The `git checkout master` command is used to switch your current working branch to the master branch in a Git repository.
git checkout master
What is the Master Branch?
The master branch (now often referred to as the main branch in newer conventions) serves as the default branch in a Git repository. It represents the primary line of development, where stable and production-ready code resides. Historically, the master branch has symbolized a reliable state for software projects, ensuring that any changes are thoroughly tested and reviewed before integration.
Understanding the significance of the master branch is crucial for effective collaboration in software development. It acts as the central hub where all developers can converge, allowing for seamless integration of diverse feature branches. By keeping the master branch clean and functional, teams can avoid potential conflicts and maintain a smooth workflow.
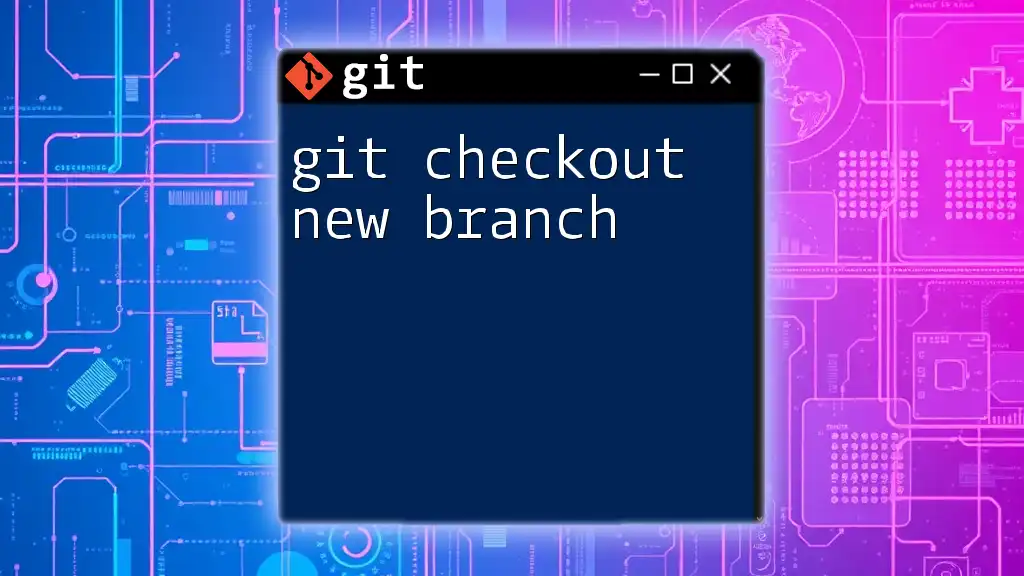
Getting Started with Git
Before diving into the specifics of the git checkout master branch command, it is essential to ensure that Git is properly installed and set up on your machine.
Prerequisites for Using Git
To start using Git effectively, you must first install it. Depending on your operating system, the installation process may vary. Visit the official [Git website](https://git-scm.com/) for detailed instructions.
Once you have Git installed, familiarize yourself with several basic commands:
-
Initialize a new repository:
git init
-
Clone an existing repository: This is often your starting point if you want to work on another project's code. Use the following command:
git clone https://github.com/username/repository.git
Cloning not only gives you a local copy of the repository but also sets up a connection to the original remote repository.
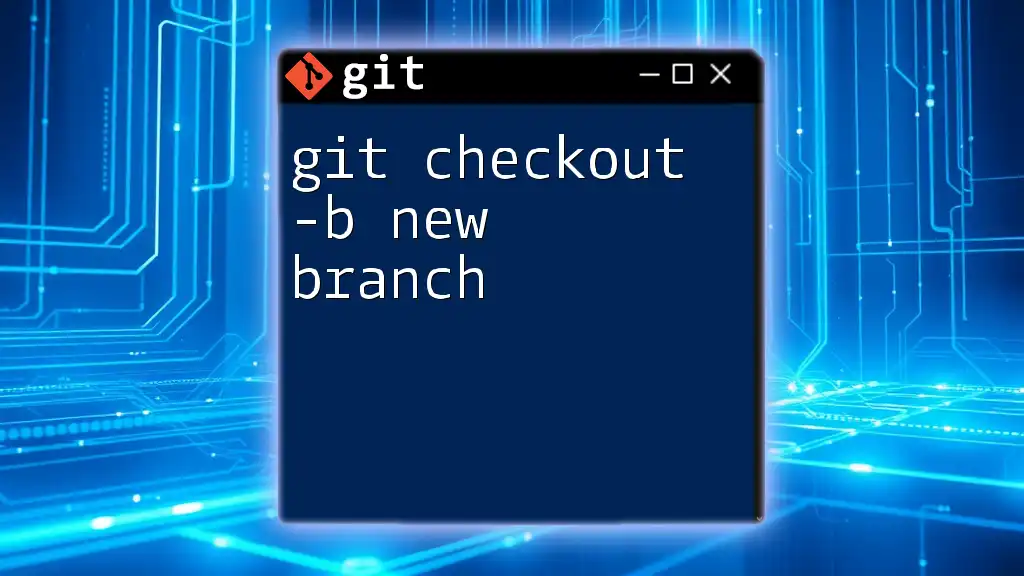
Checking Out the Master Branch
What Does 'Checkout' Mean?
The checkout command is fundamental to navigating and working with branches in Git. When you execute this command, you are quite literally "checking out" a specific branch from your repository, making it the active working branch.
While the primary use of the checkout command is to switch between branches, it can also be used to revert files back to their state in a previous commit, thus indicating its versatility.
How to Checkout the Master Branch
To switch to the master branch, you can simply run the following command:
git checkout master
Executing this command changes your working directory to reflect the state of the master branch. If you've set up your branch correctly, your environment will now mirror the current state of the code in the master branch, letting you start working on it or make necessary updates.
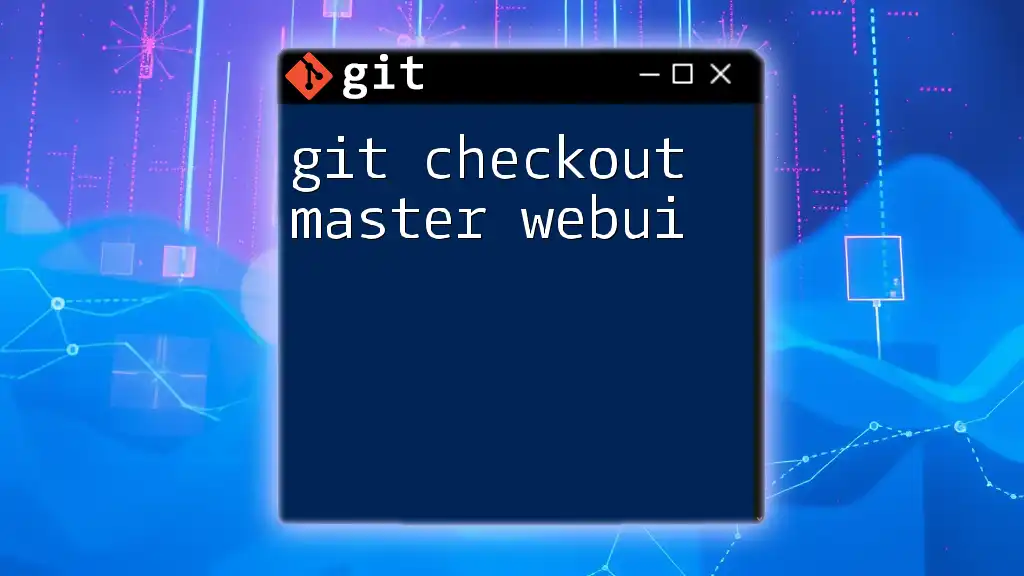
Common Scenarios Involving Checkout Master
Switching from Another Branch
Imagine you're working on a feature branch called `feature-x` and you need to return to the master branch to address some critical bug. The typical sequence would look like this:
git checkout feature-x
# make some changes...
git checkout master
In this process, you first switch back to the feature branch to complete your modifications before transitioning to the master branch. Each checkout updates your working directory to match the content of the selected branch.
Updating the Master Branch from the Remote Repository
Keeping your local master branch synchronized with the remote repository is vital. To fetch and apply updates, follow these steps:
git fetch origin
git checkout master
git pull origin master
- `git fetch origin` retrieves the latest changes from the remote repository but doesn’t merge them into your current working branch. It’s a safeguard, ensuring you can review updates before they affect your local files.
- `git checkout master` switches to your local master branch.
- `git pull origin master` merges the fetched changes into your local master branch, updating it with any new commits that have been made on the remote.
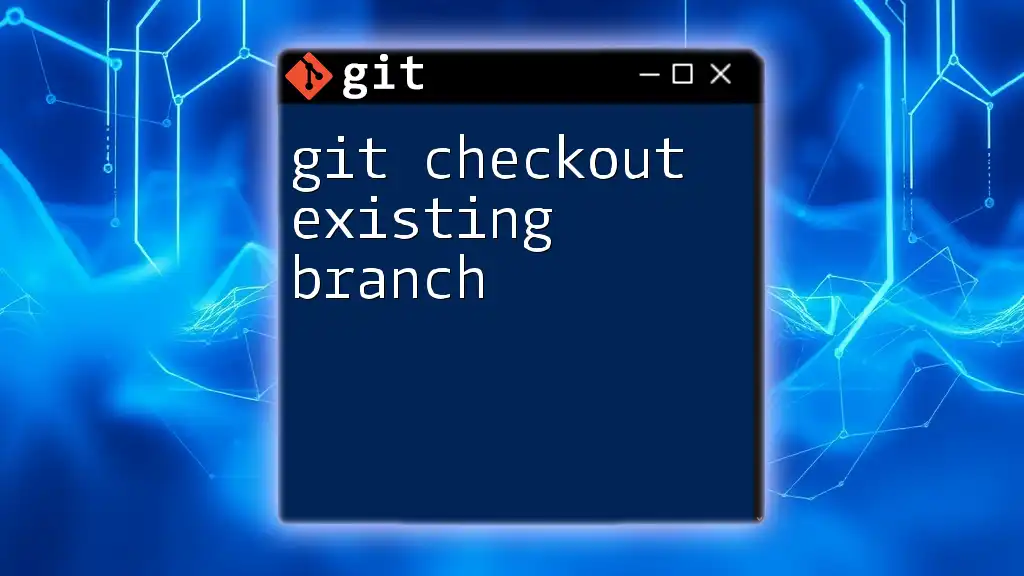
Best Practices for Working with the Master Branch
Keeping the Master Branch Clean
Maintaining a clean master branch is crucial for stabilizing your project. Here are a few essential practices:
- Commit Messages: Utilize clear and concise messages for your commits. This helps team members understand the history and purpose of changes.
- Thorough Reviews: Before merging into the master branch, ensure that your code has passed through a rigorous review or approval process. This reduces the likelihood of introducing bugs into production.
Using Feature Branches Effectively
Utilizing feature branches—temporary branches created for developing specific features or fixes—enhances collaboration. Instead of working directly on the master branch, create a new branch for every feature:
git checkout -b feature/some-feature
This process isolates your changes, letting you test and refine features without jeopardizing the integrity of the master branch.
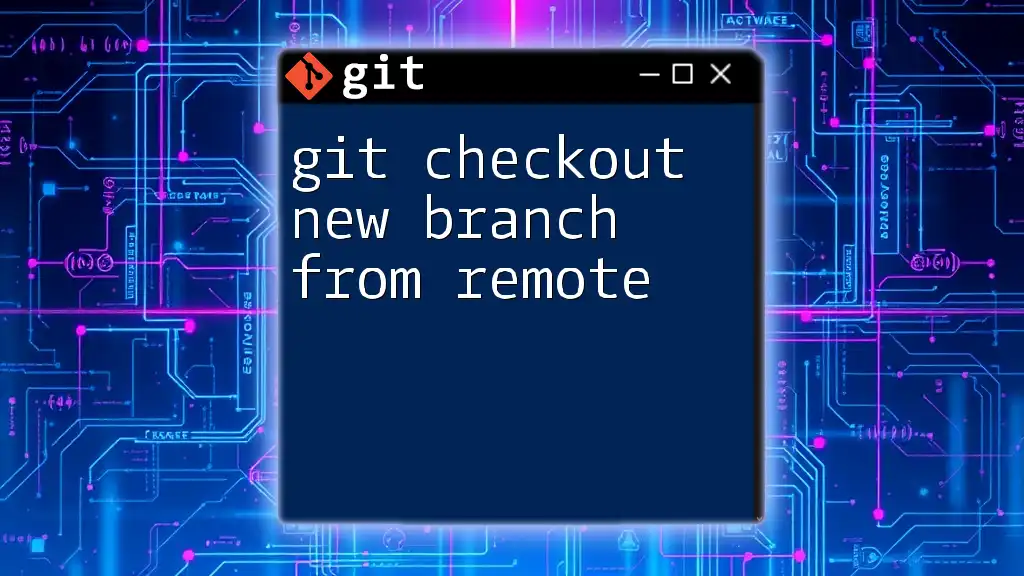
Troubleshooting Common Issues
While working with Git, you might encounter errors when trying to checkout the master branch. One common issue is having local changes in your working directory that would be overwritten by the checkout.
For instance, if you see an error like:
error: Your local changes to the following files would be overwritten by checkout:
You can resolve this by stashing your changes temporarily:
git stash
git checkout master
This command saves your changes without committing them, allowing you to switch branches without conflict. After you checkout to master, you can pop the stash to reapply your changes when you're ready.
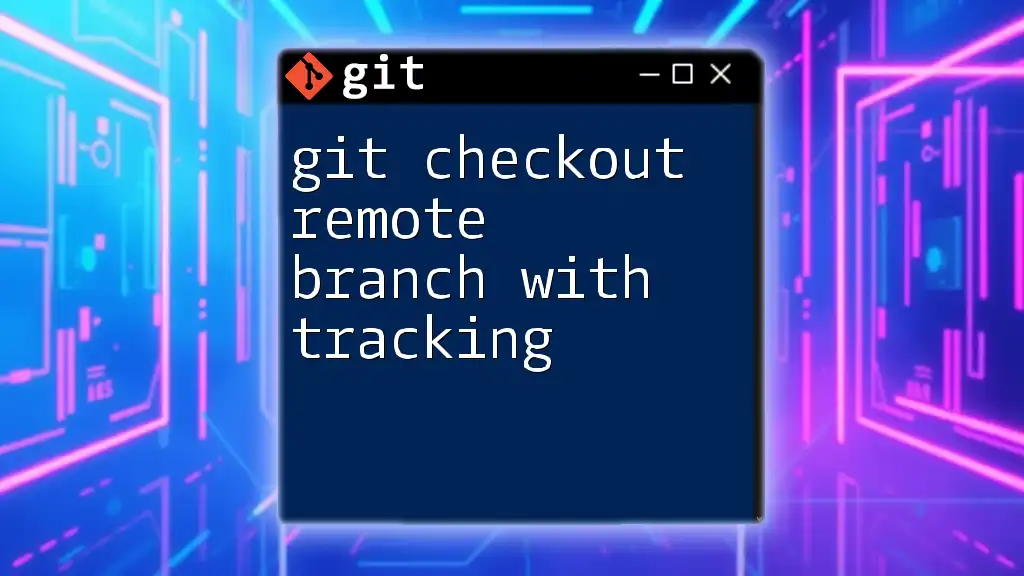
Conclusion
The git checkout master branch command is a fundamental aspect of version control in Git. By mastering this command, along with a solid understanding of the broader mechanics of branching, you can significantly enhance your productivity as a developer.
Take the time to practice your Git skills in various scenarios, and soon you'll feel confident in managing branches and maintaining a clean workflow. The key to success lies in understanding the functionality behind commands and applying best practices to your development process.
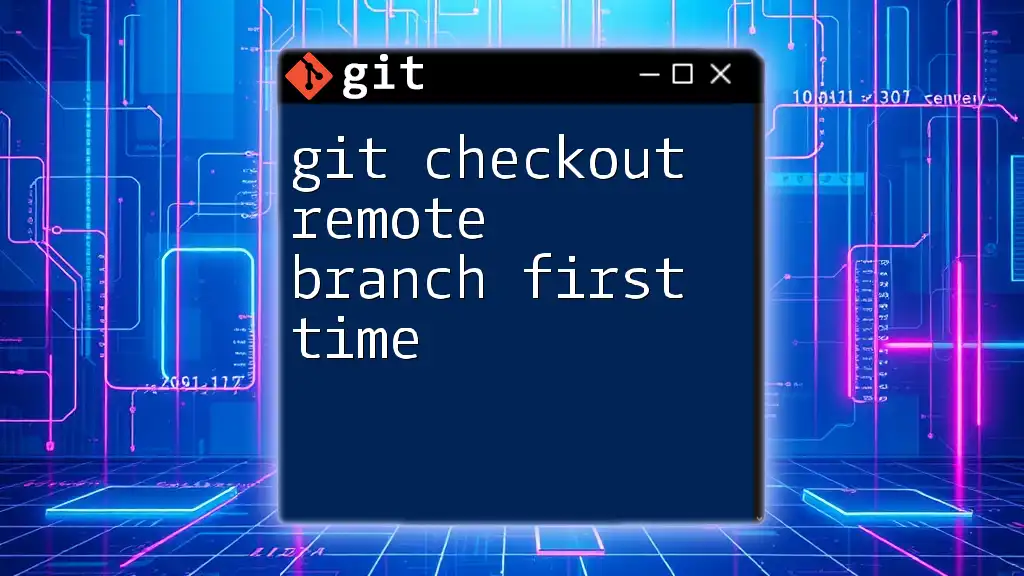
Call to Action
We invite you to explore more tips and in-depth tutorials on using Git effectively. Check out our courses to expand your knowledge of Git commands and elevate your coding skills!