To check out a specific file from another branch in Git, use the following command:
git checkout <branch-name> -- <path/to/your/file>
Understanding Git Branches
What is a Git Branch?
In Git, a branch is essentially a pointer to a specific commit in the repository's history. Branches allow developers to work on different features or fixes simultaneously without affecting the main codebase, typically known as the `main` or `master` branch. The isolated environment provided by branches is crucial for maintaining code stability while facilitating collaboration among teams.
Typical Use Cases for Switching Files Between Branches
There are several scenarios where you might need to checkout files from another branch:
- When you need a bug fix from a different branch without merging the entire branch into your current one.
- If you want to review changes made by others without switching branches.
- To retrieve a configuration file or any resource file that has been modified in another branch.
By checking out only the necessary files, you can keep your working directory clean and avoid unnecessary complexity in merging.
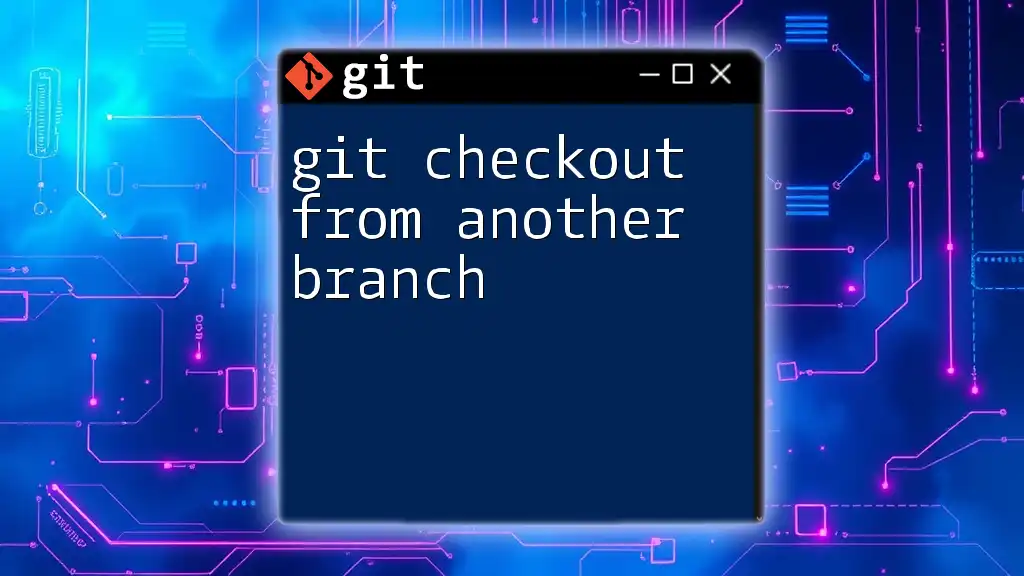
Pre-requisites
Basic Knowledge of Git Commands
Before you dive into using the `git checkout` command to checkout files from another branch, it’s important to be familiar with some basic Git commands. Knowing how to check your repository’s status, view the history, and manage branches will make the process smoother.
Setting Up Your Repository
If you’re new to Git and want to practice, start by cloning a sample repository. You can use the following command:
git clone https://github.com/your-repo/sample-repo.git
Once you have your repository set up, you can create and switch branches using:
git checkout -b new-branch
This will create and switch to a new branch, preparing you for further operations.
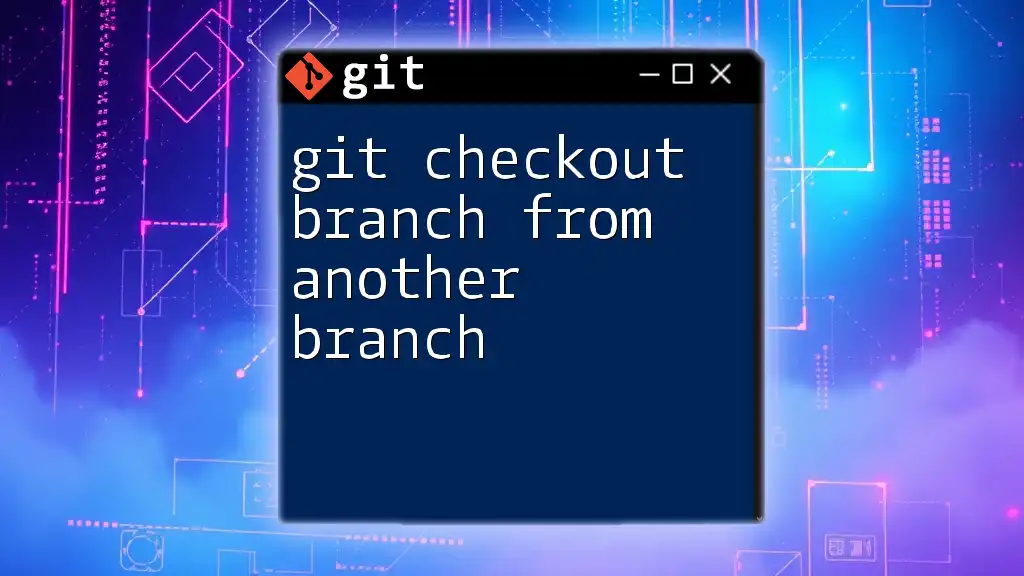
The `git checkout` Command
What is the `git checkout` Command?
The `git checkout` command serves various purposes, including switching branches, restoring working tree files, and even creating new branches. For our focus, we are interested in how it allows us to retrieve a specific file from another branch without merging the changes.
Syntax of the `git checkout` Command
Here is the syntax for using `git checkout` to retrieve a file from a different branch:
git checkout [branch-name] -- [file-path]
Each part of the command has its significance:
- `[branch-name]`: The name of the branch from which you want to retrieve the file.
- `[file-path]`: The relative path to the file you want to checkout.
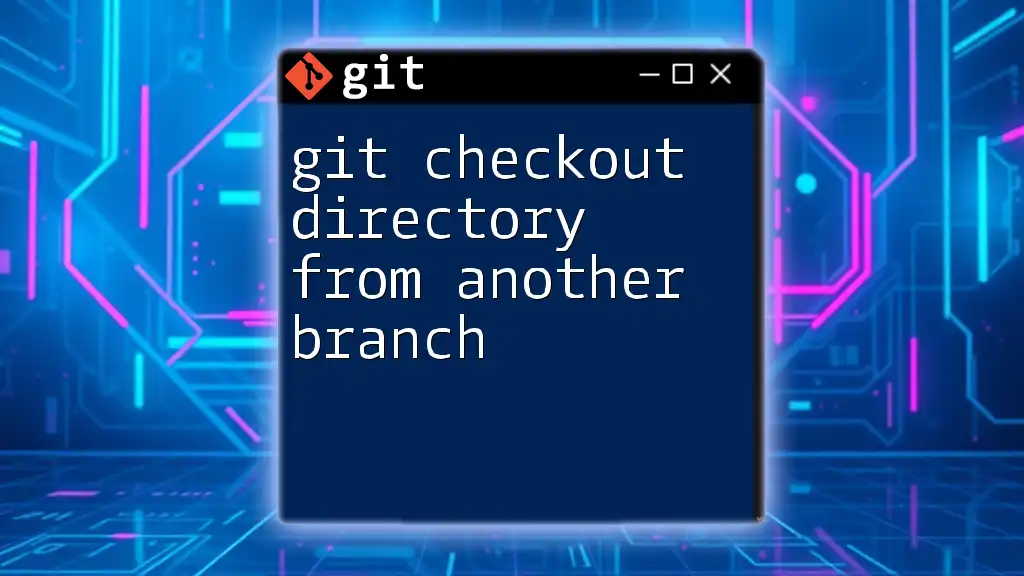
Checking Out a File from Another Branch
Step-by-Step Instructions
- Identify the Branch and File You Want to Checkout Before running the checkout command, identify the target branch and the specific file you want to retrieve. You can do this using Git commands:
git branch
git ls-files
These commands help you list all branches and see the files tracked in the repository.
- Using the `git checkout` Command With the target branch name and file path identified, you can now use the command to checkout the file. For example:
git checkout feature-branch -- path/to/your/file.txt
By executing this command, you retrieve the specified file from `feature-branch`.
- Verifying the Changes After checking out the file, it's essential to verify if the operation was successful. Use the following commands:
git status
git diff
The `git status` command will show you if there are changes in the working directory. Meanwhile, `git diff` will allow you to see the differences between the current version and the last committed version of the file.
Example Scenario
Imagine a situation where you need to retrieve a configuration file named `config.yaml` from a branch called `development`. Here’s how you would do it:
git checkout development -- config.yaml
After executing this command, you can run `git status` to see if `config.yaml` is in the modified state, indicating that the file was successfully checked out from the `development` branch.
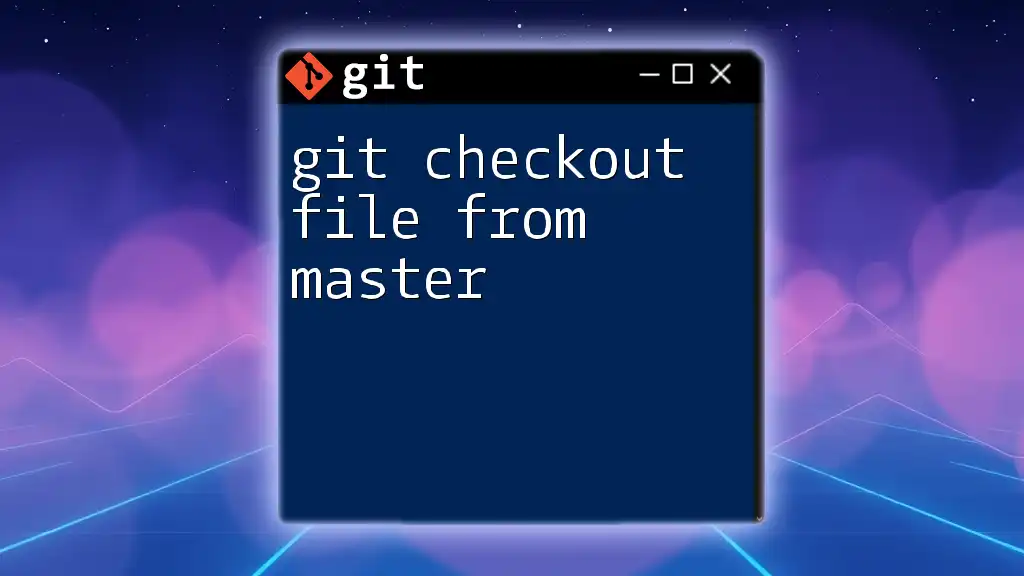
Handling Common Issues
Conflicts During Checkout
In some cases, you may encounter conflicts if the file you are trying to checkout has been modified in your current branch. If this happens, Git will prevent you from proceeding with the checkout operation.
To resolve this, you can choose to keep your changes or take the version from the branch you're checking out from using:
git checkout --ours path/to/file # Keep the current branch's version
git checkout --theirs path/to/file # Take the incoming branch's version
Accidental Changes
If you accidentally checkout the wrong file, you can revert the changes using:
git checkout HEAD -- path/to/file
This command will restore the file to its last committed state, undoing your checkout.
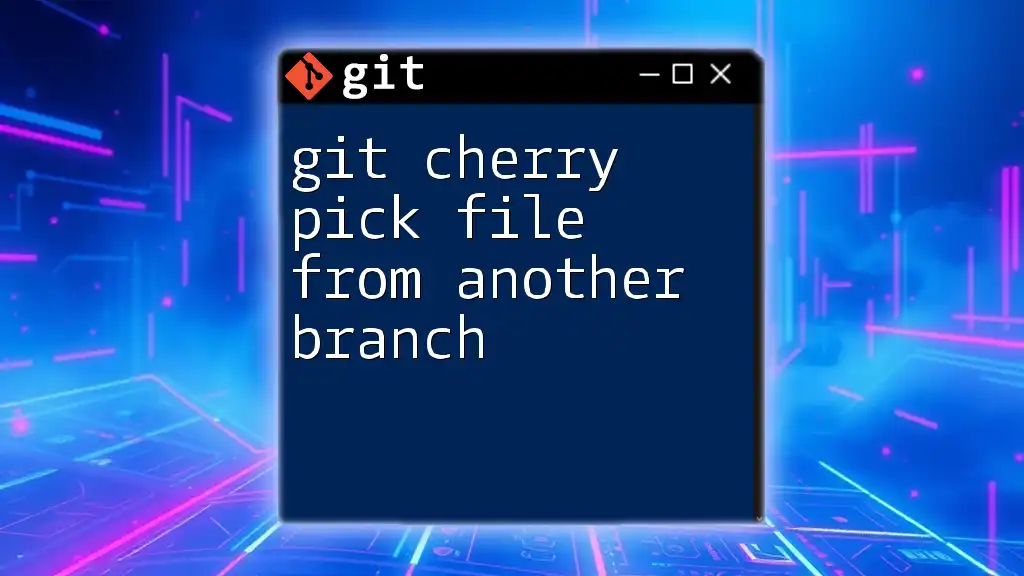
Best Practices
Before Checking Out Files
Always ensure that you have committed or stashed any existing changes in your working directory before checking out files. This precaution prevents potential data loss and keeps your working environment stable.
Regularly Pulling Updates
It's good practice to regularly sync with the remote repository. Use the `git pull` command to ensure you have the latest code updates from your collaborative partners. This motion minimizes the chances of encountering conflicts when checking out files.
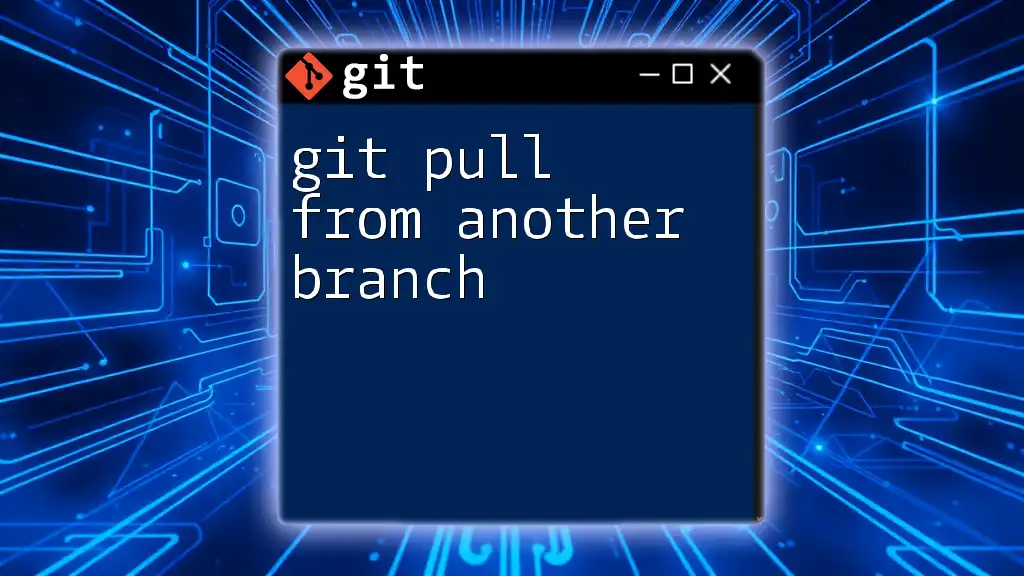
Conclusion
Understanding how to use the "git checkout file from another branch" functionality is essential for efficient version control. It allows for flexibility and ease in managing changes across varying branches, keeping your workflow seamless and organized.
Practice using the commands provided in this guide to become more comfortable with checking out files, and remember to stay updated on best practices for effective collaboration and code management.
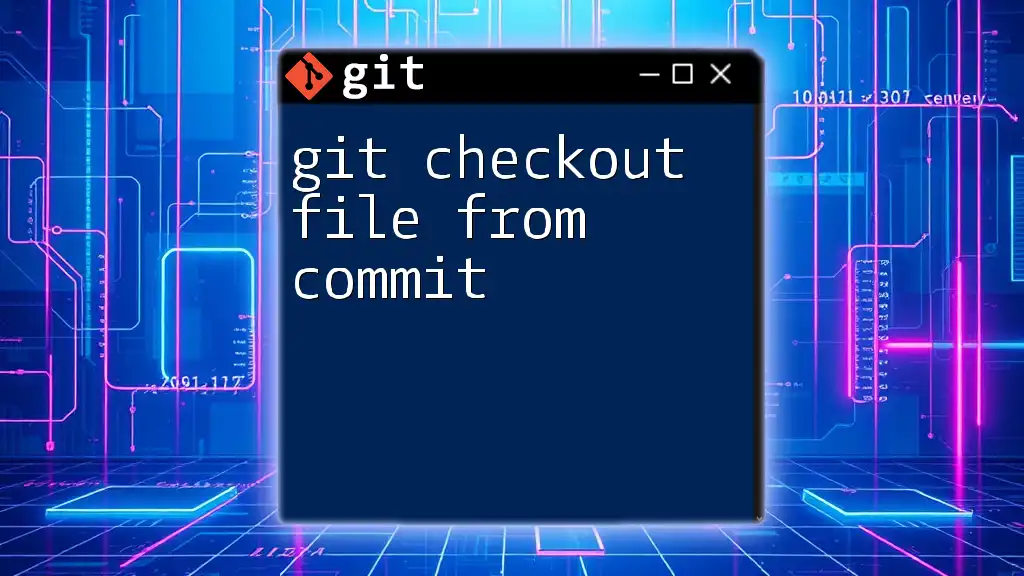
Additional Resources
For those eager to expand their knowledge, consider exploring the official Git documentation or reference materials that cover advanced Git features and tips.
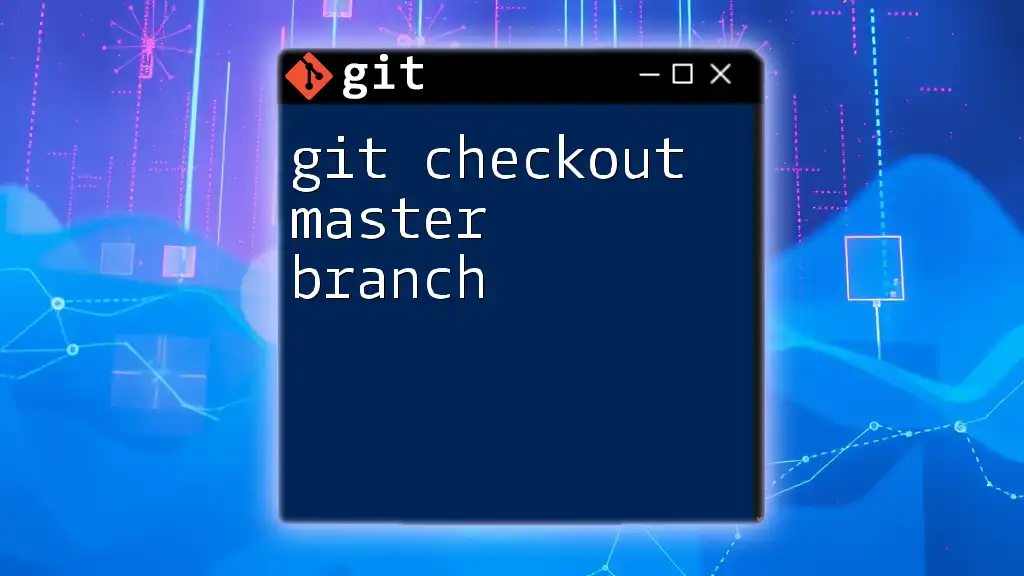
Call to Action
Stay connected for more tutorials and the latest tips on using Git effectively in your projects. Sign up for our newsletter and follow us on social media for updates!