To restore a specific file from the last committed state in your Git repository, you can use the `git checkout` command followed by the file name.
git checkout -- path/to/your/file.txt
Understanding `git checkout`
What is `git checkout`?
`git checkout` is a fundamental command in Git that allows users to switch branches or restore working tree files. When we talk about checking out a single file, we refer to the ability to retrieve a specific version of a file from a different commit or just restore it to its last committed state. This command is crucial for maintaining and managing versions of files as you make changes over time.
Date and Version Control Context
In the world of version control, every change made to a repository is tracked through commits. Each commit represents a snapshot of the project at a given point in time. This means that if you ever need to access an older version of a file — whether you mistakenly modified it or need to revert to a previous implementation — `git checkout` serves as an efficient tool to accomplish that.
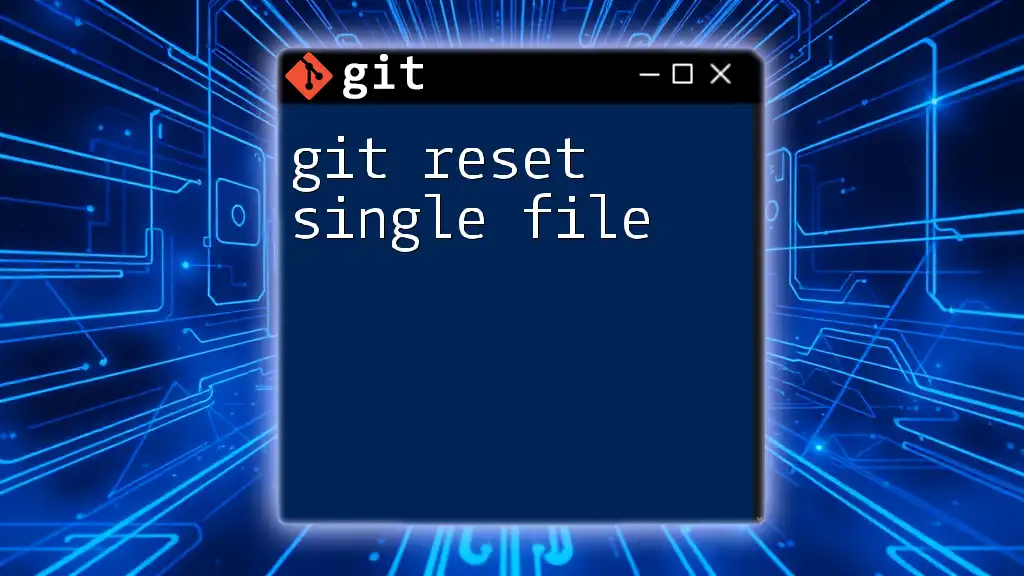
Using `git checkout` to Restore a Single File
Basic Syntax of the Command
To check out a single file, use the following syntax:
git checkout <commit_hash> -- <file_path>
Breaking this down:
- `<commit_hash>` is the identifier of the commit from which you wish to retrieve the file.
- `--` indicates that what follows are files rather than branches or commits.
- `<file_path>` is the path of the file you want to check out.
Recovering the Latest Committed Version of a File
To restore the latest committed version of a file — say, because you've made unintended changes but haven't staged them — simply use:
git checkout -- <file_path>
This command will revert the specified file back to its last committed state. It’s different from staging changes; it permanently reverts any changes made in the working directory since the last commit.
Checking Out a File from a Specific Commit
If you need to check out a specific version of a file, follow these steps:
- Identify the commit from which you want to restore the file using:
git log
- Once you've found the desired commit hash, use:
git checkout <commit_hash> -- <file_path>
For example, if you need to restore a configuration file from an earlier commit to fix a bug, this command comes in handy.
However, be cautious: when checking out a file in this manner, any changes to the current version of that file will be lost unless they are staged or committed.
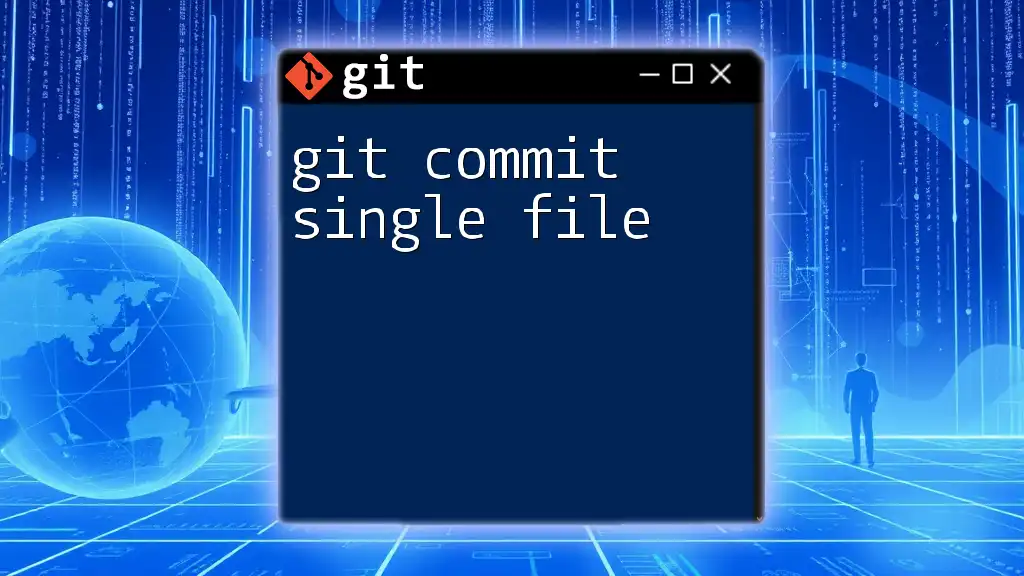
Practical Examples
Example 1: Restoring a Deleted File
Imagine you accidentally deleted a file that you need back. Here’s how to restore it:
- Delete the file using:
rm deleted-file.txt
- To restore it, run:
git checkout HEAD -- deleted-file.txt
This command pulls the last committed version of `deleted-file.txt` back into your working directory, effectively undoing the delete action.
Example 2: Reverting Changes in a Modified File
Suppose you've made several changes to a file, but you decide that you don’t want to keep those changes before staging them. The command to revert the modifications is simple:
git checkout -- modified-file.txt
Executing this command will discard all local changes to `modified-file.txt` since the last commit, bringing it back to its pristine state.
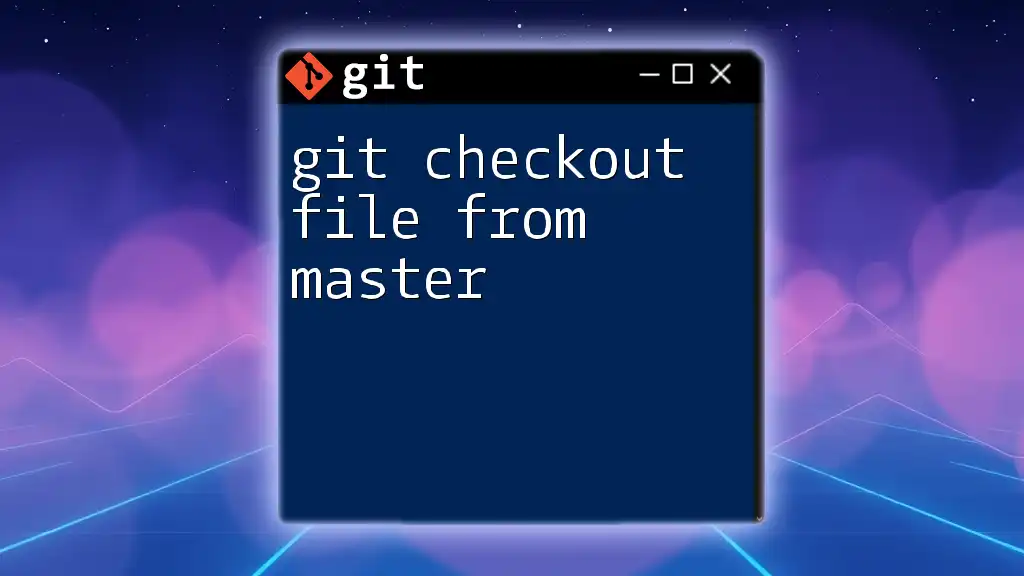
Common Mistakes and Best Practices
Misusing `git checkout`
Using `git checkout` improperly can lead to unintended data loss. For instance, if you forget to stage changes before checking out a file, those uncommitted changes are gone forever. Therefore, it's vital to ensure that any important work is saved before running this command.
Best Practices for Using `git checkout`
- Double-check the file path and commit hash: A small typo can lead to unexpected results.
- Always have access to backups: Keep regular backups of your work to mitigate risks.
- Commit before you checkout: Whenever possible, make a commit before using `git checkout` to ensure that you don't lose any changes.
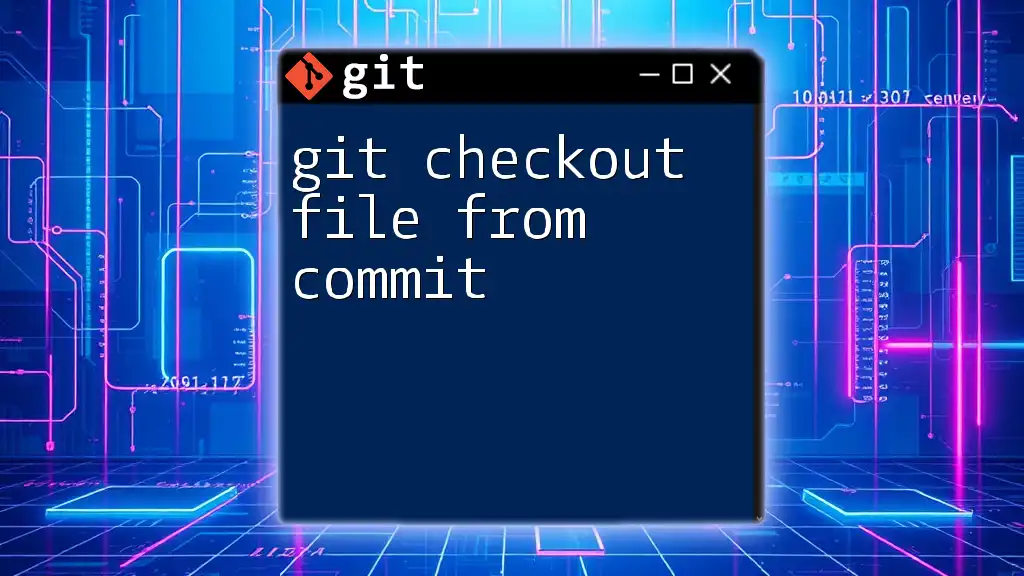
Alternatives to `git checkout`
Introduction to `git restore`
As of more recent versions of Git, `git restore` was introduced, allowing for more intuitive file restoration. The command functions similarly for restoring a file:
git restore <file_path>
This command offers a clearer context for managing file states and is intended to address some confusion around `git checkout`.
Brief Overview of Other Related Commands
- `git reset`: This command can be used to change the state of the index and working directory but works differently compared to `checkout`.
- `git revert`: This creates a new commit that undoes the changes made in a previous commit, contrasting with `checkout`, which simply retrieves a file version.
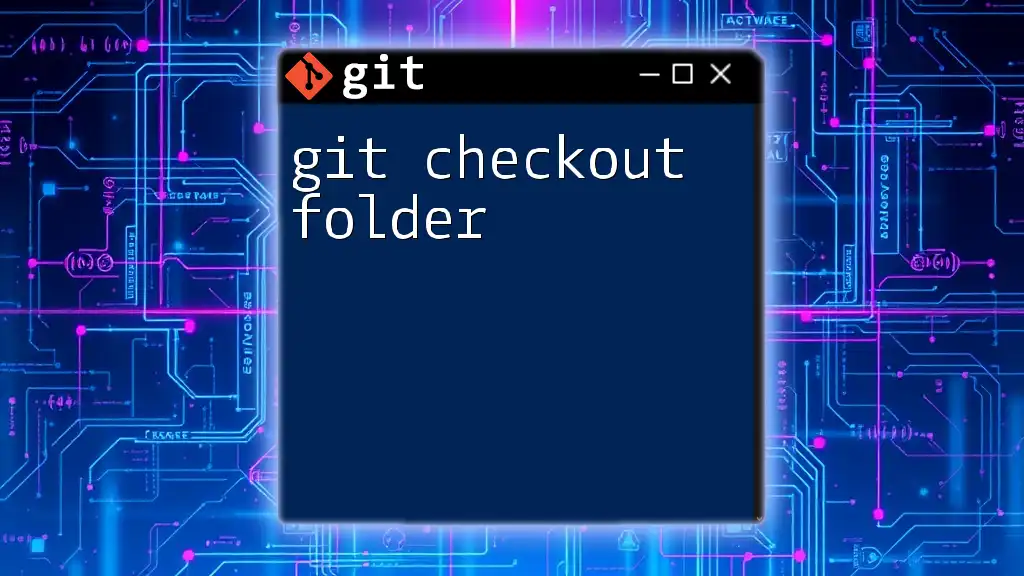
Conclusion
The `git checkout single file` command is a vital tool in the Git ecosystem, empowering developers to manage their files and version effectively. Understanding its usage not only enhances your version control skills but also safeguards against data loss. Always practice caution with this command to ensure you are restoring the right version while preserving your important changes. For those eager to learn more, increasing your Git knowledge can significantly enhance your development workflow.