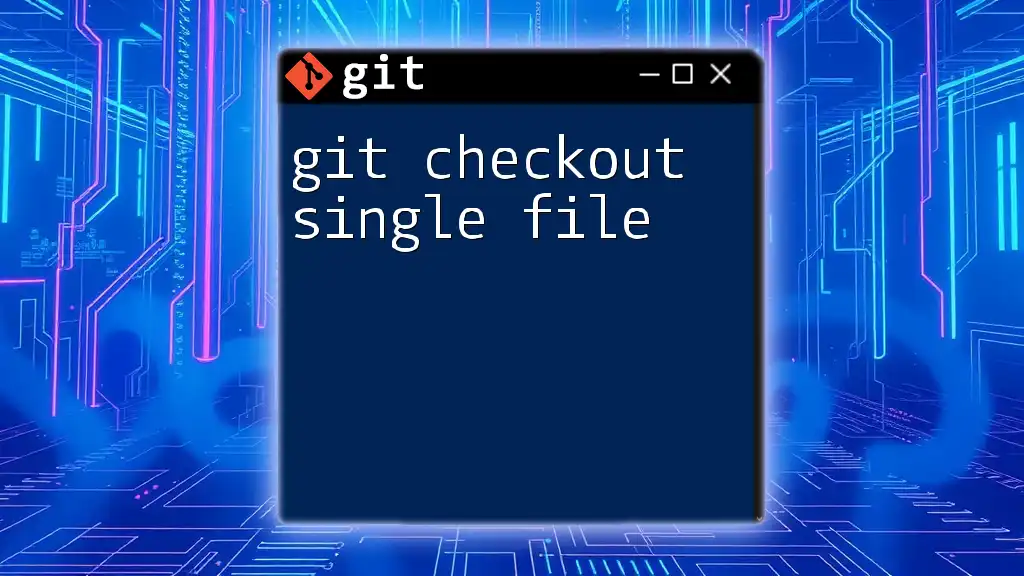
Advanced Techniques
Amending a Commit
Sometimes, you may realize that you need to make changes to your last commit. Git allows you to amend a commit using the `git commit --amend` command, which can modify the content and message of the prior commit. For example:
git commit --amend -m "Updated commit message to reflect latest changes"
This command is particularly useful when you forget to include changes or want to clarify the purpose of the commit.
Committing with a Patch
If you only want to stage parts of a file for your commit, you can use Git's interactive staging mode with the `git add -p` command. This command presents you with a series of changes in your file and asks if you want to stage them. This is especially handy when a file has multiple changes and you only want to commit specific portions.
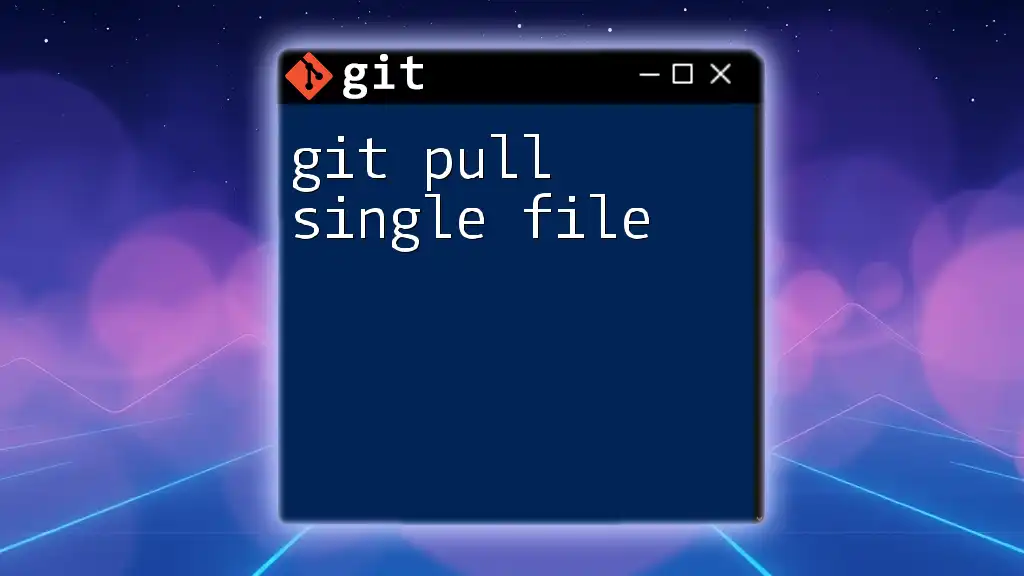
Best Practices for Committing in Git
Frequent, Small Commits
Committing frequently with smaller changes helps maintain clear project history and simplifies debugging efforts. Each commit becomes a distinct point in your project’s timeline, making it easier to identify when and where issues arose.
Use of Structured Branches
Creating and using structured branches for different features or bug fixes allows you to experiment without affecting the main codebase. By committing your changes in branches, you can keep the main branch clean and only merge stable, reviewed features.
Consistent Commit Messages
Consistency in your commit messages is crucial. Creating a commit message template can help maintain uniformity. This could include sections for the type of change (e.g., feature, fix), a concise description, and references to related issues or pull requests.
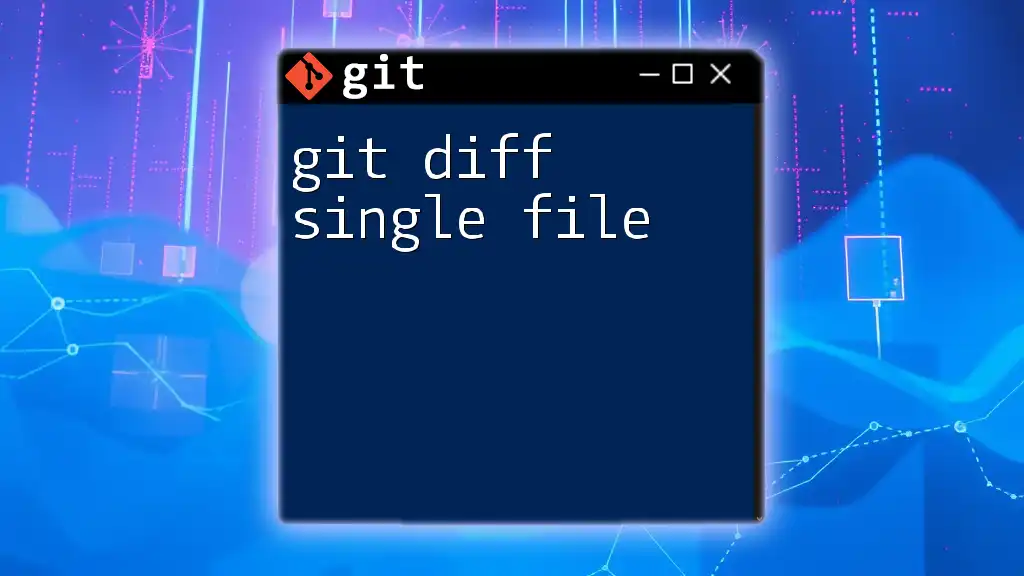
Troubleshooting Common Issues
What if You Forget to Stage a File?
If you realize post-commit that you forgot to stage a file, don’t worry. You can simply make your changes to that file, stage it using `git add`, and commit again. These operations create an additional commit, and it’s recommended to write a message that ties this commit to the previous one for context.
Dealing with Commit Conflicts
Merge conflicts can occur when multiple changes are made to the same line in a file by different team members. Understanding how to resolve these conflicts is essential for collaborative workflows. Git will prompt you to manually address these conflicts, allowing you to decide which changes to keep. After resolving, you can stage and commit the reconciled changes as you normally would.
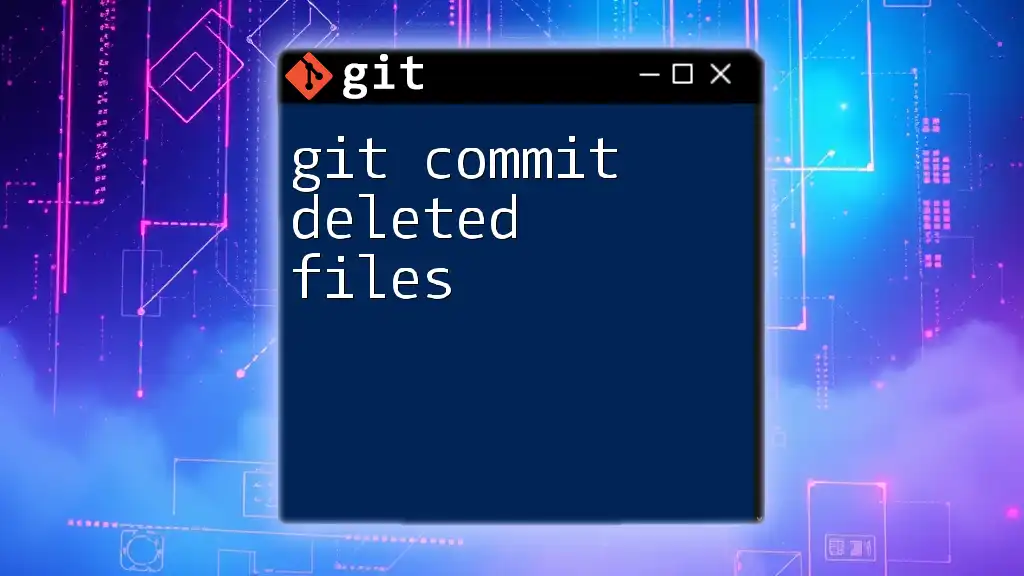
Conclusion
Committing a single file in Git is a fundamental skill that can significantly enhance your workflow. By following best practices such as writing meaningful commit messages, staging thoughtfully, and utilizing branches, you can develop a cleaner, more manageable codebase. Practice committing single files in your projects to solidify your understanding and improve your collaborative efforts.
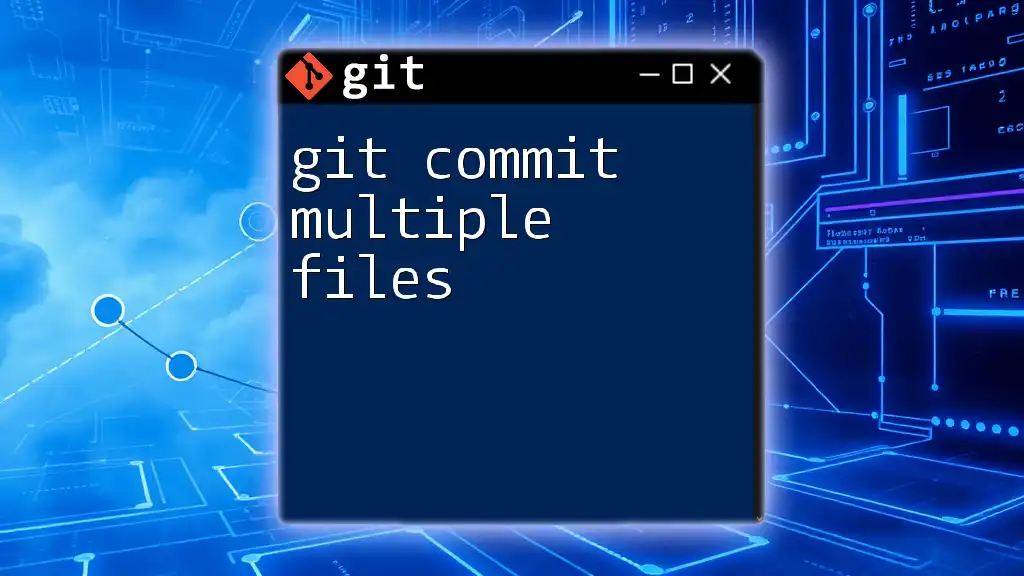
Additional Resources
For those looking to deepen their understanding of Git, consider exploring the official Git documentation and other comprehensive resources available online. With practice and familiarity, you'll become proficient in managing your projects with Git.